1 使用GraphQLObjectType 定义type(类型)
不使用ConstructingTypes定义方式如下:
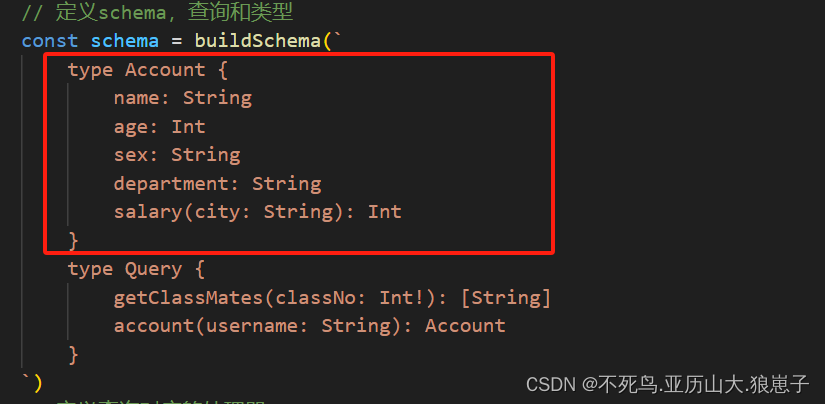
使用ConstructingTypes定义方式如下:
更接近于构造函数方式
var AccountType = new graphql.GraphQLObjectType({
name: 'Account',
fields: {
name: { type: graphql.GraphQLString },
age: { type: graphql.GraphQLInt },
sex: { type: graphql.GraphQLString },
department: { type: graphql.GraphQLString }
}
});
2 使用GraphQLObjectType 定义Query(查询)
不使用ConstructingTypes定义方式如下:
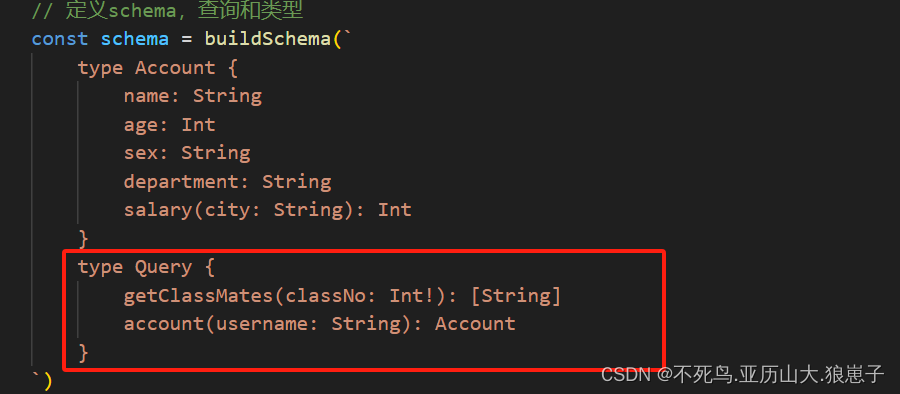
使用ConstructingTypes定义方式如下:
var queryType = new graphql.GraphQLObjectType({
name: 'Query',
fields: {
account: {
type: AccountType,
// `args` describes the arguments that the `user` query accepts
args: {
username: { type: graphql.GraphQLString }
},
resolve: function (_, { username }) {
const name = username;
const sex = 'man';
const age = 18;
const department = '开发部';
return {
name,
sex,
age,
department
}
}
}
}
});
3 创建schema
var schema = new graphql.GraphQLSchema({ query: queryType });
4 代码实现如下
const express = require('express');
const graphql = require('graphql');
const grapqlHTTP = require('express-graphql').graphqlHTTP;
var AccountType = new graphql.GraphQLObjectType({
name: 'Account',
fields: {
name: { type: graphql.GraphQLString },
age: { type: graphql.GraphQLInt },
sex: { type: graphql.GraphQLString },
department: { type: graphql.GraphQLString }
}
});
var queryType = new graphql.GraphQLObjectType({
name: 'Query',
fields: {
account: {
type: AccountType,
// `args` describes the arguments that the `user` query accepts
args: {
username: { type: graphql.GraphQLString }
},
resolve: function (_, { username }) {
const name = username;
const sex = 'man';
const age = 18;
const department = '开发部';
return {
name,
sex,
age,
department
}
}
}
}
});
var schema = new graphql.GraphQLSchema({ query: queryType });
const app = express();
app.use('/graphql', grapqlHTTP({
schema: schema,
graphiql: true
}))
// 公开文件夹,供用户访问静态资源
app.use(express.static('public'))
app.listen(3000);