本文通过对Android蓝牙协议栈Bluedroid init_stack_internal
函数进行深度剖析,全面揭示了蓝牙协议栈的初始化机制。通过分析MessageLoopThread
、btif_init_bluetooth
等核心组件,展示了Bluedroid如何实现线程管理、跨层通信和实时调度。
一、概述
Bluedroid作为Android系统的默认蓝牙协议栈,其初始化过程是一个复杂而精密的系统工程。本文以init_stack_internal
函数为切入点,深入分析了蓝牙协议栈的启动流程:
-
分层架构:展示了从操作系统抽象层到硬件适配层,再到协议栈核心层的完整初始化顺序
-
线程模型 :详细解析了
MessageLoopThread
的实现,包括线程创建、消息循环和实时调度 -
跨层通信:探讨JNI桥接层和HAL回调机制的设计原理
-
模块化管理 :介绍
INTEROP_MODULE
和STACK_CONFIG_MODULE
等高级功能模块的作用
在初始化过程中,各模块之间存在严格的顺序约束。例如,硬件抽象层(GD_SHIM)必须在协议栈核心(BTIF)之前启动,以确保硬件特性被正确识别;而操作系统抽象层(OSI)则需优先于硬件抽象层,提供线程、锁等底层支持。这种强顺序约束保证了协议栈的稳定性和可靠性。
二、源码刨析
init_stack_internal
cpp
packages/modules/Bluetooth/system/btif/src/stack_manager.cc
static bluetooth::core::CoreInterface* interfaceToProfiles;
static void init_stack_internal(bluetooth::core::CoreInterface* interface) {
// 接口绑定与基础环境准备
// all callbacks out of libbluetooth-core happen via this interface
interfaceToProfiles = interface; // 保存跨层回调接口,用于协议事件上报(如连接状态、数据接收)
module_management_start(); // 启动模块管理系统(动态加载/卸载)
main_thread_start_up(); // 初始化主线程环境(消息队列、事件循环)
// 基础支撑层
module_init(get_local_module(DEVICE_IOT_CONFIG_MODULE)); // 设备物联网配置(IoT特性开关)
module_init(get_local_module(OSI_MODULE)); // 操作系统抽象层(线程/锁/定时器)
// 硬件交互层
module_start_up(get_local_module(GD_SHIM_MODULE)); // 硬件抽象层启动(关键路径)
// 协议栈核心层
module_init(get_local_module(BTIF_CONFIG_MODULE));
btif_init_bluetooth(); // BTIF核心初始化
// 功能增强层
module_init(get_local_module(INTEROP_MODULE)); // 互操作性配置(修复特定设备兼容问题)
module_init(get_local_module(STACK_CONFIG_MODULE)); // 协议栈运行时配置(功耗策略、调试开关)
// 同步机制
// stack init is synchronous, so no waiting necessary here
stack_is_initialized = true; // 通知上层可安全使用蓝牙功能
}
初始化蓝牙协议栈的内部组件。通过一系列模块调用构建了蓝牙系统的基础架构,采用同步初始化模式(无需等待回调)。
OSI_MODULE
必须优先于GD_SHIM_MODULE
,提供线程/锁等底层支持。
GD_SHIM_MODULE
作为硬件交互层,需在协议栈配置(BTIF_CONFIG
)前启动,确保硬件特性被正确识别。
INTEROP_MODULE
加载设备黑名单/白名单,解决已知设备配对问题。
STACK_CONFIG_MODULE
动态调整协议栈行为(如BLE扫描间隔、连接参数)。
执行流程:
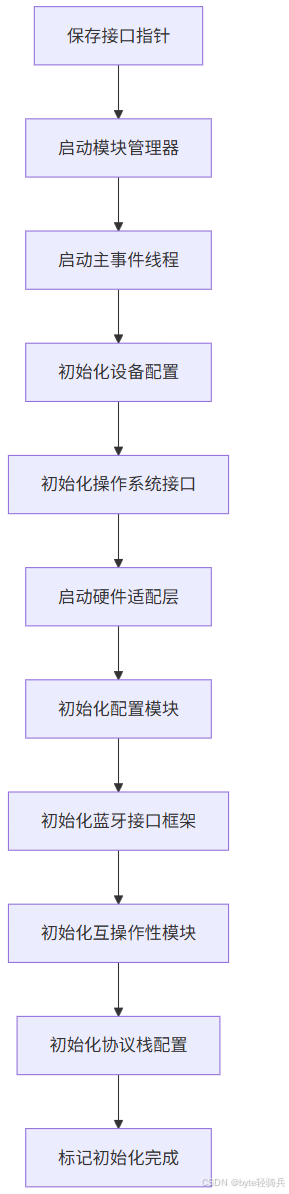
分层架构:
cpp
┌───────────────────────┐
│ 应用层 │
├───────────────────────┤
│ 接口框架层(BTIF) │
├───────────────────────┤
│ 协议栈核心层 │
├───────────────────────┤
│ 硬件适配层(GD_SHIM) │
├───────────────────────┤
│ 操作系统抽象层(OSI) │
└───────────────────────┘
初始化顺序设计原则
|--------|---------------------|--------------------|-----------|
| 阶段 | 模块 | 依赖关系 | 必要性级别 |
| 1 | OSI_MODULE | 无 | 必须 |
| 2 | GD_SHIM_MODULE | OSI_MODULE | 必须 |
| 3 | BTIF_CONFIG_MODULE | GD_SHIM_MODULE | 必须 |
| 4 | INTEROP_MODULE | BTIF_CONFIG_MODULE | 可选 |
| 5 | STACK_CONFIG_MODULE | BTIF_CONFIG_MODULE | 可选 |
-
强顺序约束:硬件抽象层(GD_SHIM)必须在协议栈核心(BTIF)之前启动
-
弱顺序约束:互操作性模块可延迟加载(按需启用特定设备兼容规则)
典型执行路径耗时(参考值)
cpp
[Thread-Main]
module_management_start() [0.2ms]
main_thread_start_up() [1.8ms]
DEVICE_IOT_CONFIG_MODULE init [0.5ms]
OSI_MODULE init [3.1ms]
GD_SHIM_MODULE start [15.6ms] // 含硬件自检
BTIF_CONFIG_MODULE init [0.9ms]
btif_init_bluetooth() [8.2ms] // 创建20+内部通道
INTEROP_MODULE init [2.3ms] // 加载1000+设备规则
STACK_CONFIG_MODULE init [0.7ms]
Total: ~33ms
①module_management_start
cpp
packages/modules/Bluetooth/system/btcore/src/module.cc
void module_management_start(void) {}
②main_thread_start_up
cpp
packages/modules/Bluetooth/system/gd/common/i_postable_context.h
// 1.核心接口设计(IPostableContext):定义跨线程任务提交的统一接口
namespace bluetooth {
namespace common {
class IPostableContext {
public:
virtual ~IPostableContext(){};
virtual void Post(base::OnceClosure closure) = 0; //通过纯虚函数实现行为抽象
};
} // namespace common
} // namespace bluetooth
packages/modules/Bluetooth/system/common/message_loop_thread.h
// 2. 消息循环线程实现(MessageLoopThread)
namespace bluetooth {
namespace common {
/**
* An interface to various thread related functionality
*/
class MessageLoopThread final : public IPostableContext {
public:
/**
* Create a message loop thread with name. Thread won't be running until
* StartUp is called.
*
