引导用户按照流程完成任务的导航条。
何时使用
当任务复杂或者存在先后关系时,将其分解成一系列步骤,从而简化任务。
案例:步骤条组件
核心代码:
html
<template>
<a-steps
:current="1"
:items="[
{
title: 'Finished',
description,
},
{
title: 'In Progress',
description,
subTitle: 'Left 00:00:08',
},
{
title: 'Waiting',
description,
},
]"
></a-steps>
</template>
<script lang="ts" setup>
const description = 'This is a description.';
</script>
vue3示例:
html
<script setup>
const description = '关于步骤的描述文本';
</script>
<template>
<!--
:current="1":当前步骤,是从0开始的
:items: 具体的步骤,是一个数组,数组元素支持如下属性
title:标题
subTitle:副标题
description:描述
-->
<a-steps
:current="1"
:items="[
{
title: '已完成',
description,
},
{
title: '进行中',
description,
subTitle: '副标题',
},
{
title: '待办事项',
description,
},
]"
></a-steps>
</template>

案例:小型步骤条
迷你版的步骤条,通过设置 <Steps size="small">
启用.
核心代码:
html
<template>
<a-steps
:current="1"
size="small"
:items="[
{
title: 'Finished',
},
{
title: 'In Progress',
},
{
title: 'Waiting',
},
]"
></a-steps>
</template>
如何实现:size="small"
vue3示例:
html
<script setup>
const description = '关于步骤的描述文本';
const items = [
{
title: '已完成',
description,
},
{
title: '进行中',
description,
subTitle: '副标题',
},
{
title: '待办事项',
description,
},
]
</script>
<template>
<a-steps :current="1" :items="items"/>
<a-divider>小步骤条</a-divider>
<a-steps :current="1" :items="items" size="small"/>
</template>

案例:带图标的步骤条
通过设置 Steps.Step 的 icon 属性,可以启用自定义图标。
核心代码:
html
<template>
<a-steps :items="items"></a-steps>
</template>
<script lang="ts" setup>
import { h } from 'vue';
import {
UserOutlined,
SolutionOutlined,
LoadingOutlined,
SmileOutlined,
} from '@ant-design/icons-vue';
import { StepProps } from 'ant-design-vue';
const items = [
{
title: 'Login',
status: 'finish',
icon: h(UserOutlined),
},
{
title: 'Verification',
status: 'finish',
icon: h(SolutionOutlined),
},
{
title: 'Pay',
status: 'process',
icon: h(LoadingOutlined),
},
{
title: 'Done',
status: 'wait',
icon: h(SmileOutlined),
},
] as StepProps[];
</script>
第一步:导入图标
js
import { h } from 'vue';
import {
UserOutlined,
SolutionOutlined,
LoadingOutlined,
SmileOutlined,
} from '@ant-design/icons-vue';
第二步:渲染图标
json
{
title: 'Verification',
status: 'finish',
icon: h(SolutionOutlined),
}
vue3示例:
html
<script setup>
import { h } from 'vue';
import {
UserOutlined,
SolutionOutlined,
LoadingOutlined,
SmileOutlined,
} from '@ant-design/icons-vue';
const description = '关于步骤的描述文本';
const items = [
{
title: '已完成',
description,
icon: h(UserOutlined),
},
{
title: '进行中',
description,
subTitle: '副标题',
icon: h(SolutionOutlined),
},
{
title: '待办事项',
description,
icon: h(LoadingOutlined),
},
]
</script>
<template>
<div class="p-28 bg-indigo-50">
<a-steps :current="1" :items="items"/>
</div>
</template>
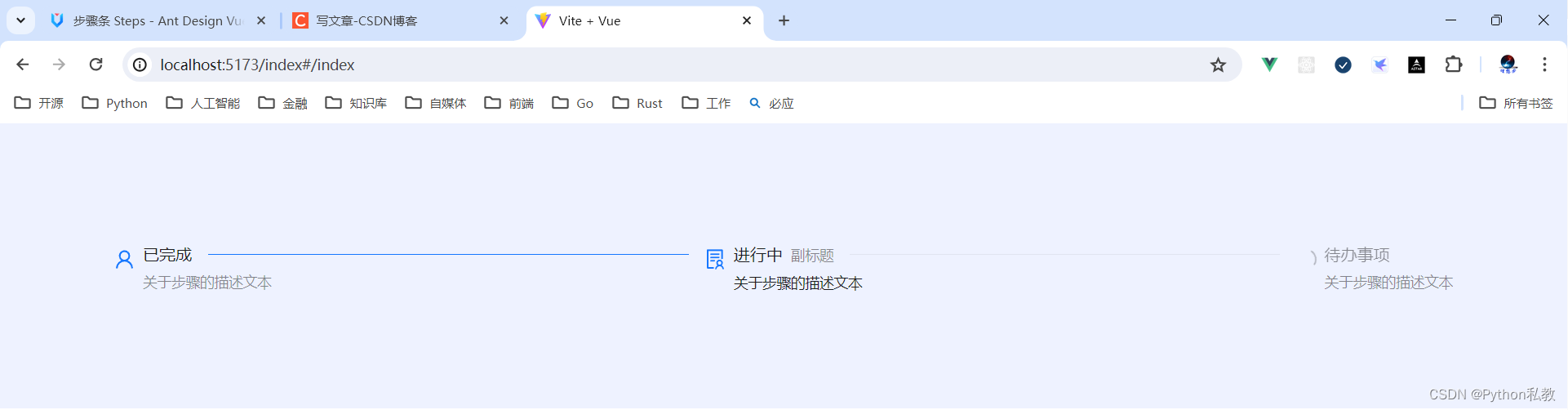
案例:步骤切换
通常配合内容及按钮使用,表示一个流程的处理进度。
核心代码:
html
<template>
<div>
<a-steps :current="current" :items="items"></a-steps>
<div class="steps-content">
{{ steps[current].content }}
</div>
<div class="steps-action">
<a-button v-if="current < steps.length - 1" type="primary" @click="next">Next</a-button>
<a-button
v-if="current == steps.length - 1"
type="primary"
@click="message.success('Processing complete!')"
>
Done
</a-button>
<a-button v-if="current > 0" style="margin-left: 8px" @click="prev">Previous</a-button>
</div>
</div>
</template>
<script lang="ts" setup>
import { ref } from 'vue';
import { message } from 'ant-design-vue';
const current = ref<number>(0);
const next = () => {
current.value++;
};
const prev = () => {
current.value--;
};
const steps = [
{
title: 'First',
content: 'First-content',
},
{
title: 'Second',
content: 'Second-content',
},
{
title: 'Last',
content: 'Last-content',
},
];
const items = steps.map(item => ({ key: item.title, title: item.title }));
</script>
<style scoped>
.steps-content {
margin-top: 16px;
border: 1px dashed #e9e9e9;
border-radius: 6px;
background-color: #fafafa;
min-height: 200px;
text-align: center;
padding-top: 80px;
}
.steps-action {
margin-top: 24px;
}
[data-theme='dark'] .steps-content {
background-color: #2f2f2f;
border: 1px dashed #404040;
}
</style>
第一步:绑定当前步骤 :current="current"
html
<a-steps :current="current" :items="items"></a-steps>
第二步:展示步骤内容
html
<div class="steps-content">
{{ steps[current].content }}
</div>
第三步:展示切换内容
html
<div class="steps-action">
<a-button v-if="current < steps.length - 1" type="primary" @click="next">Next</a-button>
<a-button
v-if="current == steps.length - 1"
type="primary"
@click="message.success('Processing complete!')"
