师从黑马程序员
数据库介绍
数据库就是存储数据的库
数据组织:库->表->数据
数据库和SQL的关系
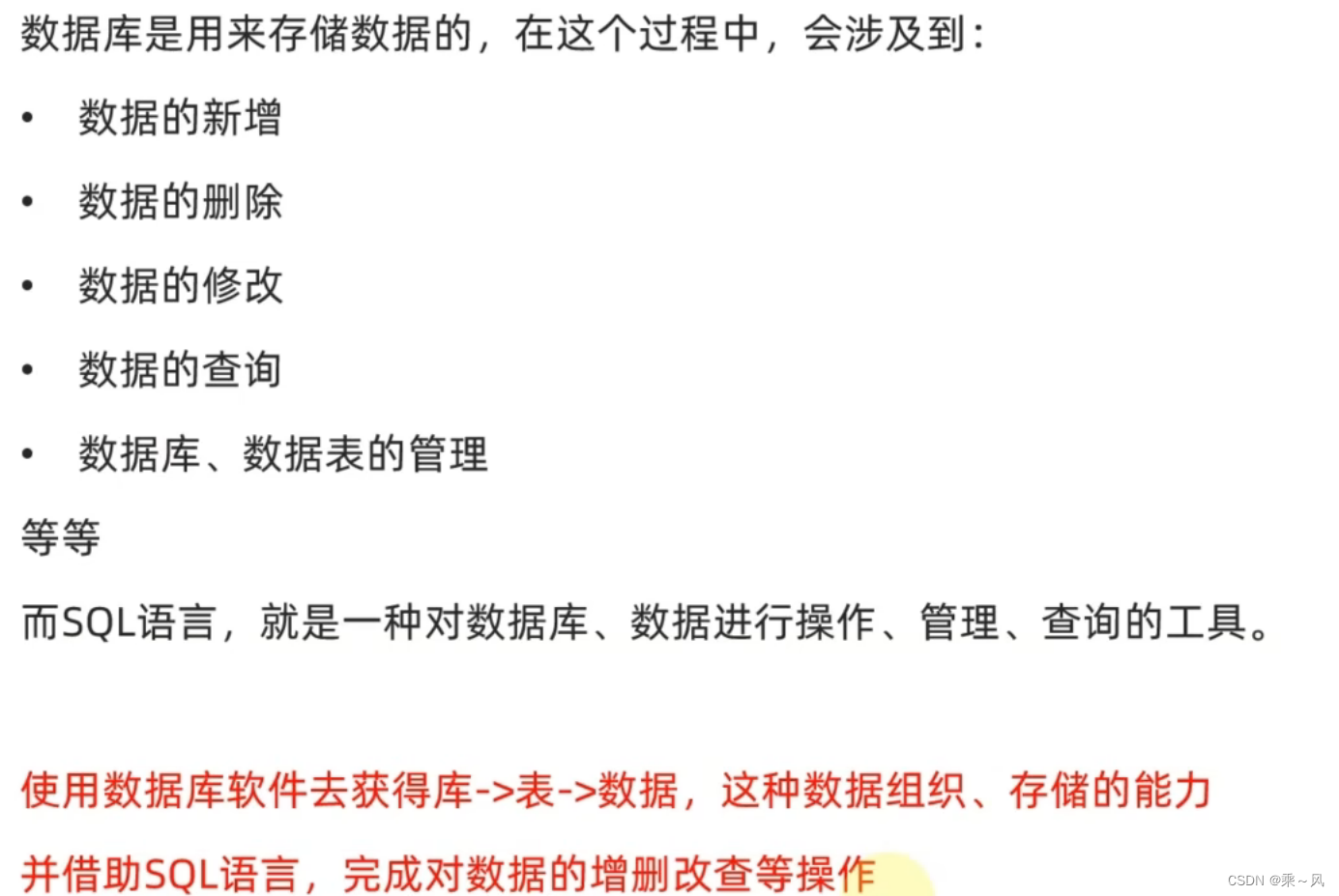
MySQL的基础命令
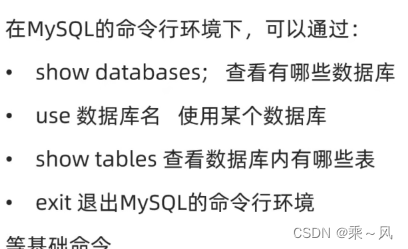
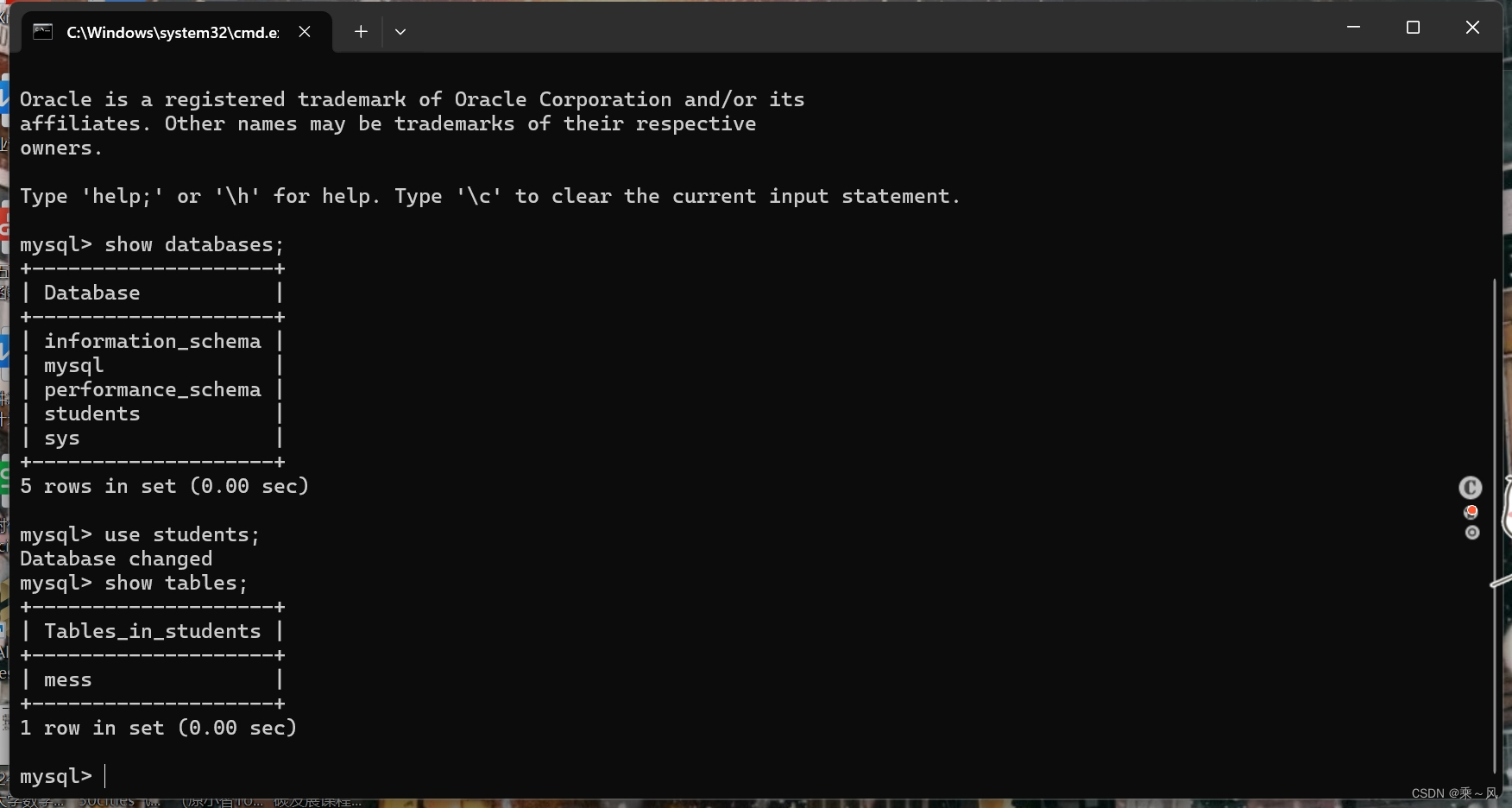
SQL基础
SQL语言的分类
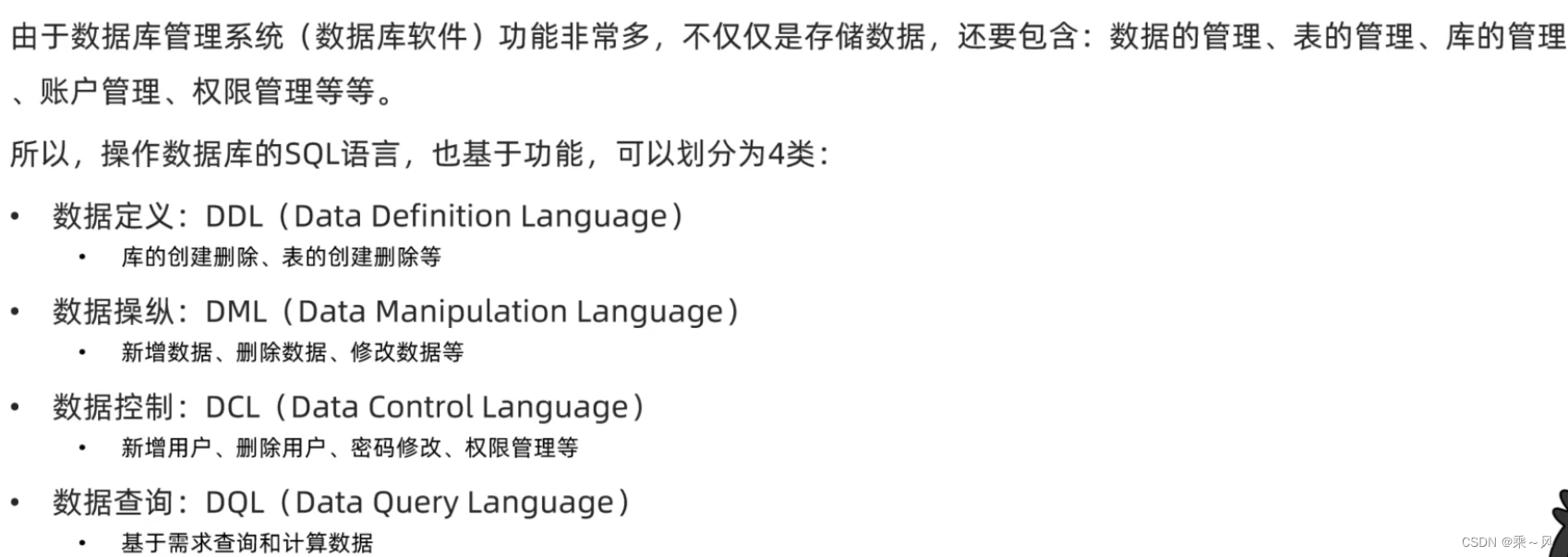
SQL的语法特征
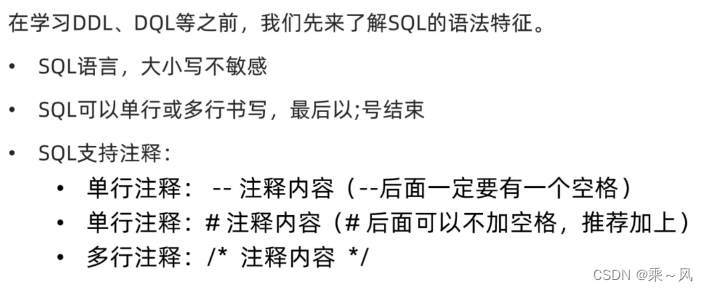
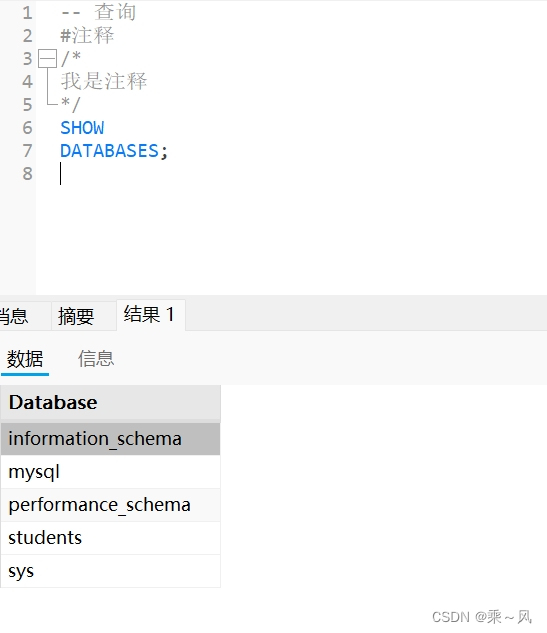
DDL-库管理
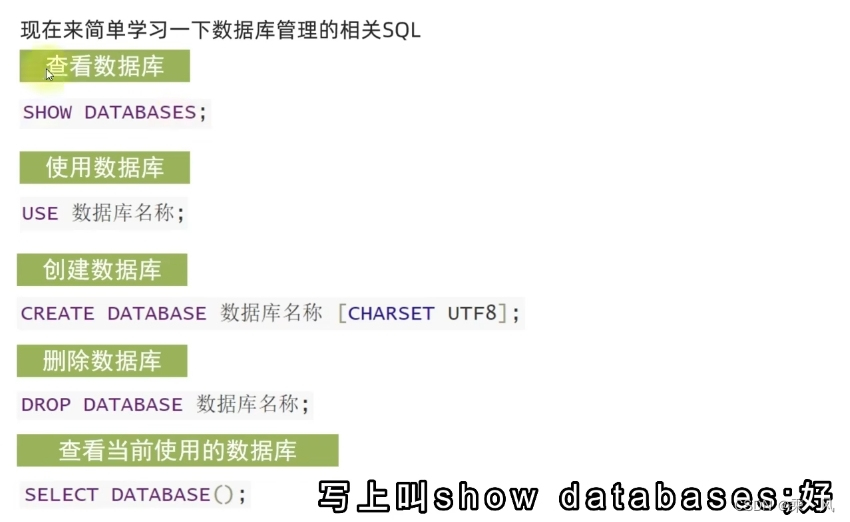
sql
show DATABASES;
use sys;
SELECT database();
CREATE DATABASE test CHARSET utf-8;
SHOW DATABASES;
-- drop DATABASE test01;
DDL-表管理
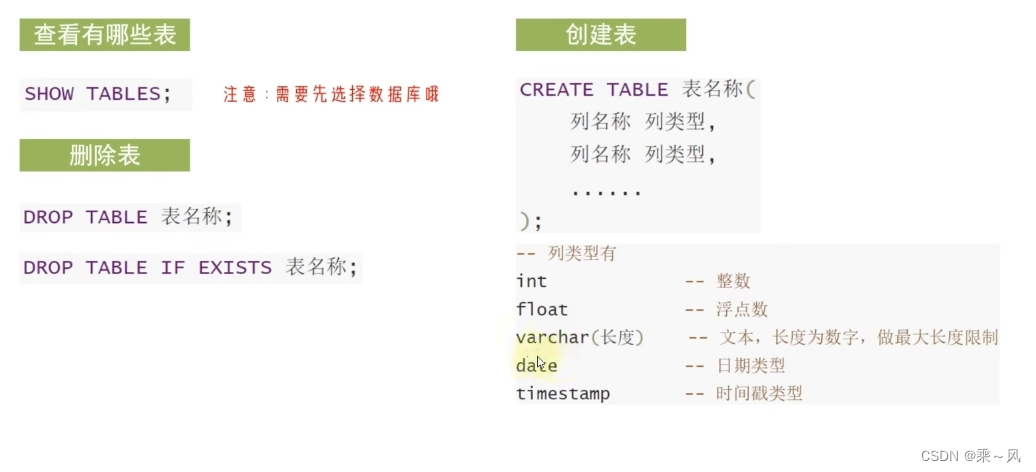
