1.启动项目,为项目添加gameplayability插件

2.添加abilitysystemcomponent的c++类
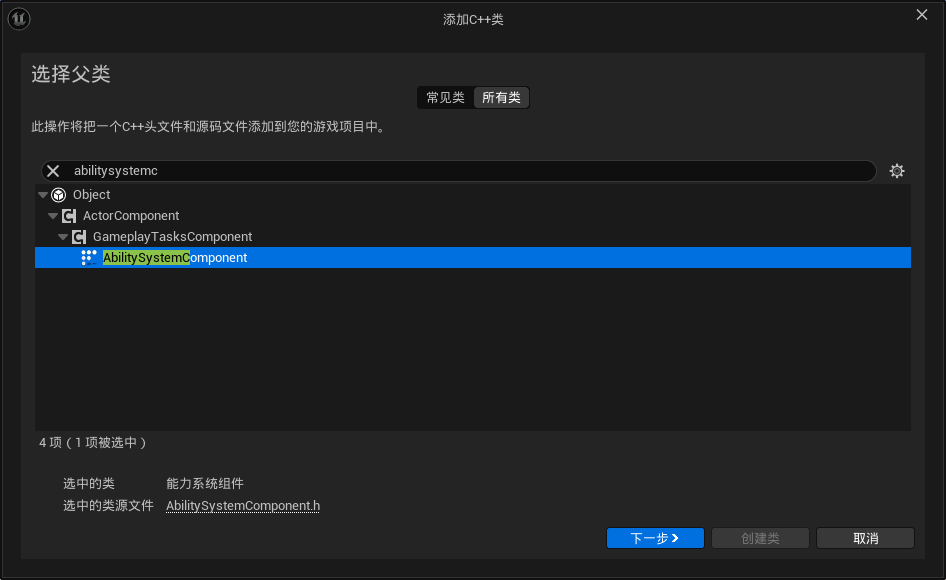
3.添加attributeset的c++类
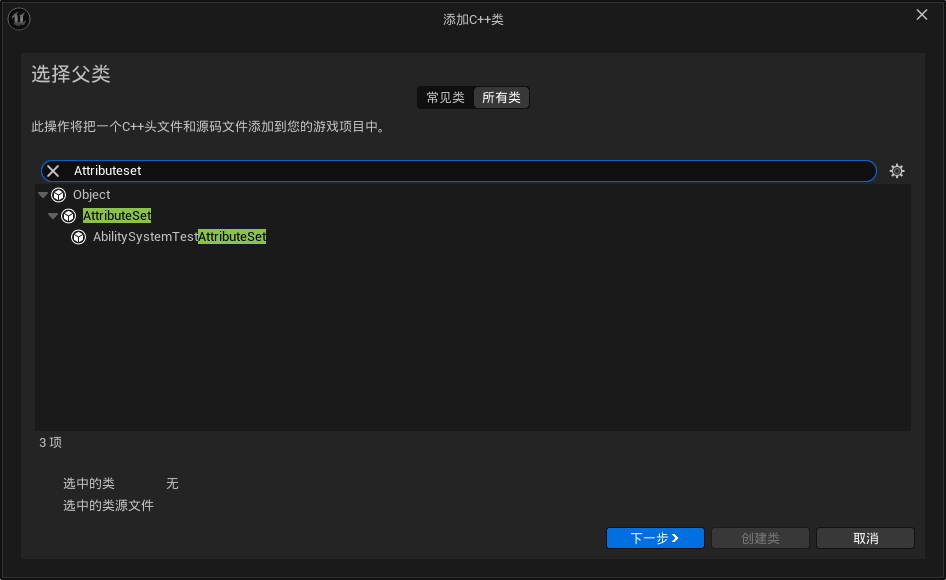
4.往build.cs内添加模块
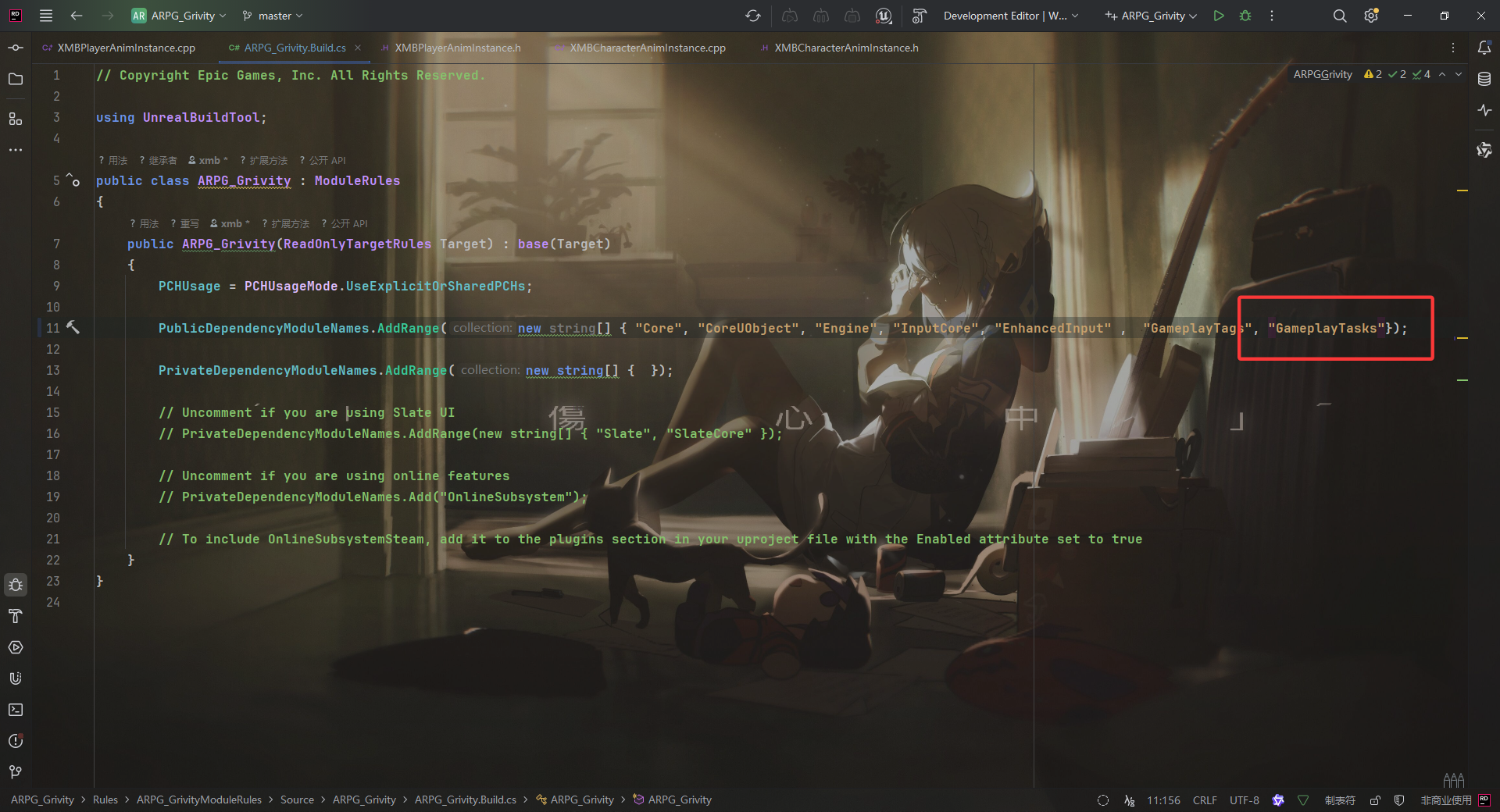
5.进入CharacterBase内,添加gameplayasystem和attributbeset,覆写PossessedBy()和GetAbilitysystemcomponent()
class ARPG_GRIVITY_API ACharacterBase : public ACharacter, public IAbilitySystemInterface
{
GENERATED_BODY()
public:
ACharacterBase();
//创建能力组件的获取方法
UXMBAbilitySystemComponent* GetXMBAbilitySystemComponent() const {return XMBAbilitySystemComponent;}
//创建属性集的获取方法
UXMBAttributeSet* GetXMBAttributeSet() const {return XMBAttributeSet;}
//Begin IAbilitySystemInterface Interface
virtual UAbilitySystemComponent* GetAbilitySystemComponent() const override;
//End IAbilitySystemInterface Interface
protected:
//创建能力组件
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = "AbilitySystem")
UXMBAbilitySystemComponent* XMBAbilitySystemComponent;
