本篇介绍一下使用vue3-openlayers加载天地图切片,三种方法:
- 使用tianditu(ol-source-tianditu内部实现其实用的wmts)
- 使用wmts(ol-source-wmts)
- 使用xyz(ol-source-xyz)
1 需求
- vue3-openlayers加载天地图
2 分析
主要是不同类型source的使用
3 实现
3.1 ol-source-tianditu
javascript
<template>
<ol-map
:loadTilesWhileAnimating="true"
:loadTilesWhileInteracting="true"
style="width: 100%; height: 100%"
ref="mapRef"
>
<ol-view
ref="view"
:center="center"
:rotation="rotation"
:zoom="zoom"
:projection="projection"
/>
<ol-tile-layer>
<ol-source-tianditu
layerType="img"
:projection="projection"
:tk="key"
:hidpi="true"
ref="sourceRef"
></ol-source-tianditu>
</ol-tile-layer>
<ol-tile-layer>
<ol-source-tianditu
:isLabel="true"
layerType="img"
:projection="projection"
:tk="key"
:hidpi="true"
></ol-source-tianditu>
</ol-tile-layer>
<!-- 下面的注记加载等价于上面的 -->
<!-- <ol-tile-layer>
<ol-source-tianditu
layerType="cia"
:projection="projection"
:tk="key"
:hidpi="true"
></ol-source-tianditu>
</ol-tile-layer> -->
</ol-map>
</template>
<script setup lang="ts">
const center = ref([121, 31]);
const projection = ref('EPSG:4326');
const zoom = ref(5);
const rotation = ref(0);
const mapRef=ref();
const key = '换成申请的天地图key';
