c++面相对象的三大特性为:封装、集成、多态
c++ 认为万事万物都皆为对象,对象上有其属性和行为
一、类和对象(封装)
(一)封装的意义
封装是c++面相对象的三大特性之一
封装的意义:
- 将属性和行为作为一个整体,表现生活中的事物
- 将属性和行为加以权限控制
封装意义一:
- 在设计类的时候,属性和行为卸载一起,表现事物
- 语法: class 类名 {访问权限: 属性 / 行为};
示例1:设计一个圆类,求圆的周长
cpp
#include <iostream>
using namespace std;
const double PI = 3.14;
//设计一个圆类,求圆的周长
//圆求周长的公式:2*PI*半径
class Circle {
//访问权限
//公共权限
public:
//属性
//半径
int m_r;
//行为
//h获取圆的周长
double calculateZC() {
return 2 * PI * m_r;
}
};
int main(){
//通过圆类 创建具体的圆(对象)
Circle c1;
//给圆对象的属性进行赋值
c1.m_r = 10;
cout << "圆的周长为:" << c1.calculateZC() << endl;
system("pause");
return 0;
}
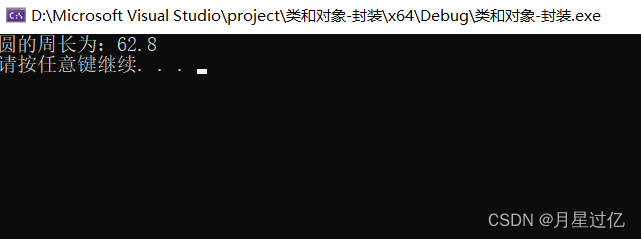
示例2:设计一个学生类,属性有姓名和学号,乐意给姓名和学号赋值,可以显示学生的姓名和学号。
cpp
#include<iostream>
#include<string>
using namespace std;
//学生类
class Student {
//类中的属性和行为 我们统一称为成员
//属性 成员属性 成员变量
//行为 成员函数 成员方法
public:
string s_name;//姓名
int s_id;//学号
//设置姓名
void setName(string name) {
s_name = name;
}
//设置学号
void setId(int id) {
s_id = id;
}
//显示姓名
void showName() {
cout<<"姓名:"<<s_name<<endl;
}
void showId() {
cout << "学号:" << s_id << endl;
}
};
int main() {
Student s1;
s1.setName("张三");
s1.setId(1001);
s1.showName();
s1.showId();
system("pause");
return 0;
}
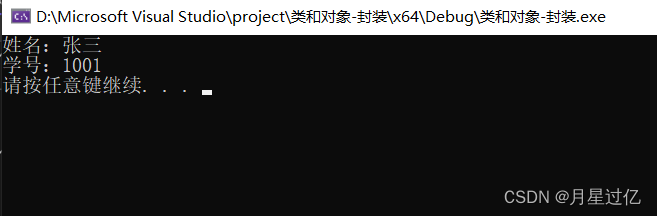
封装意义二:
类在设计时,可以把属性和行为放在不同的权限下,加以控制
访问权限有三种:
- public 公共权限
- protected 保护权限
- private 私有权限
cpp
#include <iostream>
using namespace std;
//访问权限
//三种
//公共权限 public 成员类内可以访问,类外可以访问
//保护权限 protected 类内可以访问,类外不能访问 儿子也可以访问父亲中保护的内容
//私有权限 private 类内可以访问,类外不能访问 儿子不可以访问父亲私有的内容
class Person {
//公共权限
public:
string m_Name;//姓名
protected:
//保护权限
string m_Car;//汽车
private:
//私有权限
int m_Password;//银行卡密码
//类内可以访问
private:
void func() {
m_Name = "张三";
m_Car = "宝马";
m_Password = 123456;
}
};
int main() {
//实例化具体对象
Person p1;
p1.m_Name = "李四";
//类外访问不到
//p1.m_Car = "奔驰";
//p1.m_Password = 654321;
//p1.func();
system("pause");
return 0;
}
(二)struct 和class的区别
在c++中struct和class唯一的区别就在于默认的访问权限不同
区别:
- struct 默认权限为公共
- class 默认权限为私有
cpp
#include <iostream>
using namespace std;
//struct 和class的区别
//struct 默认权限是 公共 public
//class 默认权限是 私有 private
class C1 {
int m_A;//默认权限 是私有
};
struct C2 {
int m_A;//默认权限 是公共
};
int main() {
C1 c1;
//c1.m_A = 10; //无法访问 访问权限是私有
C2 c2;
c2.m_A = 20; //可以访问 访问权限是公共
system("pause");
return 0;
}
(三)成员属性设置私有
**优点1:**将所有成员属性设置为私有,可以自己控制读写权限
**优点2:**对于写权限,我们可以检测数据的有效性
cpp
#define _CRT_SECURE_NO_WARNINGS
#include <iostream>
#include <string>
using namespace std;
//成员属性设置为私有
//1、可以自己控制读写权限
//2、对于写可以检测数据的有效性
//人类
class Person {
public:
//设置姓名
void setName(string name) {
m_Name = name;
}
//获取姓名
string getName() {
return m_Name;
}
//设置年龄
void setAge(int age) {
if (age >= 0 && age <= 150) {
m_Age = age;
}
else {
cout << "年龄不合法" << endl;
}
}
//获取年龄
int getAge() {
return m_Age;
}
//设置偶像
void setIdol(string idol) {
m_Idol = idol;
}
//获取偶像
string getIdol() {
return m_Idol;
}
private:
string m_Name;//姓名 可读可写
int m_Age=18;//年龄 只读
string m_Idol;//偶像 只写
};
int main() {
Person p;
p.setName("张三");
cout<<"姓名:" << p.getName()<<endl;
p.setAge(160);
cout << "年龄:" << p.getAge() << endl;
//p.setIdol("小明");
//cout << "偶像:" << p.getIdol() << endl;
system("pause");
return 0;
}
二、对象的初始化和清理
(一)构造函数和析构函数
对象的初始化和清理也是两个非常重要的安全问题
一个对象或者变量没有初始状态,对其使用后果是未知的
同样的使用完一个对象或变量,没有及时清理,也会造成一定的安全问题
c++利用了构造函数和析构函数解决了上述问题,这两个函数将会被编译器自动调用,完成对象的初始化和清理工作。
对象的初始化和清理工作是编译器强制要求我们做的事情,因此如果我们不提供构造和析构,编译器会提供编译器提供的构造函数和析构函数的空实现。
- 构造函数:主要作用在于创建对象时为对象的成员属性赋值,构造函数由编译器自动调用,无需手动调用
- 析构函数:主要作用在于对象销毁系统自动调用,执行一些清理工作。
**构造函数语法:**类名(){ }
- 构造函数,没有返回值也不写void
- 函数名称与类名相同
- 构造函数可以有参数,因此可以发生重载
- 程序在调用对象时候会自动调用构造,无须手动调用,而且只会调用一次
析构函数语法: ~类名(){ }
- 析构函数,没有返回值也不写void
- 函数名称与类名相同,在名称前加符号~
- 析构函数不可以有参数,因此不可以发生重载
- 程序在对象销毁前会自动调用析构,无须手动调用,而且只会调用一次
cpp
#include <iostream>
using namespace std;
//对象的初始化和清理
//1、构造函数和析构函数
class Person {
//1.1 构造函数
//没有返回值 不用写void
//函数名 与类名相同
//构造函数可以有参数,可以发生重载
//创建对象时,构造函数会自动调用,而且只调用一次
public:
Person() {
cout<<"Person()构造函数的调用"<<endl;
}
//2、析构函数
//没有返回值 不用写void
//函数名 与类名相同 在名称前加~
//构造函数不可以有参数,不可以发生重载
//对象在销毁前,析构函数会自动调用,而且只调用一次
~Person() {
cout<<"~Person()析构函数的调用"<<endl;}
};
void test01() {
Person p1;
}
int main() {
test01();
system("pause");
return 0;
}
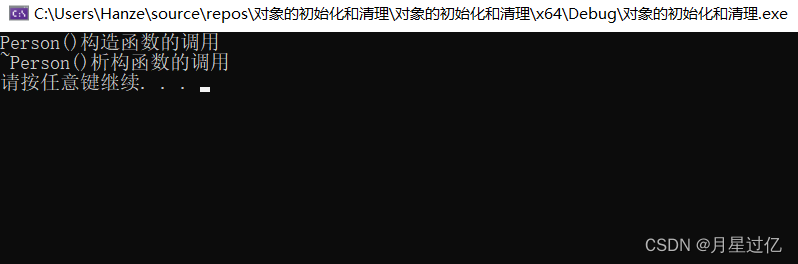
(二)构造函数的分类及调用
两种分类方式:
- 按参数分为:有参构造和无参构造
- 按类型分为:普通构造和拷贝构造
三种调用方式:
- 括号法
- 显示法
- 隐式转换法
cpp
#include<iostream>
using namespace std;
//1.构造函数的分类及调用
//分类
// 按照参数分类 无参构造和有参构造
// 按照类型分类 普通构造函数和拷贝构造函数
class Person {
//构造函数
//无参构造(默认构造函数)
public:
Person() {
age = 18;
cout << "Person()构造函数调用" << endl;
}
//有参构造
Person(int a) {
age = a;
cout << "Person()构造函数调用" << endl;
}
//拷贝构造函数
Person(const Person& p) {
//将传入的人身上的属性,拷贝到我身上
age = p.age;
cout << "Person()拷贝构造函数调用" << endl;
}
//析构函数
~Person() {
cout << "~Person()析构函数调用" << endl;
