项目成果 项目网盘
导入资源包 放入Assets文件Assets资源文件
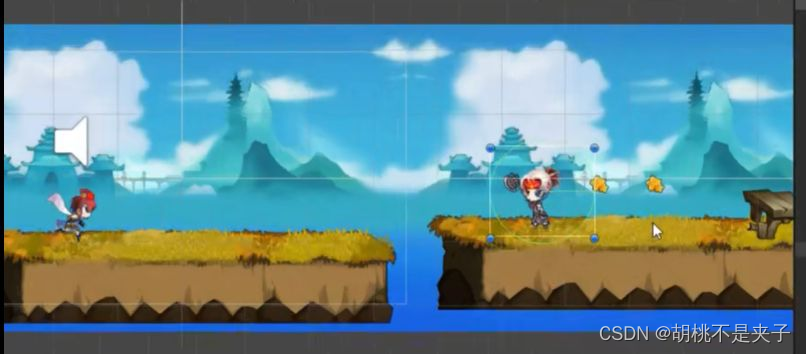
游戏流程分析
摄像机size调小,让图片占满屏幕
人跑本质,相对运动,图片无限向右滚动
图片720,缩小100倍第二个图片x为7.2每unit px100两张图片刚好挨着连贯
空对象BgControl,方便管理
reset
放两张图片
创脚本Bgcontrol,拖到该对象上
层级-10
脚本文件夹,c#脚本
地面对象
层级-5
碰撞组件box collider 2d,编辑碰撞器。
挂脚本
三个地面都拖成预设体
前两个地面固定,后一个随机
Prefabs预设体文件夹
ground拖进去
coin进去
切片
多张
sprite editor
切片
应用
对象声音,挂脚本
单例
创建groundcontrol脚本
脚本挂到地面对象上
预设体相当于类和对象
背景 -10
地面 -5地面预设体给标签ground 碰撞器
声音 挂脚本
动画 窗口 跑 跳 死亡 过渡 设置参数
无退出,过渡0,isjump true
主角 层级10 标签player 碰撞组件 刚体组件 冻结旋转 重力缩放2,只提供重力加速度,加脚本(跳跃,地面上才能跳跃,播放声音,动画切换),
button UI 原图像 原像素显示,过渡 精灵切换 高亮图像 点击 玩家的跳跃 导航none
金币 脚本 碰撞器 吃的触发
桌子 放1号地面上 碰撞器 标签地面 预设体
1地应用所有
死亡 空对象die 碰撞器 编辑触发器 标签die
player脚本
敌人 圆形碰撞器 1地 标签enemy 1地应用所有 预设体 触发
层级
切片
脚本代码
PlayerControl
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerControl : MonoBehaviour
{
//血量
public static int Hp=1;
//刚体组件
private Rigidbody2D rbody;
//动画组件
private Animator ani;
private bool isGround;
void Start()
{
//获取刚体组件
rbody = GetComponent<Rigidbody2D>();
//获取动画组件
ani = GetComponent<Animator>();
}
void Update()
{
//如果按了空格键
if (Input.GetKeyDown(KeyCode.Space))
{
//跳跃
Jump();
}
}
//跳跃
public void Jump()
{
if (isGround == true)
{
//给刚体一个向上的力
rbody.AddForce(Vector2.up * 400);
//播放跳跃声音
AudioManager.Instance.Play("跳");
}
}
//发生碰撞
private void OnCollisionEnter2D(Collision2D collision)
{
//判断如果是地面
if(collision.collider.tag=="Ground")
{
isGround = true;
//结束跳跃
ani.SetBool("IsJump", false);
}
//如果是死亡边界
if(collision.collider.tag=="Die" && Hp > 0)
{
//血量为0
Hp = 0;
//
//播放死亡声音
AudioManager.Instance.Play("Boss死了");
//播放死亡动画
ani.SetBool("IsDie", true);
}
}
//结束碰撞
private void OnCollisionExit2D(Collision2D collision)
{
//判断如果是地面
if (collision.collider.tag == "Ground")
{
isGround = false;
//开始跳跃
ani.SetBool("IsJump", true);
}
}
//如果碰到敌人
private void OnTriggerEnter2D(Collider2D collision)
{
if (collision.tag == "Enemy")
{
//血量为0
Hp = 0;
//
//播放死亡声音
AudioManager.Instance.Play("Boss死了");
//播放死亡动画
ani.SetBool("IsDie", true);
}
}
}
CoinControl
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CoinControl : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
//如果产生触发
private void OnTriggerEnter2D(Collider2D collision)
{
//播放吃金币的声音
AudioManager.Instance.Play("金币");
//销毁自己
Destroy(gameObject);
}
}
AudioMnager
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class AudioManager : MonoBehaviour
{
//单例
public static AudioManager Instance;
//播放组件
private AudioSource player;
void Start()
{
//单例
Instance = this;
//获取播放组件
player = GetComponent<AudioSource>();
}
//播放音效
public void Play(string name)
{
//通过名称获取音频片段
AudioClip clip = Resources.Load<AudioClip>(name);
//播放
player.PlayOneShot(clip);
}
}
BgControl
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BgControl : MonoBehaviour
{
//速度 每帧移动0.2像素
public float Speed = 0.2f;
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update() //update是每一帧会调用一次
{
//如果玩家血量为0
if(PlayerControl.Hp==0)
{
return;
}
//遍历背景,背景就是子物体
foreach (Transform tran in transform)
{
//获取子物体的位置
Vector3 pos = tran.position;
//按照速度向左侧移动
pos.x -= Speed * Time.deltaTime; //每秒向左侧移动0.2
//判断是否出了屏幕
if (pos.x < -7.2f)
{
//把图片移动到右边
pos.x += 7.2f * 2;
}
//位置赋给子物体
tran.position = pos;
}
}
}
GroundControl
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GroundControl : MonoBehaviour
{//速度
public float Speed = 2f;
//要随机的地面数组
public GameObject[] GroundPrefabs;
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update() //update是每一帧会调用一次
{
//如果玩家血量为0
if (PlayerControl.Hp == 0)
{
return;
}
//遍历背景,背景就是子物体
foreach (Transform tran in transform)
{
//获取子物体的位置
Vector3 pos = tran.position;
//按照速度向左侧移动
pos.x -= Speed * Time.deltaTime; //每秒向左侧移动0.2
//判断是否出了屏幕
if (pos.x < -7.2f)
{
//创建新的地面
Transform newTrans = Instantiate(GroundPrefabs[Random.Range(0, GroundPrefabs.Length)], transform).transform;
// ,后transform确定父子关系 .后transform拿到新地面的transform组件
//获取新地面的位置
Vector2 newPos = newTrans.position;
//设置新地面的位置
newPos.x = pos.x + 7.2f * 2;
//位置设置回去
newTrans.position = newPos;
//销毁旧的地面(出了屏幕的地面)
Destroy(tran.gameObject);
}
//位置赋给子物体
tran.position = pos;
}
}
}