sql
use students;
show TABLES;
CREATE TABLE student(
id int,
name VARCHAR(10),
age int
);
drop table student;
DML-数据操作
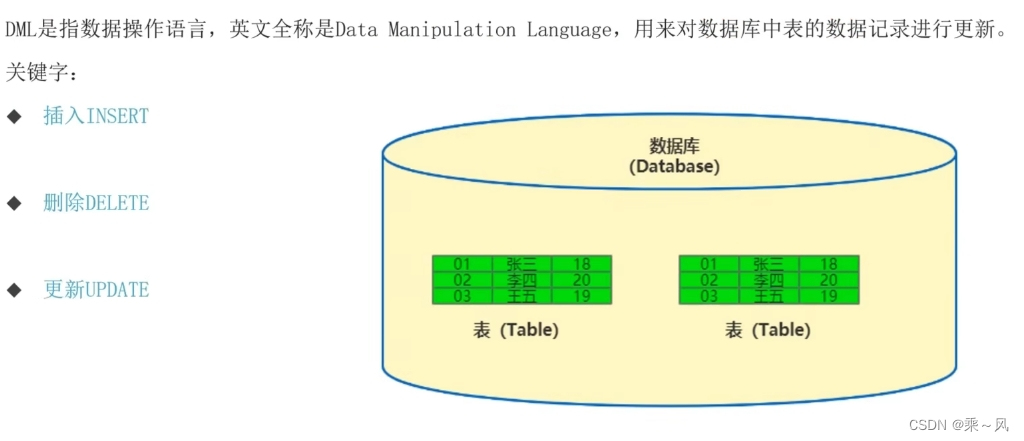
数据插入-INSERT
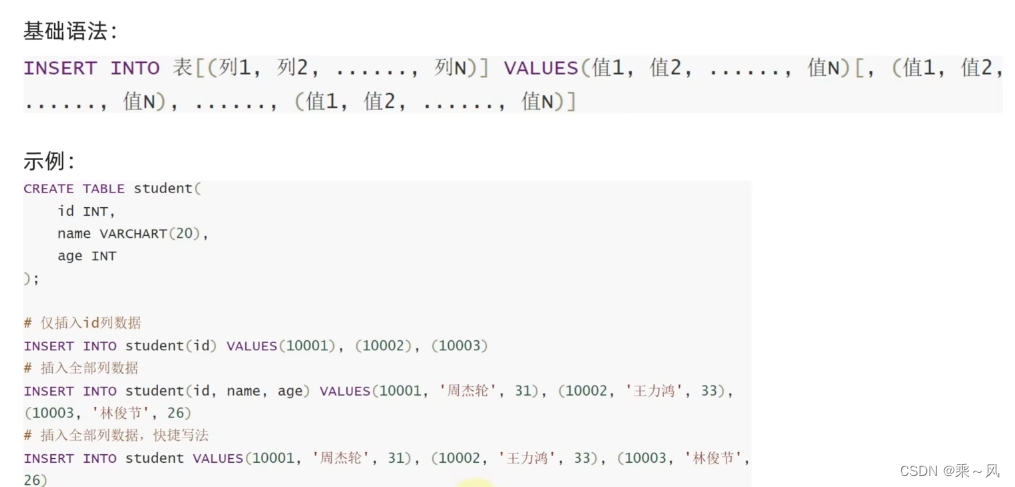
sql
create table student(
id int,
name VARCHAR(10),
age int
);
insert into student(id) VALUES(1),(2),(3);
# 等价于
-- insert into student(id) VALUES(1);
-- insert into student(id) VALUES(1);
-- insert into student(id) VALUES(1);
insert into student(id,name,age) VALUES(4,'周杰伦',31),(5,'领军解',33);
数据删除-DELETE
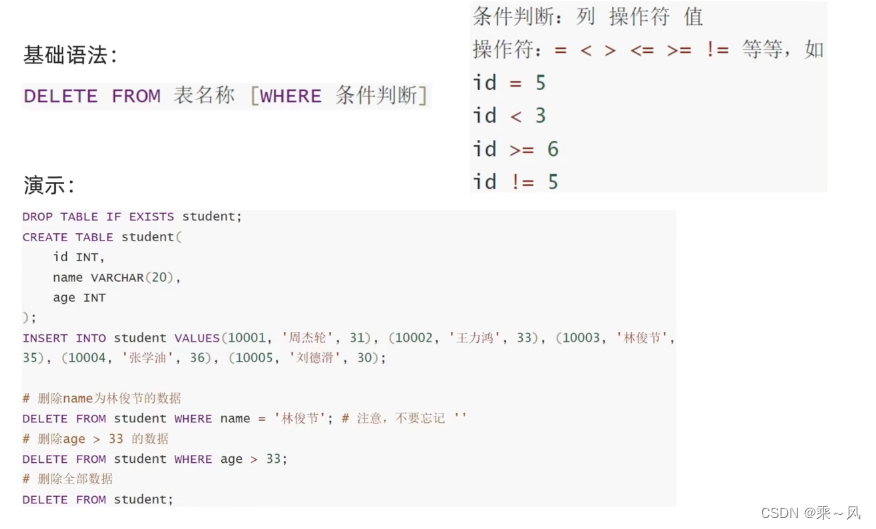