}
int age;
};
//调用
void test02(){
//1.括号法
Person p1; //调用无参构造函数
Person p2(10); //调用有参构造函数
Person p3(p2); //调用拷贝构造函数
//注意事项1
//调用默认构造函数时不要加()
//因为下面这行代码,编译器会认为是一个函数的声明
Person p4;
void func(); //声明一个函数,但是没有定义
cout << "p2.age=" << p2.age << endl;
cout << "p3.age=" << p3.age << endl;
//2.显示法
Person p5 = Person(10); //调用有参构造函数
Person p6 = Person(p2); //调用拷贝构造函数
Person(10); //匿名对象 特点当前行指向结束后,系统会立即回收掉匿名对象
//注意事项2
//不要利用拷贝构造函数初始化匿名对象 编译器会认为 Person(p3)==Person(p3) 重定义
//Person(p3);//错误
//3.隐式转换法
Person p7 = 10; //调用有参构造函数 相当于 Person p7 =Person(10)
Person p8 = p2; //调用拷贝构造函数 相当于 Person p8 =Person(p2)
//注意事项3
}
int main() {
test02();
}
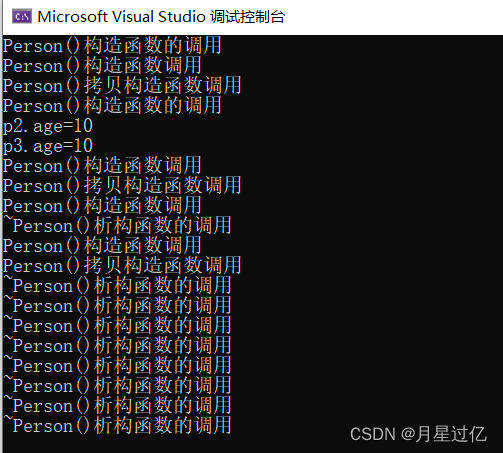
(三)拷贝构造函数调用时机
c++中拷贝构造函数调用时机通常有三种情况
- 使用一个已经创建完毕的对象来初始化一个新对象
- 值传递的方式给函数参数传值
- 以值方式返回局部对象
cpp
#include <iostream>
using namespace std;
//拷贝构造函数调用时机
//1.使用一个已经创建完毕的对象来初始化一个新对象
//2.值传递的方式给函数参数传值
//3.以值方式返回局部对象
class Person {
public:
Person() {
cout << "Person()默认构造函数调用" << endl;
}
Person(int age) {
m_Age = age;
cout << "Person(int age)构造函数调用" << endl;
}
Person(const Person& p) {
m_Age = p.m_Age;
cout << "Person(const Person& p)拷贝构造函数调用" << endl;
}
~Person() {
cout << "~Person()析构函数调用" << endl;
}
int m_Age;
};
//1.使用一个已经创建完毕的对象来初始化一个新对象
void test001() {
Person p1(20);
Person p2(p1);
cout << "p1.m_Age=" << p1.m_Age << endl;
cout << "p2.m_Age=" << p2.m_Age << endl;
}
//2.值传递的方式给函数参数传值
void doWork(Person p) {
}
void test002() {
Person p3;
doWork(p3);
}
//3.以值方式返回局部对象
Person doWork2() {
Person p4;
cout << (int*)&p4 << endl;
return p4;
}
void test003() {
Person p5 = doWork2();
cout << (int*)&p5 << endl;
}
int main() {
//test001();
//test002();
test003();
system("pause");
return 0;
}
(四)构造函数调用规则
默认情况下,c++编译器至少给一个类添加3个函数
- 默认构造函数(无参,函数体为空)
- 默认析构函数(无参,函数体为空)
- 默认拷贝构造函数,对属性进行拷贝
构造函数调用规则如下:
- 如果用户定义有参构造函数,c++不在提供无参构造,但是会提供默认拷贝构造
- 如果用户定义拷贝构造函数,c++不会再提供其它构造函数
cpp
#include <iostream>
using namespace std;
//默认情况下,c++编译器至少给一个类添加3个函数
//默认构造函数(无参,函数体为空)
//默认析构函数(无参,函数体为空)
//默认拷贝构造函数,对属性进行拷贝
class Person {
public:
//Person() {
// cout << "Person 的默认构造函数调用" << endl;
//}
//Person(int age) {
// m_age = age;
// cout << "Person 的有参构造函数调用" << endl;
//}
Person(const Person& p) {
m_age = p.m_age;
cout << "Person 的拷贝构造函数调用" << endl;
}
//~Person() {
// cout << "Person 的默认析构函数调用" << endl;
//}
int m_age;
};
void test12() {
Person p1;
p1.m_age = 18;
Person p2(p1);
cout << "p2 age: " << p2.m_age << endl;
}
int main() {
system("pause");
return 0;
}
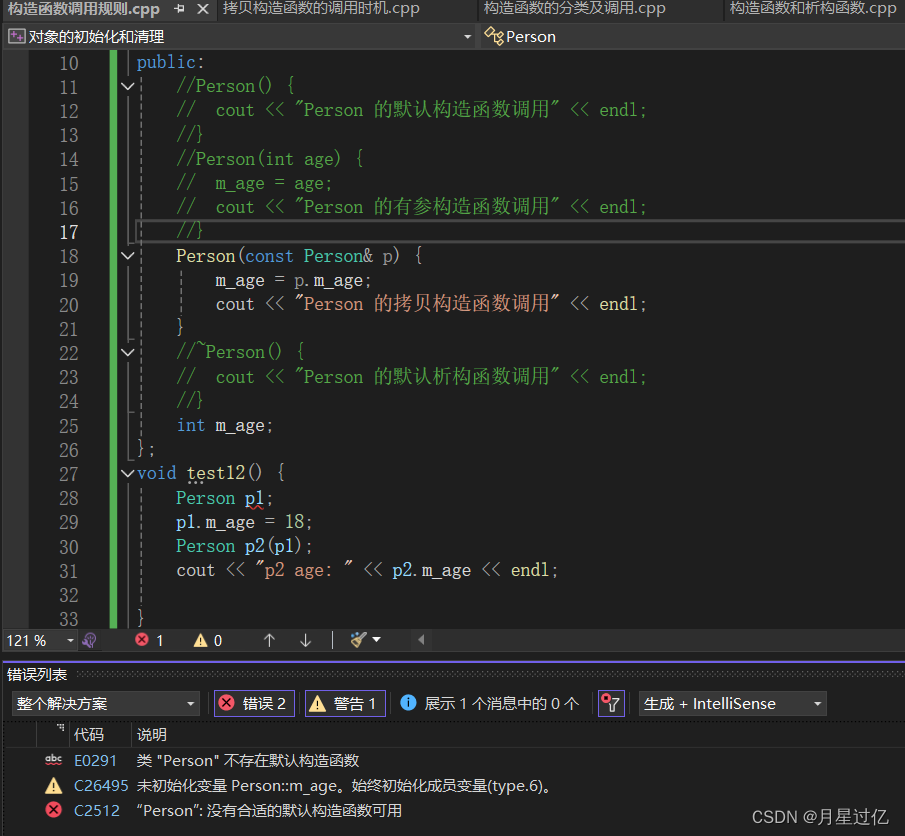
(五)深拷贝与浅拷贝
浅拷贝:简单的赋值拷贝操作
深拷贝:在堆区重新申请空间,进行拷贝操作
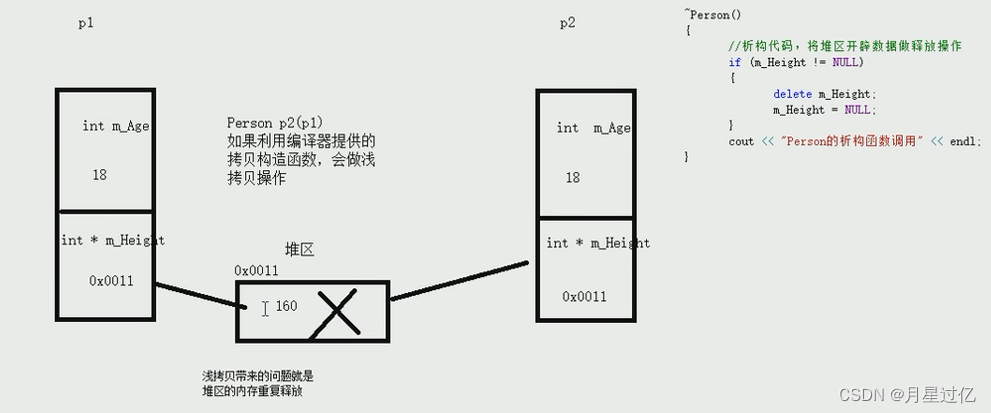
cpp
#include <iostream>
#include <string>
using namespace std;
//深拷贝与浅拷贝
class Person {
public:
Person() {
cout << "Person的默认函数调用" << endl;
}
Person(int age,int height) {
p_age = age;
p_Height = new int(height);
cout << "Person的有参构造函数调用" << endl;
}
//自己实现拷贝构造函数 解决浅拷贝带来的问题
Person(const Person& p) {
p_age = p.p_age; //浅拷贝
p_Height = new int(*p.p_Height);//深拷贝
cout << "Person的拷贝构造函数调用" << endl;
}
~Person() {
//析构代码,将堆区开辟数据做释放操作
if (p_Height!= NULL) {
delete p_Height;
p_Height = NULL;
}
cout<<"Person的析构函数调用"<<endl;
}
public:
int p_age;
int* p_Height;
};
void test006() {
Person p1(18, 170);
Person p2(p1);//值传递 浅拷贝
cout << "p1的年龄为:" << p1.p_age <<"p1身高为:"<< *p1.p_Height << endl;
cout << "p2的年龄为:" << p2.p_age << "p2身高为:" << *p2.p_Height << endl;
}
int main() {
test006();
system("pause");
return 0;
}
(六)初始化列表
作用:
C++提供了初始化列表语法,用来初始化属性
语法:构造函数():属性1(值1),属性2(值2)....{ }
cpp
#include <iostream>
using namespace std;
class Person {
public:
// 使用初始化列表正确初始化属性
Person(int a , int b , int c ) : m_A(a), m_B(b), m_C(c) {
}
int m_A;
int m_B;
int m_C;
};
void testt() {
Person p(10, 20, 30);
cout << "m_A:" << p.m_A << endl;
cout << "m_B:" << p.m_B << endl;
cout << "m_C:" << p.m_C << endl;
}
int main() {
testt();
system("pause");
return 0;
}
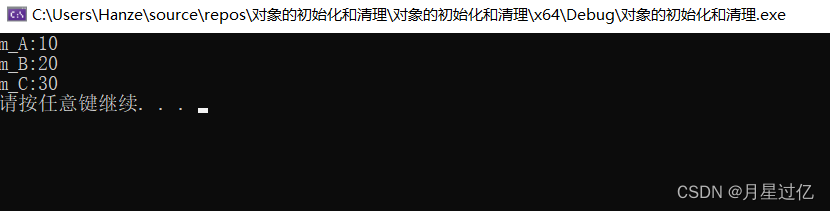
(七)类对象作为类成员
c++类中的成员可以是另一个类的对象,我们称该成员为对象成员
cpp
#include <iostream>
using namespace std;
//类对象作为类成员
class Phone {
public:
Phone(string Pname) {
cout << "Phone对象构造函数" << endl;
m_Pname = Pname;
}
~Phone() {
cout << "Phone对象析构函数" << endl;
}
//品牌
string m_Pname;
};
class Person
{public:
Person(string name, string pName):m_name(name), m_phone(pName){
cout << "Person对象构造函数" << endl;
}
~Person() {
cout << "Person对象析构函数" << endl;
}
//姓名
string m_name;
//手机
Phone m_phone;
};
// 当其他类对象作为本类成员,构造时候先构造类对象再构造自身,析构的顺序与构造相反
void testt02() {
Person p1("张三", "小米");
cout << p1.m_name << "的手机品牌是:" << p1.m_phone.m_Pname << endl;
}
int main() {
testt02();
system("pause");
return 0;
}
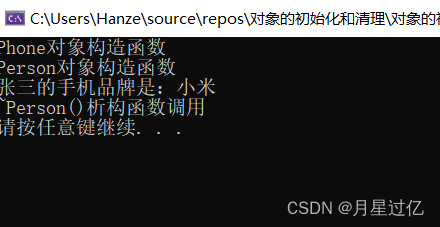
(八)静态成员
静态成员就是在成员变量和成员函数前加上关键字static,称为静态成员
静态成员分为:
- 静态成员变量
