C# 模式匹配
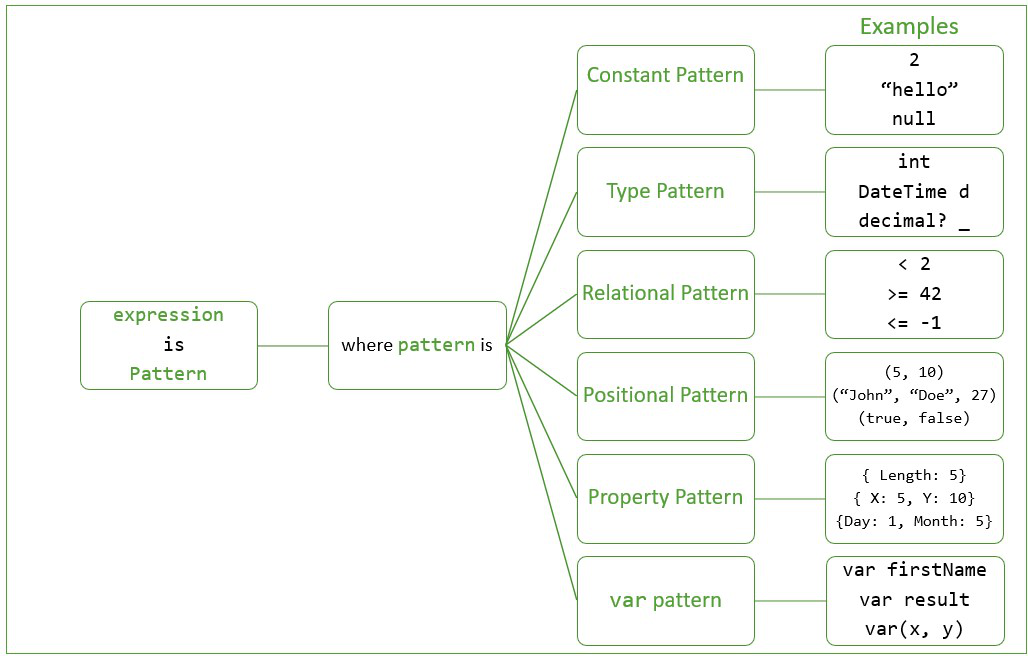
https://www.geeksforgeeks.org/pattern-matching-in-c-sharp/
https://www.codeproject.com/Articles/5368148/Your-Quick-Guide-to-Pattern-Matching-in-Csharp
什是模式匹配
模式匹配是一种在代码中识别和提取数据的机制。它允许您以声明式的方式检查对象的形状,而无需编写冗长的条件语句。
模式匹配的优势
- Type-Testing 类型测试
- Nullable-type checking 可空类型检查
- Type casting and assignment 类型转换和分配
- High readability 可读性高
- Concise syntax 简洁的语法
- Less convoluted code 代码更简洁
模式匹配的主要特性包括
- 类型模式:检查对象是否属于特定的类型。
- 属性模式:检查对象是否具有特定的属性。
- 位置模式:检查对象的具体结构。
- var 模式:将匹配的值存储在变量中以供后续使用。
- 恒定模式 :与常量值进行测试,常量值可以包括
int
、float
、char
、string
、bool
、,用const
、null
声明的字段。 - 弃元模式 :与 discard 运算符
_
的模式匹配,匹配任何内容,包括null
,它的用法在新的开关表达式中大放异彩,以匹配默认或否则情况。 - 元组模式:从位置模式的特殊派生,您可以在其中测试同一表达式中类型的多个属性。
- 列表模式:列表匹配模式是 C# 中模式匹配的最新补充,使用列表模式,您可以将列表或数组与一组顺序元素进行匹配。
模式匹配的主要应用场景
- 类型判断和转换 :
- 替代传统的
is
和as
操作符,提供更简洁的语法。 - 在
switch
语句中进行类型匹配。
- 替代传统的
- 属性检查 :
- 检查对象是否具有某些属性,并基于属性值采取不同的操作。
- 在
if
语句中进行属性模式匹配。
- 数据解构 :
- 对元组、记录类型等复杂数据结构进行解构,提取感兴趣的部分。
- 在
var
模式中使用位置模式匹配。
- 列表和数组处理 :
- 使用模式匹配检查列表或数组的长度和元素位置。
- 在
switch
语句中对列表进行模式匹配。
- 错误处理 :
- 在
try-catch
语句中使用模式匹配捕获特定类型的异常。 - 在
switch
语句中对异常类型进行模式匹配。
- 在
- 领域驱动设计 :
- 使用模式匹配表达领域概念,提高代码的可读性和可维护性。
- 在领域模型中使用模式匹配进行数据验证和转换。
示例
-
类型判断和转换:
csharpobject obj = "Hello, world!"; if (obj is string s) { Console.WriteLine(s.ToUpper()); // 输出: HELLO, WORLD! } switch (obj) { case string s: Console.WriteLine($"It's a string: {s}"); break; case int i: Console.WriteLine($"It's an integer: {i}"); break; default: Console.WriteLine("It's something else"); break; }
-
属性检查:
csharpvar person = new { Name = "Alice", Age = 30 }; if (person is { Age: >= 18 }) { Console.WriteLine($"{person.Name} is an adult."); }
-
数据解构:
csharpvar point = (10, 20); if (point is (int x, int y)) { Console.WriteLine($"X: {x}, Y: {y}"); } var list = new List<int> { 1, 2, 3, 4, 5 }; if (list is [_, _, int middle, _, _]) { Console.WriteLine($"The middle element is: {middle}"); }
-
列表和数组处理:
csharpvar numbers = new List<int> { 1, 2, 3, 4, 5 }; switch (numbers) { case []: Console.WriteLine("The list is empty."); break; case [int first]: Console.WriteLine($"The list has one element: {first}"); break; case [int first, int second]: Console.WriteLine($"The list has two elements: {first}, {second}"); break; case var all: Console.WriteLine($"The list has {all.Count} elements."); break; }