>
Done
</a-button>
<a-button v-if="current > 0" style="margin-left: 8px" @click="prev">Previous</a-button>
</div>
第四步:编写切换方法
js
import { message } from 'ant-design-vue';
const current = ref<number>(0);
const next = () => {
current.value++;
};
const prev = () => {
current.value--;
};
vue3示例:
html
<script setup>
import {h, ref} from 'vue';
import {
UserOutlined,
SolutionOutlined,
LoadingOutlined,
SmileOutlined,
} from '@ant-design/icons-vue';
const current = ref(0)
const description = '关于步骤的描述文本';
const items = [
{
title: '已完成',
description,
icon: h(UserOutlined),
},
{
title: '进行中',
description,
subTitle: '副标题',
icon: h(SolutionOutlined),
},
{
title: '待办事项',
description,
icon: h(LoadingOutlined),
},
]
</script>
<template>
<div class="p-28 bg-indigo-50">
<a-steps :current="current" :items="items"/>
<div class="container bg-red-300 mt-3 min-h-72 p-3">
{{items[current].title}}
</div>
<div class="container bg-red-300 mt-3 p-3">
<a-button type="primary" @click="current--">上一步</a-button>
<a-button type="primary" @click="current++">下一步</a-button>
</div>
</div>
</template>
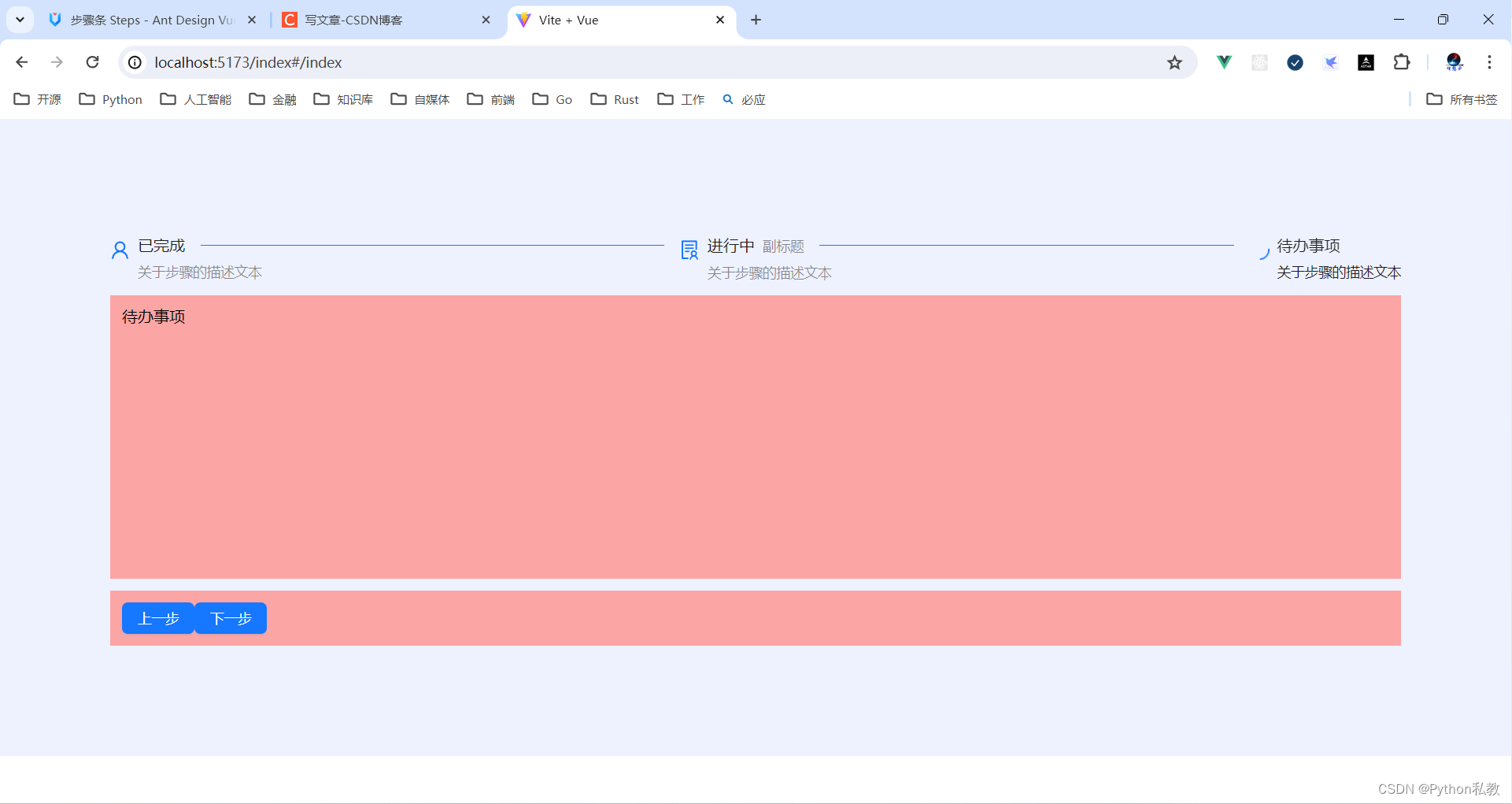
案例:垂直步骤条
核心代码:
html
<template>
<a-steps
direction="vertical"
:current="1"
:items="[
{
title: 'Finished',
description,
},
{
title: 'In Progress',
description,
},
{
title: 'Waiting',
description,
},
]"
></a-steps>
</template>
<script lang="ts" setup>
const description = 'This is a description.';
</script>
如何实现:direction="vertical"
vue3示例:
html
<script setup>
import {h, ref} from 'vue';
import {
UserOutlined,
SolutionOutlined,
LoadingOutlined,
SmileOutlined,
} from '@ant-design/icons-vue';
const current = ref(0)
const description = '关于步骤的描述文本';
const items = [
{
title: '已完成',
description,
icon: h(UserOutlined),
},
{
title: '进行中',
description,
subTitle: '副标题',
icon: h(SolutionOutlined),
},
{
title: '待办事项',
description,
icon: h(LoadingOutlined),
},
]
</script>
<template>
<div class="p-28 bg-indigo-50">
<a-steps :current="current" :items="items" direction="vertical"/>