//创建属性集
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = "AbilitySystem")
UXMBAttributeSet* XMBAttributeSet;
//Begin Apawn Interface
virtual void PossessedBy(AController* NewController) override;
//End Apawn Interface
};
6.在构造函数里创建,覆写函数体内
#include "Character/CharacterBase.h"
#include "AbilitySystem/XMBAttributeSet.h"
#include "AbilitySystem/XMBAbilitySystemComponent.h"
ACharacterBase::ACharacterBase()
{
PrimaryActorTick.bCanEverTick = false;
//初始禁用 Tick,适合需要手动控制更新的场景。
PrimaryActorTick.bStartWithTickEnabled = false;
//禁用贴花接收,适用于性能优化或特定视觉需求。
GetMesh()->bReceivesDecals = false;
XMBAbilitySystemComponent = CreateDefaultSubobject<UXMBAbilitySystemComponent>(TEXT("XMBAbilitySystemComponent"));
XMBAttributeSet = CreateDefaultSubobject<UXMBAttributeSet>(TEXT("XMBAttributeSet"));
}
UAbilitySystemComponent* ACharacterBase::GetAbilitySystemComponent() const
{
return GetXMBAbilitySystemComponent();
}
void ACharacterBase::PossessedBy(AController* NewController)
{
Super::PossessedBy(NewController);
if (XMBAbilitySystemComponent)
{
XMBAbilitySystemComponent->InitAbilityActorInfo(this, this);
}
}
7.进入到玩家控制的角色内(XMBCharacter),对PossessedBy覆写
//Begin Apawn Interface
virtual void PossessedBy(AController* NewController) override;
//End Apawn Interface
void AXMBCharacter::PossessedBy(AController* NewController)
{
Super::PossessedBy(NewController);
if (XMBAbilitySystemComponent && XMBAttributeSet)
{
GEngine->AddOnScreenDebugMessage(-1,5.f, FColor::Red, FString::Printf(TEXT("Owner: %s, Avatar : %s"),*XMBAbilitySystemComponent->GetOwnerActor()->GetActorLabel(),*XMBAbilitySystemComponent->GetAvatarActor()->GetActorLabel()));
}
}
8.启动,能从日志里看到信息
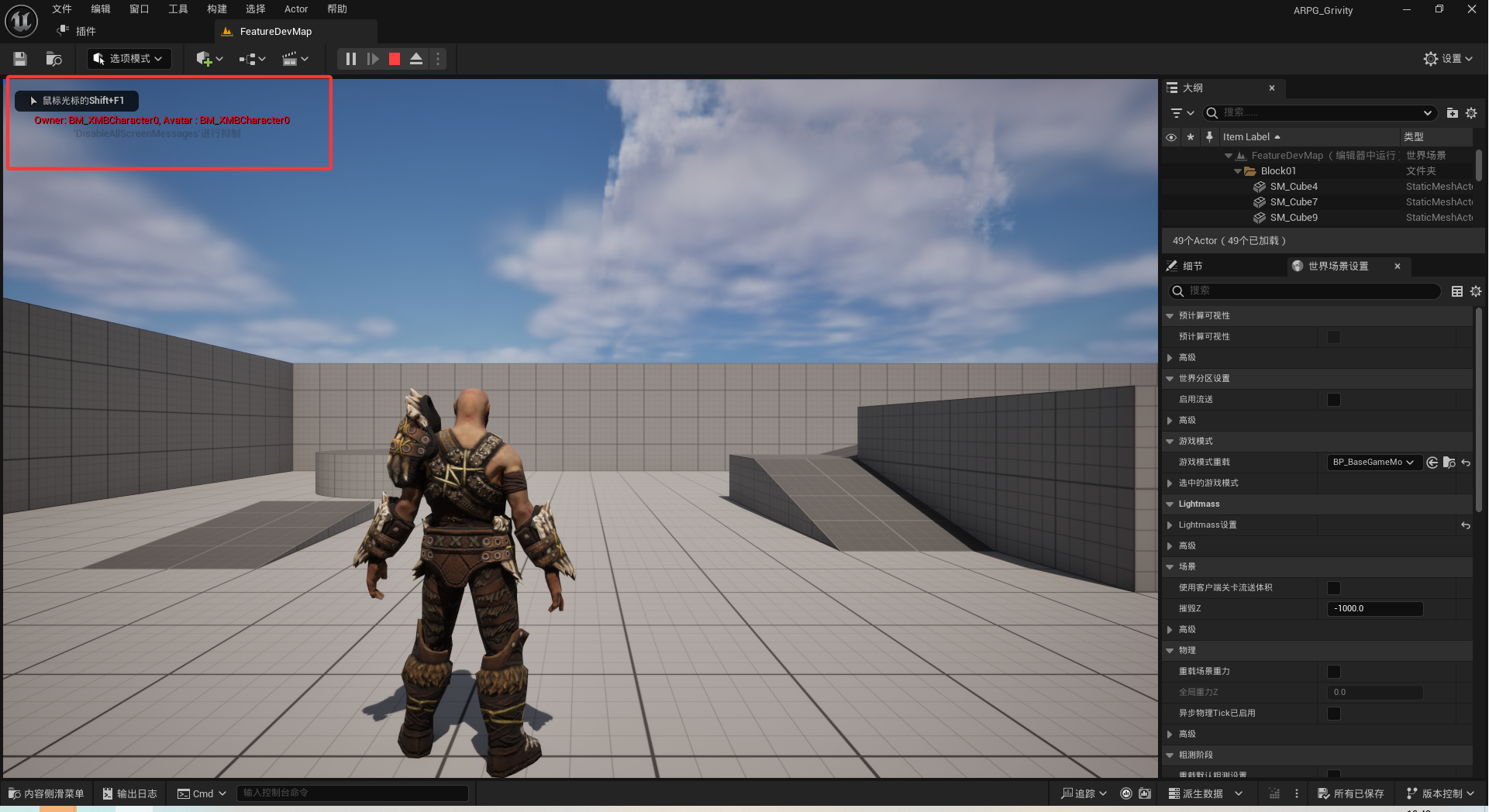
9.我们需要给AbilitySystemComponent赋予Ability,当玩家尝试激活Ability时,实际上是从ASC中激活能力。对于激活的这一部分,我们可以自定义Ability的激活方式。
继续创建一个GameplayAbility的c++类,然后在其内创建一个激活策略的枚举,覆写两个函数
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "Abilities/GameplayAbility.h"
#include "XMBGameplayAbility.generated.h"
//创建一个激活能力的策略枚举
UENUM()
enum class EXMBAbilityActivationPolicy : uint8
{
OnTriggered,
OnGiven
};
/**
*
*/
UCLASS()
class ARPG_GRIVITY_API UXMBGameplayAbility : public UGameplayAbility
{
GENERATED_BODY()
public:
protected:
//设置激活策略
UPROPERTY(EditDefaultsOnly, Category = "XMBAbility")
EXMBAbilityActivationPolicy AbilityActivationPolicy = EXMBAbilityActivationPolicy::OnTriggered;
//~ Begin UGameplayAbility Interface
virtual void OnGiveAbility(const FGameplayAbilityActorInfo* ActorInfo, const FGameplayAbilitySpec& Spec) override;
virtual void EndAbility(const FGameplayAbilitySpecHandle Handle, const FGameplayAbilityActorInfo* ActorInfo, const FGameplayAbilityActivationInfo ActivationInfo, bool bReplicateEndAbility, bool bWasCancelled) override;
//~ End UGameplayAbility Interface
};
// Fill out your copyright notice in the Description page of Project Settings.