-
错误处理:
csharptry { // 某些可能引发异常的操作 } catch (DivideByZeroException ex) { Console.WriteLine($"Caught DivideByZeroException: {ex.Message}"); } catch (Exception ex) { Console.WriteLine($"Caught general Exception: {ex.Message}"); }
-
领域驱动设计:
csharppublic record Person(string Name, int Age); var person = new Person("Alice", 30); if (person is { Age: >= 18 }) { Console.WriteLine($"{person.Name} is an adult."); }
-
恒定模式:
c#public bool IsFreshProduce(FoodModel food) { return food?.Category?.ID is (int) ProductCategoryEnum.FreshProduce; }
-
弃元模式:
c#//如果未提供食物的储存温度或数字与以下范围不匹配,则将引发自定义异常 public int GetFoodStorageTemperature (StorageRequirementEnum storageRequirement) => storageRequirement switch { StorageRequirementEnum.Freezer => -18, StorageRequirementEnum.Refrigerator => 4, StorageRequirementEnum.RoomTemperature => 25, _ => throw new InvalidStorageRequirementException() }; // switch (storageRequirement) // { // case StorageRequirementEnum.Freezer: // return -18; // case StorageRequirementEnum.Refrigerator: // return 4; // case StorageRequirementEnum.RoomTemperature: // return 25; // default: // throw new Exception(); // }
-
元组模式:
c#//转基因食品检测 public string GetFoodDescription(FoodModel food) => (food.NonGMO, food.Category.ID) switch { (true, (int)ProductCategoryEnum.FreshProduce) => "Non-GMO Fresh Product", (true, (int)ProductCategoryEnum.Dairy) => "Non-GMO Dairy", (false, (int)ProductCategoryEnum.Meats) => "GMO Meats. Avoid!", (_, _) => "Invalid Food Group" }; // switch (food.NonGMO, food.Category.ID) // { // case (true, (int)ProductCategoryEnum.FreshProduce): // return "Non-GMO Fresh Product"; // case (true, (int)ProductCategoryEnum.Dairy): // return "Non-GMO Dairy"; // case (false, (int)ProductCategoryEnum.Meats): // return "GMO Meats. Avoid!"; // default: // return "Invalid Food Group"; // }
-
列表模式:
c#
public (int?, int?) FindNumberOneAndNumberFour()
{
int[] numbers = { 1, 2, 3, 4, 5 };
// Match if 2nd value is anything, 3rd is greater than or equal 3, fifth is 5
if (numbers is [var numberOne, _, >= 3, int numberFour, 5])
{
return (numberOne, numberFour);
}
return (null, null);
}
这些案例展示了模式匹配在各种场景下的应用,包括类型判断、属性检查、数据解构、列表处理、错误处理以及领域建模等。通过使用模式匹配,您可以编写更简洁、可读性更强的代码,同时也能在某些情况下提高性能。熟练掌握这些模式匹配的使用技巧,将有助于您提高代码质量和开发效率。
获得永久免费的无限 GPT 查询次数 ,点击链接并下载Monica插件,即可参加限时活动。
https://monica.im/invitation?c=OWBJ2ZBE