<div class="container bg-red-300 mt-3 min-h-72 p-3">
{{items[current].title}}
</div>
<div class="container bg-red-300 mt-3 p-3">
<a-button type="primary" @click="current--">上一步</a-button>
<a-button type="primary" @click="current++">下一步</a-button>
</div>
</div>
</template>
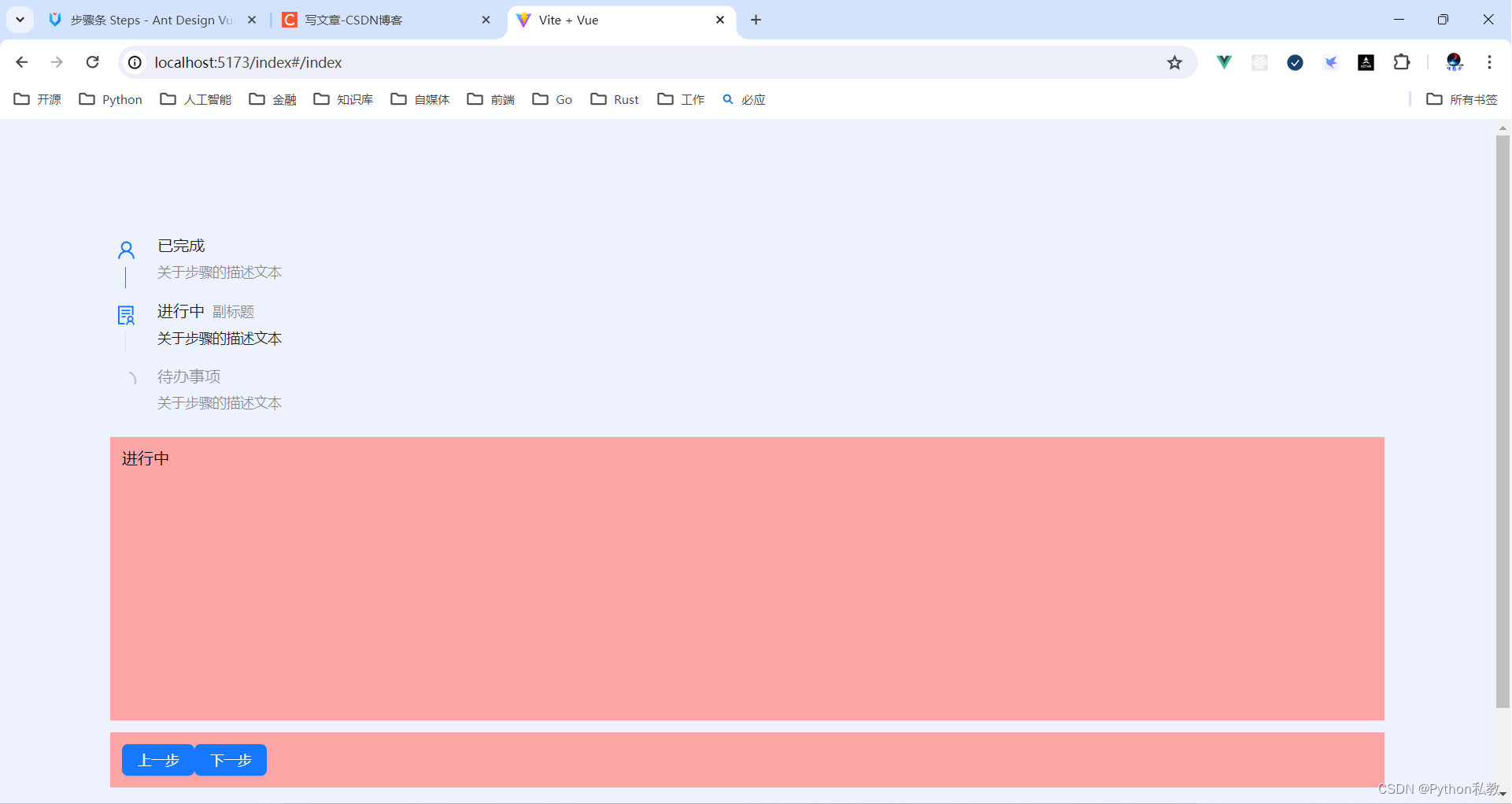
案例:步骤运行错误
使用 Steps 的 status 属性来指定当前步骤的状态。
核心代码:
html
<template>
<a-steps
v-model:current="current"
status="error"
:items="[
{
title: 'Finished',
description,
},
{
title: 'In Process',
description,
},
{
title: 'Waiting',
description,
},
]"
></a-steps>
</template>
<script lang="ts" setup>
import { ref } from 'vue';
const current = ref<number>(1);
const description = 'This is a description.';
</script>
如何实现:status="error"
vue3示例:
html
<script setup>
import {h, ref} from 'vue';
import {
UserOutlined,
SolutionOutlined,
LoadingOutlined,
SmileOutlined,
} from '@ant-design/icons-vue';
const current = ref(0)
const description = '关于步骤的描述文本';
const items = [
{
title: '已完成',
description,
icon: h(UserOutlined),
},
{
title: '进行中',
description,
subTitle: '副标题',
icon: h(SolutionOutlined),
},
{
title: '待办事项',
description,
icon: h(LoadingOutlined),
},
]
</script>
<template>
<div class="p-28 bg-indigo-50">
<a-steps :current="current" :items="items" direction="vertical" status="error"/>
<div class="container bg-red-300 mt-3 min-h-72 p-3">
{{items[current].title}}
</div>
<div class="container bg-red-300 mt-3 p-3">
<a-button type="primary" @click="current--">上一步</a-button>
<a-button type="primary" @click="current++">下一步</a-button>
</div>
</div>
</template>
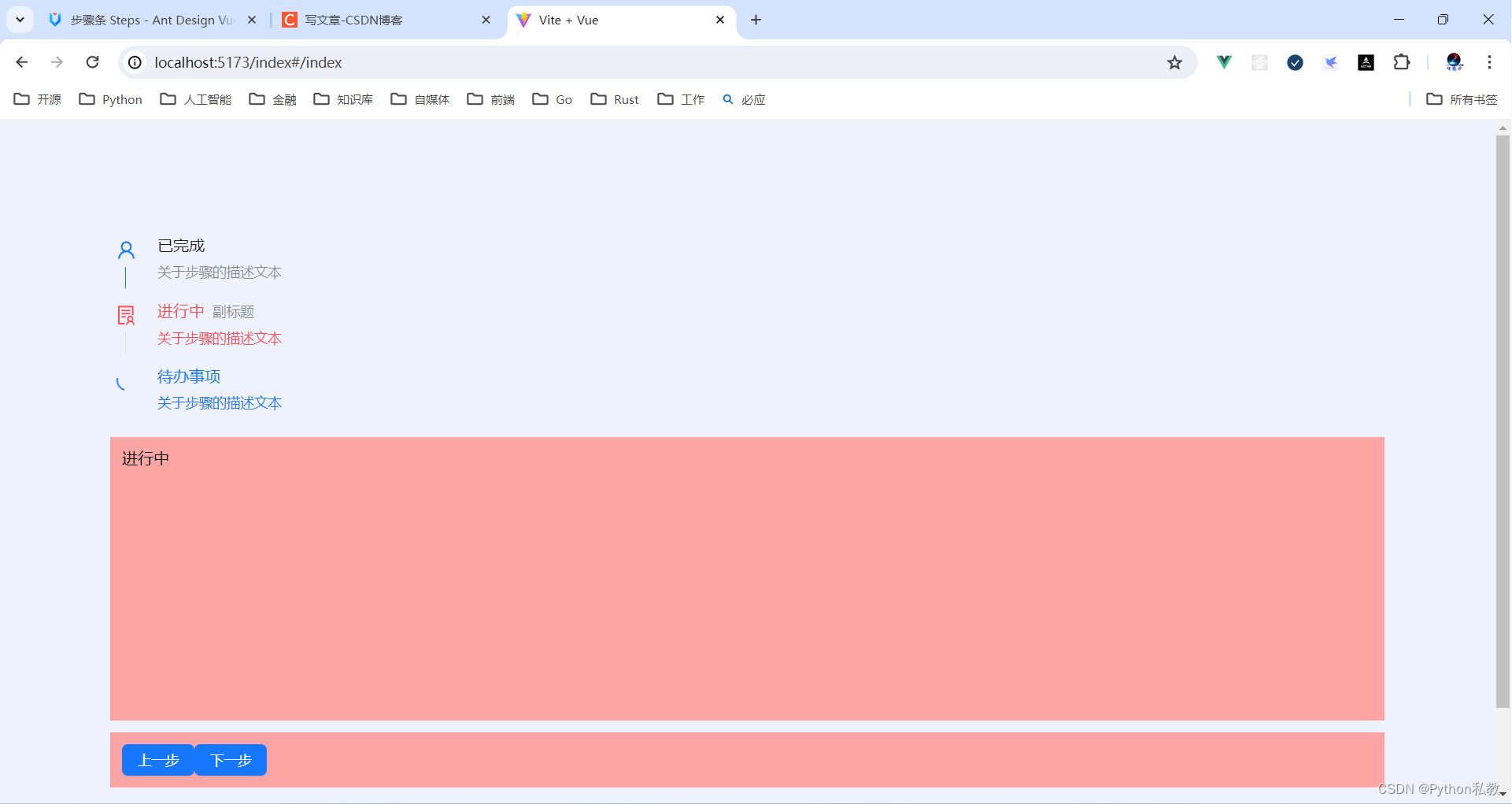
案例:点状步骤条
包含步骤点的进度条。
核心代码:
html
<template>
<div>
<a-steps
progress-dot
:current="1"
:items="[
{
title: 'Finished',
description: 'This is a description.',
},
{
title: 'In Progress',
description: 'This is a description.',
},
{
title: 'Waiting',
description: 'This is a description.',
},
]"
></a-steps>
<a-divider />
<a-steps
progress-dot
