1、查找轮廓随机用不同的颜色画出
python
import cv2
import numpy as np
def get_contour_colors(num_contours):
# 定义颜色表 (BGR 格式)
colors = [
(255, 0, 0),
(255, 50, 0),
(255, 100, 0),
(255, 150, 0),
(255, 200, 0),
(255, 255, 0),
(200, 255, 0),
(150, 255, 0),
(100, 255, 0),
(50, 255, 0),
(0, 255, 0),
(0, 255, 50),
(0, 255, 100),
(0, 255, 150),
(0, 255, 200),
(0, 255, 255),
(0, 200, 255),
(0, 150, 255),
(0, 100, 255),
(0, 50, 255),
(0, 0, 255),
]
# 返回一个颜色表
return [colors[i % len(colors)] for i in range(num_contours)]
def fill_contours(img, contours):
# 创建空白的图像,用来画轮廓表
filled_img = np.zeros_like(img)
num_contours = len(contours)
# 颜色表
colors = get_contour_colors(num_contours)
for i, contour in enumerate(contours):
cv2.drawContours(filled_img, [contour], -1, colors[i], -1)
return filled_img
# 读取原图,原图是RGB图
img = cv2.imread('1.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 查找轮廓
contours, _ = cv2.findContours(gray, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_NONE)
# 填充轮廓
filled_img = fill_contours(img, contours)
cv2.imshow('Filled Contours', filled_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
原图:
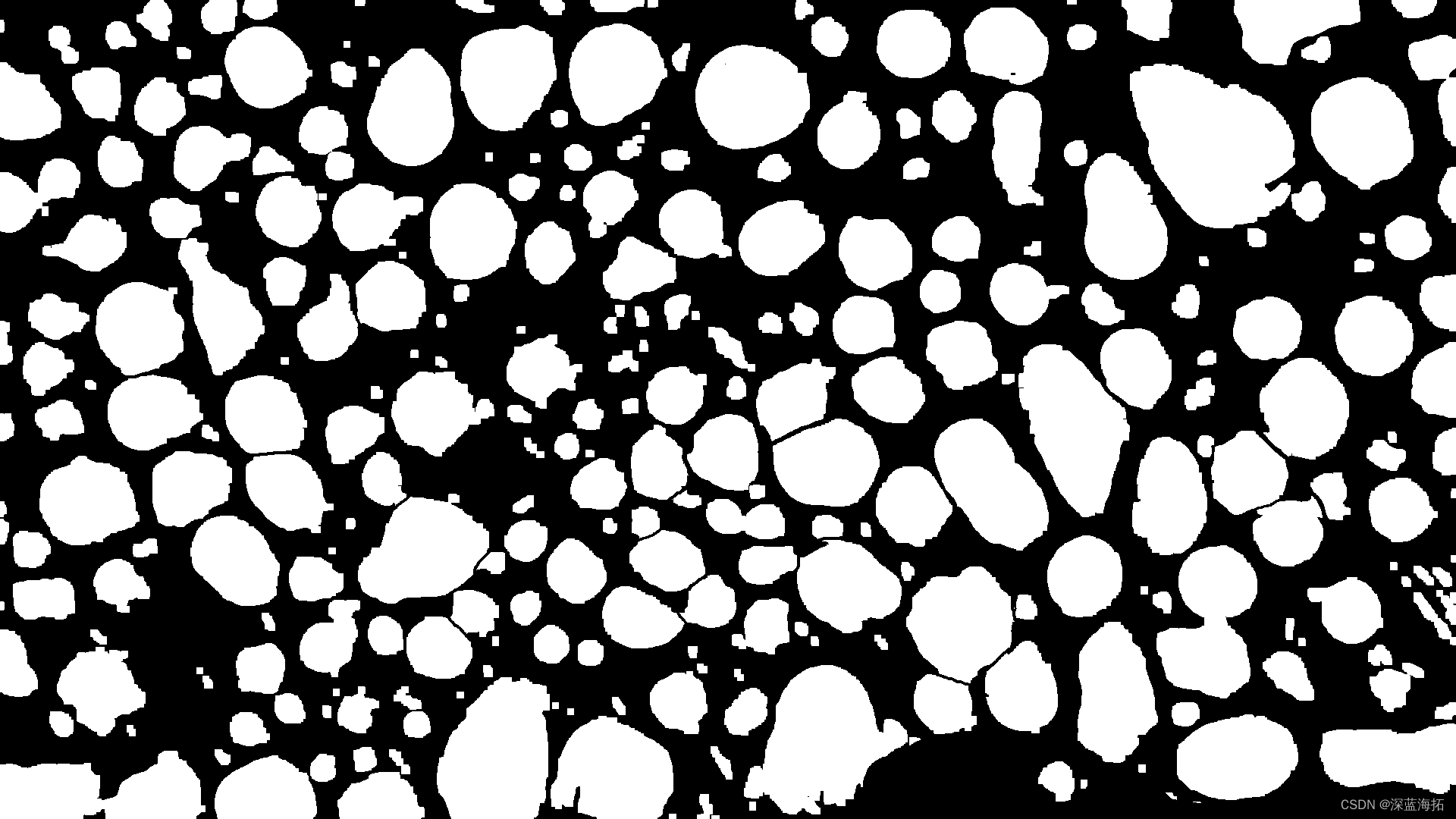
输出:
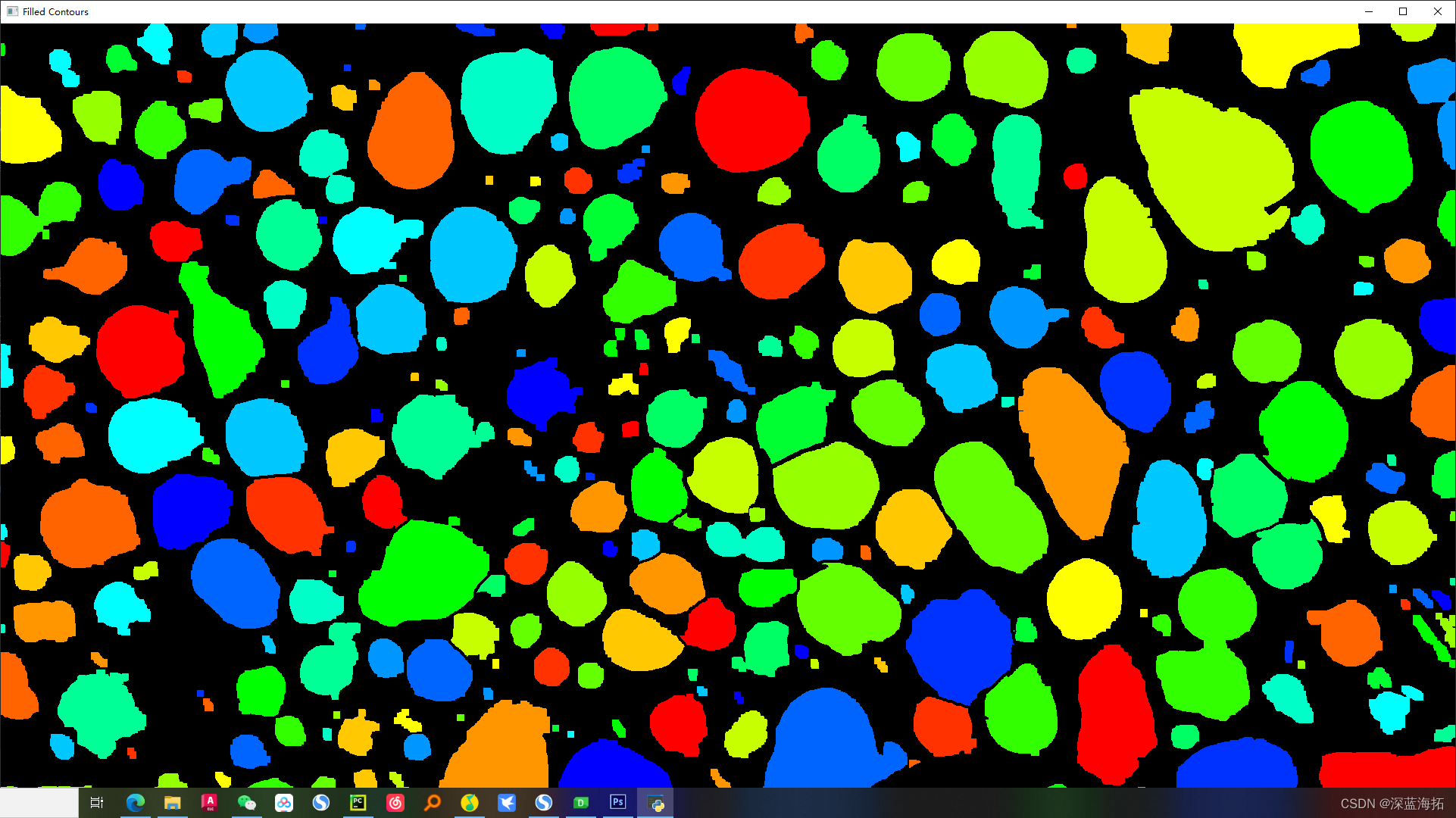
2、查找轮廓按大小用不同的颜色画出
python
import cv2
import numpy as np
def get_color(k, start_color=(255, 0, 0), end_color=(0, 0, 255)):
out_color = [] # 输出的颜色
for i in range(3):
single_color = int((end_color[i] - start_color[i]) * k + start_color[i])
out_color.append(single_color)
for j in range(3):
if out_color[j] > 255:
out_color[j] = 255
elif out_color[j] < 0:
out_color[j] = 0
return out_color
def fill_contours(img, contours):
# 创建空白的图像,用来画轮廓表
filled_img = np.zeros_like(img)
# num_contours = len(contours)
dims = [] # 所有的轮廓的尺寸
for contour in contours:
rect = cv2.minAreaRect(contour) # 获取最小外接矩形