- 所有对象共享同一份数据
- 在编译阶段分配内存
- 类内声明,类外初始化
- 静态成员函数
- 所有对象共享一个函数
- 静态成员函数只能访问静态成员变量
cpp
#include <iostream>
using namespace std;
//静态成员变量
class Person {
public:
//静态成员函数
static void func() {
m_A = 100; //修改静态成员变量
//m_B = 200; 静态函数不能访问非静态的成员变量
cout << "static void func()调用" << endl;
}
//1.所有对象都共享同一份数据
//2.编译阶段就分配内存
//3.类内声明,类外初始化操作
static int m_A; //类内声明
int m_B;
};
int Person::m_A = 10;//类外初始化
void test66() {
Person p;
cout << p.m_A << endl; //输出10
Person p2;
p2.m_A = 200; //修改静态成员变量
cout << p.m_A << endl; //输出200
}
void test67() {
//静态成员变量 不属于某个对象,所有对象都共享同一份数据
// 因此静态成员变量有两种访问方式
//1.通过对象进行访问
Person p;
cout << p.m_A << endl;
//2.通过类名进行访问
cout << Person::m_A << endl; //输出10
}
void test68() {
//1.通过对象来访问
Person p;
p.func(); //调用静态成员函数
//2.通过类名来访问
Person::func(); //调用静态成员函数
}
int main() {
test66();
test67();
test68();
system("pause");
return 0;
}
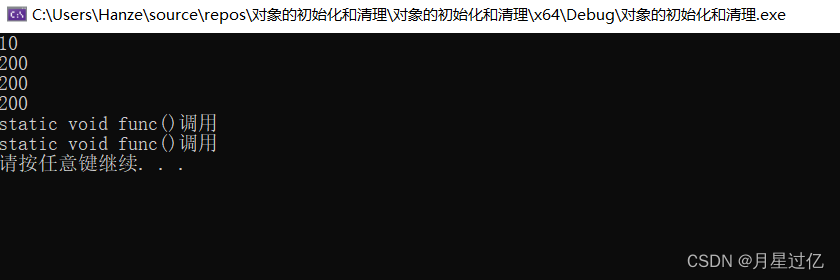
三、c++对象模型和this指针
(一)成员变量和成员函数分开存储
在c++中,类内的成员变量和成员函数分开存储
只有非静态成员变量才属于类的对象上
cpp
#include<iostream>
using namespace std;
//成员变量和成员函数分开存储
class Person {
public:
int m_A;//非静态成员变量,属于类的对象
static int m_B;//非静态成员变量
void func() {//不属于类的对象 不计在类的对象大小中
}
static void staticFunc() {}//静态成员函数,属于类本身,不属于类的对象 不计在类的对象大小中
};
int Person::m_B = 100; //静态成员变量,属于类本身,不属于类的对象 不计在类的对象大小中
void test01() {
Person p;
//空对象占用的内存空间为: 1
//c++编译器会给每个空对象也分配一个字节的空间,是为了区分空对象占内存的位置
//每个空对象也应该有一个独一无二的地址 所以分配1字节的内存空间 来区分空对象
cout << "sizeof(Person)=" << sizeof(p) << endl; // 1字节
}
int main() {
test01();
system("pause");
return 0;
}
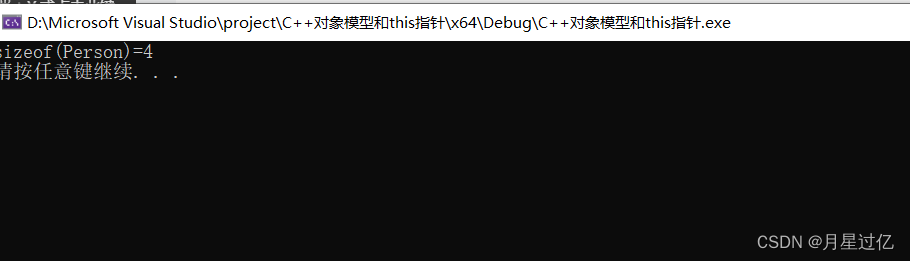
(二)this指针概念
每一个非静态成员函数智慧诞生一份函数实例,也就是说多个同类型的对象会共用一块代码
那么问题是:这一块代码是如何区分哪个对象调用的自己呢?
c++通过提供特殊的对象指针,this指针,解决上述问题,this指针指向被调用的成员函数所属的对象。
- this指针是隐含每一个非静态成员函数内的一种指针
- this指正不需要定义,直接使用即可
this指针的用途
- 当形参和成员变量同名时,可以用this指针来区分
- 在类的非静态成员函数中返回对象本身,可使用return *this
cpp
#include <iostream>
using namespace std;
class Person {
public:
Person(int age) {
//this指针指向被调用的成员函数所属的对象,谁调用指向谁
this->age = age;
}
Person& PersonAddAge(Person& p) {
this->age += p.age;
return *this;
}
int age;
};
//1.解决名称冲突
void test03() {
Person p(20);
cout << "age:" << p.age << endl;
}
//返回对象使用 *this
void test04() {
Person p1(10);
Person p2(10);
p2.PersonAddAge(p1).PersonAddAge(p1).PersonAddAge(p1);
cout << "p2age:" << p2.age << endl;
}
int main() {
test03();
test04();
system("pause");
return 0;
}
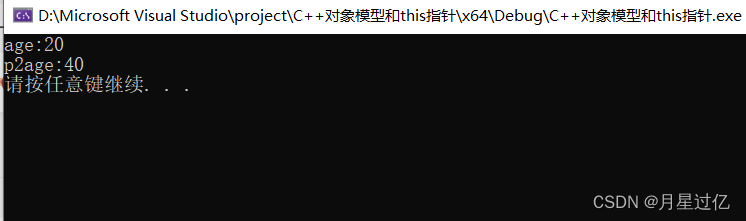
(三)空指针访问成员函数
c++中空指针也是可以调用成员函数的,但是也要注意有没有用到this指针
如果用到this指正,需要加以判断保证代码的健壮性
cpp
#include <iostream>
using namespace std;
//空指针调用成员函数
class Person {
public:
void showClassName() {
cout << "Person" << endl;
}
//报错原因是因为传入的指针是位NULL
void showPersonAge() {
if (this == NULL) {
cout << "this is NULL" << endl;
return;
}
cout << "Person age is " << this->m_Age << endl;
}
int m_Age;
};
void test05() {
Person* p = NULL;
p->showClassName(); //空指针调用成员函数,会导致程序崩溃
p->showPersonAge();//空指针调用成员函数,会导致程序崩溃
}
int main() {
test05();
system("pause");
return 0;
}
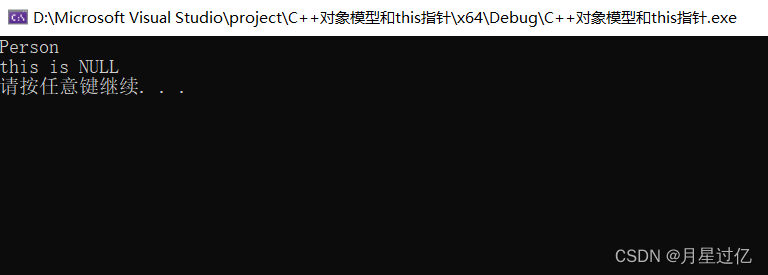
(四)const修饰成员函数
常函数
- 成员函数后加const后我们称为这个函数为常函数
- 常函数内不可以修改成员属性
- 成员属性声明时加关键字mutable后,在常函数中依然可以修改
常对象:
- 声明对象前加const称改对象为常对象
- 常对象只能调用函数
cpp
#include <iostream>
using namespace std;
//常函数
class Person {
public:
//this指针的本质 是指针常量 指针的指向是不可以修改的
//Person * const this;指针指向的值可以修改
void showPerson() const//限定指针指向的值不可以修改
{
//this-> m_A = 100;this指针不可以修改指针的指向
this->m_B = 200; //可以修改变量的值
cout << "m_B=" << this->m_B << endl;
}
void showPerson2() {
this->m_A = 100;
}
int m_A;
mutable int m_B; //特殊变量,即使在常函数中,也能修改变量的值
};
//常对象
void test06() {
const Person p; //常对象
//p.m_A = 100; //常对象不能修改变量的值
p.m_B = 200; //可以修改变量的值
//常对象只能调用常函数
p.showPerson(); //调用常函数
//常对象不能调用普通成员函数
//p.showPerson2(); //调用常函数
}
int main() {
Person p;
p.showPerson(); //调用常函数
system("pause");
return 0;
}
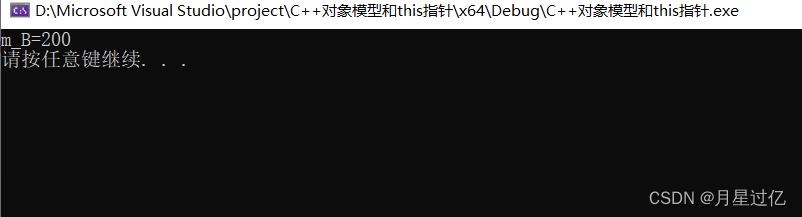
四、友元
友元的目的就是让一一个函数或者类访问另一个类中的私有成员
友元的关键字为 friend
友元的三种实现:
- 全局函数做友元
- 类做友元
- 成员函数做友元
(一)全局函数做友元
cpp
#include <iostream>
#include <string>
using namespace std;
//建筑物类
class Building {
//声明 goodGay全局函数作为Buildding 类的好朋友 可以访问私有成员
friend void goodGay(Building* building);
public:
Building() {
m_SettingRoom = "客厅";
m_Bedroom = "卧室";
}
string m_SettingRoom;//客厅
private:
string m_Bedroom; //卧室
};
//全局函数
void goodGay(Building* building) {
cout << "好基友全局函数 正在访问:" << building->m_SettingRoom << endl;
cout << "好基友全局函数 正在访问:" << building->m_Bedroom << endl;
}
void test01() {
Building building;
goodGay(&building);
}
int main() {
test01();
system("pause");
return 0;
}
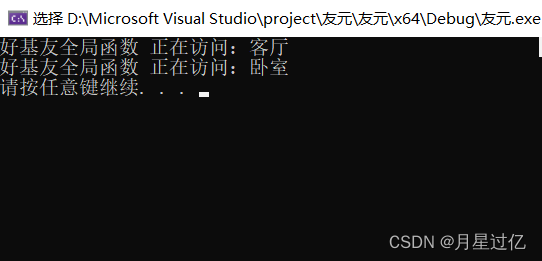
(二)类做友元
cpp
#include <iostream>
using namespace std;
//类做友元
class Building;
class GoodGay {
public:
GoodGay();
void visit();//参观函数访问Building中的属性
Building *building;
};