sql
DELETE from student WHERE id =4;
数据更新-Update
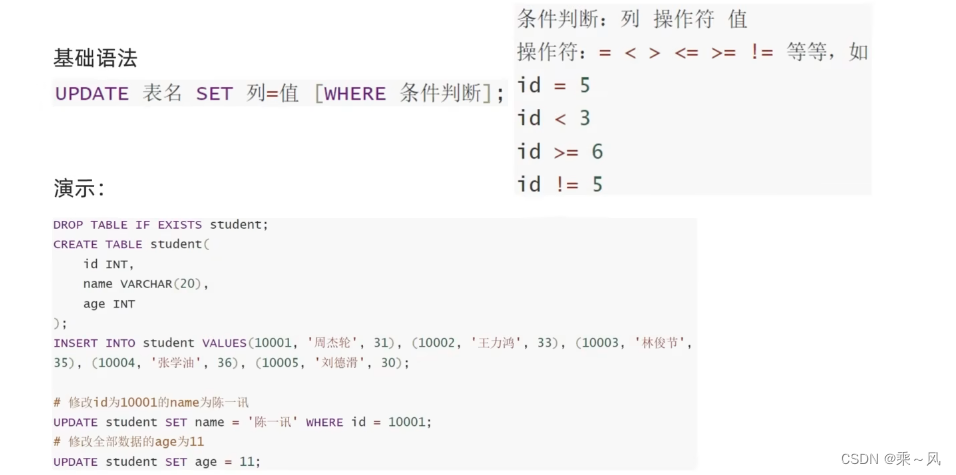
sql
UPDATE student set name ='张学友' where id ='5';
注:字符串的值,出现在SQL语句中,必须要用单引号
DQL-数据查询
基础数据查询

sql
select id,name,age from student;
select * from student;
SELECT * from student where age>20;
select * from student where name ='张学友';
分组聚合
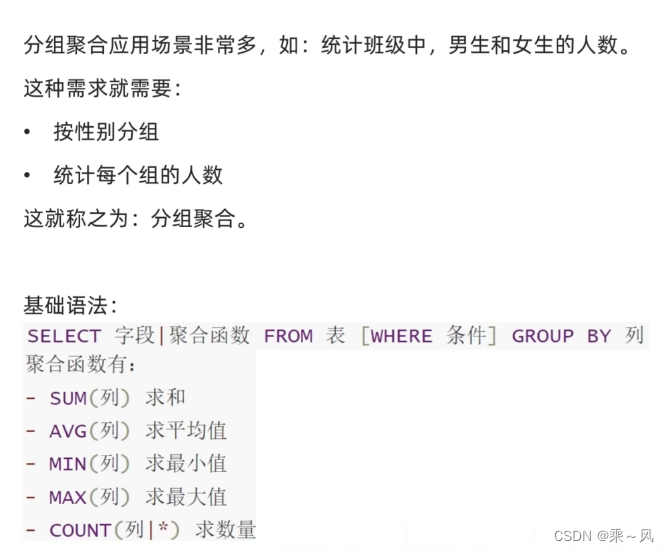
sql
select id,avg(age) from student group by id;
-- 在非聚合函数中,GROUP BY 后出现什么,select 才能出现什么,但对像聚合函数avg()不适用
sql
select id,avg(age),sum(age),min(age),max(age),count(*) from student group by id;