:current="1"
direction="vertical"
:items="[
{
title: 'Finished',
description: 'This is a description. This is a description.',
},
{
title: 'Finished',
description: 'This is a description. This is a description.',
},
{
title: 'In Progress',
description: 'This is a description. This is a description.',
},
{
title: 'Waiting',
description: 'This is a description.',
},
{
title: 'Waiting',
description: 'This is a description.',
},
]"
></a-steps>
</div>
</template>
如何实现:progress-dot
vue3示例:
html
<script setup>
import {h, ref} from 'vue';
const current = ref(0)
const description = '关于步骤的描述文本';
const items = [
{
title: '已完成',
description,
},
{
title: '进行中',
description,
subTitle: '副标题',
},
{
title: '待办事项',
description,
},
]
</script>
<template>
<div class="p-28 bg-indigo-50">
<a-steps :current="current" :items="items" direction="vertical" status="error" progress-dot/>
<div class="container bg-red-300 mt-3 min-h-72 p-3">
{{items[current].title}}
</div>
<div class="container bg-red-300 mt-3 p-3">
<a-button type="primary" @click="current--">上一步</a-button>
<a-button type="primary" @click="current++">下一步</a-button>
</div>
</div>
</template>
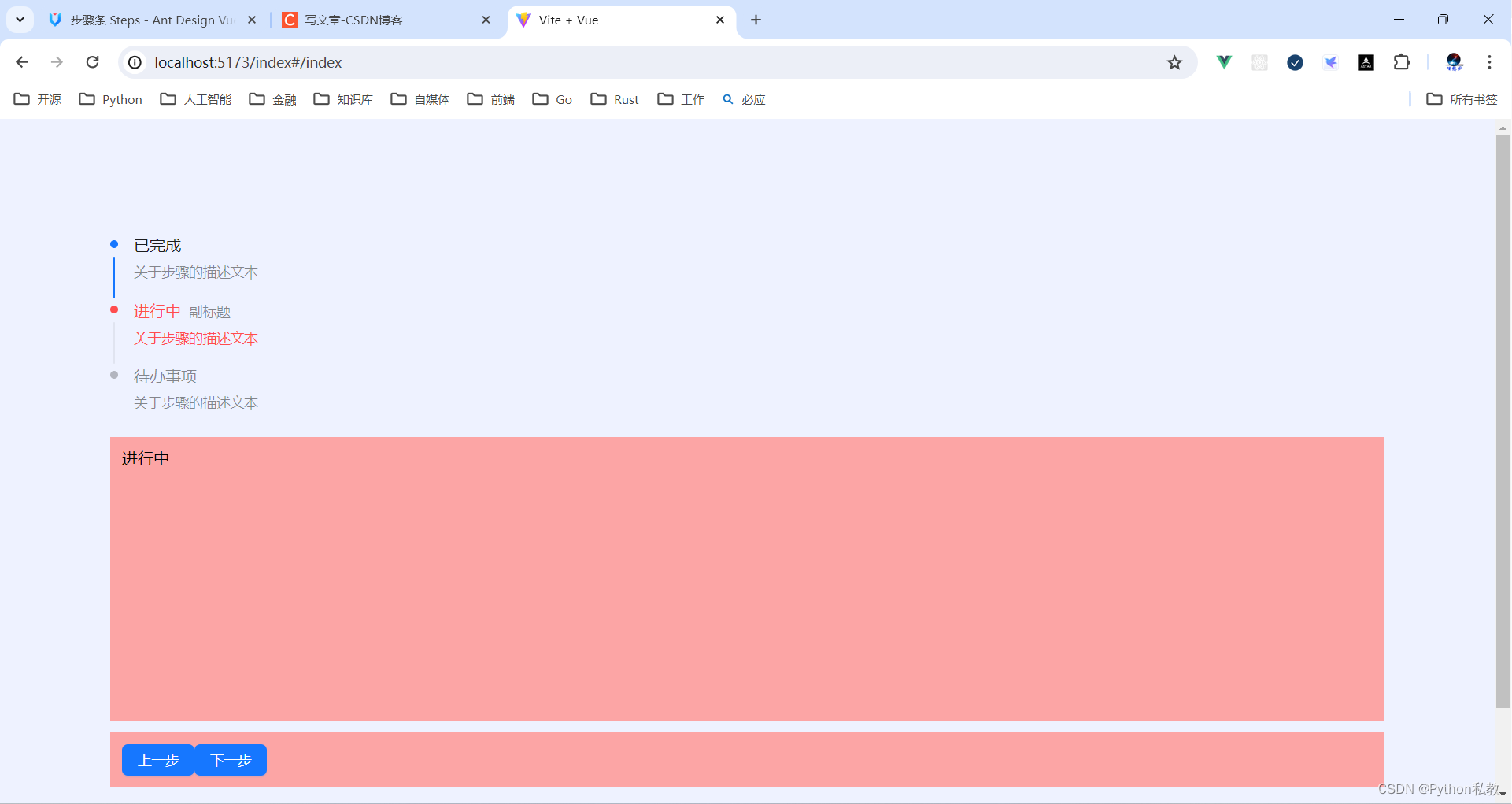
案例:步骤可点击
设置 v-model 后,Steps 变为可点击状态。
核心代码:
html
<template>
<div>
<a-steps
v-model:current="current"
:items="[
{
title: 'Step 1',
description,
},
{
title: 'Step 2',
description,
},
{
title: 'Step 3',
description,
},
]"
></a-steps>
<a-divider />
<a-steps
v-model:current="current"
direction="vertical"
:items="[
{
title: 'Step 1',
description,
},
{
title: 'Step 2',
description,
},
{
title: 'Step 3',
description,
},
]"
></a-steps>
</div>
</template>
<script lang="ts" setup>
import { ref } from 'vue';
const current = ref<number>(0);
const description = 'This is a description.';
</script>
如何实现:v-model:current="current"
vue3示例:
html
<script setup>
import {h, ref} from 'vue';
const current = ref(0)
const description = '关于步骤的描述文本';
const items = [
{
title: '已完成',
description,
},
{