class Building {
friend class GoodGay;//声明GoodGay为友元类
public:
Building();
string m_SettingRoom;//客厅
private:
string m_Bedroom; //卧室
};
//类外写成员函数
Building::Building() {
string m_SettingRoom;//客厅
string m_Bedroom; //卧室
}
GoodGay::GoodGay() {
//创建建筑物对象
building = new Building;
}
void GoodGay::visit() {
cout << "GoodGay正在参观" << building->m_SettingRoom << "客厅" << endl;
//访问building对象的私有属性
cout << "GoodGay正在参观" << building->m_Bedroom << "卧室" << endl;
}
void test01() {
GoodGay gg;
gg.visit();
}
int main() {
test01();
system("pause");
return 0;
}
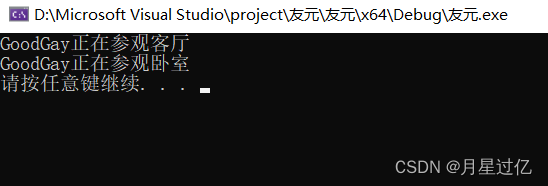
(三)成员函数做友元
cpp
#include <iostream>
#include <string>
using namespace std;
class Building;
class GoodGay {
public:
GoodGay();
void visit();//让 visit()函数能够访问Builing类的私有成员变量
void visit2();//让 visit2()函数不能够访问Builing类的私有成员变量
Building* building;
};
class Building {
//告诉编译器 GoodGay类下的visit()函数可以访问Building类的私有成员变量
friend void GoodGay:: visit();
public:
Building();
public:
string m_SittingRoom;//客厅
private:
string m_BedRoom;//卧室
};
Building::Building() {
m_SittingRoom = "客厅";
m_BedRoom = "卧室";
}
void GoodGay:: visit() {
cout<<"visit函数正在访问"<<building->m_SittingRoom<<endl;
cout<<"visit函数正在访问"<<building->m_BedRoom<<endl;
}
void GoodGay:: visit2() {
cout<<"visit2函数正在访问"<<building->m_SittingRoom<<endl;
//cout<<"visit2函数正在访问"<<building->m_BedRoom<<endl;
}
GoodGay::GoodGay() {
building = new Building();
}
void test01() {
GoodGay g;
g.visit();
g.visit2();
}
int main() {
test01();
system("pause");
return 0;
}
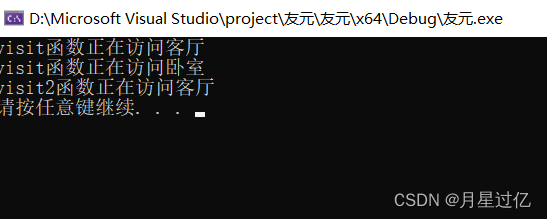
五、运算符重载
运算符重载概念:对已有的运算符重新进行定义,赋予其另一种功能,以适应不同的数据类型
(一)加号运算符重载
作用:实现两个自定义数据类型相加的运算
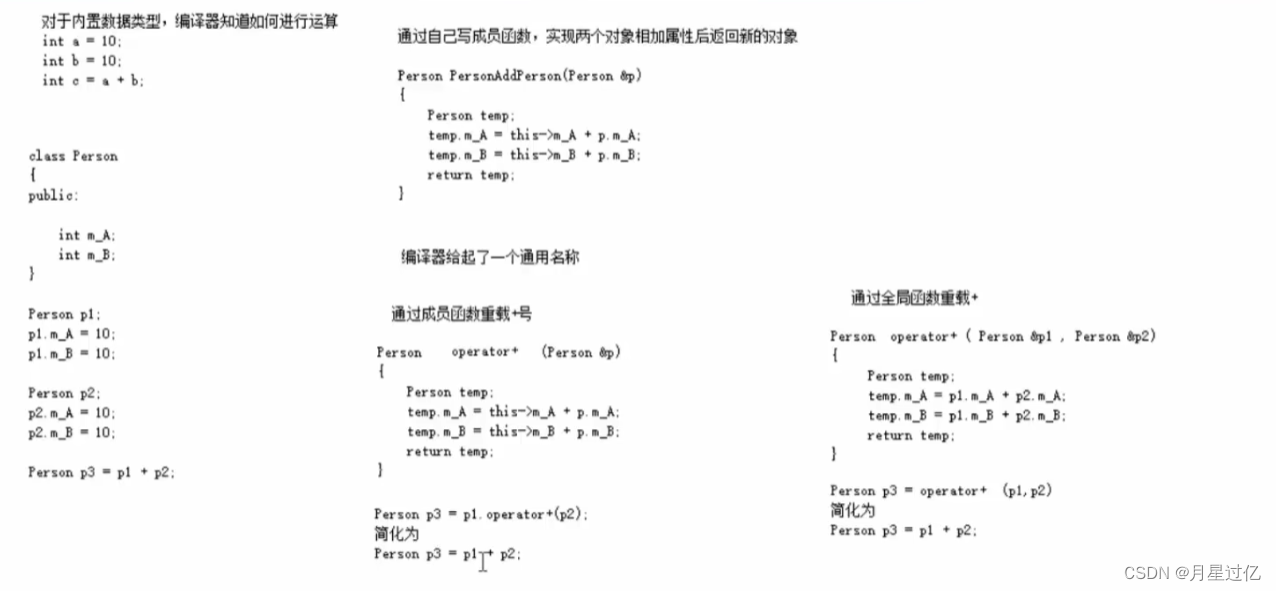
cpp
#include <iostream>
using namespace std;
//加号运算符重载
class Person {
public:
//1.成员函数重载+号
//Person operator+(Person& p) {
//Person temp;
//temp.m_A =this -> m_A + p.m_A;
//temp.m_B =this -> m_B + p.m_B;
//return temp;
//}
int m_A;
int m_B;
};
//2.全局函数重载+号
Person operator+(Person& p1, Person& p2) {
Person temp;
temp.m_A = p1.m_A + p2.m_A;
temp.m_B = p1.m_B + p2.m_B;
return temp;
//运算符重载 函数也可以重载
}
void test01(){
Person p1, p2;
p1.m_A = 10;
p1.m_B =10;
p2.m_A = 10;
p2.m_B = 10;
Person p3 = p1 + p2;
cout << "p3.m_A = " << p3.m_A << endl;
cout << "p3.m_B = " << p3.m_B << endl;
}
//2.全局函数重载+号
int main() {
test01();
system("pause");
return 0;
}
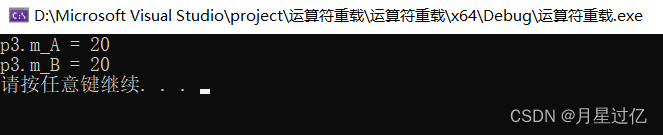
(二)左移运算符重载
作用:可以输出自定义数据类型
cpp
#include <iostream>
using namespace std;
//左移运算符重载
class Person
{
public:
//利用成员函数 重载左移运算符 p.operator <<(cout) 简化版本 p<< cout
// 不会利用成员函数重载<<运算符,因为无法实现 cout在左侧
//
/*void operator <<() {
}*/
int m_A;
int m_B;
};
//只能利用全局函数重载<<运算符
// 不能利用成员函数重载<<运算符
ostream& operator << ( ostream& cout, Person& p) {
cout << "m_A = " << p.m_A << " m_B = " << p.m_B << endl;
return cout;
}
void test02() {
Person p1;
p1.m_A = 10;
p1.m_B = 10;
cout << "p1.m_A = " << p1.m_A << endl;
cout << "p1.m_B = " << p1.m_B << endl;
cout << p1<<endl;
}
int main() {
test02();
system("pause");
return 0;
}
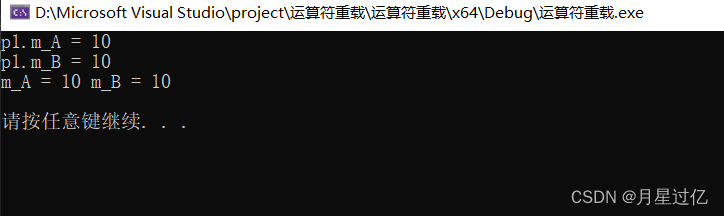
(三)递增运算符重载
cpp
#include <iostream>
using namespace std;
//递增运算符重载
//自定义整型
class MyInteger {
friend ostream& operator <<(ostream& cout, MyInteger myInt);
public:
MyInteger() {
m_Num = 0;
}
//重载前置++运算符 返回引用是为了一直对一个数据进行递增操作
MyInteger& operator ++() {
//先进行++运算
m_Num++;
//将自身返回
return *this;
}
//重载后置++运算符
//void operator ++(int) int 代表占位参数,可以用于区分前置和后置递增
MyInteger operator ++(int){ //后置递增返回值 前置递增返回引用
//先记录当时结果
MyInteger temp = *this;
//后递增
m_Num++;
//最后将记录结果返回
return temp; //局部变量 函数结束后销毁 所以只能返回值
}
private:
int m_Num;
};
//重载左移运算符
ostream& operator <<(ostream& cout, MyInteger myInt) {
cout<<myInt.m_Num;
return cout;
}
void test03() {
MyInteger myInt;
cout << ++myInt << endl;
}
void test04() {
MyInteger myInt;
cout << myInt++ << endl;
}
int main() {
test03();
test04();
system("pause");
return 0;
}
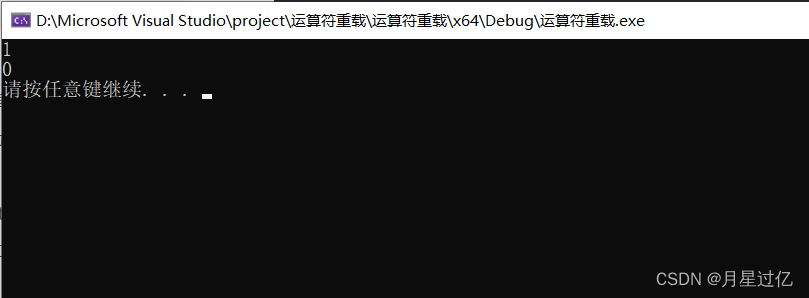
(四)赋值运算符重载
c++编译器至少给一个类添加4个函数
- 默认构造函数(无参,函数体为空)
- 默认析构函数(无参,函数体为空)
- 默认拷贝构造函数,对属性进行值拷贝
- 赋值运算符 operator =,对属性进行值拷贝
如果类中有属性指向堆区,做赋值操作时也会出现深浅拷贝的问题
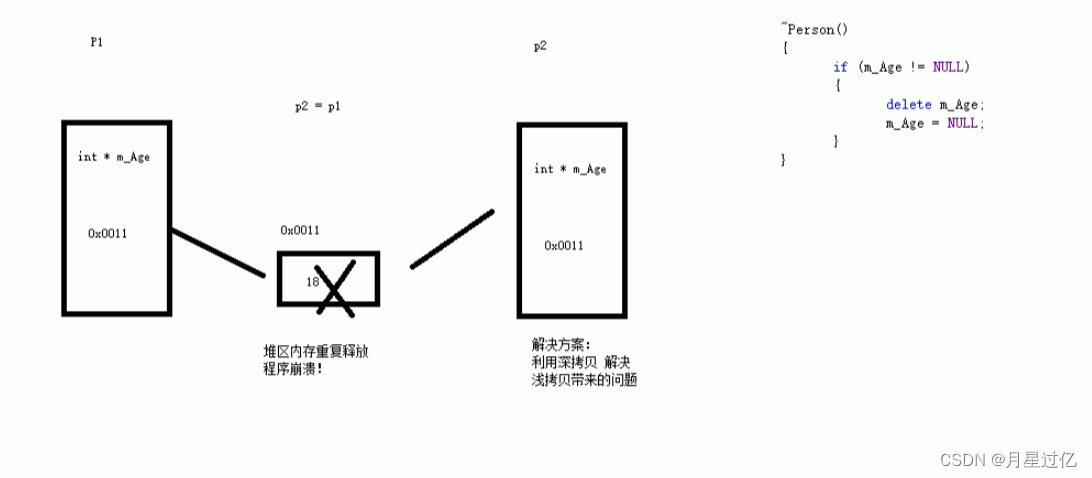
cpp
#include <iostream>
using namespace std;
//赋值运算符重载
class Person {
public:
Person(int age) {
m_Age = new int(age);
}
~Person() {
if (m_Age != NULL) {
delete m_Age;
m_Age = NULL;
}
}
//重载赋值运算符
Person& operator=(Person& p) {
//编译器提供浅拷贝
//this->m_Age = p.m_Age;
//应该先判断是否有属性在堆区,如果有先释放干净,然后再深拷贝
if (m_Age != NULL) {
delete m_Age;
m_Age = NULL;
}
//深拷贝
m_Age = new int(*p.m_Age);
return *this;
}
int * m_Age;
};
void test06() {
Person p1(20);
Person p2(30);
Person p3(40);
p2 = p1= p3;//赋值操作
cout << "p1 age: " <<* p1.m_Age << endl;
cout << "p2 age: " <<* p2.m_Age << endl;
cout << "p3 age: " <<* p3.m_Age << endl;