排序分页
结果排序
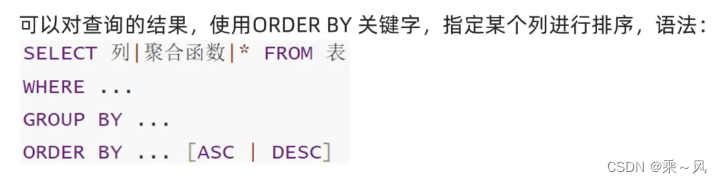
结果分页限制
sql
select * from student where age >20 order by age ASC;
-- asc升序 de 降序
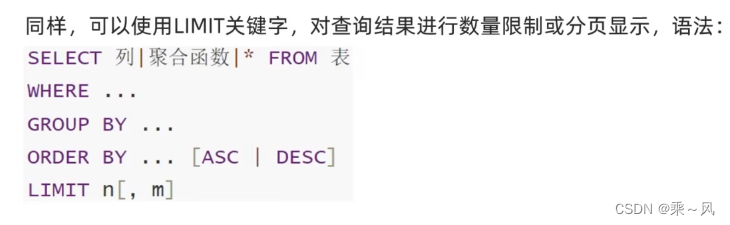
sql
select * from student limit 5;
-- 只查询5条数据
SELECT * from student LIMIT 10,5;
-- 6跳过前10条数据,然后查询5条数据
-- 可以和其他语句结合
select age,count(*) from student where age>20 group by age ORDER BY age LIMIT 3;
Python操作MySQL语句
创建到MySQL的数据库链接
sql
from pymysql import Connection
#构建到MySQL数据库的链接
conn=Connection(
host="localhost", #主机名
port=3306, #端口
user="root", #账户
password="5863AXzy@" #密码
)