title: '进行中',
description,
subTitle: '副标题',
},
{
title: '待办事项',
description,
},
]
</script>
<template>
<div class="p-28 bg-indigo-50">
<a-steps v-model:current="current" :items="items" status="error" progress-dot/>
<div class="container bg-red-300 mt-3 min-h-72 p-3">
{{items[current].title}}
</div>
<div class="container bg-red-300 mt-3 p-3">
<a-button type="primary" @click="current--">上一步</a-button>
<a-button type="primary" @click="current++">下一步</a-button>
</div>
</div>
</template>
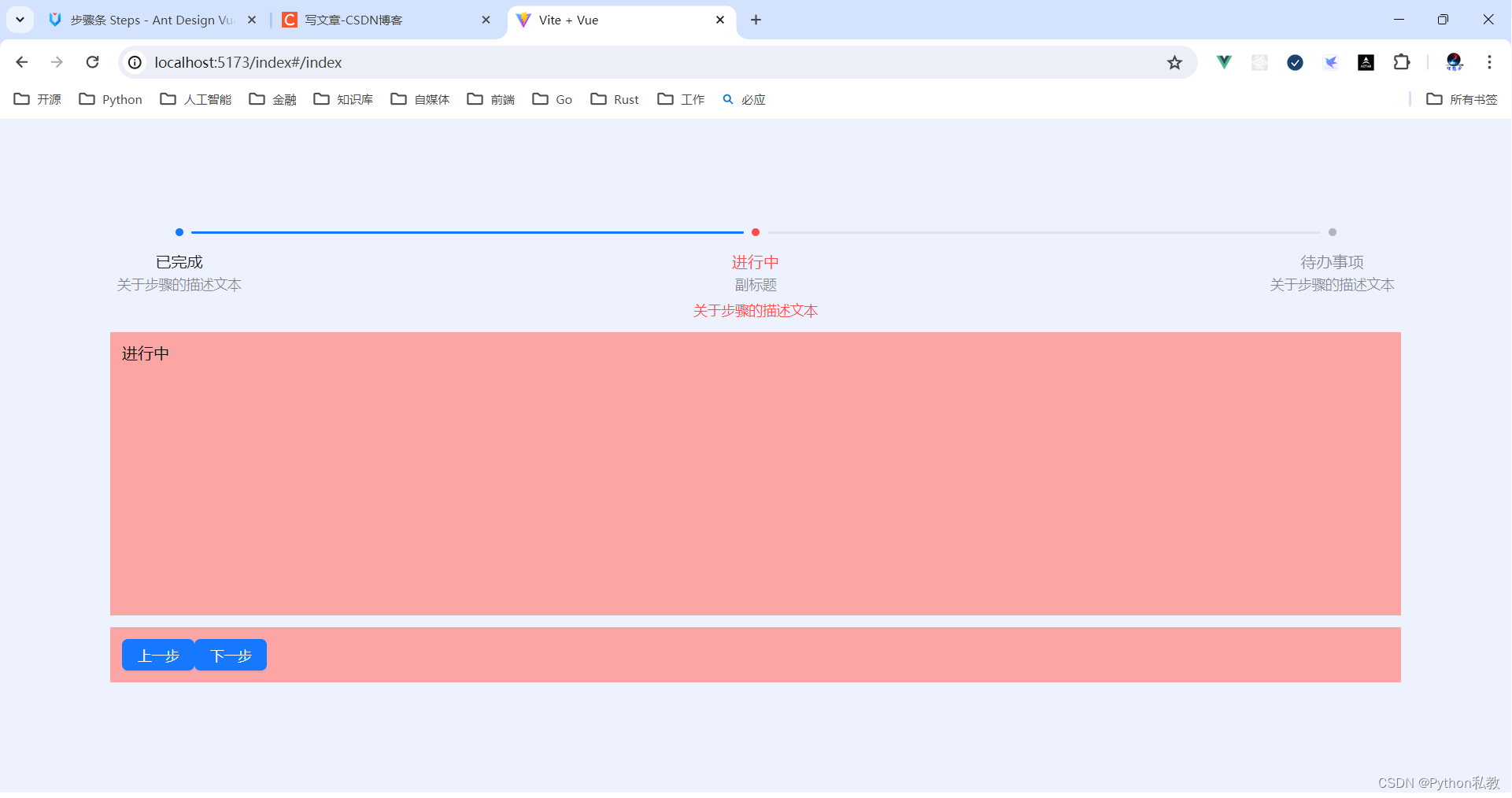
案例:导航步骤条
核心代码:
html
<template>
<div>
<a-steps
v-model:current="current"
type="navigation"
size="small"
:style="stepStyle"
:items="[
{
title: 'Step 1',
subTitle: '00:00:05',
status: 'finish',
description: 'This is a description.',
},
{
title: 'Step 2',
subTitle: '00:01:02',
status: 'process',
description: 'This is a description.',
},
{
title: 'Step 3',
subTitle: 'waiting for longlong time',
status: 'wait',
description: 'This is a description.',
},
]"
></a-steps>
<a-steps
v-model:current="current"
type="navigation"
:style="stepStyle"
:items="[
{
status: 'finish',
title: 'Step 1',
},
{
status: 'process',
title: 'Step 2',
},
{
status: 'wait',
title: 'Step 3',
},
{
status: 'wait',
title: 'Step 4',
},
]"
></a-steps>
<a-steps
v-model:current="current"
type="navigation"
size="small"
:style="stepStyle"
:items="[
{
status: 'finish',
title: 'finish 1',
},
{
status: 'finish',
title: 'finish 2',
},
{
status: 'process',
title: 'current process',
},
{
status: 'wait',
title: 'wait',
disabled: true,
},
]"
></a-steps>
</div>
</template>
<script lang="ts" setup>
import { ref } from 'vue';
const current = ref<number>(0);
const stepStyle = {
marginBottom: '60px',
boxShadow: '0px -1px 0 0 #e8e8e8 inset',
};
</script>
如何实现:type="navigation"
vue3示例:
html
<script setup>
import {h, ref} from 'vue';
const current = ref(0)
const description = '关于步骤的描述文本';
const items = [
{
title: '已完成',
description,
},
{
title: '进行中',
description,
subTitle: '副标题',
