【简要介绍】
在OpenCVSharp中,FileStorage
类用于将数据(包括OpenCV的Mat
类型数据)序列化为XML或YAML格式的文件,以及从这些文件中反序列化数据。以下是关于FileStorage
类用法的详细说明:
写入数据(序列化)
-
创建FileStorage对象 :
使用
FileStorage
类的构造函数创建一个新的FileStorage
对象,并指定文件名和模式(写入或读取)。csharp复制代码
|---|---------------------------------------------------------------------------|
| |FileStorage fs = new FileStorage("output.xml", FileStorage.Mode.Write);
| -
写入数据 :
使用
Write
方法将数据写入文件。对于基本数据类型(如int
,float
,string
),可以直接写入。对于Mat
类型的数据,需要指定一个名称作为键。csharp复制代码
|---|---------------------------------------------------|
| |fs.Write("int_value", 123);
|
| |fs.Write("float_value", 3.14f);
|
| |fs.Write("mat_name", myMat); // 假设myMat是一个Mat对象
| -
释放资源 :
在写入完成后,使用
Release
方法释放FileStorage
对象占用的资源。csharp复制代码
|---|-----------------|
| |fs.Release();
|
读取数据(反序列化)
-
创建FileStorage对象 :
与写入类似,但这次需要指定模式为读取。
csharp复制代码
|---|--------------------------------------------------------------------------|
| |FileStorage fs = new FileStorage("output.xml", FileStorage.Mode.Read);
| -
读取数据 :
使用索引器
[]
通过键名来访问数据。对于Mat
类型的数据,可以直接将其转换为Mat
对象。csharp复制代码
|---|-------------------------------------------------|
| |int intValue = (int)fs["int_value"];
|
| |float floatValue = (float)fs["float_value"];
|
| |Mat loadedMat = (Mat)fs["mat_name"];
|注意:键名(如"int_value"、"float_value"、"mat_name")必须与写入时使用的名称一致。
-
释放资源 :
在读取完成后,同样需要释放资源。
csharp复制代码
|---|-----------------|
| |fs.Release();
|
注意事项
- 在使用
FileStorage
时,需要确保在读取或写入过程中不要出现错误,否则可能会导致数据丢失或文件损坏。 - 对于复杂的数据结构(如包含多个
Mat
对象的列表或字典),可能需要使用更复杂的序列化策略。 - 在处理大量数据时,需要注意内存管理和性能问题,确保应用程序的稳定性和响应性。
序列化和反序列化Mat
对象
对于Mat
对象,OpenCVSharp提供了直接的序列化和反序列化方法。这意味着你可以直接将Mat
对象写入文件,然后再从文件中读取回来,而无需手动处理每个像素值。这在处理图像数据时非常有用,因为它可以大大简化数据的存储和传输过程。
【界面展示】
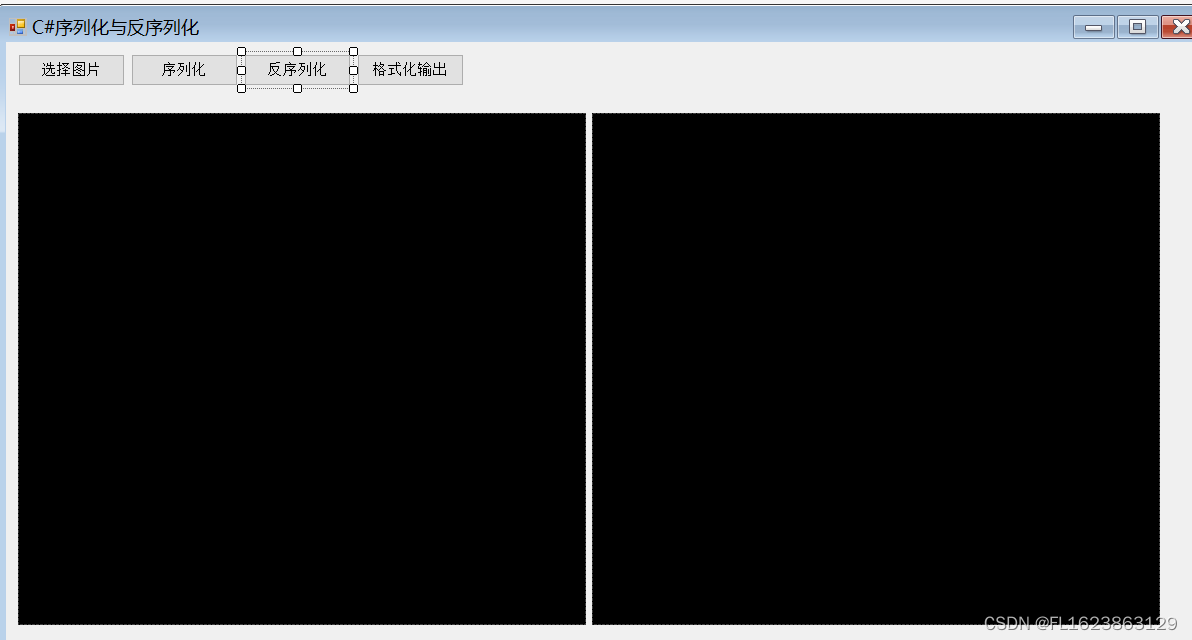
【实现意义】
序列化和反序列化可以将图像数据存储为特定格式作为分析使用,格式化输出则有利于对图像数据进行肉眼分析。
【实现代码】
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Diagnostics;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using OpenCvSharp;
namespace FIRC
{
public partial class Form1 : Form
{
Mat src = new Mat();
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog openFileDialog = new OpenFileDialog();
openFileDialog.Filter = "图文件(*.*)|*.jpg;*.png;*.jpeg;*.bmp";
openFileDialog.RestoreDirectory = true;
openFileDialog.Multiselect = false;
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
src = Cv2.ImRead(openFileDialog.FileName);
pictureBox1.Image = OpenCvSharp.Extensions.BitmapConverter.ToBitmap(src);
}
}
private void button2_Click(object sender, EventArgs e)
{
if(pictureBox1.Image==null)
{
return;
}
FileStorage fileStorage = new FileStorage("image.data", FileStorage.Modes.Write);
fileStorage.Write("image", src);
fileStorage.Release();
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void button3_Click(object sender, EventArgs e)
{
if(File.Exists("image.data"))
{
FileStorage fileStorage = new FileStorage("image.data", FileStorage.Modes.Read);
Mat resultMat = fileStorage["image"].ToMat();
pictureBox2.Image = OpenCvSharp.Extensions.BitmapConverter.ToBitmap(resultMat); //Mat转Bitmap
}
}
private void button4_Click(object sender, EventArgs e)
{
if (pictureBox1.Image == null)
{
return;
}
//Console.WriteLine(src.ToString());
Console.WriteLine(Cv2.Format(src,FormatType.NumPy));
//Console.WriteLine(Cv2.Format(src, FormatType.CSV));
//Console.WriteLine(Cv2.Format(src, FormatType.MATLAB));
//Console.WriteLine(Cv2.Format(src, FormatType.Python));
}
}
}
【测试环境】
vs2019
netframework4.7.2
opencvsharp4.8.0
【源码下载】