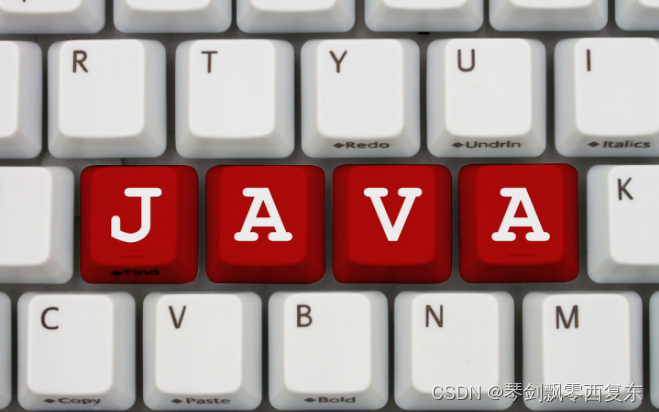
注解简介
在今天的每日一注解中,我们将探讨@ResponseStatus
注解。@ResponseStatus
是Spring框架中的一个注解,用于为控制器方法指定HTTP响应状态码和理由短语。
注解定义
@ResponseStatus
注解用于标记控制器方法或异常类,以指示HTTP响应的状态码和理由短语。以下是一个基本的示例:
java
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MyController {
@GetMapping("/notfound")
@ResponseStatus(HttpStatus.NOT_FOUND)
public String notFound() {
return "Resource not found";
}
}
在这个示例中,当访问/notfound
路径时,Spring会返回404状态码,并在响应体中包含"Resource not found"消息。
注解详解
@ResponseStatus
注解可以用来为控制器方法或自定义异常类指定HTTP响应状态码和理由短语。这在需要返回特定HTTP状态码时非常有用。
- value: 指定HTTP状态码。
- reason: 指定理由短语(可选)。
使用场景
@ResponseStatus
广泛用于Spring MVC应用程序中,用于设置HTTP响应状态码。例如,处理资源未找到、权限不足、服务器内部错误等情况。
示例代码
以下是一个使用@ResponseStatus
注解的代码示例,展示了如何处理自定义异常并返回特定的HTTP状态码:
java
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MyController {
@GetMapping("/resource")
public String getResource() {
if (resourceNotFound()) {
throw new ResourceNotFoundException();
}
return "Resource content";
}
private boolean resourceNotFound() {
// 模拟资源未找到的情况
return true;
}
@ExceptionHandler(ResourceNotFoundException.class)
@ResponseStatus(HttpStatus.NOT_FOUND)
public String handleResourceNotFound(ResourceNotFoundException e) {
return e.getMessage();
}
}
@ResponseStatus(value = HttpStatus.NOT_FOUND, reason = "Resource Not Found")
class ResourceNotFoundException extends RuntimeException {
public ResourceNotFoundException() {
super("Resource not found");
}
}
在这个示例中,当getResource
方法检测到资源未找到时,会抛出ResourceNotFoundException
异常,并返回404状态码和相应的错误消息。
常见问题
问题:如何在返回响应时设置自定义理由短语?
解决方案 :可以在@ResponseStatus
注解中使用reason
属性设置自定义理由短语。
java
@ResponseStatus(value = HttpStatus.FORBIDDEN, reason = "Access Denied")
public class AccessDeniedException extends RuntimeException {
public AccessDeniedException() {
super("Access denied");
}
}
问题:如何处理HTTP状态码的全局异常?
解决方案 :可以使用@ControllerAdvice
和@ExceptionHandler
注解全局处理特定异常,并返回相应的HTTP状态码。
java
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(AccessDeniedException.class)
@ResponseStatus(HttpStatus.FORBIDDEN)
public String handleAccessDeniedException(AccessDeniedException e) {
return e.getMessage();
}
}
小结
通过今天的学习,我们了解了@ResponseStatus
的基本用法和应用场景。明天我们将探讨另一个重要的Spring注解------@ControllerAdvice
。
相关链接
希望这个示例能帮助你更好地理解和应用@ResponseStatus
注解。如果有任何问题或需要进一步的帮助,请随时告诉我。