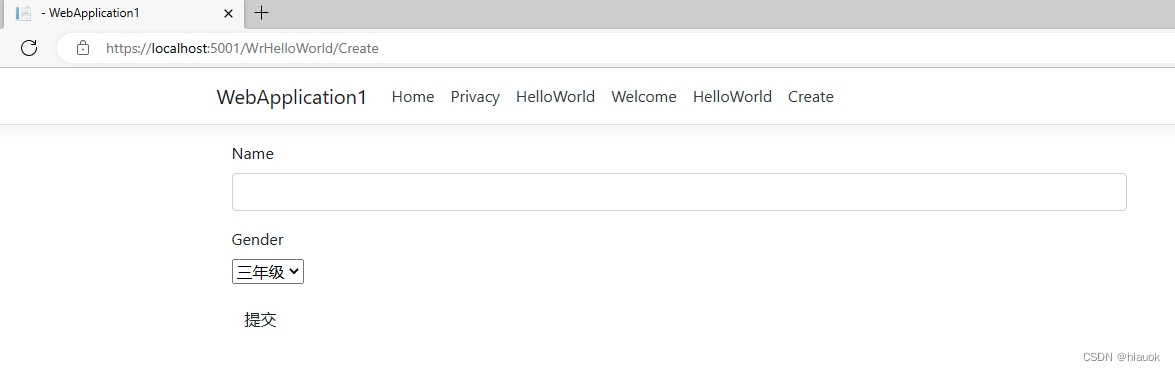
Controllers
WrHelloWorldController.cs
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.Rendering;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace WebApplication1.Controllers
{
public class WrHelloWorldController : Controller
{
// GET: WrHelloWorldController
public ActionResult Index()
{
return View();
}
// GET: WrHelloWorldController/Details/5
public ActionResult Details(int id)
{
return View();
}
// GET: WrHelloWorldController/Create
public ActionResult Create()
{
var plst = new List<object>();
foreach (var x in Enum.GetValues(typeof(Models.Gender)))
{
plst.Add(new
{
ID = Convert.ToInt32(x),
Name = x.ToString()
});
}
SelectList sellist1 = new SelectList(plst, "ID", "Name","3");
ViewData["GenderList"] =sellist1;
return View();
}
// POST: WrHelloWorldController/Create
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create(IFormCollection collection)
{
try
{
return RedirectToAction(nameof(Index));
}
catch
{
return View();
}
}
// GET: WrHelloWorldController/Edit/5
public ActionResult Edit(int id)
{
return View();
}
// POST: WrHelloWorldController/Edit/5
[HttpPost]
[ValidateAntiForgeryToken] //防止跨网站请求伪造令牌
public ActionResult Edit(int id, IFormCollection collection)
{
try
{
return RedirectToAction(nameof(Index));
}
catch
{
return View();
}
}
// GET: WrHelloWorldController/Delete/5
public ActionResult Delete(int id)
{
return View();
}
// POST: WrHelloWorldController/Delete/5
[HttpPost]
[ValidateAntiForgeryToken] //防止跨网站请求伪造令牌
public ActionResult Delete(int id, IFormCollection collection)
{
try
{
return RedirectToAction(nameof(Index));
}
catch
{
return View();
}
}
}
}
Models
StudentViewModel.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Threading.Tasks;
namespace WebApplication1.Models
{
public class StudentViewModel
{
public string Name { get; set; }
public string Age { get; set; }
public string Height { get; set; }
[EnumDataType(typeof(Gender))]
public Gender Gender { get; set; }
}
public enum Gender
{
一年级=1,
二年级=2,
三年级=3,
四年级=4,
五年级=5,
六年级=6
}
}
Views
Create.cshtml
@model WebApplication1.Models.StudentViewModel
@using (Html.BeginForm("Create", "WrHelloWorld", FormMethod.Post, new { @class = "form-horizontal", role = "form" }))
{
@Html.AntiForgeryToken()
<div class="form-group">
@Html.LabelFor(model => model.Name, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.TextBoxFor(model => model.Name, null, htmlAttributes: new { @class = "form-control" })
@Html.ValidationMessageFor(model => model.Name, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Gender, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.DropDownList("3",ViewData["GenderList"] as SelectList)
@Html.ValidationMessageFor(model => model.Gender, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="提交" class="btn btn-default" />
</div>
</div>
}