const sourceRef = ref(null);
// layerType img, vec, ter, cia, cta
// 'vec', 'cva' 矢量底图, 矢量注记
// 'img', 'cia' 影像底图, 影像注记
// 'ter', 'cta' 地形晕渲, 地形注记
onMounted(() => {
const source = sourceRef.value?.source;
// 调用source上的方法
});
</script>
<style scoped lang="scss">
</style>
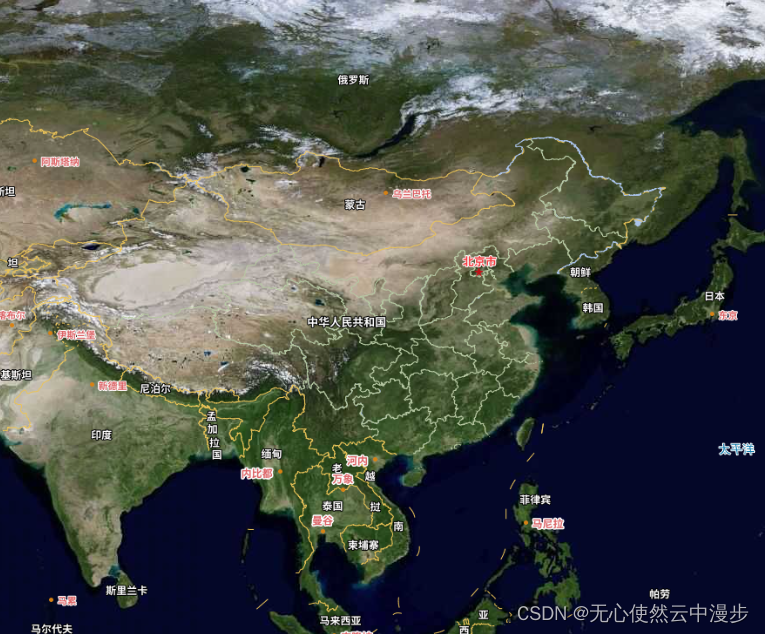
3.2 wmts
javascript
<template>
<ol-map
:loadTilesWhileAnimating="true"
:loadTilesWhileInteracting="true"
style="width: 100%; height: 100%"
ref="mapRef"
>
<ol-view
ref="view"
:center="center"
:rotation="rotation"
:zoom="zoom"
:projection="projection"
/>
<ol-tile-layer>
<ol-source-wmts
ref="sourceRef"
:attributions="attribution"
:url="url"
:projection="projection"
:matrixSet="matrixSet"
:format="format"
:layer="layerImage"
:styles="styleName"
></ol-source-wmts>
</ol-tile-layer>
<ol-tile-layer>
<ol-source-wmts
:attributions="attribution"
:url="url_address"
:projection="projection"
:matrixSet="matrixSet"
:format="format"
:layer="layerAddress"
:styles="styleName"
></ol-source-wmts>
</ol-tile-layer>
</ol-map>
</template>
<script setup lang="ts">
const center = ref([121, 31]);
const projection = ref('EPSG:4326');
const zoom = ref(5);
const rotation = ref(0);
