本想送你一本沉思录,可该迷途知返的人是我
------ 24.6.18
设计模式
设计模式(Design pattern) ,是一套被反复使用、经过分类编目的、代码设计经验的总结,使用设计模式是为了可重用代码、保证代码可靠性、程序的重用性,稳定性 。
1995 年,GoF(Gang of Four,四人组)合作出版了《设计模式:可复用面向对象软件的基础》一书,某收录了 23种设计模式。<大话设计模式>
总体来说设计模式分为三大类:
创建型模式,共五种:工厂方法模式、抽象工厂模式、单例模式、建造者模式、原型模式。-->创建对象
结构型模式,共七种:适配器模式、装饰器模式、代理模式、外观模式、桥接模式、组合模式、享元模式。---> 对功能进行增强
行为型模式,共十一种:策略模式、模板方法模式、观察者模式、迭代子模式、责任链模式、命令模式、备忘录模式、状态模式、访问者模式、中介者模式、解释器模式。
一、模板方法设计模式
模板方法(Template Method)模式: 定义一个操作中的算法的骨架,而将一些步骤延迟到子类中。明确了一部分功能,而另一部分功能不明确。需要延伸到子类中实现
示例:
java
public class QuanJuDe extends Hotel{
@Override
public void eatCai(){
System.out.println("薄饼");
System.out.println("卷烤鸭");
System.out.println("蘸酱");
System.out.println("葱丝");
System.out.println("吃烤鸭");
}
}
java
public class ZhangLiang extends Hotel{
@Override
public void eatCai(){
System.out.println("选菜");
System.out.println("选味道");
System.out.println("吃菜");
}
}
java
public class Demo300ModelPattern {
public static void main(String[] args) {
QuanJuDe quanJuDe = new QuanJuDe();
quanJuDe.eat();
System.out.println("__________________");
ZhangLiang zhangLiang = new ZhangLiang();
zhangLiang.eat();
}
}
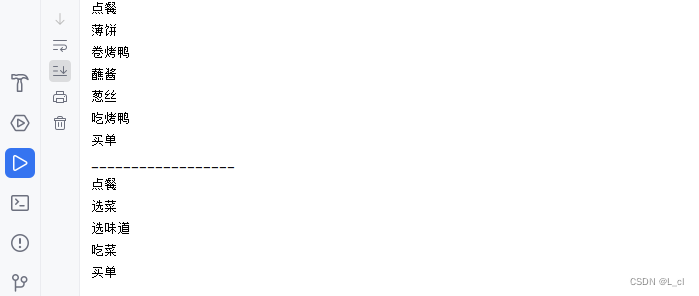
二、单例模式
1.目的
让一个类只产生一个对象,供外界使用
2.分类:
a.饿汉式
b.懒汉式
a、饿汉式
java
public class SingletonHun {
/*
防止外界随意使用构造方法new对象,我们需要将构造私有化
*/
private SingletonHun(){
}
/*
为了赶紧new对象,我们new对象的时候变成静态的,让其随着类的加载而加载
为了不让外界随便使用类名调用此静态对象,我们将其变成private
*/
private static SingletonHun singleton = new SingletonHun();
/*
为了将内部new出来的对象给外界我们可以定义 一个方法,将内部的对象返回给外界
*/
public static SingletonHun getSingleton(){
return singleton;
}
}
java
public class Demo301Test {
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
SingletonHun singleton = SingletonHun.getSingleton();
System.out.println(singleton);
}
}
}
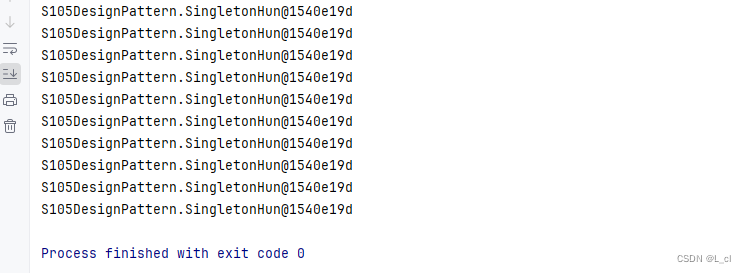
b、懒汉式
java
public class SingletonLazy {
/*
防止外界随意使用构造方法new对象,我们需要将构造私有化
*/
private SingletonLazy() {
}
/*
懒汉式,不着急new对象
*/
private static SingletonLazy singletonLazy = null;
/*
为了将内部new出来的对象给外界
定义一个方法,将内部new出来的对象返回
*/
public static SingletonLazy getSingletonLazy() {
// 加锁 如果singletonLazy不是null,就没必要抢锁了,直接返回,是null在抢锁
if (singletonLazy == null) {
synchronized (SingletonLazy.class) {
if (singletonLazy == null) {
singletonLazy = new SingletonLazy();
}
}
}
return singletonLazy;
}
}
java
public class Demo302Test02 {
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
SingletonLazy singleton = SingletonLazy.getSingletonLazy();
System.out.println(singleton);
}
}
}
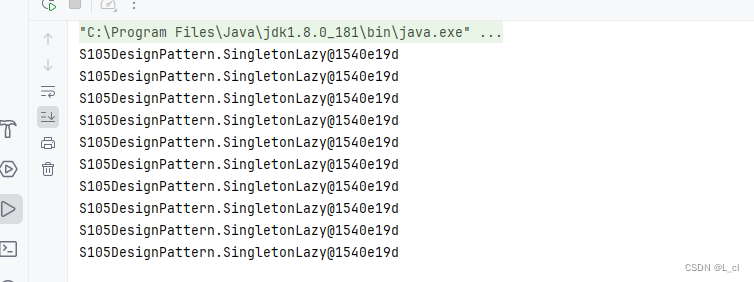
小结:构造私有
对象私有 静态的