* @param thread_name name of this worker thread
*/
explicit MessageLoopThread(const std::string& thread_name);
MessageLoopThread(const MessageLoopThread&) = delete;
MessageLoopThread& operator=(const MessageLoopThread&) = delete;
/**
* Destroys the message loop thread automatically when it goes out of scope
*/
~MessageLoopThread();
/**
* Start the underlying thread. Blocks until all thread infrastructure is
* setup. IsRunning() and DoInThread() should return true after this call.
* Blocks until the thread is successfully started.
*
* Repeated call to this method will only start this thread once
*/
void StartUp();
/**
* Post a task to run on this thread
*
* @param from_here location where this task is originated
* @param task task created through base::Bind()
* @return true if task is successfully scheduled, false if task cannot be
* scheduled
*/
bool DoInThread(const base::Location& from_here, base::OnceClosure task);
/**
* Shutdown the current thread as if it is never started. IsRunning() and
* DoInThread() will return false after this call. Blocks until the thread is
* joined and freed. This thread can be re-started again using StartUp()
*
* Repeated call to this method will only stop this thread once
*
* NOTE: Should never be called on the thread itself to avoid deadlock
*/
void ShutDown();
/**
* Get the current thread ID returned by PlatformThread::CurrentId()
*
* On Android platform, this value should be the same as the tid logged by
* logcat, which is returned by gettid(). On other platform, this thread id
* may have different meanings. Therefore, this ID is only good for logging
* and thread comparison purpose
*
* @return this thread's ID
*/
// 线程属性访问
base::PlatformThreadId GetThreadId() const;
/**
* Get this thread's name set in constructor
*
* @return this thread's name set in constructor
*/
std::string GetName() const;
/**
* Get a string representation of this thread
*
* @return a string representation of this thread
*/
std::string ToString() const;
/**
* Check if this thread is running
*
* @return true iff this thread is running and is able to do task
*/
bool IsRunning() const;
/**
* Attempt to make scheduling for this thread real time
*
* @return true on success, false otherwise
*/
bool EnableRealTimeScheduling();
/**
* Return the weak pointer to this object. This can be useful when posting
* delayed tasks to this MessageLoopThread using Timer.
*/
base::WeakPtr<MessageLoopThread> GetWeakPtr();
/**
* Return the message loop for this thread. Accessing raw message loop is not
* recommended as message loop can be freed internally.
*
* @return message loop associated with this thread, nullptr if thread is not
* running
*/
btbase::AbstractMessageLoop* message_loop() const;
/**
* Post a task to run on this thread after a specified delay. If the task
* needs to be cancelable before it's run, use base::CancelableClosure type
* for task closure. For example:
* <code>
* base::CancelableClosure cancelable_task;
* cancelable_task.Reset(base::Bind(...)); // bind the task
* same_thread->DoInThreadDelayed(FROM_HERE,
* cancelable_task.callback(), delay);
* ...
* // Cancel the task closure
* same_thread->DoInThread(FROM_HERE,
* base::Bind(&base::CancelableClosure::Cancel,
* base::Unretained(&cancelable_task)));
* </code>
*
* Warning: base::CancelableClosure objects must be created on, posted to,
* cancelled on, and destroyed on the same thread.
*
* @param from_here location where this task is originated
* @param task task created through base::Bind()
* @param delay delay for the task to be executed
* @return true if task is successfully scheduled, false if task cannot be
* scheduled
*/
bool DoInThreadDelayed(const base::Location& from_here,
base::OnceClosure task,
std::chrono::microseconds delay);
/**
* Wrapper around DoInThread without a location.