# print(conn.get_server_info())#打印出数据库的基本信息
#执行非查询性质SQL
# cursor=conn.cursor() #获取游标对象
# #选择数据库
# conn.select_db("students")
# #执行sql #传入sql语句
# cursor.execute("create table students_pymysql(id int);")#创建一个在students库下的students_pymysql表 #分号可不写
#执行查询性质的SQL
cursor=conn.cursor() #获取游标对象
#选择数据库
conn.select_db("students")
#执行sql #传入sql语句
cursor.execute("select * from student")
#拿到上述语句的结果
results=cursor.fetchall() #生成的是元组
for r in results:
print(r)
#关闭链接
conn.close()
commit-提交
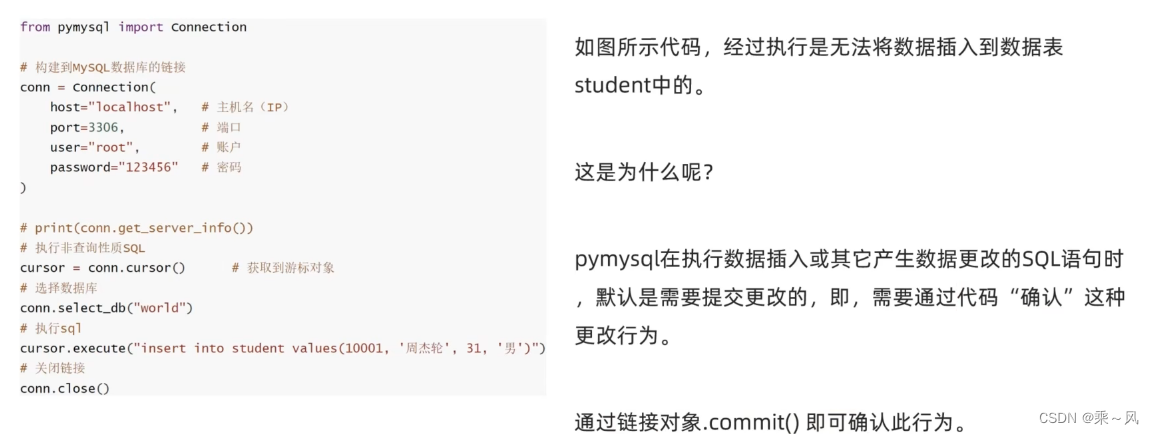
sql
from pymysql import Connection
#构建到MySQL数据库的链接