},
{
title: '待办事项',
description,
},
]
</script>
<template>
<div class="p-28 bg-indigo-50">
<a-steps v-model:current="current" :items="items" status="error"
progress-dot
type="navigation"/>
<div class="container bg-red-300 mt-3 min-h-72 p-3">
{{items[current].title}}
</div>
<div class="container bg-red-300 mt-3 p-3">
<a-button type="primary" @click="current--">上一步</a-button>
<a-button type="primary" @click="current++">下一步</a-button>
</div>
</div>
</template>
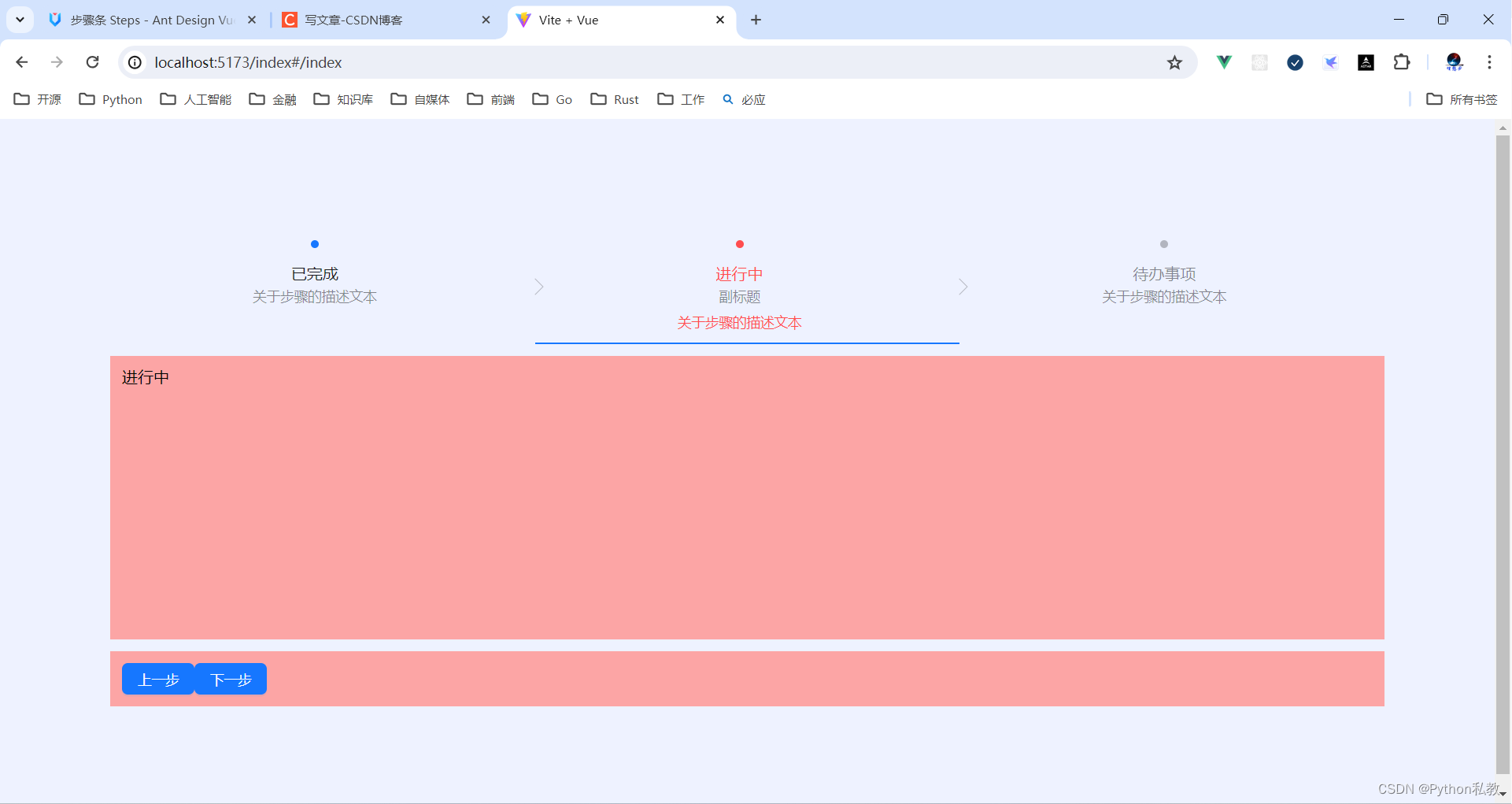
案例:步骤条进度
核心代码:
html
<template>
<a-steps
v-model:current="current"
:percent="60"
:items="[
{
title: 'Finished',
description,
},
{
title: 'In Progress',
subTitle: 'Left 00:00:08',
description,
},
{
title: 'Waiting',
description,
},
]"
></a-steps>
<a-steps
v-model:current="current"
:percent="60"
size="small"
:items="[
{
title: 'Finished',
description,
},
{
title: 'In Progress',
subTitle: 'Left 00:00:08',
description,
},
{
title: 'Waiting',
description,
},
]"
></a-steps>
</template>
<script lang="ts" setup>
import { ref } from 'vue';
const current = ref<number>(1);
const description = 'This is a description.';
</script>
如何实现::percent="60"
vue3示例:
html
<script setup>
import {h, ref} from 'vue';
const current = ref(0)
const description = '关于步骤的描述文本';
const items = [
{
title: '已完成',
description,
},
{
title: '进行中',
description,
subTitle: '副标题',
},
{
title: '待办事项',
description,
},
]
</script>
<template>
<div class="p-28 bg-indigo-50">
<a-steps v-model:current="current" :items="items"
:percent="88"
type="navigation"/>
<div class="container bg-red-300 mt-3 min-h-72 p-3">
{{items[current].title}}
</div>
<div class="container bg-red-300 mt-3 p-3">
<a-button type="primary" @click="current--">上一步</a-button>
<a-button type="primary" @click="current++">下一步</a-button>
</div>
</div>
</template>
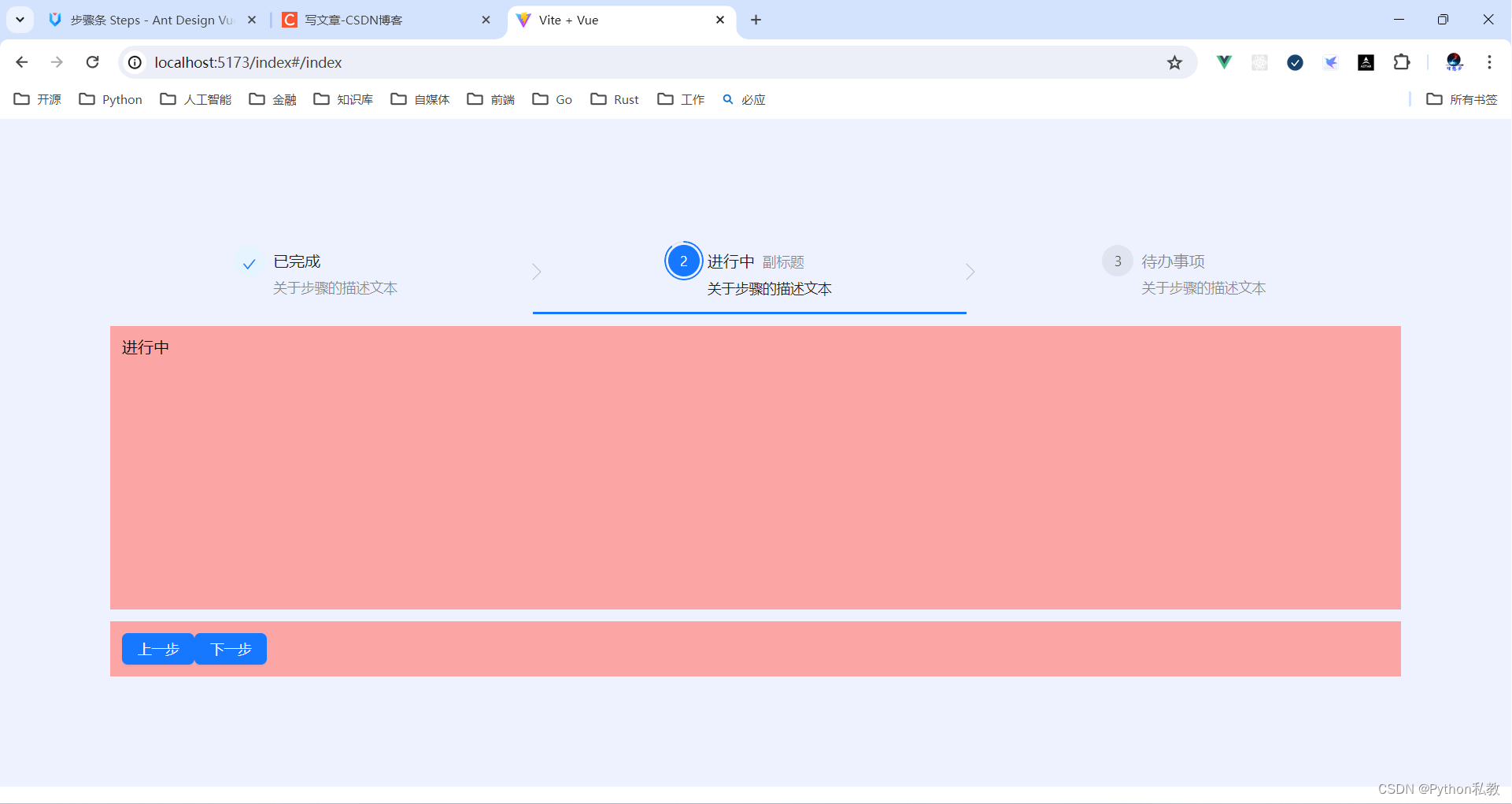
案例:标签位置
核心代码:
html
<template>
<div>
<a-steps :current="1" label-placement="vertical" :items="items" />
<br />