*/
void Post(base::OnceClosure closure) override;
template <typename Functor, typename... Args>
auto BindOnce(Functor&& functor, Args&&... args) {
return common::ContextualOnceCallback(
common::BindOnce(std::forward<Functor>(functor),
std::forward<Args>(args)...),
this);
}
template <typename Functor, typename T, typename... Args>
auto BindOnceOn(T* obj, Functor&& functor, Args&&... args) {
return common::ContextualOnceCallback(
common::BindOnce(std::forward<Functor>(functor),
common::Unretained(obj), std::forward<Args>(args)...),
this);
}
template <typename Functor, typename... Args>
auto Bind(Functor&& functor, Args&&... args) {
return common::ContextualCallback(
common::Bind(std::forward<Functor>(functor),
std::forward<Args>(args)...),
this);
}
template <typename Functor, typename T, typename... Args>
auto BindOn(T* obj, Functor&& functor, Args&&... args) {
return common::ContextualCallback(
common::Bind(std::forward<Functor>(functor), common::Unretained(obj),
std::forward<Args>(args)...),
this);
}
private:
/**
* Static method to run the thread
*
* This is used instead of a C++ lambda because of the use of std::shared_ptr
*
* @param context needs to be a pointer to an instance of MessageLoopThread
* @param start_up_promise a std::promise that is used to notify calling
* thread the completion of message loop start-up
*/
static void RunThread(MessageLoopThread* context,
std::promise<void> start_up_promise);
/**
* Actual method to run the thread, blocking until ShutDown() is called
*
* @param start_up_promise a std::promise that is used to notify calling
* thread the completion of message loop start-up
*/
void Run(std::promise<void> start_up_promise);
mutable std::recursive_mutex api_mutex_;
const std::string thread_name_;
btbase::AbstractMessageLoop* message_loop_;
base::RunLoop* run_loop_;
std::thread* thread_;
base::PlatformThreadId thread_id_;
// Linux specific abstractions
pid_t linux_tid_;
base::WeakPtrFactory<MessageLoopThread> weak_ptr_factory_;