const mapRef = ref();
const format = ref('image/png');
const styleName = ref('default');
const attribution = ref('Tiles © <a href="https://www.tianditu.gov.cn/">天地图</a>');
const key = '换成申请的天地图key';
const sourceRef = ref(null);
const layerImage = ref('img');
const layerAddress = ref('cia');
const matrixSet = ref('c');//c: 经纬度投影 w: 墨卡托投影
// 'vec', 'cva' 矢量底图, 矢量注记
// 'img', 'cia' 影像底图, 影像注记
// 'ter', 'cta' 地形晕渲, 地形注记
// 影像底图
const url = ref(`http://t{0-6}.tianditu.com/${layerImage.value}_${matrixSet.value}/wmts?tk=${key}`);
// 影像注记
const url_address = ref(
`http://t{0-6}.tianditu.com/${layerAddress.value}_${matrixSet.value}/wmts?tk=${key}`
);
onMounted(() => {
const map=mapRef.value?.map
const source = sourceRef.value?.source;
});
</script>
<style scoped lang="scss"></style>
</script>
<style scoped lang="scss">
</style>
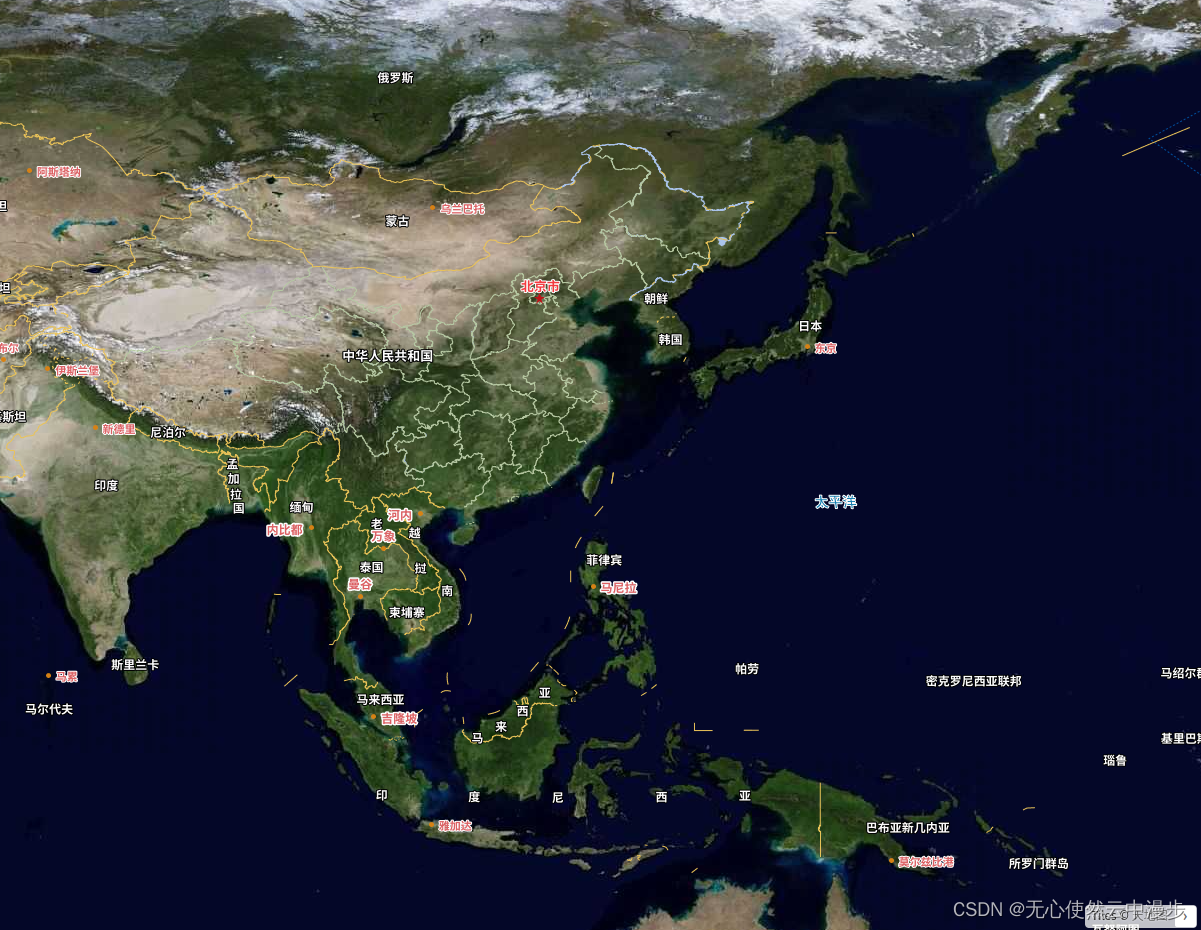
3.3 xyz
javascript
<template>
<ol-map
:loadTilesWhileAnimating="true"
:loadTilesWhileInteracting="true"
style="width: 100%; height: 100%"
ref="mapRef"
>
<ol-view
ref="view"
:center="center"
:rotation="rotation"
:zoom="zoom"
:projection="projection"
/>
<ol-tile-layer>
