一、226.翻转二叉树
题目链接:https://leetcode.cn/problems/invert-binary-tree/
文章讲解:https://programmercarl.com/0226.翻转二叉树.html#算法公开课
视频讲解:https://www.bilibili.com/video/BV1fA4y1o715
1.1 初见思路
- 看到树的题目,就先考虑使用递归,递归写起来方便
- 递归的三要素
1.2 具体实现
java
class Solution {
public TreeNode invertTree(TreeNode root) {
if(root==null){
return root;
}
//前序,中左右,中的操作是把中的左右节点互换
reverse(root);
invertTree(root.left);
invertTree(root.right);
return root;
}
void reverse(TreeNode node){
TreeNode temp = new TreeNode();
temp = node.left;
node.left=node.right;
node.right=temp;
}
}
1.3 重难点
二、 101. 对称二叉树
题目链接:https://leetcode.cn/problems/symmetric-tree/submissions/540690035/
文章讲解:https://programmercarl.com/0101.对称二叉树.html#算法公开课
视频讲解:https://www.bilibili.com/video/BV1ue4y1Y7Mf
2.1 初见思路
- 判断节点的左子树跟右子树是否相同,
2.2 具体实现
java
class Solution {
public boolean isSymmetric(TreeNode root) {
//使用后序遍历,判断左节点和右节点是否相同,将结果返回给中节点
return compare(root.left, root.right);
}
private boolean compare(TreeNode left, TreeNode right) {
if (left == null && right != null) {
return false;
}
if (left != null && right == null) {
return false;
}
if (left == null && right == null) {
return true;
}
if (left.val != right.val) {
return false;
}
// 比较外侧
boolean compareOutside = compare(left.left, right.right);
// 比较内侧
boolean compareInside = compare(left.right, right.left);
return compareOutside && compareInside;
}
}
2.3 重难点
- 思路转换成代码实现有难度,要考虑到左节点和右节点是否相等的各种场景
三、 104.二叉树的最大深度
题目链接:https://leetcode.cn/problems/maximum-depth-of-binary-tree/description/
文章讲解:https://programmercarl.com/0104.二叉树的最大深度.html
视频讲解:https://www.bilibili.com/video/BV1Gd4y1V75u
3.1 初见思路
- 获取左子树的深度和右子树的深度,取较大者+1,为中节点的深度
3.2 具体实现
java
class Solution {
public int maxDepth = 0;
public int maxDepth(TreeNode root) {
if(root==null){
return 0;
}
//采用后序遍历,左右中
int leftD = maxDepth(root.left);
int rightD = maxDepth(root.right);
return Math.max(leftD,rightD)+1;
}
}
3.3 重难点
四、 111.二叉树的最小深度
题目链接:https://leetcode.cn/problems/remove-element/
文章讲解:https://programmercarl.com/0111.二叉树的最小深度.html
视频讲解:https://www.bilibili.com/video/BV1QD4y1B7e2
4.1 初见思路
- 使用前序遍历:左右中
4.2 具体实现
java
class Solution {
public int minDepth(TreeNode root) {
if (root == null) {
return 0;
}
int leftDepth = minDepth(root.left);
int rightDepth = minDepth(root.right);
if (root.left == null) {
return rightDepth + 1;
}
if (root.right == null) {
return leftDepth + 1;
}
// 左右结点都不为null
return Math.min(leftDepth, rightDepth) + 1;
}
}
4.3 重难点
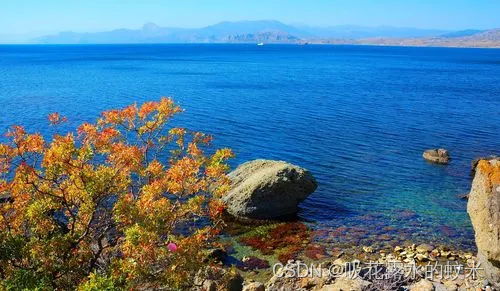