bool shutting_down_;
};
inline std::ostream& operator<<(std::ostream& os,
const bluetooth::common::MessageLoopThread& a) {
os << a.ToString();
return os;
}
} // namespace common
} // namespace bluetooth
// 3. 主事件线程初始化(main_thread_start_up)
packages/modules/Bluetooth/system/stack/btu/main_thread.cc
// 线程实例化:使用静态局部变量确保全局唯一实例(单例模式)
static MessageLoopThread main_thread("bt_main_thread");
void main_thread_start_up() {
// 线程启动流程
main_thread.StartUp();
if (!main_thread.IsRunning()) {
log::fatal("unable to start btu message loop thread.");
}
// 实时调度优先级
if (!main_thread.EnableRealTimeScheduling()) {
#if defined(__ANDROID__)
log::fatal("unable to enable real time scheduling");
#else
log::error("unable to enable real time scheduling");
#endif
}
}
通过MessageLoopThread
实现了蓝牙协议栈的主事件循环线程,确保蓝牙核心操作在专用线程上高效执行。通过实时调度和严格的错误检查,保证了蓝牙通信的可靠性和低延迟特性,同时兼顾了跨平台兼容性。
启动步骤:
-
调用
StartUp()
创建原生线程,初始化消息循环。 -
通过
base::RunLoop
阻塞等待线程就绪(内部通过std::promise
同步)。 -
启用实时调度(需
CAP_SYS_NICE
权限,Android需android.permission.REAL_TIME
)。
异步任务模型:
cpp
┌───────────────────────┐ ┌───────────────────────┐
│ 调用线程 │ │ 消息循环线程 │
│ │ │ │
│ DoInThread(closure) ├───►│ message_loop_.Post() │
│ │ │ │
│ │ │ closure.Run() │
└───────────────────────┘ └───────────────────────┘
消息循环架构:
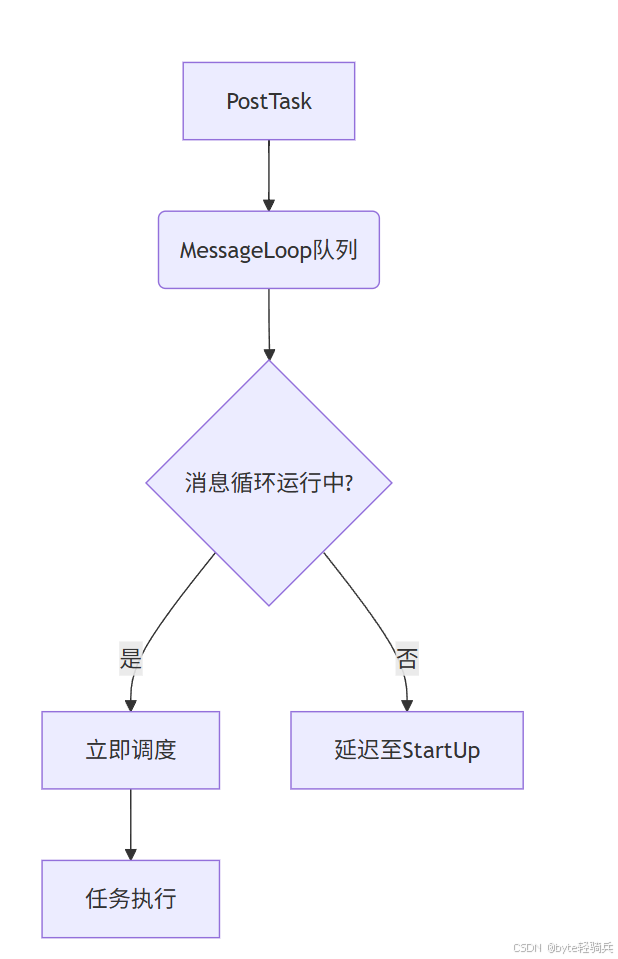
MessageLoopThread::StartUp
cpp
packages/modules/Bluetooth/system/common/message_loop_thread.cc
void MessageLoopThread::StartUp() {
// 1. 同步原语初始化
// 主线程通过future.wait()阻塞等待
// 工作线程通过promise.set_value()通知初始化完成
std::promise<void> start_up_promise;
std::future<void> start_up_future = start_up_promise.get_future();
// 2. 线程启动临界区
{
// 使用recursive_mutex允许同一线程多次加锁(防止重入问题)
std::lock_guard<std::recursive_mutex> api_lock(api_mutex_);
if (thread_ != nullptr) { // 通过检查thread_指针避免重复启动
LOG(WARNING) << __func__ << ": thread " << *this << " is already started";
return;
}
// 线程创建:
//调用std::thread构造函数创建新线程
//静态成员函数RunThread作为入口点
//通过std::move转移promise所有权到新线程
