-
类类型属性赋值 : 在XML配置中,可以使用
<property>
元素为类类型属性赋值。通过指定属性的名称和值,可以直接将其他Bean的实例引用注入到目标Bean的属性中。这种方式可以建立对象之间的关联关系。例如,可以将一个Address对象注入到Person对象的address属性中。 -
数组类型属性赋值 : XML配置中,可以使用
<property>
元素为数组类型属性赋值。通过使用<array>
或<list>
元素,可以定义一个数组或列表,并使用<value>
元素指定数组或列表的元素值。这样可以将多个值一次性赋给数组类型的属性。 -
集合类型属性赋值 : 在XML配置中,可以使用
<property>
元素为集合类型属性赋值。通过使用<list>
、<set>
、<map>
或<props>
元素,可以定义一个列表、集合、映射或属性集,并使用相应的元素指定集合的元素值。这样可以一次性赋给集合类型的属性。
类类型属性赋值
类类型属性赋值是指将一个类的实例赋值给另一个类的属性。在Java中,类类型属性是指一个类中的属性的类型是另一个类。
我们创建如下类:
java
public class Clazz {
private Integer clazzId;
private String clazzName;
public Integer getClazzId() {
return clazzId;
}
public void setClazzId(Integer clazzId) {
this.clazzId = clazzId;
}
public String getClazzName() {
return clazzName;
}
public void setClazzName(String clazzName) {
this.clazzName = clazzName;
}
@Override
public String toString() {
return "Clazz{" +
"clazzId=" + clazzId +
", clazzName='" + clazzName + '\'' +
'}';
}
public Clazz() {
}
public Clazz(Integer clazzId, String clazzName) {
this.clazzId = clazzId;
this.clazzName = clazzName;
}
}
然后创建一个Student类
java
public class student{
private Integer stundentId;
private String studentName;
private Clazz clazz;
public Integer getStundentId() {
return stundentId;
}
public void setStundentId(Integer stundentId) {
this.stundentId= stundentId;
}
public String getStudentName() {
return clazzName;
}
public void setClazzName(String studentName) {
this.studentName= studentName;
}
public Clazz getClazz() {
return clazz;
}
public void setClazz(Clazz clazz) {
this.clazz = clazz;
}
}
方式一: 引用外部已经声明的bean
这个是在我们的spring的applicationContext.xml文件中配置的
配置Clazz类型的bean:
xml
<bean id="clazzOne" class="com.miaow.spring.bean.Clazz">
<property name="clazzId" value="1111"></property>
<property name="clazzName" value="财源滚滚班"></property>
</bean>
为Student中的Clazz属性赋值:
xml
<bean id="studentFour" class="com.miaow.spring.bean.Student">
<property name="studentId" value="1004"></property>
<property name="studentName" value="赵六"></property>
<!-- ref属性:引用IOC容器中某个bean的id,将所对应的bean为属性赋值 -->
<property name="clazz" ref="clazzOne"></property>
</bean>
错误演示
java
<bean id="studentFour" class="com.miaow.spring.bean.Student">
<property name="studentId" value="1004"></property>
<property name="studentName" value="赵六"></property>
<property name="clazz" value="clazzOne"></property>
</bean>
如果错把ref属性写成了value属性,会抛出异常: Caused by: java.lang.IllegalStateException:Cannot convert value of type 'java.lang.String' to required type 'com.miaow.spring.bean.Clazz' for property 'clazz': no matching editors or conversion strategy found
意思是不能把String类型转换成我们要的Clazz类型,说明我们使用value属性时,Spring只把这个属性看做一个普通的字符串,不会认为这是一个bean的id,更不会根据它去找到bean来赋值
内部bean的方式
同样的在applicationContext.xml文件中配置如下代码:
xml
<bean id="studentFour" class="com.miaow.spring.bean.Student">
<property name="studentId" value="1004"></property>
<property name="studentName" value="赵六"></property>
<property name="clazz">
<!-- 在一个bean中再声明一个bean就是内部bean -->
<!-- 内部bean只能用于给属性赋值,不能在外部通过IOC容器获取,因此可以省略id属性 -->
<bean id="clazzInner" class="com.miaow.spring.bean.Clazz">
<property name="clazzId" value="2222"></property>
<property name="clazzName" value="远大前程班"></property>
</bean>
</property>
</bean>
首先,通过 <bean>