<a-steps :current="1" :percent="60" label-placement="vertical" :items="items" />
<br />
<a-steps :current="1" size="small" label-placement="vertical" :items="items" />
</div>
</template>
<script lang="ts" setup>
import { ref } from 'vue';
const items = ref([
{
title: 'Finished',
description: 'This is a description.',
},
{
title: 'In Progress',
description: 'This is a description.',
},
{
title: 'Waiting',
description: 'This is a description.',
},
]);
</script>
如何实现:label-placement="vertical"
vue3示例:
html
<script setup>
import {h, ref} from 'vue';
const current = ref(0)
const description = '关于步骤的描述文本';
const items = [
{
title: '已完成',
description,
},
{
title: '进行中',
description,
subTitle: '副标题',
},
{
title: '待办事项',
description,
},
]
</script>
<template>
<div class="p-28 bg-indigo-50">
<a-steps v-model:current="current" :items="items"
:percent="88"
label-placement="vertical"
type="navigation"/>
<div class="container bg-red-300 mt-3 min-h-72 p-3">
{{items[current].title}}
</div>
<div class="container bg-red-300 mt-3 p-3">
<a-button type="primary" @click="current--">上一步</a-button>
<a-button type="primary" @click="current++">下一步</a-button>
</div>
</div>
</template>
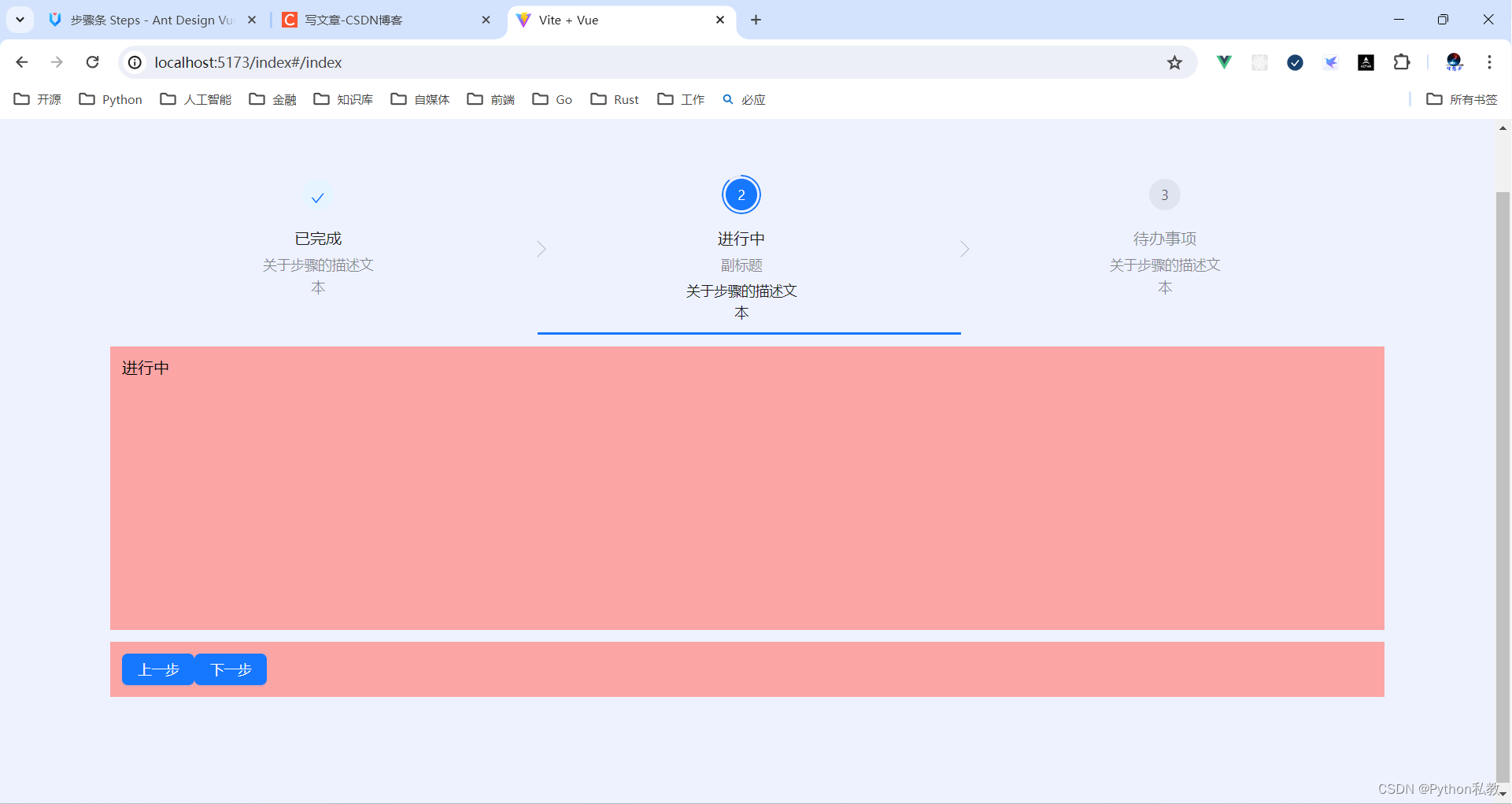
案例:内联步骤条
内联类型的步骤条,适用于列表内容场景中展示对象所在流程、当前状态的情况。
核心代码:
html
<template>
<a-list :data-source="data">
<template #renderItem="{ item }">
<a-list-item>
<a-list-item-meta
description="Ant Design, a design language for background applications, is refined by Ant UED Team"
>
<template #title>
<a href="https://www.antdv.com/">{{ item.title }}</a>
</template>
<template #avatar>
<a-avatar src="https://joeschmoe.io/api/v1/random" />
</template>
</a-list-item-meta>
<a-steps
style="margin-top: 8px"
type="inline"
:current="item.current"
:status="item.status"
:items="items"
/>
</a-list-item>
</template>
</a-list>
</template>
<script lang="ts" setup>
const data = [
{
title: 'Ant Design Title 1',
current: 0,
},
{
title: 'Ant Design Title 2',
current: 1,
status: 'error',
},
{