thread_ = new std::thread(&MessageLoopThread::RunThread, this,
std::move(start_up_promise));
}
// 3. 等待初始化完成
//当前线程在此处阻塞,直到工作线程调用promise.set_value()
// 确保StartUp()返回时,工作线程已完成所有初始化(消息循环已启动)
start_up_future.wait();
}
通过promise/future
机制实现了线程启动的同步初始化,确保调用者在返回时可以安全使用线程。
主要职责:
-
线程安全启动:确保同一线程实例不会被重复启动
-
同步初始化:阻塞调用线程,直到新线程完全初始化并准备好接收任务
-
资源管理:创建底层线程并关联消息循环
MessageLoopThread::RunThread
cpp
packages/modules/Bluetooth/system/common/message_loop_thread.cc
// Non API method, should not be protected by API mutex
void MessageLoopThread::RunThread(MessageLoopThread* thread,
std::promise<void> start_up_promise) {
thread->Run(std::move(start_up_promise));
}
RunThread
作为线程入口函数,通过静态方法和promise/future
机制,实现了以下目标:
-
安全地将控制权转移到实例方法
Run()
-
确保线程初始化完成后才返回
StartUp()
调用 -
分离线程创建与执行逻辑,遵循单一职责原则
该设计是多线程编程中的典型模式,通过静态入口点实现实例方法的线程化执行,同时保证初始化的同步性。
1. 为什么使用 promise/future
机制?
- 同步启动流程:
cpp
主线程 工作线程
StartUp()
|
v
创建线程 ───────────> RunThread()
| |
v v
future.wait() <─────── promise.set_value()
|
v
线程已就绪
- 确保
StartUp()
返回时线程已完全初始化
2. 为什么不直接在 StartUp()
中调用 Run()
?
-
线程隔离:
-
Run()
包含消息循环的阻塞操作 -
必须在新线程中执行以避免阻塞调用线程
-
-
生命周期管理:
-
线程资源的创建与销毁分离
-
通过
StartUp()
/ShutDown()
控制生命周期
-
3. 为什么需要静态方法?
-
std::thread
无法直接调用非静态成员函数 -
静态方法提供独立于实例的调用入口
-
通过显式传入
MessageLoopThread*
实现对实例的访问
MessageLoopThread::Run
cpp
packages/modules/Bluetooth/system/common/message_loop_thread.cc
void MessageLoopThread::Run(std::promise<void> start_up_promise) {
// 1. 初始化阶段
{
std::lock_guard<std::recursive_mutex> api_lock(api_mutex_);
LOG(INFO) << __func__ << ": message loop starting for thread "
<< thread_name_;
base::PlatformThread::SetName(thread_name_); // 设置线程名称:便于调试工具识别
// 创建消息循环对象
message_loop_ = new btbase::AbstractMessageLoop(); // 处理异步消息
run_loop_ = new base::RunLoop(); // 提供事件循环机制
// 记录线程 ID
thread_id_ = base::PlatformThread::CurrentId(); // 跨平台线程 ID
linux_tid_ = static_cast<pid_t>(syscall(SYS_gettid)); // Linux 特定的线程 ID
// 通知启动完成: 解除StartUp()方法的阻塞
start_up_promise.set_value();
}
// 2. 事件循环阶段
// Blocking until ShutDown() is called