#include "AbilitySystem/Abilities/XMBGameplayAbility.h"
#include "AbilitySystem/XMBAbilitySystemComponent.h"
//在Ability被分配给AbilitySystemComponent后立即调用
void UXMBGameplayAbility::OnGiveAbility(const FGameplayAbilityActorInfo* ActorInfo, const FGameplayAbilitySpec& Spec)
{
Super::OnGiveAbility(ActorInfo, Spec);
if (AbilityActivationPolicy == EXMBAbilityActivationPolicy::OnGiven)
{
if (ActorInfo && !Spec.IsActive())
{
//Spec.Handle是FGameplayAbilitySpec对象的句柄,表示一个具体的能力实例
ActorInfo->AbilitySystemComponent->TryActivateAbility(Spec.Handle);
}
}
}
void UXMBGameplayAbility::EndAbility(const FGameplayAbilitySpecHandle Handle,
const FGameplayAbilityActorInfo* ActorInfo, const FGameplayAbilityActivationInfo ActivationInfo,
bool bReplicateEndAbility, bool bWasCancelled)
{
Super::EndAbility(Handle, ActorInfo, ActivationInfo, bReplicateEndAbility, bWasCancelled);
if (AbilityActivationPolicy == EXMBAbilityActivationPolicy::OnGiven)
{
if (ActorInfo)
{
//Handle是FGameplayAbilitySpec对象的句柄,表示要清除的能力实例。
ActorInfo->AbilitySystemComponent->ClearAbility(Handle);
}
}
}
10.启动引擎,右键即可创建一个刚才自己创建的gameplayability类,
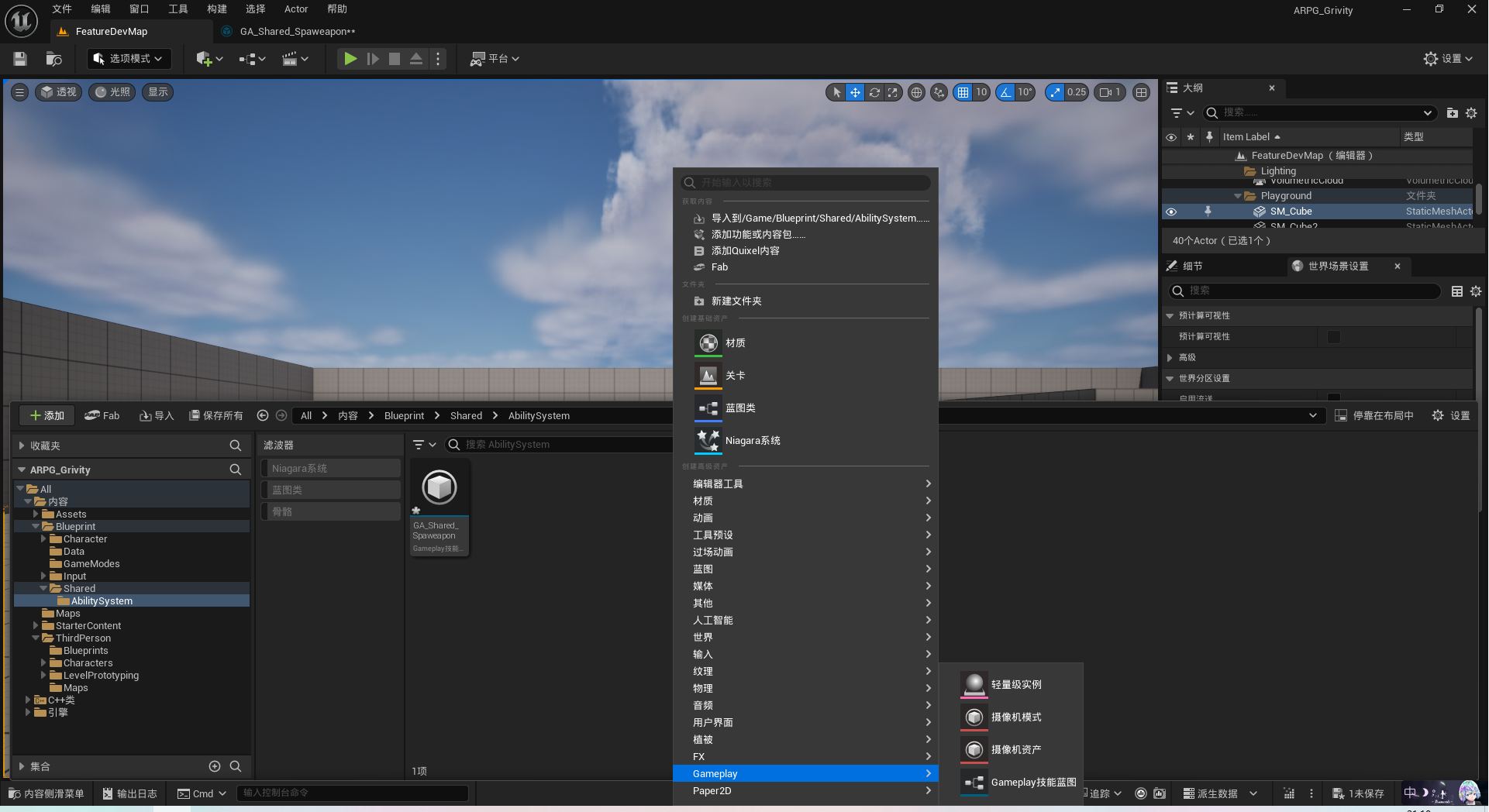