title: 'Ant Design Title 3',
current: 2,
},
{
title: 'Ant Design Title 4',
current: 1,
},
];
const items = [
{
title: 'Step 1',
description: 'This is a Step 1.',
},
{
title: 'Step 2',
description: 'This is a Step 2.',
},
{
title: 'Step 3',
description: 'This is a Step 3.',
},
];
</script>
属性
整体步骤条。
参数 | 说明 | 类型 | 默认值 | 版本 |
---|---|---|---|---|
current (v-model) | 指定当前步骤,从 0 开始记数。在子 Step 元素中,可以通过 status 属性覆盖状态, 1.5.0 后支持 v-model |
number | 0 | |
direction | 指定步骤条方向。目前支持水平(horizontal )和竖直(vertical )两种方向 |
string | horizontal | |
initial | 起始序号,从 0 开始记数 | number | 0 | |
labelPlacement | 指定标签放置位置,默认水平放图标右侧,可选vertical 放图标下方 |
string | horizontal |
|
percent | 当前 process 步骤显示的进度条进度(只对基本类型的 Steps 生效) |
number | - | 3.0 |
progressDot | 点状步骤条,可以设置为一个 作用域插槽,labelPlacement 将强制为vertical |
Boolean or v-slot:progressDot="{index, status, title, description, prefixCls, iconDot}" | false | |
responsive | 当屏幕宽度小于 532px 时自动变为垂直模式 | boolean | true | 3.0 |
size | 指定大小,目前支持普通(default )和迷你(small ) |
string | default | |
status | 指定当前步骤的状态,可选 wait process finish error |
string | process | |
type | 步骤条类型,有 default 和 navigation 两种 |
string | default |
1.5.0 |
items | 配置选项卡内容 | StepItem[] | [] |
内嵌属性
type="inline"
(4.0+)
参数 | 说明 | 类型 | 默认值 | 版本 |
---|---|---|---|---|
current | 指定当前步骤,从 0 开始记数。在子 Step 元素中,可以通过 status 属性覆盖状态 |
number | 0 | |
initial | 起始序号,从 0 开始记数 | number | 0 | |
status | 指定当前步骤的状态,可选 wait process finish error |
string | process |
|
items | 配置选项卡内容,不支持 icon subtitle |
StepItem | [] |
事件
事件名称 | 说明 | 回调参数 | 版本 | |
---|---|---|---|---|
change | 点击切换步骤时触发 | (current) => void | - | 1.5.0 |
步骤属性
步骤条内的每一个步骤。
参数 | 说明 | 类型 | 默认值 | 版本 |
---|---|---|---|---|
description | 步骤的详情描述,可选 | string | slot | - | |
disabled | 禁用点击 | boolean | false | 1.5.0 |
icon | 步骤图标的类型,可选 | string | slot | - | |
status | 指定状态。当不配置该属性时,会使用 Steps 的 current 来自动指定状态。可选:wait process finish error |
string | wait |
|
subTitle | 子标题 | string | slot | - | 1.5.0 |
title | 标题 | string | slot | - |