// 持续处理队列中的任务,直到调用RunLoop::Quit()
// 线程在此处阻塞,直到外部调用ShutDown()
run_loop_->Run(); // 启动一个阻塞的事件循环
// 3. 资源清理阶段
{
std::lock_guard<std::recursive_mutex> api_lock(api_mutex_);
thread_id_ = -1;
linux_tid_ = -1;
// 释放消息循环对象
delete message_loop_;
message_loop_ = nullptr;
delete run_loop_;
run_loop_ = nullptr;
LOG(INFO) << __func__ << ": message loop finished for thread "
<< thread_name_;
}
}
Run()
方法实现了一个典型的消息循环线程:
-
初始化阶段:创建消息处理基础设施并通知主线程
-
运行阶段:持续处理任务队列,保持线程活跃(阻塞执行)
-
清理阶段:在线程退出时释放资源
生产者 - 消费者模型
cpp
┌─────────────┐ ┌─────────────┐
│ 调用线程 │ │ 工作线程 │
│ │ │ │
│ DoInThread() ─── 任务 ────► │ RunLoop │
│ │ │ (阻塞) │
│ ShutDown() ─── Quit() ────► │ │
└─────────────┘ └─────────────┘
-
任务投递 :外部线程通过
DoInThread()
将任务加入队列 -
任务执行 :工作线程在
RunLoop
中持续处理队列任务
MessageLoopThread::EnableRealTimeScheduling
cpp
packages/modules/Bluetooth/system/common/message_loop_thread.cc
bool MessageLoopThread::EnableRealTimeScheduling() {
// 1. 线程状态检查
std::lock_guard<std::recursive_mutex> api_lock(api_mutex_); // 使用递归锁api_mutex_保护共享资源
if (!IsRunning()) { // 确保在设置优先级时线程处于运行状态
LOG(ERROR) << __func__ << ": thread " << *this << " is not running";
return false;
}
// 2. 实时调度参数配置
// sched_priority范围通常为 1-99(数值越高优先级越高)
struct sched_param rt_params = {.sched_priority =
kRealTimeFifoSchedulingPriority};
// 3. 系统调用设置优先级
int rc = sched_setscheduler(linux_tid_, SCHED_FIFO, &rt_params); // 设置线程的调度策略和优先级
if (rc != 0) {
LOG(ERROR) << __func__ << ": unable to set SCHED_FIFO priority "
<< kRealTimeFifoSchedulingPriority << " for linux_tid "
<< std::to_string(linux_tid_) << ", thread " << *this
<< ", error: " << strerror(errno);
return false;
}
return true;
}
实现MessageLoopThread
类的实时调度优先级设置功能。EnableRealTimeScheduling()
方法的核心功能是:
-
将当前线程的调度策略设置为
SCHED_FIFO
(先进先出实时调度) -
为线程分配指定的实时优先级(
kRealTimeFifoSchedulingPriority
) -
检查并处理可能的权限错误和系统限制
通过设置**SCHED_FIFO
**实时调度策略,确保蓝牙协议栈线程能够获得低延迟的 CPU 响应,满足蓝牙通信的时序要求。但需要注意:
-
权限管理:需通过系统配置或 root 权限启用
-
优先级平衡:过高的优先级可能导致系统不稳定
在实际部署中,结合系统负载和其他关键进程的优先级进行综合配置,避免因过度优化导致系统整体性能下降。