标签定义了一个名为 studentFour 的Bean,并指定了它的类为 com.miaow.spring.bean.Student。
在 <bean>
标签中,使用 <property>
标签为 studentFour Bean 的属性赋值。其中,name 属性指定了要赋值的属性名,value 属性指定了要赋的值。
在 <property>
标签中,使用 <bean>
标签定义了一个内部Bean,即 clazzInner。内部Bean的类为 com.miaow.spring.bean.Clazz。
在 <bean>
标签中,使用 <property>
标签为 clazzInner Bean 的属性赋值。同样,使用 name 属性指定了要赋值的属性名,value 属性指定了要赋的值。
级联属性赋值
在Spring中,级联属性赋值是指在给一个对象的属性赋值时,同时给该对象的属性的属性(即子属性)赋值。这样可以方便地一次性设置多层嵌套属性的值。
xml
<bean id="studentFour" class="com.miaow.spring.bean.Student">
<property name="studentId" value="1004"></property>
<property name="studentName" value="赵六"></property>
<!-- 一定先引用某个bean为属性赋值,才可以使用级联方式更新属性 -->
<property name="clazz" ref="clazzOne"></property>
<property name="clazz.clazzId" value="3333"></property>
<property name="clazz.clazzName" value="最强王者班"></property>
</bean>
首先,通过 <bean>
标签定义了一个名为 studentFour 的Bean,并指定了它的类为 com.miaow.spring.bean.Student。
然后,使用 <property>
标签为 studentFour Bean 的属性赋值。其中,name 属性指定了要赋值的属性名,value 属性指定了要赋的值。
接下来,通过 <property>
标签引用了一个名为 clazzOne 的Bean,并将其赋值给 studentFour 的 clazz 属性。这是级联属性赋值的前提,需要先引用某个Bean为属性赋值。
最后,使用级联属性赋值的方式,通过在 <property>
标签中使用点号 . 来表示级联属性的层级关系,设置了 studentFour 的 clazz 对象的 clazzId 和 clazzName 属性的值。
联机属性测试方法:
java
@Test
public void CascadeValueTest(){
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Student student = (Student) context.getBean("studentFive");
System.out.println(student);
System.out.println(student.getClassz().toString());
}
数组类型属性赋值的方式
在Spring的XML配置文件中,可以使用 或 元素为数组类型属性赋值。以下是两种常见的方式:
使用 <array>
元素:
在Student中添加了如下代码:
java
private String[] hobbies;
public String[] getHobbies() {
return hobbies;
}
public void setHobbies(String[] hobbies) {
this.hobbies = hobbies;
}
在XML文件中新增如下
xml
<property name="hobbies">
<array>
<value>吃饭</value>
<value>玩游戏</value>
<value>打麻将</value>
</array>
</property>
使用 <list>
进行赋值
xml
<property name="hobbies">
<list>
<value>吃饭</value>
<value>玩游戏</value>
<value>打麻将</value>
</list>
</property>
在上述示例中,通过 <list>
元素包裹多个<value>
元素,每个 <value>
元素表示数组中的一个元素。
无论是使用 <list>
还是 <array>
元素,都可以在其中添加多个 <value>
元素来定义数组的元素。每个 <value>
元素的内容即为数组的一个元素的值。
为集合类型属性赋值
为List集合类型属性赋值
在Clazz中添加如下代码:
java
private List<Student> students;
public List<Student> getStudents() {
return students;
}
public void setStudents(List<Student> students) {
this.students = students;
}
配置bean:
xml
<bean id="clazzTwo" class="com.miaow.spring.bean.Clazz">
<property name="clazzId" value="4444"></property>
<property name="clazzName" value="Javaee0222"></property>
<property name="students">
<list>
<ref bean="studentOne"></ref>
<ref bean="studentTwo"></ref>
<ref bean="studentThree"></ref>
</list>
</property>
</bean>
<bean id="studentOne" class="com.miaow.spring.bean.Student">
<property name="studentId" value="1004"></property>
<property name="studentName" value="赵六"></property>
</bean>
<bean id="studentTwo" class="com.miaow.spring.bean.Student">
<property name="studentId" value="1005"></property>
<property name="studentName" value="赵七"></property>
</bean>
<bean id="studentThree" class="com.miaow.spring.bean.Student">
<property name="studentId" value="1006"></property>
<property name="studentName" value="王五"></property>
</bean>
若为Set集合类型属性赋值,只需要将其中的list标签改为set标签即可
为Map集合类型属性赋值
我们创建一个新类Teacher