conn=Connection(
host="localhost", #主机名
port=3306, #端口
user="root", #账户
password="5863AXzy@" #密码
)
#执行查询性质的SQL
cursor=conn.cursor() #获取游标对象
#选择数据库
conn.select_db("students")
#执行sql #传入sql语句
cursor.execute("insert into student values(1,'张学友',23)")
#通过commit确认
conn.commit()
#关闭链接
conn.close()
自动commit
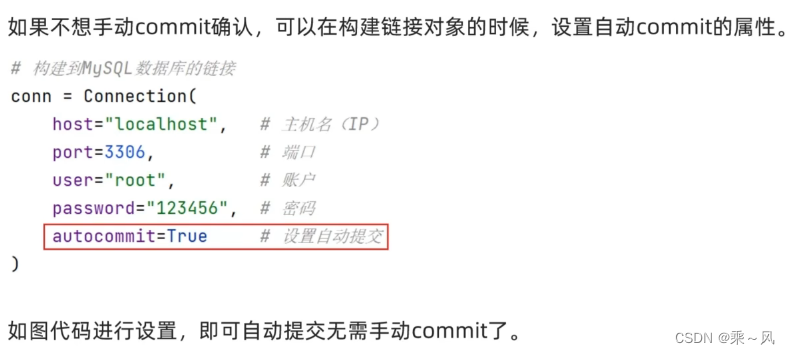
sql
from pymysql import Connection
#构建到MySQL数据库的链接
conn=Connection(
host="localhost", #主机名
port=3306, #端口
user="root", #账户
password="5863AXzy@", #密码
autocommit=True #自动提交
)
#执行查询性质的SQL
cursor=conn.cursor() #获取游标对象