SCHED_FIFO
是一种实时调度策略,具有以下特性:
高优先级任务可抢占低优先级任务
同优先级任务按 FIFO 顺序执行
任务会一直运行直到主动放弃 CPU 或被更高优先级任务抢占
1. 为何需要实时调度?
蓝牙协议栈的实时性需求:
蓝牙数据包处理对时序要求严格
低延迟响应对于保持连接稳定性至关重要
实时调度可减少上下文切换,确保关键任务及时执行
2. 为何选择 SCHED_FIFO?
调度特性匹配:
蓝牙协议栈需要确定性的响应时间
SCHED_FIFO
避免了时间片轮转带来的不确定性适用于短时间、高优先级的关键任务
3. 为何使用 linux_tid_而非 pthread_self ()?
Linux 系统实现差异:
pthread_self()
返回的是 POSIX 线程 ID(仅在进程内唯一)
linux_tid_
是内核级线程 ID(全局唯一)
sched_setscheduler()
需要内核级线程 ID
③基础支撑层初始化
-
DEVICE_IOT_CONFIG_MODULE:
-
管理设备级IoT特性开关(如BLE Mesh、AoA定位)
-
持久化存储设备行为画像(连接间隔偏好、重试策略)
-
-
OSI_MODULE:
-
提供跨平台统一API:线程(
osi_thread
)、互斥锁(osi_mutex
)、定时器(osi_alarm
) -
实现内存池管理,减少动态内存分配开销
-
④硬件交互层启动
-
GD_SHIM_MODULE(Google Direct硬件抽象层):
-
提供统一HCI接口,兼容不同蓝牙芯片(如Broadcom/Qualcomm)
-
实现Vendor Specific HCI命令封装(如芯片固件加载、RF参数校准)
-
管理硬件资源:电源状态(Active/Sniff/Hold)、射频开关
-
⑤协议栈核心初始化
-
BTIF_CONFIG_MODULE:
-
加载
/etc/bluetooth/bt_stack.conf
配置文件 -
管理运行时参数:SDP缓存大小、SCO链路数、协议日志级别
-
-
btif_init_bluetooth():
-
注册JNI回调接口(
com_android_bluetooth.cpp
) -
初始化适配层状态机(Powered/Discoverable/Connectable)
-
btif_init_bluetooth
cpp
/*******************************************************************************
*
* Function btif_init_bluetooth
*
* Description Creates BTIF task and prepares BT scheduler for startup
*
* Returns bt_status_t
*
******************************************************************************/
bt_status_t btif_init_bluetooth() {
log::info("entered");
// 1. 进程退出管理初始化:创建一个全局的退出管理器,用于注册和执行程序退出时的清理任务
exit_manager = new base::AtExitManager();
// 2. JNI 环境初始化
jni_thread_startup();
// 3. 触发线程事件回调
GetInterfaceToProfiles()->events->invoke_thread_evt_cb(ASSOCIATE_JVM);
log::info("finished");
return BT_STATUS_SUCCESS;
}
通过创建退出管理器、初始化 JNI 环境和触发线程事件,为蓝牙功能的启动奠定基础。
关键步骤:
-
创建进程退出管理机制
-
初始化 JNI(Java Native Interface)环境
-
触发线程事件回调以关联 Java 虚拟机(JVM)
-
为蓝牙协议栈的启动做准备
分层架构:
cpp
┌───────────────────────┐
│ Android Java 层 │
│ (Bluetooth Framework)│
├───────────────────────┤
│ JNI 桥接层 │
│ (btif_init_bluetooth)│
├───────────────────────┤