java
public class Teacher {
private Integer teacherId;
private String teacherName;
public Integer getTeacherId() {
return teacherId;
}
public void setTeacherId(Integer teacherId) {
this.teacherId = teacherId;
}
public String getTeacherName() {
return teacherName;
}
public void setTeacherName(String teacherName) {
this.teacherName = teacherName;
}
public Teacher(Integer teacherId, String teacherName) {
this.teacherId = teacherId;
this.teacherName = teacherName;
}
public Teacher() {
}
@Override
public String toString() {
return "Teacher{" +
"teacherId=" + teacherId +
", teacherName='" + teacherName + '\'' +
'}';
}
}
在Student类中添加如下代码:
java
private Map<String, Teacher> teacherMap;
public Map<String, Teacher> getTeacherMap() {
return teacherMap;
}
public void setTeacherMap(Map<String, Teacher> teacherMap) {
this.teacherMap = teacherMap;
}
在bean中配置
xml
<bean id="teacherOne" class="com.miaow.spring.bean.Teacher">
<property name="teacherId" value="10010"></property>
<property name="teacherName" value="大宝"></property>
</bean>
<bean id="teacherTwo" class="com.miaow.spring.bean.Teacher">
<property name="teacherId" value="10086"></property>
<property name="teacherName" value="二宝"></property>
</bean>
<bean id="studentFour" class="com.miaow.spring.bean.Student">
<property name="studentId" value="1004"></property>
<property name="studentName" value="赵六"></property>
<!-- ref属性:引用IOC容器中某个bean的id,将所对应的bean为属性赋值 -->
<property name="clazz" ref="clazzOne"></property>
<property name="hobbies">
<array>
<value>吃饭</value>
<value>玩游戏</value>
<value>打麻将</value>
</array>
</property>
<property name="teacherMap">
<map>
<entry>
<key>
<value>10010</value>
</key>
<ref bean="teacherOne"></ref>
</entry>
<entry>
<key>
<value>10086</value>
</key>
<ref bean="teacherTwo"></ref>
</entry>
</map>
</property>
</bean>
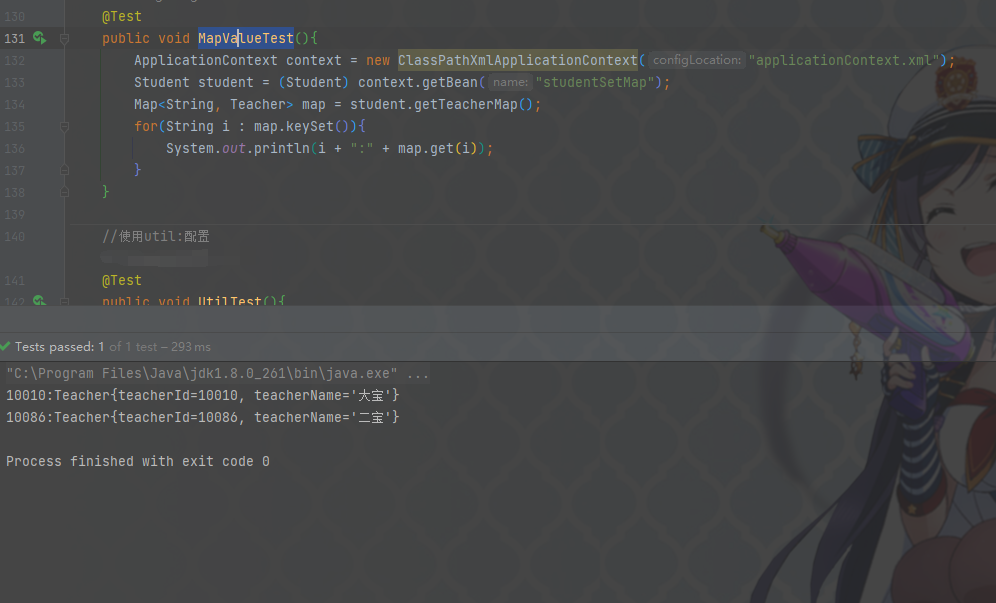
引用集合类型的bean
xml
<!--list集合类型的bean-->
<util:list id="students">
<ref bean="studentOne"></ref>
<ref bean="studentTwo"></ref>
<ref bean="studentThree"></ref>
</util:list>
<!--map集合类型的bean-->
<util:map id="teacherMap">
<entry>
<key>
<value>10010</value>
</key>
<ref bean="teacherOne"></ref>
</entry>
<entry>
<key>
<value>10086</value>
</key>
<ref bean="teacherTwo"></ref>
</entry>
</util:map>
<bean id="clazzTwo" class="com.miaow.spring.bean.Clazz">
<property name="clazzId" value="4444"></property>
<property name="clazzName" value="Javaee0222"></property>
<property name="students" ref="students"></property>
</bean>
<bean id="studentFour" class="com.miaow.spring.bean.Student">
<property name="studentId" value="1004"></property>
<property name="studentName" value="赵六"></property>
<!-- ref属性:引用IOC容器中某个bean的id,将所对应的bean为属性赋值 -->
<property name="clazz" ref="clazzOne"></property>
<property name="hobbies">
<array>
<value>抽烟</value>
<value>喝酒</value>
<value>烫头</value>
</array>
</property>
<property name="teacherMap" ref="teacherMap"></property>
</bean>
使用util:list、util:map标签必须引入相应的命名空间,可以通过idea的提示功能选择
- 引用顺序 : 在使用
<util:list>
引用集合类型时,确保被引用的Bean已经在配置文件中定义并初始化。否则,可能会导致引用失败或引用到空集合。 - 集合类型Bean的命名 : 当使用
<util:list>
引用集合类型时,确保为集合类型的Bean提供唯一的ID。这样可以确保在引用时能够准确地指定要引用的Bean。 - 集合类型Bean的作用域: 集合类型的Bean的作用域通常是单例(singleton)。确保在引用集合类型的Bean时,了解其作用域,并确保在适当的范围内引用。