<ol-source-xyz :projection="projection" :url="imageUrl" />
</ol-tile-layer>
<ol-tile-layer :opacity="0.9">
<ol-source-xyz :projection="projection" :url="addressUrl" />
</ol-tile-layer>
</ol-map>
</template>
<script setup lang="ts">
const center = ref([121, 31]);
const projection = ref('EPSG:4326');
const zoom = ref(5);
const rotation = ref(0);
const mapRef = ref();
const key = '换成申请的天地图key';
const sourceRef = ref(null);
const layerImage = ref('img');
const layerAddress = ref('cia');
const matrixSet = ref('c');//c: 经纬度投影 w: 墨卡托投影
// 'vec', 'cva' 矢量底图, 矢量注记
// 'img', 'cia' 影像底图, 影像注记
// 'ter', 'cta' 地形晕渲, 地形注记
// 影像底图
const imageUrl = ref(
`https://t{0-7}.tianditu.gov.cn/DataServer?T=${layerImage.value}_${matrixSet.value}&tk=${key}&x={x}&y={y}&l={z}`
);
// 影像注记
const addressUrl = ref(
`https://t{0-7}.tianditu.gov.cn/DataServer?T=${layerAddress.value}_${matrixSet.value}&tk=${key}&x={x}&y={y}&l={z}`
);
onMounted(() => {
const map = mapRef.value?.map;
const source = sourceRef.value?.source;
});
</script>
<style scoped lang="scss"></style>
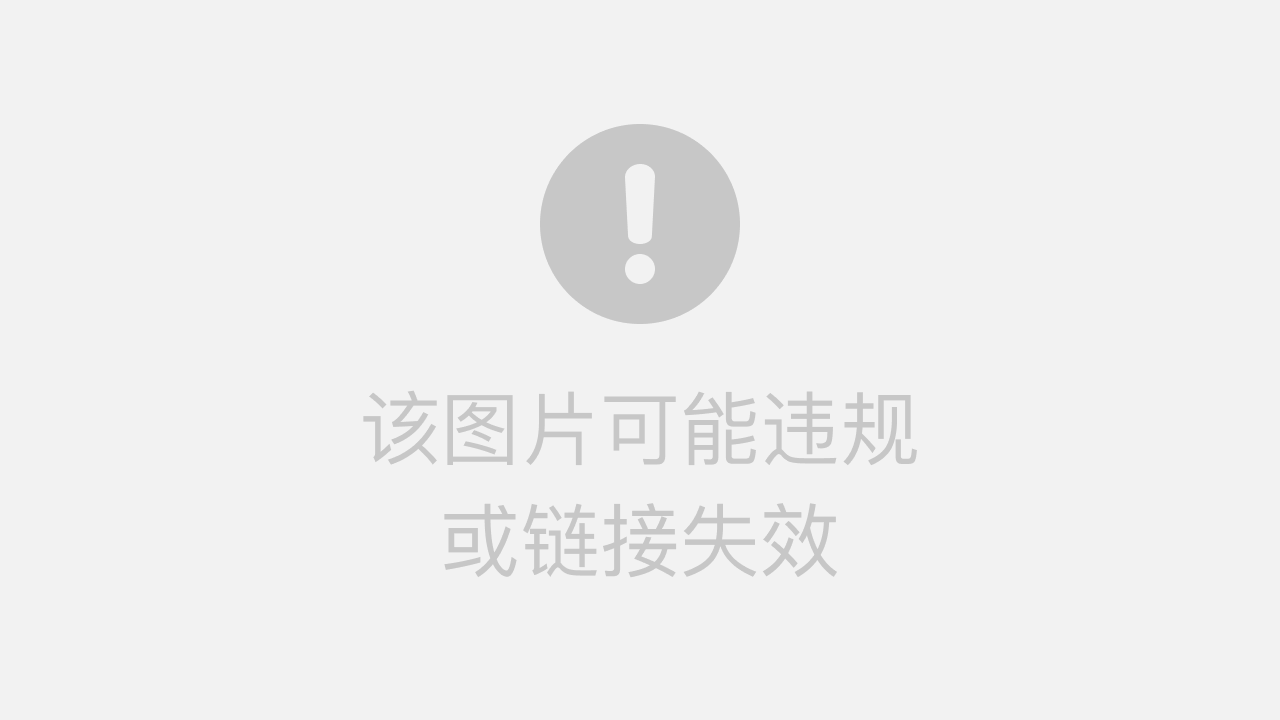
加载注记图层后,切片虽然显示png,但是好像不是透明的,只能在注记图层增加透明度控制
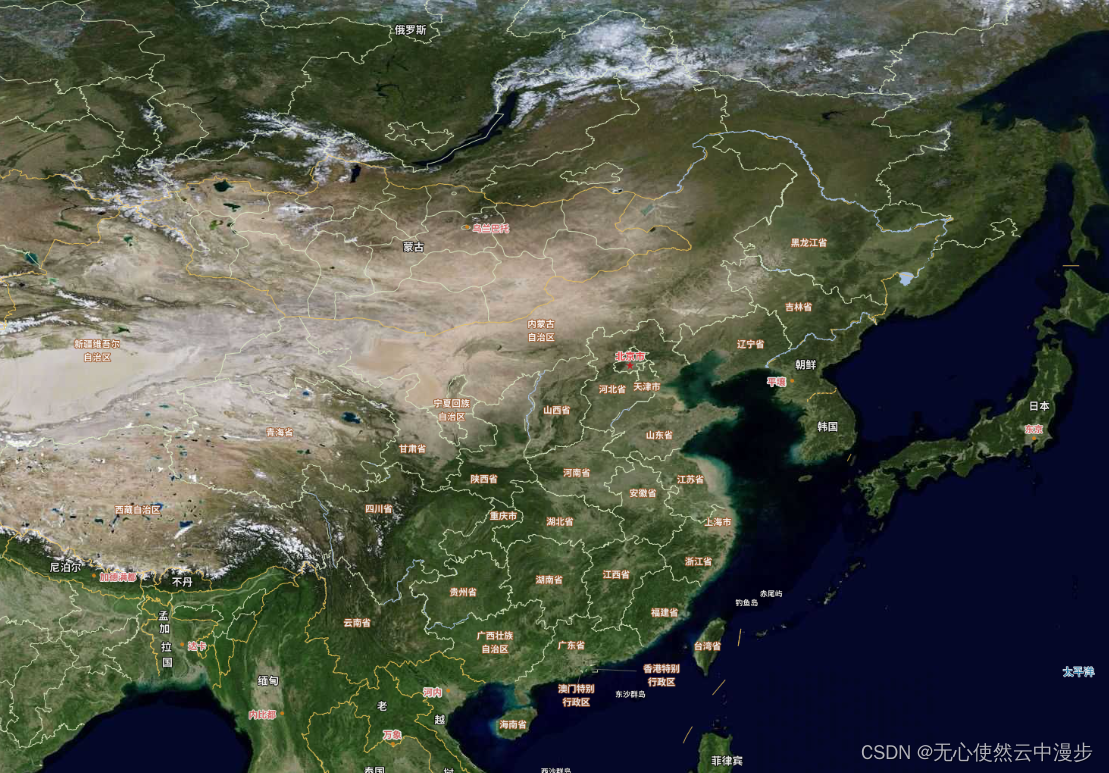