│ 蓝牙协议栈 C++ 层 │
│ (libbluetooth-core) │
└───────────────────────┘
-
作用:
-
隔离 Java 层与 C++ 层的实现细节
-
提供统一的蓝牙功能接口
-
设计模式:
使用 RAII(资源获取即初始化)模式管理程序生命周期
观察者模式:通过事件回调机制实现组件间解耦
jni_thread_startup
cpp
packages/modules/Bluetooth/system/btif/src/btif_jni_task.cc
static bluetooth::common::MessageLoopThread jni_thread("bt_jni_thread");
void jni_thread_startup() { jni_thread.StartUp(); }
定义了一个专用于处理 JNI(Java Native Interface)交互的线程,并提供了启动该线程的接口:
-
创建名为 "bt_jni_thread" 的专用线程
-
通过
jni_thread_startup()
函数启动该线程
创建专用的 JNI 线程,实现蓝牙协议栈与 Android Java 层的安全交互。通过线程隔离,避免了 JNI 调用的线程安全问题,同时保持了蓝牙核心逻辑与上层应用的解耦。
invoke_thread_evt_cb
cpp
packages/modules/Bluetooth/system/btif/src/bluetooth.cc
void invoke_thread_evt_cb(bt_cb_thread_evt event) {
do_in_jni_thread(FROM_HERE, base::BindOnce(
[](bt_cb_thread_evt event) {
HAL_CBACK(bt_hal_cbacks, thread_evt_cb,
event);
if (event == DISASSOCIATE_JVM) {
bt_hal_cbacks = NULL;
}
},
event));
}
实现蓝牙协议栈中的线程事件回调机制,通过将事件处理统一到 JNI 线程,确保了 HAL 回调的线程安全性。核心功能是:
-
将线程事件(如 JVM 关联 / 解除关联)异步投递到 JNI 线程执行
-
通过 HAL 回调接口通知上层事件
-
在 JVM 解除关联时清理回调指针
跨线程通信模型:
cpp
┌───────────────────────┐ ┌───────────────────────┐
│ 调用线程 │ │ JNI 线程 │
│ │ │ │
│ invoke_thread_evt_cb ├───►│ thread_evt_cb │
│ (任意线程) │ │ (固定线程) │
└───────────────────────┘ └───────────────────────┘
-
将 HAL 回调统一到 JNI 线程执行,避免多线程竞态
-
简化上层处理逻辑,无需关心线程安全
线程时序保障:
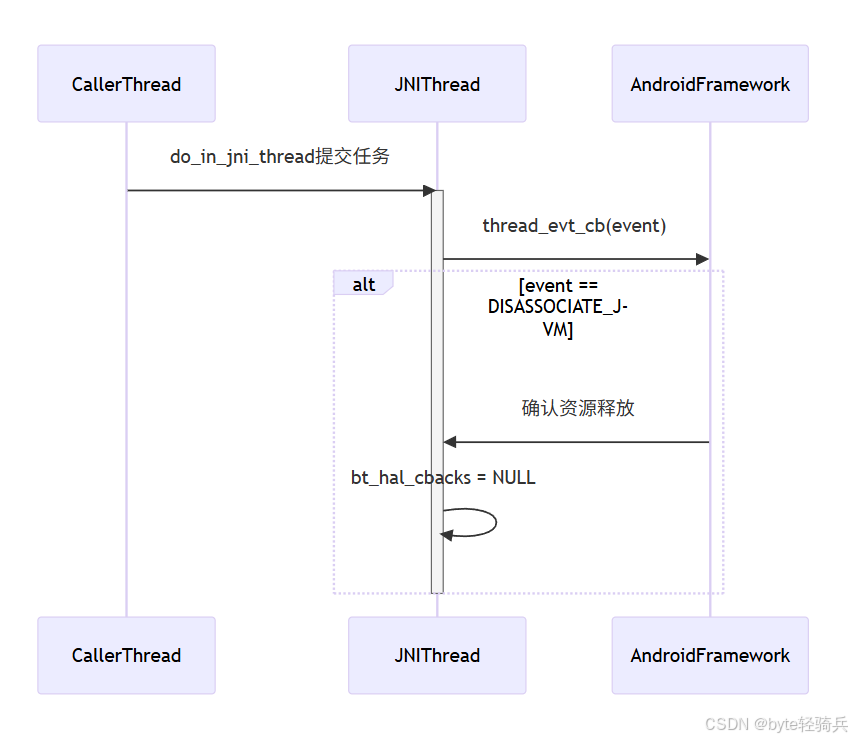
⑥高级功能模块
-
INTEROP_MODULE:
-
维护设备兼容性数据库(如避免特定Android手机与Car Kit的AVRCP版本冲突)
-
实现动态工作区(Dynamic Workaround):在运行时绕过已知协议栈缺陷
-
-
STACK_CONFIG_MODULE:
-
热配置管理:支持通过DBus动态修改参数(如BLE扫描窗口无需重启协议栈)
-
提供调试接口:HCI日志捕获、协议分析开关
-
三、时序图
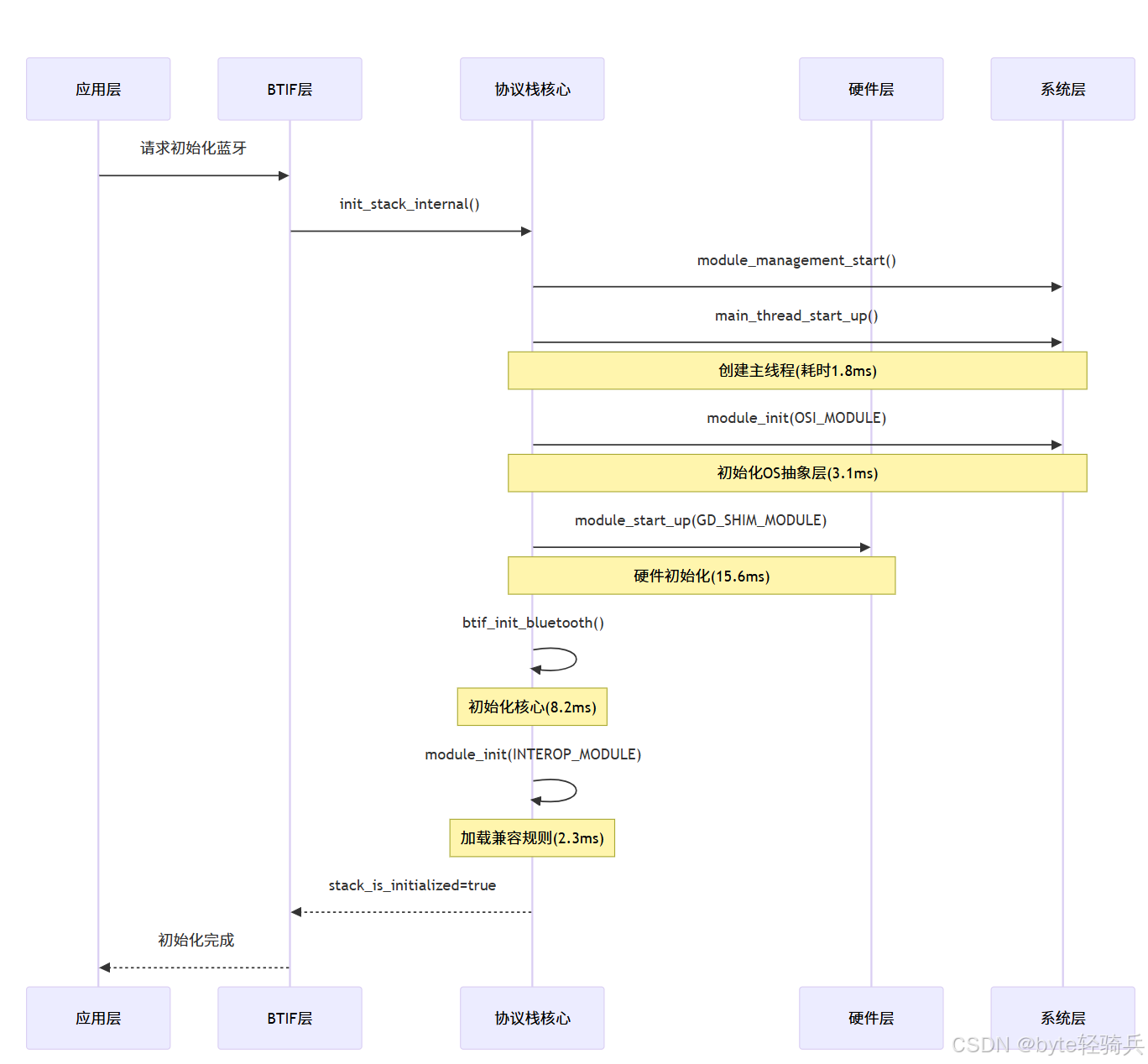
主事件线程启动时序图:
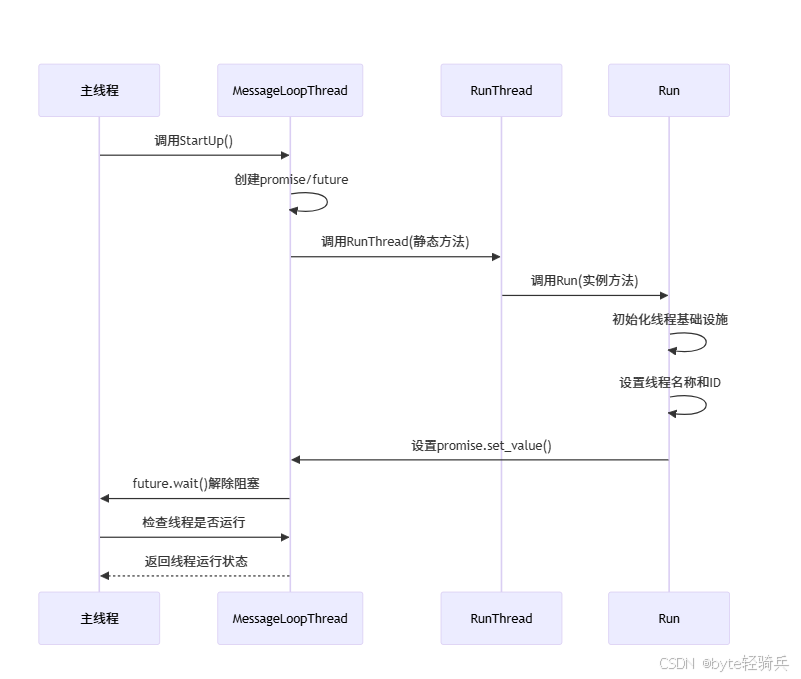
四、流程图
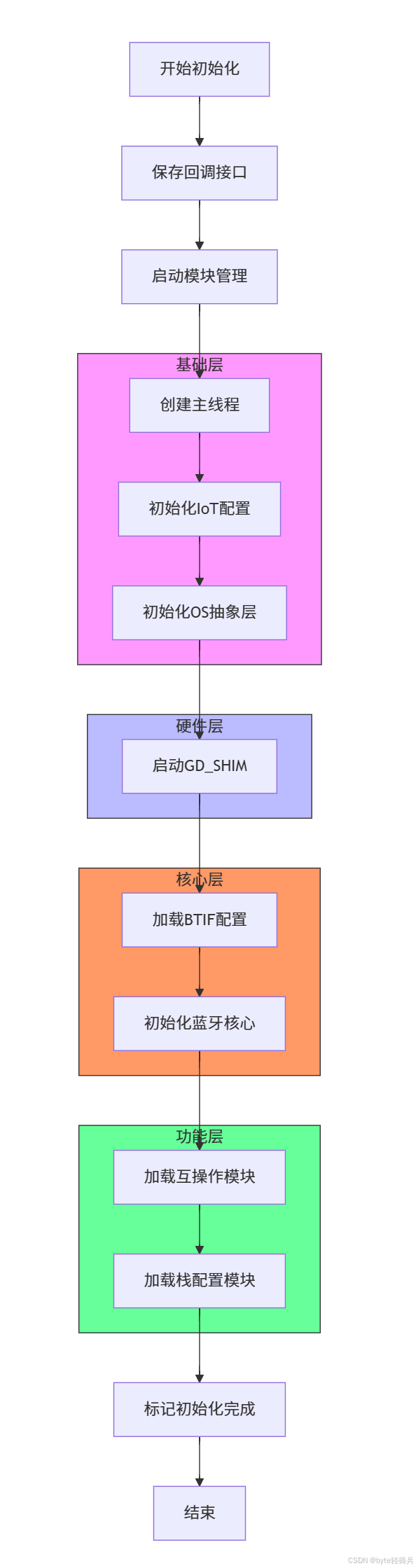
实时调度流程图:
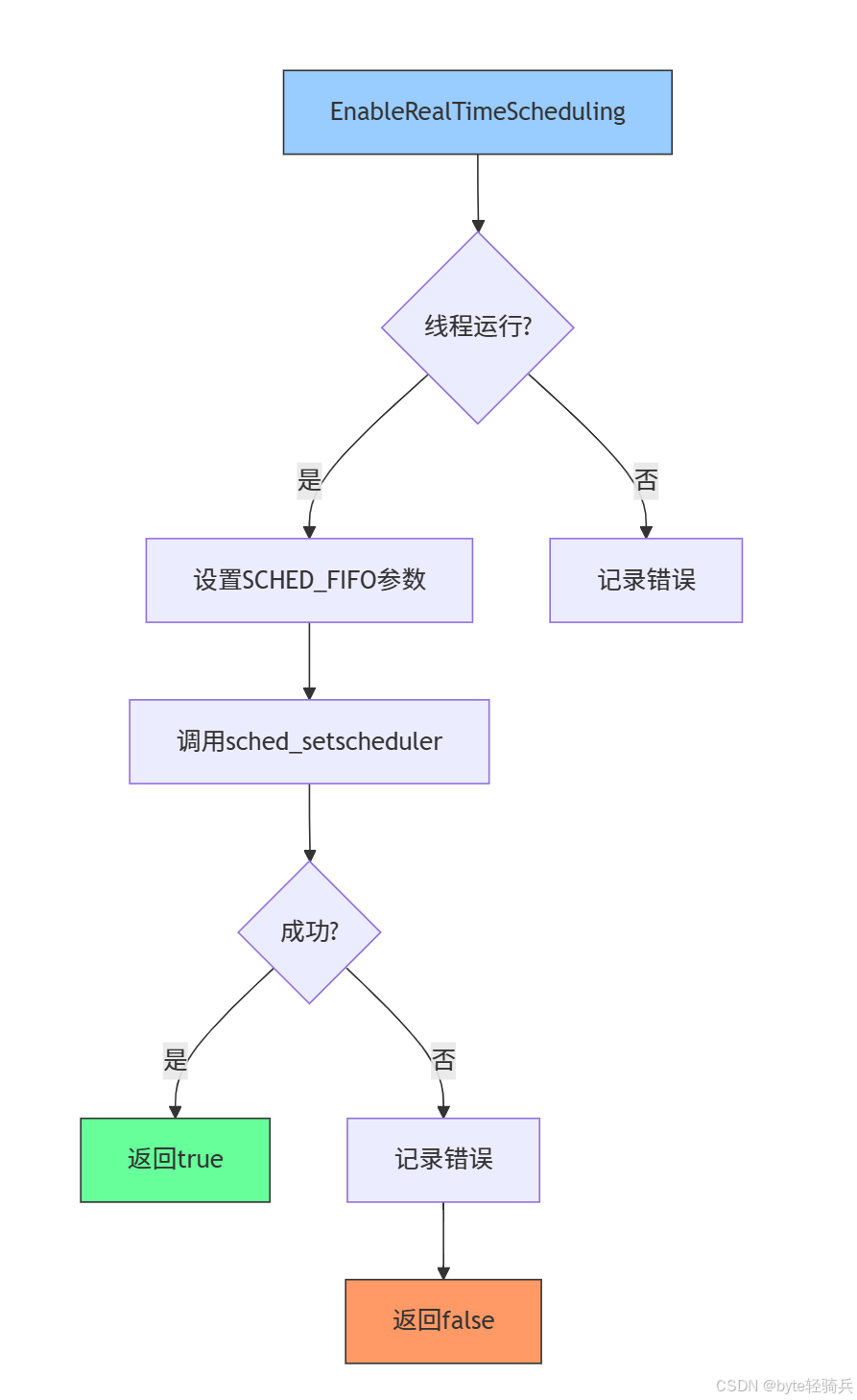
五、总结
通过对init_stack_internal
函数的源码拆解,清晰呈现了 Android Bluedroid 协议栈初始化的完整脉络。从模块的强 / 弱顺序约束,到线程同步机制的设计,再到各功能层的初始化细节。理解这些底层逻辑,不仅有助于解决蓝牙启动等实际开发问题,还能为协议栈优化、新功能扩展提供理论支撑,希望对从事 Android 蓝牙开发与嵌入式相关工作的技术人员有实践指导意义。