#选择数据库
conn.select_db("students")
#执行sql #传入sql语句
cursor.execute("insert into student values(1,'林俊杰',23)")
#关闭链接
conn.close()
综合案例
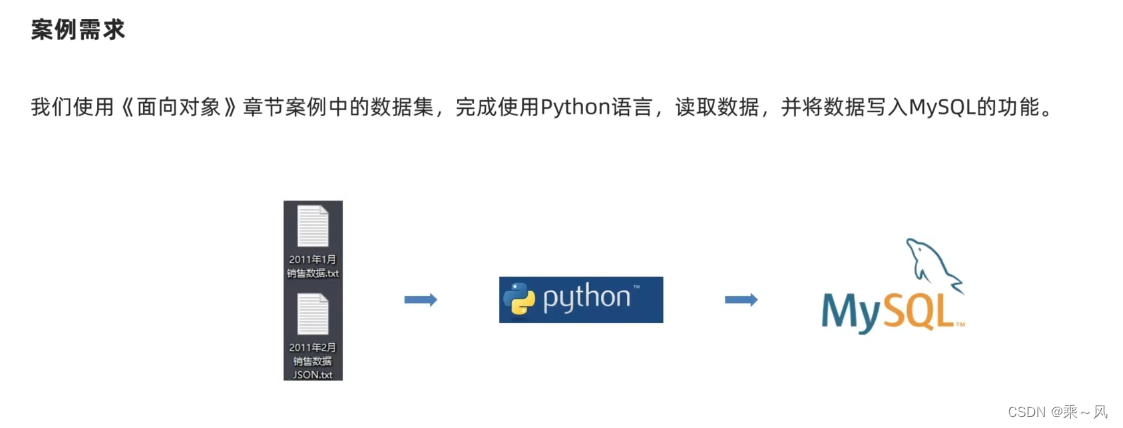
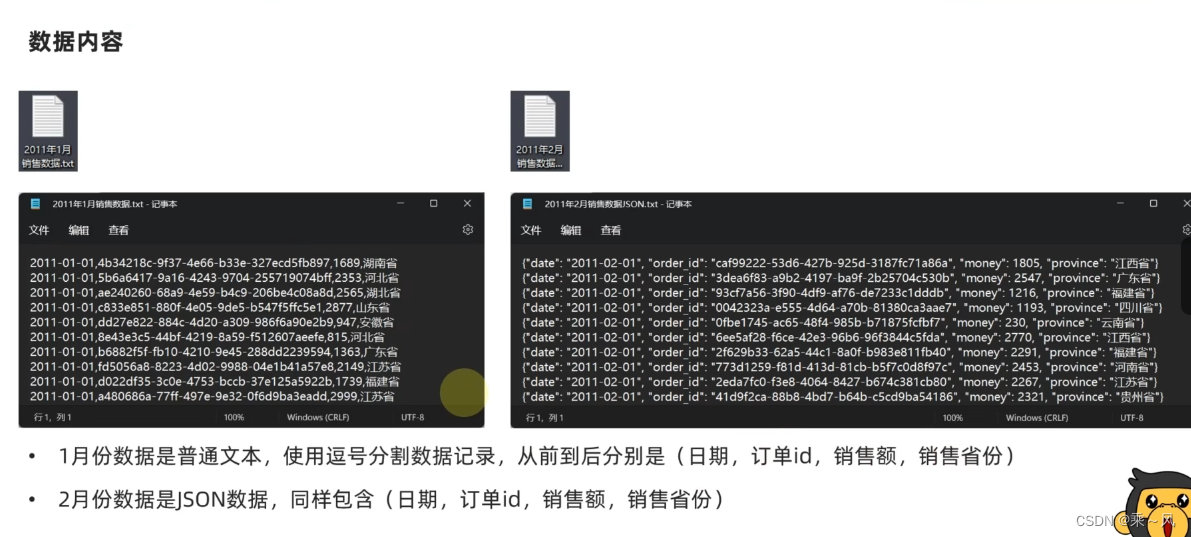
DDL定义
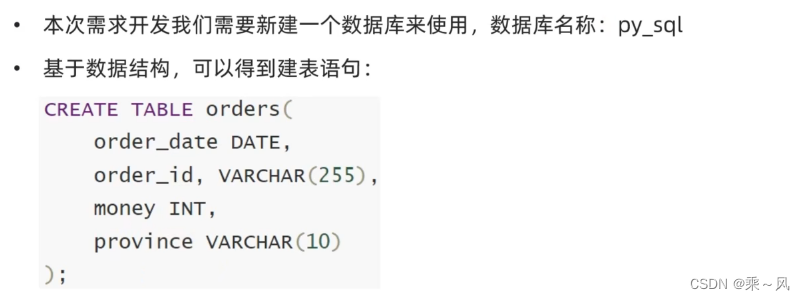
sql
"""
SQL 综合案例,读取文件,写入MySQL
"""
from file_define import TextFileReader, JsonFileReader
from data_define import Record
from pymysql import Connection
text_file_reader = TextFileReader("D:/2011年1月销售数据.txt")
json_file_reader = JsonFileReader("D:/2011年2月销售数据JSON.txt")
jan_data: list[Record] = text_file_reader.read_data()
feb_data: list[Record] = json_file_reader.read_data()
# 将2个月份的数据合并为1个list来存储
all_data: list[Record] = jan_data + feb_data
#构建MySQL链接对象
conn=Connection(
host="localhost",
port=3306,
user="root",
password="5863AXzy@",
autocommit=True
)
#获得游标对象
cursor=conn.cursor()
#选择数据库
conn.select_db("py_sql")
#组织SQL语句
for record in all_data:
sql = "INSERT INTO orders(order_date, order_id, money, province) VALUES (%s, %s, %s, %s)"
cursor.execute(sql, (record.date, record.order_id, record.money, record.province))
conn.close()
若有侵权,请联系作者