}
int main() {
test06();
system("pause");
return 0;
}
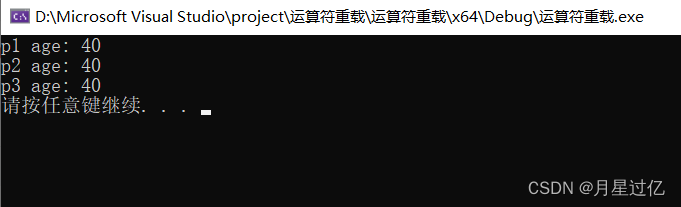
(五)关系运算符重载
cpp
#include <iostream>
using namespace std;
//关系运算符重载
class Person{
public:
Person(string name, int age) {
m_Name = name;
m_Age = age;
}
//重载==号
bool operator ==(Person& p) {
if (this->m_Name == p.m_Name && this->m_Age == p.m_Age) {
return true;
}
return false;
}
string m_Name;
int m_Age;
};
void test08() {
Person p1("Tom", 18);
Person p2("Tom", 18);
if (p1 == p2) {
cout << "p1 == p2" << endl;
}else{
cout << "p1!= p2" << endl;
}
}
int main() {
test08();
system("pause");
return 0;
}
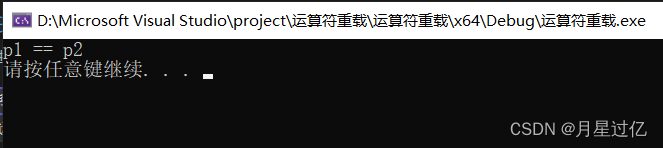
(六)函数调用运算符重载
- 函数调用运算符()也可以重载
- 由于重载后使用方式非常想函数的调用,因此称为仿函数
- 仿函数没有固定写法,非常灵活。
cpp
#include <iostream>
#include <string>
using namespace std;
//函数调用运算符重载
//打印输出类
class MyPrint {
public:
//重载函数调用运算符
void operator()(string test) {
cout << test << endl;
}
};
class MyAdd {
public:
//重载函数调用运算符
int operator()(int a, int b) {
return a + b;
}
};
void test09() {
MyPrint myPrint;
myPrint("Hello, world!");//由于重载后使用方式非常想函数的调用,因此称为仿函数
MyAdd myAdd;
cout << myAdd(10, 20) << endl;
//匿名对象调用
cout << "MyAdd()(100, 200)=" << MyAdd()(100, 200) << endl;
}
int main() {
test09();
system("pause");
return 0;
}
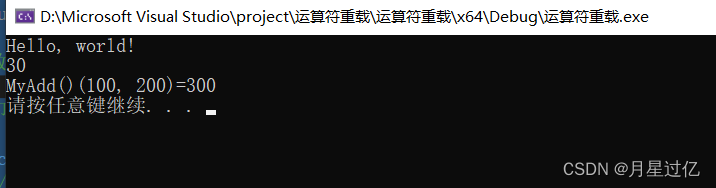
六、继承
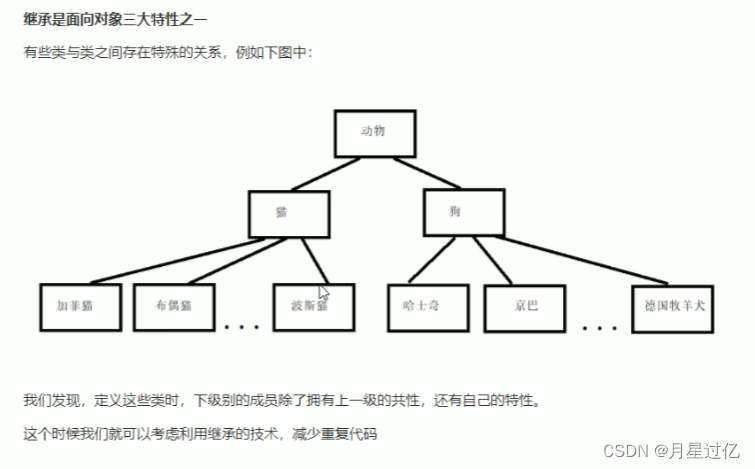
(一)继承的基本语法
总结:
继承的好处:可以减少重复的代码
继承语法 class 子类:继承方式 父类名称{};
子类也称为派生类
父类也称为基类
cpp
#include <iostream>
using namespace std;
//普通实现页面
//class Java {
//public:
// void header() {
// cout << "首页、公开课、登录、注册...(公共头部)" << endl;
// }
// void footer() {
// cout<<"帮助中心、交流合作、站内地图...(公共底部)"<<endl;
// }
// void left() {
// cout << "Java、Python、C++、...(公共分类列表)" << endl;
// }
// void content() {
// cout << "Java学科视频" << endl;
// }
//
//};
//class Python {
//public:
// void header() {
// cout << "首页、公开课、登录、注册...(公共头部)" << endl;
// }
// void footer() {
// cout << "帮助中心、交流合作、站内地图...(公共底部)" << endl;
// }
// void left() {
// cout << "Java、Python、C++、...(公共分类列表)" << endl;
// }
// void content() {
// cout << "Python学科视频" << endl;
// }
//
//};
//class Cpp{
//public:
// void header() {
// cout << "首页、公开课、登录、注册...(公共头部)" << endl;
// }
// void footer() {
// cout << "帮助中心、交流合作、站内地图...(公共底部)" << endl;
// }
// void left() {
// cout << "Java、Python、C++、...(公共分类列表)" << endl;
// }
// void content() {
// cout << "Python学科视频" << endl;
// }
//
//};
//继承实现页面
// 继承好处 减少代码重复
// 继承语法 class 子类:继承方式 父类名称{};
// 子类也称为派生类
// 父类也称为基类
//公共页面类
class BasePage {
public:
void header() {
cout << "首页、公开课、登录、注册...(公共头部)" << endl;
}
void footer() {
cout << "帮助中心、交流合作、站内地图...(公共底部)" << endl;
}
void left() {
cout << "Java、Python、C++、...(公共分类列表)" << endl;
}
};
//Java页面
class Java:public BasePage
{public:
void content() {
cout << "Java学科视频" << endl;
}
};
//Python页面
class Python :public BasePage
{
public:
void content() {
cout << "Python学科视频" << endl;
}
};
//C++页面
class Cpp :public BasePage
{
public:
void content() {
cout << "cpp学科视频" << endl;
}
};
void test01() {
cout << "Java下载视频页面如下:" << endl;
Java java;
java.header();
java.left();
java.content();
java.footer();
cout<<"------------------------------------------"<<endl;
cout << "Python下载视频页面如下:" << endl;
Python python;
python.header();
python.left();
python.content();
python.footer();
cout<<"-"<<endl;
cout << "------------------------------------------" << endl;
cout << "C++下载视频页面如下:" << endl;
Cpp cpp;
cpp.header();
cpp.left();
cpp.content();
cpp.footer();
}
int main() {
test01();
system("pause");
return 0;
}
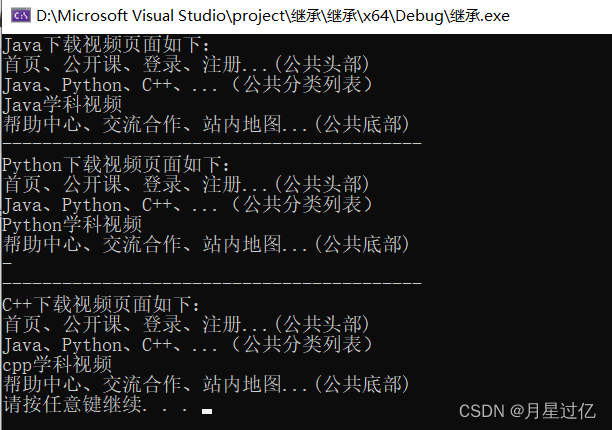
(二)继承方式
继承的语法:class 子类:继承方式 父类
继承方式一共有三种:
- 公共继承
- 保护继承
- 私有继承
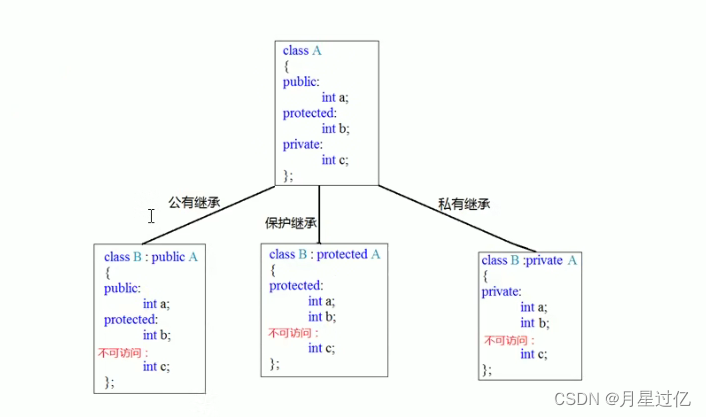
cpp
#include <iostream>
using namespace std;
//继承方式
//公共继承
class Base1 {
public:
int m_A;
protected:
int m_B;
private:
int m_C;
};
class Son1 :public Base1 {
public:
void func() {
m_A = 10;
m_B = 20;//父类中的保护变量可以访问
// m_C = 30; 父类中的私有变量不能访问
}
};
void test02() {
Son1 s1;
s1.m_A = 100;
//s1.m_B = 100; 保护权限类外不能访问
}
//保护继承
class Base2 {
public:
int m_A;
protected:
int m_B;
private:
int m_C;
};
class Son2 :protected Base2 {
public:
void func() {
m_A = 100;//父类中公共成员到子类中变为了保护
m_B = 200;
//m_C = 30; 私有权限类外不能访问
}
};
void test03() {
Son2 s2;
//s2.m_A = 1000; 保护继承类外访问不到
//s2.m_B = 2000;
}
//私有继承
class Base3 {
public:
int m_A;
protected:
int m_B;
private:
int m_C;
};
class Son3 :private Base3 {
public:
void func() {
m_A = 1000;//父类中公共成员到子类变成私有成员
m_B = 2000;//父类中保护成员到子类变成私有成员
//m_C = 3000; 私有权限类外不能访问
}
};
void test04() {
Son3 s3;
//s3.m_A = 10000; 私有继承类外访问不到
//s3.m_B = 20000;
//s3.m_C = 30000;
}
int main() {
test02();
test03();
test04();
system("pause");
return 0;
}
(三)继承中的对象模型
问题:从父类继承过来的成员,哪些属于子类对象中?
