Handling nil
Values in NSDictionary
in Objective-C
When working with Objective-C, particularly when dealing with data returned from a server, it's crucial (至关重要的) to handle nil
values appropriately (适当地) to prevent unexpected crashes. Here, we explore two common ways to insert values into a NSMutableDictionary
and how they behave when the value is nil
.
Scenario (情景)
Consider the following code:
objc
NSString *value = model.value; // Data returned from the server
NSMutableDictionary *dic = @{}.mutableCopy;
[dic setObject:value forKey:@"key"]; // Method 1
dic[@"key"] = value; // Method 2
Let's analyze what happens in each method if value
is nil
.
Method 1: setObject:forKey:
objc
[dic setObject:value forKey:@"key"];
If value
is nil
, this line of code will cause a runtime exception and crash the application. This is because NSMutableDictionary
does not allow nil
values.
Method 2: Keyed Subscript Syntax (键下标语法)
objc
dic[@"key"] = value;
If value
is nil
, using the keyed subscript syntax (键下标语法) will remove the key-value pair from the dictionary if it exists, or do nothing if it doesn't. This method is safer as it avoids crashes and handles nil
values gracefully (优雅地).
Conclusion
- Method 1 (
setObject:forKey:
) will crash if thevalue
isnil
. - Method 2 (keyed subscript
[]
) will safely remove the key ifvalue
isnil
without crashing.
Best Practice
To avoid potential (潜在的) crashes and ensure your code handles nil
values appropriately (适当地), use the keyed subscript (键下标) method or check for nil
before inserting into the dictionary:
objc
if (value != nil) {
dic[@"key"] = value;
} else {
// Handle the nil case, e.g., log or set a default value
NSLog(@"Warning: value is nil");
dic[@"key"] = @"default"; // Or choose not to set the key
}
This approach increases the robustness (健壮性) of your code by preventing unexpected crashes and handling nil
values in a controlled manner.
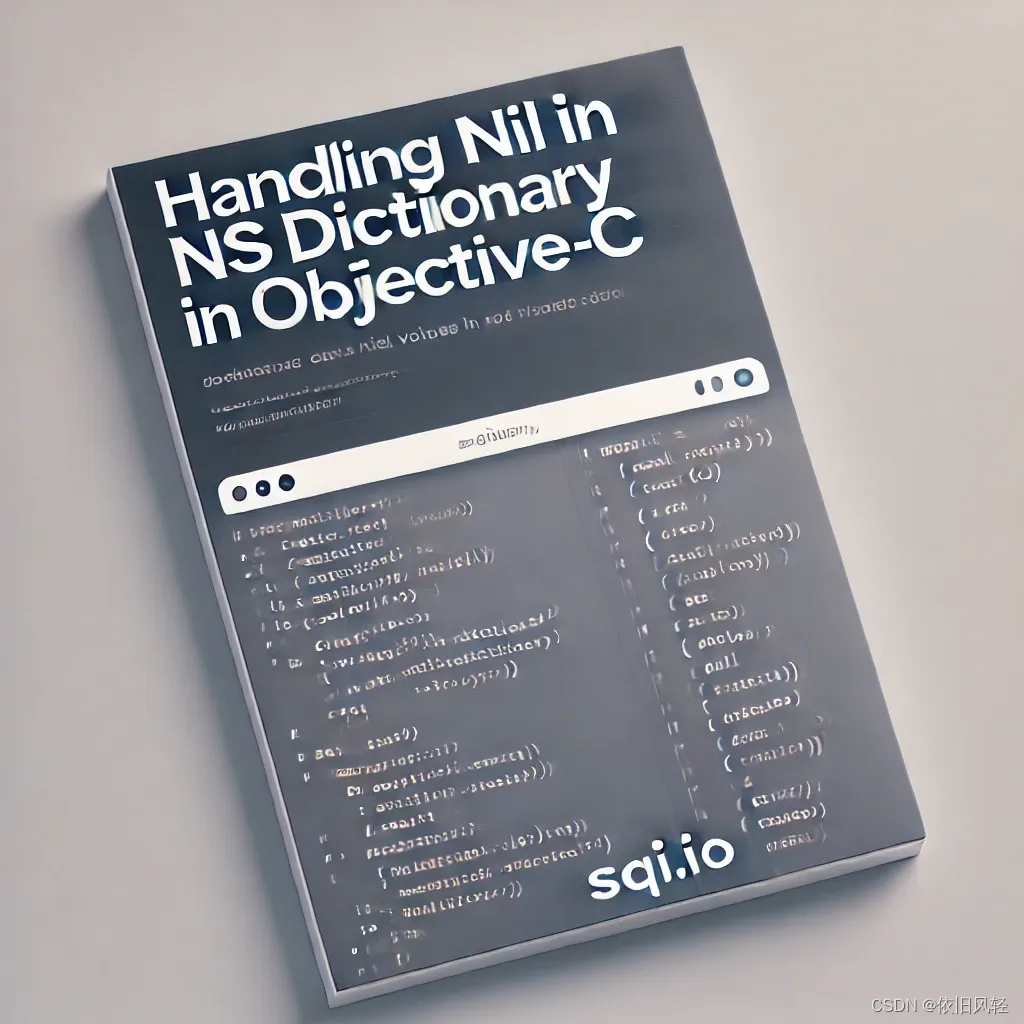