dia = rect[1][0] if rect[1][0] <= rect[1][1] else rect[1][1] # 计算轮廓的最短尺寸,并获取直径
dims.append(dia)
max_dim = max(dims) # 最大尺寸
min_dim = min(dims) # 最小尺寸
range_dim = max_dim - min_dim # 尺寸范围
for i, contour in enumerate(contours):
rect = cv2.minAreaRect(contour) # 获取最小外接矩形
dia = rect[1][0] if rect[1][0] <= rect[1][1] else rect[1][1] # 计算轮廓的最短尺寸,并获取直径
k = (dia - min_dim) / range_dim # 尺寸比例
color = get_color(k) # 获取颜色
cv2.drawContours(filled_img, [contour], -1, color, -1)
return filled_img
# 读取原图,原图是RGB图
img = cv2.imread('1.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 查找轮廓
contours, _ = cv2.findContours(gray, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_NONE)
# 填充轮廓
filled_img = fill_contours(img, contours)
cv2.imshow('Filled Contours', filled_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
输出:
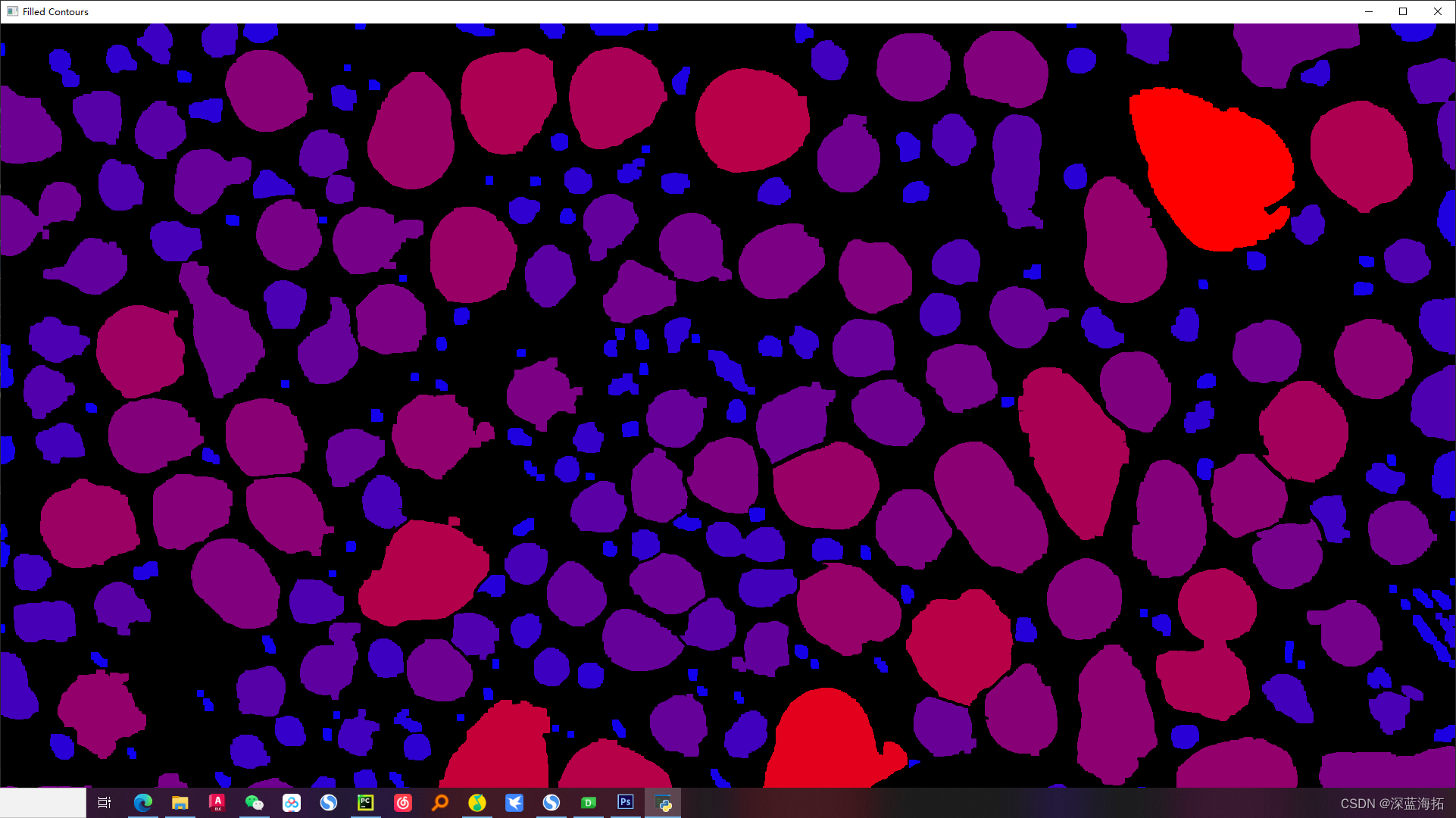