父类中私有成员属性,是被编译器隐藏了,因此访问不到,但是确实被继承了下去
cpp
#include <iostream>
using namespace std;
// 继承中的对象模型
class Base {
public:
int m_A;
protected:
int m_B;
private:
int m_C;
};
class Son :public Base {
public:
int m_D;
};
void test05() {
//16
//父类中所有非静态的成员属性都会被子类继承下去
//父类中私有成员属性,是被编译器隐藏了,因此访问不到,但是确实被继承了下去
cout<<"Size of Son=" <<sizeof(Son)<<endl;
}
int main() {
test05();
system("pause");
return 0;
}
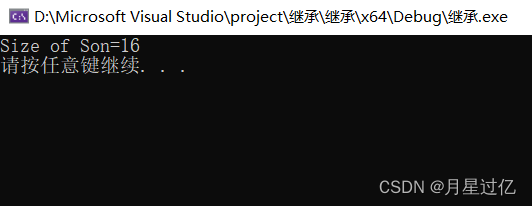
(四)继承中的构造和析构顺序
子类继承父类后,当创建子类对象,也会调用父类的构造函数
问题:父类和子类的构造和析构顺序谁先谁后?
继承中的构造和析构顺序:先构造父类,再构造子类,析构的顺序与构造的顺序相反
cpp
#include <iostream>
using namespace std;
// 继承中构造和析构顺序
class Base {
public:
Base() {
cout << "Base构造函数" << endl;
}
~Base() {
cout << "Base析构函数" << endl;
}
};
class Son:public Base {
public:
Son() {
cout << "Son构造函数" << endl;
}
~Son() {
cout << "Son析构函数" << endl;
}
};
void test001() {
//Base b;
Son s;
}
int main() {
test001();
system("pause");
return 0;
}
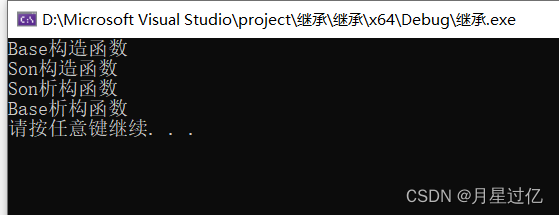
(五)继承同名成员处理方式
问题:当子类与父类出现同名成员,如何通过子类对象,访问到子类或父类中同名的数据呢?
- 访问子类同名成员 直接访问即可
- 访问父类同名成员 需要加作用域
如果子类中出现和父类同名的成员函数,子类的同名成员会隐藏掉父类中所有同名成员函数
如果想访问到父类中被隐藏的同名成员函数,需要使用Base::成员函数名(需要加作用域)
总结
- 子类对象可以直接访问到子类中的同名成员
- 子类对象加作用域可以访问到父类同名成员
- 当子类与父类拥有同名的成员函数,子类会隐藏父类只能怪同名成员函数,加作用域可以访问到父类中的同名函数
cpp
#include <iostream>
using namespace std;
//继承中同名的成员处理方式
class Base {
public:
Base() {
m_A = 100;
}
void func() {
cout << "Base-func()调用" << endl;
}
int m_A;
};
class Son :public Base {
public:
Son() {
m_A = 200;
}
void func() {
cout << "Son-func()调用" << endl;
}
int m_A;
};
void test002() {
Son s;
cout<<"Son 下的m_A:" << s.m_A << endl; //输出200
cout<<"Base 下的m_A:" << s.Base::m_A << endl; //输出100
//如果子类中出现和父类同名的成员函数,子类的同名成员会隐藏掉父类中所有同名成员函数
//如果想访问到父类中被隐藏的同名成员函数,需要使用Base::成员函数名(需要加作用域)
s.func(); //调用Son的func()
//如何调用到父类中同名成员函数?
s.Base::func(); //调用Base的func()
}
//同名的函数处理方式
void test003() {
}
int main() {
test002();
system("pause");
return 0;
}
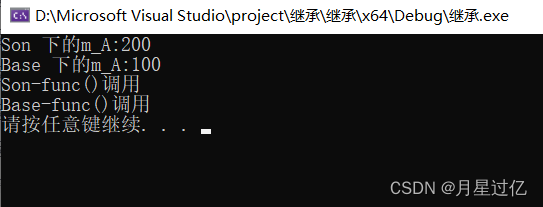
(六)继承同名静态成员处理方式
问题:继承中同名静态成员在子类对象上如何进行访问?
静态成员和非静态成员出现同名,处理方式一致
- 访问子类同名成员 直接访问即可
- 访问父类同名成员 需要加作用域
cpp
#include <iostream>
using namespace std;
//继承同名静态成员处理方式
class Base {
public:
static int m_A;
static void func() {
cout << "Base下的静态函数调用" << endl;
}
};
int Base::m_A = 100;
class Son : public Base {
public:
static int m_A;
static void func() {
cout<<"Son下的静态函数调用"<<endl;
}
};
int Son::m_A = 200;
//同名静态成员属性
void test003() {
//1、通过对象访问
Son s;
cout<<"Son下的m_A:"<<s.m_A<<endl;
cout<<"Base下的m_A:"<<s.Base::m_A<<endl;
//2、通过类名访问
cout<<"Son下的m_A:"<<Son::m_A<<endl;
//第一个::代表通过类名的方式访问 第二个::代表访问父类作用域下
cout<<"Base下的m_A:"<<Son::Base::m_A<<endl;
}
void test004() {
//1、通过对象访问
Son s;
s.func(); //Son下的静态函数调用
s.Base::func(); //Base下的静态函数调用
//2、通过类名访问
Son::func(); //Son下的静态函数调用
Son::Base::func(); //Base下的静态函数调用
}
int main() {
test003();
test004();
system("pause");
return 0;
}
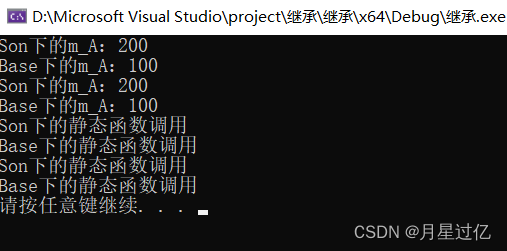
(七)多继承语法
c++允许一个类继承多个类
语法:class 子类:继承方式 父类1,继承方式 父类2...
多继承可能会引发父类中有同名的成员出现,需要加作用域区分
c++实际开发中不建议用多继承
cpp
#include <iostream>
using namespace std;
// 多继承语法
class Base1 {
public:
Base1(){
m_A = 100;
}
int m_A;
};
class Base2 {
public:
Base2() {
m_A = 100;
}
int m_A;
};
//子类 需要继承Base1和Base2
//语法:class 子类:继承方式 父类1,继承方式 父类2...
