标头:#include <regex>
相关函数:
regex_match
regex_replace
regex_search
名称 | 描述 |
---|---|
regex_match | 测试正则表达式是否与整个目标字符串相完全匹配。 |
regex_replace | 替换匹配正则表达式。 |
regex_search | 搜索正则表达式匹配项。 |
1. regex_search
成功搜索到其正则表达式 re 时,函数才返回 true
cpp
//形如"2024-01-01 01-01-01" 时间匹配正则
wregex rx(L"\\d{4}(\\-|\\/|.)\\d{1,2}(\\-|\\/|.)\\d{1,2}\\s\\d{1,2}(\\-|\\/|.)\\d{1,2}(\\-|\\/|.)\\d{1,2}(\\-|\\/|.)");
wsmatch mr;
if (regex_search(recordedInfo, mr, rx))
{
time = mr[0].str();
std::wstring iStart(time);
std::wsmatch result;
std::vector<std::wstring> vTime;
vTime.clear();
while (regex_search(iStart, result, std::wregex(L"\\d{1,}")))
{
vTime.emplace_back(result[0].str());
iStart = result.suffix();
}
//concatenate string
if (vTime.size() >= 5)
{
std::wstring temp;
temp.append(vTime[0]);
temp.append(L"-");
temp.append(vTime[1]);
temp.append(L"-");
temp.append(vTime[2]);
temp.append(L" ");
temp.append(vTime[3]);
temp.append(L":");
temp.append(vTime[4]);
if (vTime.size() >= 6)
{
temp.append(L":");
temp.append(vTime[5]);
}
time = temp;
}
}
2. regex_match
测试正则表达式是否与整个目标字符串相完全匹配。字符串与正则表达式参数 完全匹配时才返回 true。因此,如果我们得需求是:当某字符串与指定正则完全匹配时,按照指定规则抽取数据,然后具体得业务操作。那么第一步可以使用该函数。
第二步,使用 regex_search 匹配目标序列中的子字符串,并使用 regex_iterator 查找多个匹配(注意:能否匹配出多个子串,与正则有关)。
cpp
std::regex rx("c(a*)|(b)");
std::cmatch mr;
std::regex_search("xcaaay", mr, rx);
// index through submatches
for (size_t n = 0; n < mr.size(); ++n)
{
std::cout << "submatch[" << n << "]: matched == " << mr[n].matched << ",at position " << mr.position(n) << ", " << mr.length(n) << " chars, value == " << mr[n] << std::endl;
}
std::cout << std::endl;
// iterate through submatches
for (std::cmatch::iterator it = mr.begin(); it != mr.end(); ++it)
{
std::cout << "next submatch: matched == " << it->matched <<", " << it->length() << " chars, value == " << *it << std::endl;
}
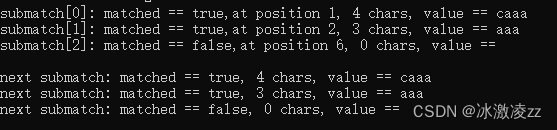