class Son :public Base1, public Base2 {
public:
Son() {
m_C = 300;
m_D = 400;
}
int m_C;
int m_D;
};
void test07() {
Son s;
cout<<"sizeof(Son)="<<sizeof(s)<<endl;
//当父类中出现了不同的成员,需要加作用域
cout<<"Base1m_A="<<s.Base1::m_A<<endl;
cout<<"Base2m_A="<<s.Base2::m_A<<endl;
}
int main() {
test07();
system("pause");
return 0;
}
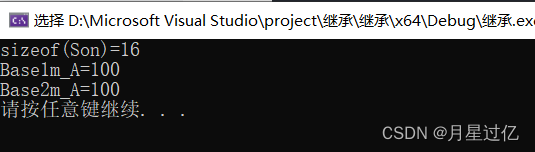
(八)菱形继承
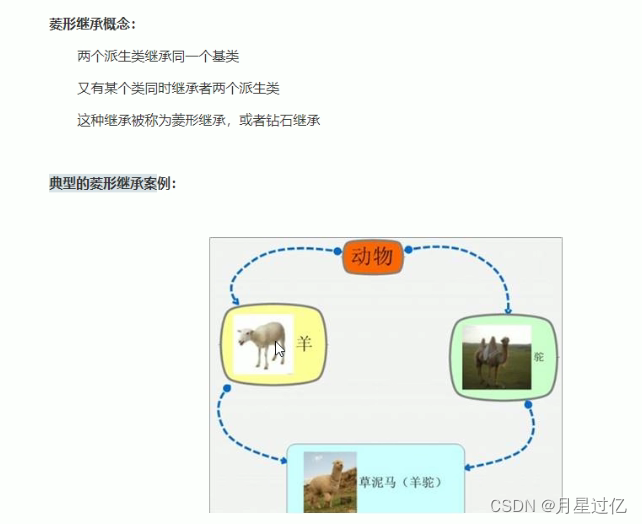
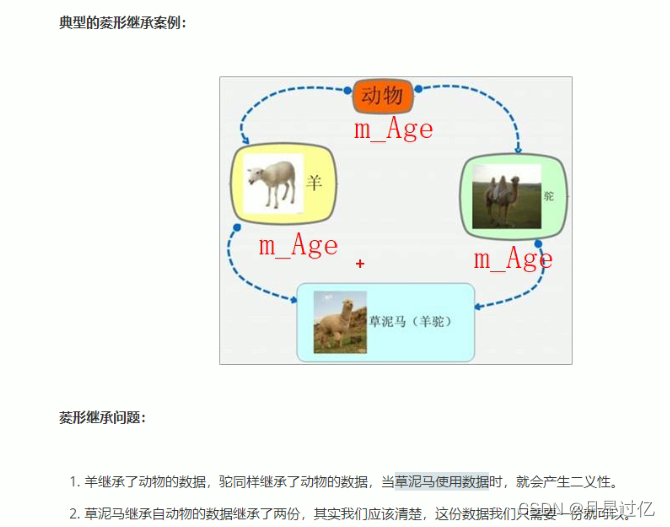
cpp
#include <iostream>
using namespace std;
//动物类
class Animal{
public:
int m_Age;
};
//利用虚继承 解决菱形继承的问题
// 继承之前 加上关键字 virtual 变为虚继承
// Animal类称为 虚基类
//羊类
class Sheep:virtual public Animal{
};
//驼类
class Tuo :virtual public Animal {
};
//羊驼类
class SheepTuo :public Sheep, public Tuo {
};
void test09() {
SheepTuo st;
st.Sheep::m_Age = 10;
st.Tuo::m_Age = 28;
//当菱形继承,两个父类拥有相同数据,需要加以作用域区分
cout<<"st.Sheep::m_Age:" << st.Sheep::m_Age << endl;
cout<<"st.Tuo::m_Age:" << st.Tuo::m_Age << endl;
cout<<"st.m_Age"<<st.m_Age<<endl;
//这份数据我们知道只要有一份就可以了,菱形继承导致有两份导致了资源的浪费
}
int main() {
test09();
system("pause");
return 0;
}
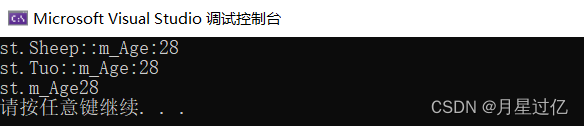
七、多态
(一)多态的基本概念
多态是c++面相对象三大特性之一
多态分为两类:
- 静态多态:函数重载和运算符重载属于静态多态,复用函数名
- 动态多态:派生类和虚函数实现运行时多态
静态多态和动态多态的区别:
- 静态多态的函数地址早绑定 -编译阶段确定函数地址
- 动态多态的函数地址晚绑定-运行阶段确定函数地址
cpp
#include <iostream>
using namespace std;
// 多态的基本概念
class Animal {
public:
//虚函数 地址晚绑定
virtual void speak() {
cout << "动物在说话" << endl;
}
};
//猫类
class Cat :public Animal {
public:
//虚函数 地址晚绑定
void speak() {
cout << "猫在叫" << endl;
}
};
//狗类
class Dog :public Animal {
public:
//虚函数 地址晚绑定
void speak() {
cout << "狗在汪汪叫" << endl;
}
};
//执行说话的函数
//地址早绑定 在编译阶段确定函数地址
//如果想执行让猫说话,那么这个函数地址就不能提前绑定,需要在运行阶段进行绑定,地址晚绑定
void doSpeak(Animal& animal) { //Animal & animal =cat;
animal.speak();
}
//动态多态满足条件
//1.有继承关系
//2.子类要重写父类的虚函数
//动态多态的使用
//父类的指针或者引用指向子类的对象
void test01() {
Cat cat;
doSpeak(cat); // 调用的是猫类的speak函数 动物在说话
Dog dog;
doSpeak(dog);
}
void test02() {
cout<<"sizeof(Animal)="<<sizeof(Animal)<<endl; //8字节(两个指针)
}
int main() {
test01();
test02();
system("pause");
return 0;
}
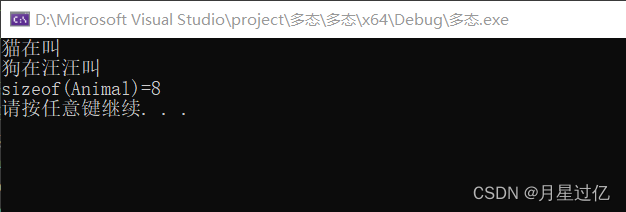
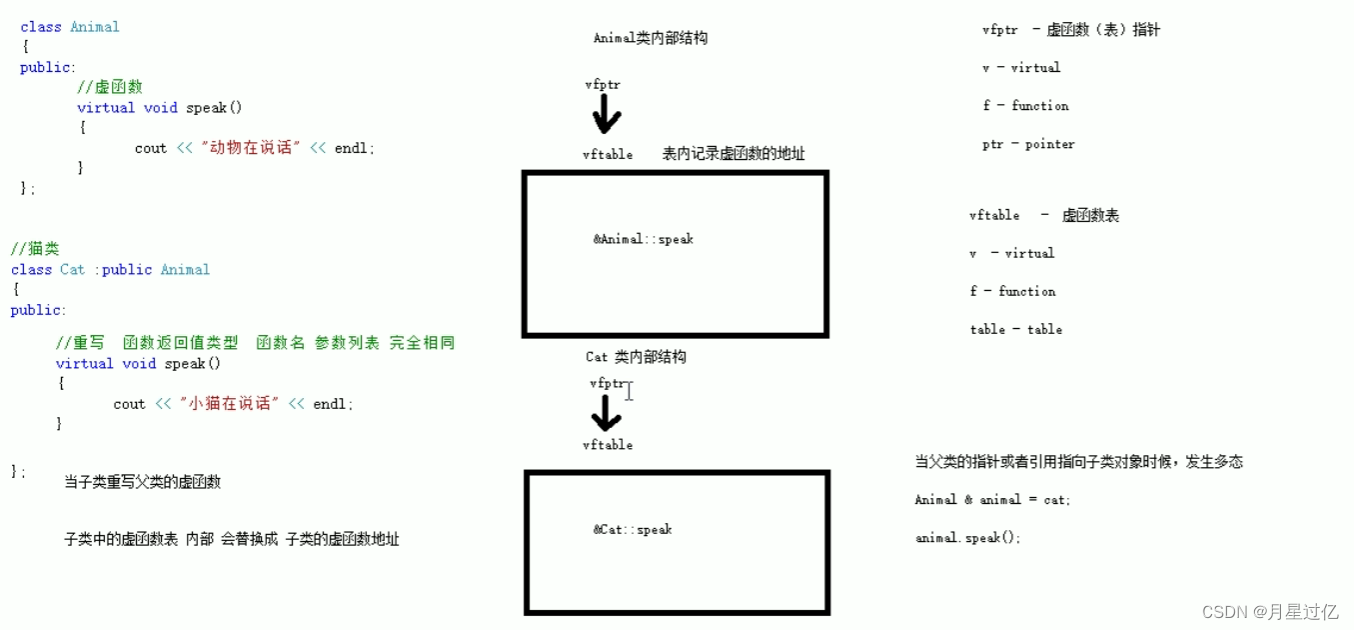
(二)多态案例一 计算器类
案例描述:
分别用普通写法和多态技术,设计实现两个操作数进行运算的计算器类
多态的优点:
- 代码组织结构清晰
- 可读性强
- 利于前期后期的扩展以及维护
cpp
#include <iostream>
#include <string>
using namespace std;
//分别利用普通写法和多态技术实现计算器
//普通写法
class Calculator {
public:
int getResult(string oper) {
if (oper == "+") {
return m_Num1 + m_Num2;
}else if (oper == "-") {
return m_Num1 - m_Num2;
}
else if (oper == "*") {
return m_Num1 * m_Num2;
}
//如果想要扩展新的功能,需要修改源码
//在真实的开发中 提倡 开闭原则
//开闭原则:对扩展进行开放,对修改进行关闭
}
int m_Num1; //操作数1
int m_Num2; //操作数2
};
void test03() {
//创建计算器对象
Calculator c;
c.m_Num1 = 10;
c.m_Num2 = 10;
cout<<c.m_Num1<<"+"<<c.m_Num2<<"="<<c.getResult("+")<<endl;
cout<<c.m_Num1<<"-"<<c.m_Num2<<"="<<c.getResult("-")<<endl;
cout<<c.m_Num1<<"*"<<c.m_Num2<<"="<<c.getResult("*")<<endl;
}
//利用多态实现计算器
//实现计算器抽象类
class AbstracatCalculator {
public:
virtual int getResult() {
return 0;
}
int m_Num1; //操作数1
int m_Num2; //操作数2
};
//加法计算器类
class AddCalculator :public AbstracatCalculator {
public:
int getResult() {
return m_Num1 + m_Num2;
}
};
//减法计算器类
class SubCalculator :public AbstracatCalculator {
public:
int getResult() {
return m_Num1 - m_Num2;
}
};
//乘法计算器类
class MulCalculator :public AbstracatCalculator {
public:
int getResult() {
return m_Num1 * m_Num2;
}
};
//利用多态技术实现计算器
void test04() {
//创建加法计算器对象
AbstracatCalculator* abc = new AddCalculator();
abc->m_Num1 = 10;
abc->m_Num2 = 10;
cout << abc->m_Num1 << "+" << abc->m_Num2 << "=" << abc->getResult() << endl;
//用完记得销毁
delete abc;
//创建减法计算器对象
abc=new SubCalculator();
abc->m_Num1 = 100;
abc->m_Num2 = 100;
cout << abc->m_Num1 << "-" << abc->m_Num2 << "=" << abc->getResult() << endl;
delete abc;
//创建乘法计算器对象
abc = new MulCalculator();
abc->m_Num1 = 100;
abc->m_Num2 = 100;
cout << abc->m_Num1 << "*" << abc->m_Num2 << "=" << abc->getResult() << endl;
delete abc;
}
int main() {
test03();
test04();
system("pause");
return 0;
}
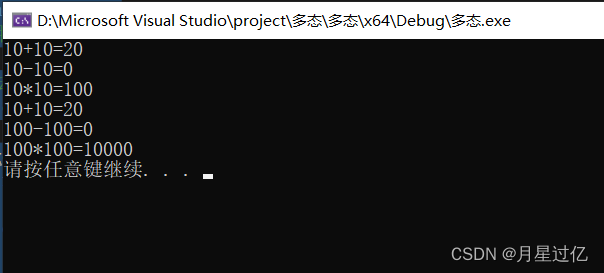
(三)纯虚函数和抽象类
在多态中,通常父类中虚函数的实现是毫无意义的,主要都是调用子类重写的内容
因此可以将虚函数改为纯虚函数
纯虚函数语法:virtual 返回值类型 函数名 (参数列表)=0;
当类中有了纯虚函数,这个类也称为抽象类
抽象类的特点:
- 无法实例化对象
- 子类必须重写抽象类中的纯虚函数,负责也属于抽象类
cpp
#include <iostream>
using namespace std;
//纯虚函数和抽象类
class Base {
public:
//纯虚函数
//只要有一个纯虚函数 这个类称为抽象类
//抽象类特点:
//1.无法实例化对象
//2.抽象类的子类必须重写父类中的纯虚函数
virtual void fun() = 0; //纯虚函数
};
class Son : public Base {
void fun() {//重写父类纯虚函数
cout << "Son::fun()" << endl;
}
};
void test06() {
//Base base; // 抽象类无法实例化对象
//Son son; // 子类为重写父类纯虚函数不可以实例化对象
Son son;
Base *base =new Son(); // 指针指向子类对象
base->fun();
}
int main() {
test06();
system("pause");
return 0;
}
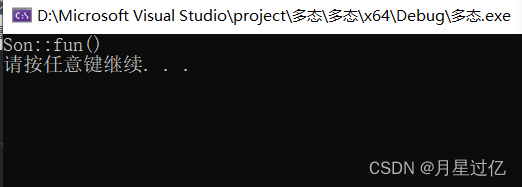
(四)多态案例二 -制作饮品
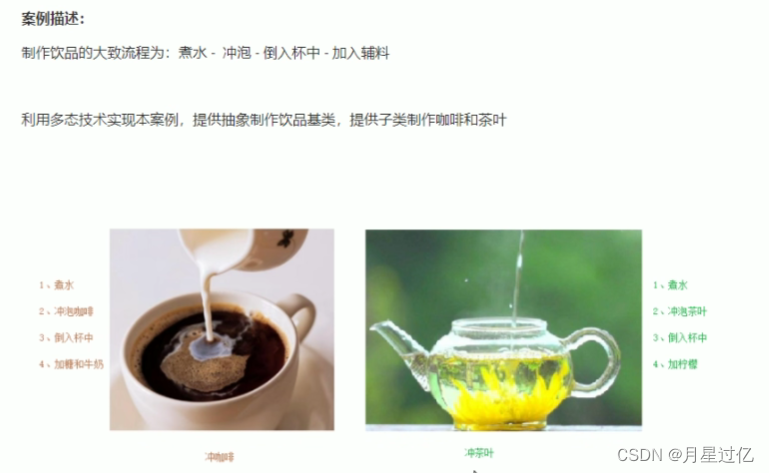
cpp
#include<iostream>
using namespace std;
//多态的案例2:制作饮品
class AbstractDrinking {
public:
//注水
virtual void boil() = 0;
//冲泡
virtual void brew() = 0;
//倒入杯子
virtual void pourInCup() = 0;
//加入辅料
virtual void PutSomething() = 0;
//制作饮品
void makeDrink() {
boil();
brew();
pourInCup();
PutSomething();
cout << "制作完成!" << endl;
}
};
//制作咖啡
class Coffee :public AbstractDrinking {
//煮水
virtual void boil() {
cout<<"煮水"<<endl;
}
//冲泡
virtual void brew() {
cout << "用沸水冲泡咖啡" << endl;
}
//倒入杯子
virtual void pourInCup() {
cout << "倒入杯子" << endl;
}
//加入辅料
virtual void PutSomething() {
cout << "加入糖和牛奶" << endl;
}
};
//制作茶
class Tea :public AbstractDrinking {
//煮水
virtual void boil() {
cout << "煮矿泉水" << endl;
}
//冲泡
virtual void brew() {
cout << "用沸水冲泡茶叶" << endl;
}
//倒入杯子
virtual void pourInCup() {
cout << "倒入杯子" << endl;
}
//加入辅料
virtual void PutSomething() {
cout << "加入茶辅料" << endl;
}
};
void doWork(AbstractDrinking* abs) {
abs->makeDrink();
delete abs; //释放
}
void test07() {
//制作咖啡
doWork(new Coffee());
//制作茶
doWork(new Tea());
}
int main() {
test07();
system("pause");
return 0;
}
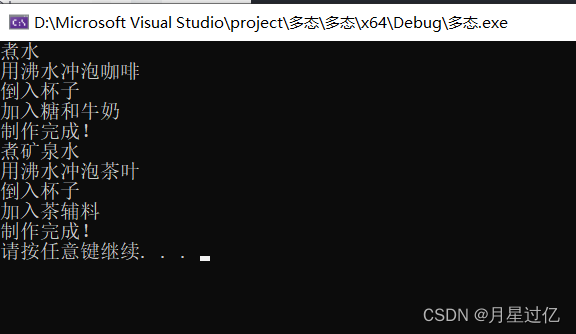
(五)虚析构和纯虚析构
多态使用时,如果子类中有属性开辟到堆区,那么父类指针在释放时无法调用到子类的析构代码
解决方式:将父类中的析构函数改为虚析构或者纯虚析构
虚析构和纯虚析构工性:
- 可以解决父类指针释放子类对象
- 都需要有具体的函数实现
虚析构和纯虚析构的区别:
- 如果是纯虚析构,该类属于抽象类,无法实例化对象
虚析构语法:
virtual ~类名(){实现}
纯虚析构语法:
virtual ~类名()=0;
类名::~类名(){ 实现}
总结:
- 虚析构或者纯虚析构就是用来解决父类指针释放子类对象
- 如果子类中没有堆区数据,可以不写为虚析构或者纯虚析构
- 拥有纯虚析构函数的类也属于抽象类
cpp
#include <iostream>
#include <string>
using namespace std;
//虚析构和纯虚析构
class Animal {
public:
Animal() {
cout << "Animal构造函数调用" << endl;
}
//纯虚函数
virtual void speak() = 0;
//virtual ~Animal() {
//cout << "Animal析构函数调用" << endl;
//}
//纯虚析构
virtual ~Animal() = 0;
};
Animal:: ~Animal(){
cout << "Animal纯虚析构函数调用" << endl;
}
class Cat :public Animal {
public:
Cat(string name) {
cout << "Cat构造函数调用" << endl;
m_Name= new string(name);
}
void speak() {
cout<<*m_Name << "喵喵喵" << endl;
}
~Cat(){
if (m_Name != NULL) {
cout << "Cat析构函数调用" << endl;
delete m_Name;
m_Name = NULL;
}
}
string* m_Name;
};
void test08() {
Animal* a = new Cat("TOM");
a->speak();
//父类指针在析构时候 不会调用子类中析构函数,导致子类如果有堆区属性,出现内存泄漏
delete a;
}
int main() {
test08();
system("pause");
return 0;
}
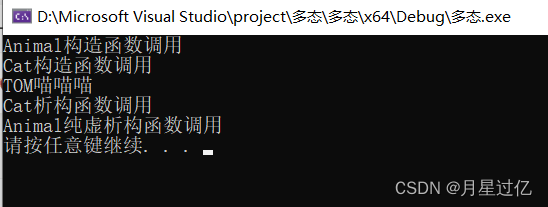
(六)多态案例三-电脑组装
cpp
#include <iostream>
using namespace std;
//抽象不同零件类
//抽象CPU类
class CPU {
public:
//抽象的计算函数
virtual void calculate() = 0;
};
//抽象显卡类
class VideoCard {
public:
//抽象的显示函数
virtual void display() = 0;
};
class Memory {
public:
//抽象的存储函数
virtual void memory() = 0;
};
//电脑类
class Computer {
public:
Computer(CPU* cpu, VideoCard* videoCard, Memory* memory) {
m_cpu = cpu;
m_videoCard = videoCard;
m_memory = memory;
}
//工作函数
void work() {
m_cpu->calculate();
m_videoCard->display();
m_memory->memory();
}
//提供析构函数 释放三个电脑零件
~Computer() {
//释放CPU零件
if (m_cpu != NULL) {
delete m_cpu;
m_cpu = NULL;
}
//释放显卡零件
if (m_videoCard != NULL) {
delete m_videoCard;
m_videoCard = NULL;
}
//释放内存条零件
if (m_memory != NULL) {
delete m_memory;
m_memory = NULL;
}
}
private:
CPU* m_cpu; //CPU的零件指针
VideoCard* m_videoCard;//显卡零件指针
Memory* m_memory;//内存条零件指针
};
//具体厂商
//Intel厂商
class IntelCPU :public CPU {
public:
void calculate() {
cout << "Intel CPU is calculating..." << endl;
}
};
class IntelVideoCard :public VideoCard {
public:
void display() {
cout << "Intel VideoCard is displaying..." << endl;
}
};
class IntelMemory :public Memory {
public:
void memory() {
cout << "Intel Memory is storing..." << endl;
}
};
//AMD厂商
class AMDCPU :public CPU {
public:
void calculate() {
cout << "AMD CPU is calculating..." << endl;
}
};
class AMDVideoCard :public VideoCard {
public:
void display() {
cout << "AMD VideoCard is displaying..." << endl;
}
};
class AMDMemory :public Memory {
public:
void memory() {
cout << "AMD Memory is storing..." << endl;
}
};
//联想厂商
class LenovoCPU :public CPU {
public:
void calculate() {
cout << "Lenovo CPU is calculating..." << endl;
}
};
class LenovoVideoCard :public VideoCard {
void display() {
cout << "Lenovo VideoCard is displaying..." << endl;
}
};
class LenovoMemory :public Memory {
public:
void memory() {
cout << "Lenovo Memory is storing..." << endl;
}
};
void test02() {
//第一台电脑零件
CPU * intelCpu = new IntelCPU();
VideoCard * intelVideoCard = new IntelVideoCard();
Memory * intelMemory = new IntelMemory();
//第一台电脑
Computer * computer1 = new Computer(intelCpu, intelVideoCard, intelMemory);
computer1->work();
delete computer1;
//第二台电脑零件
Computer computer2(new LenovoCPU, new AMDVideoCard, new IntelMemory);
computer2.work();
}
int main() {
test02();
system("pause");
return 0;
}
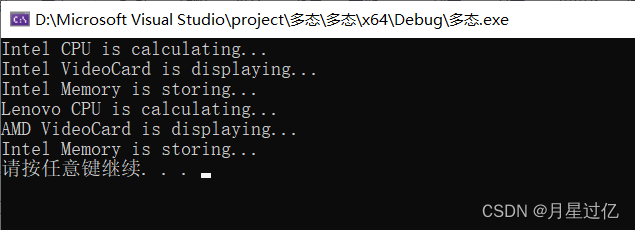