后台线程
一个进程中只有后台进程运行,该进程将会结束。
新创建的线程默认为前台线程,Java中只要有一个前台线程运行,就不会结束程序,如果只有后台线程运行,程序就会结束,可以在线程对象启动前执行setDaemon(true)语句设置该线程为后台线程。
java
class Main {
public static void main(String[] args) {
System.out.println("main()线程是后台线程吗"+Thread.currentThread().isDaemon());
DaemonThread daemon=new DaemonThread();
Thread thread=new Thread(daemon,"后台线程");
System.out.println("Daemons是后台线程吗"+thread.isDaemon());
thread.setDaemon(true);//设置为后台线程
System.out.println("Daemons是后台线程吗"+thread.isDaemon());
thread.start();
//模拟主线程main执行
int i=0;
for(;i<3;i++){
System.out.println(i);
}
}
}
class DaemonThread implements Runnable {
//模拟分线程执行
public void run(){
while(true){
System.out.println(Thread.currentThread().getName()+"正在运行");
}
}
}
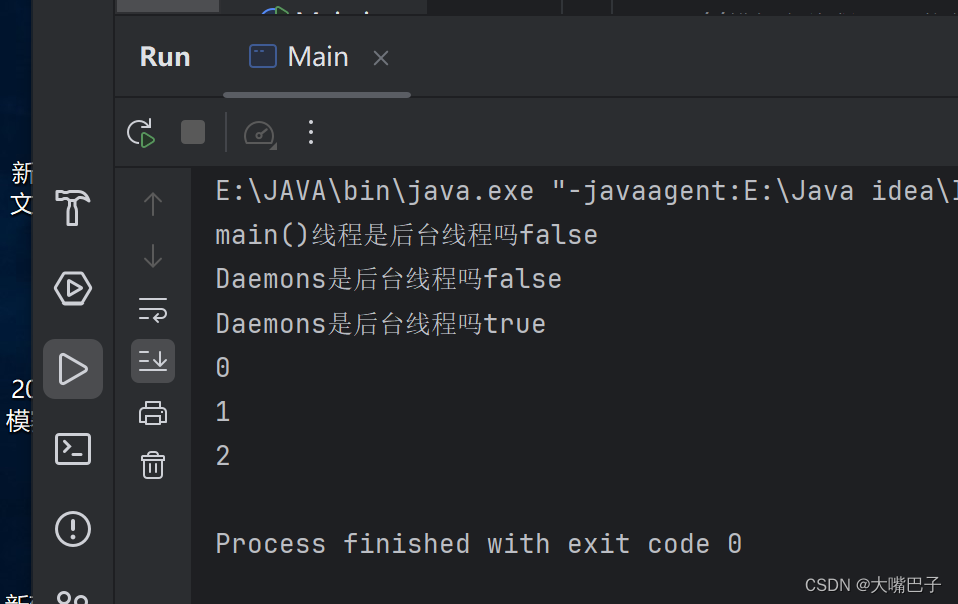
线程的优先级
使用setPriority方法,设置优先级为1到10;数字越大,优先级越高,获得CPU的使用权机会越大。
java
class Main {
public static void main(String[] args) {
Thread minPriority =new Thread(new maxpriority(),"优先级较高线程");
Thread maxPriority=new Thread(new minpriority(),"优先级较低线程");
minPriority.setPriority(Thread.MIN_PRIORITY);//设置优先级为1
maxPriority.setPriority(Thread.MAX_PRIORITY);//设置优先级为10
//开启两个线程
minPriority.start();
maxPriority.start();
}
}
class maxpriority implements Runnable{
public void run(){
for(int i=0;i<3;i++) {
System.out.println(Thread.currentThread().getName() + "正在输出" + i);
}
}
}
class minpriority implements Runnable{
public void run(){
for(int i=0;i<3;i++) {
System.out.println(Thread.currentThread().getName() + "正在输出" + i);
}
}
}
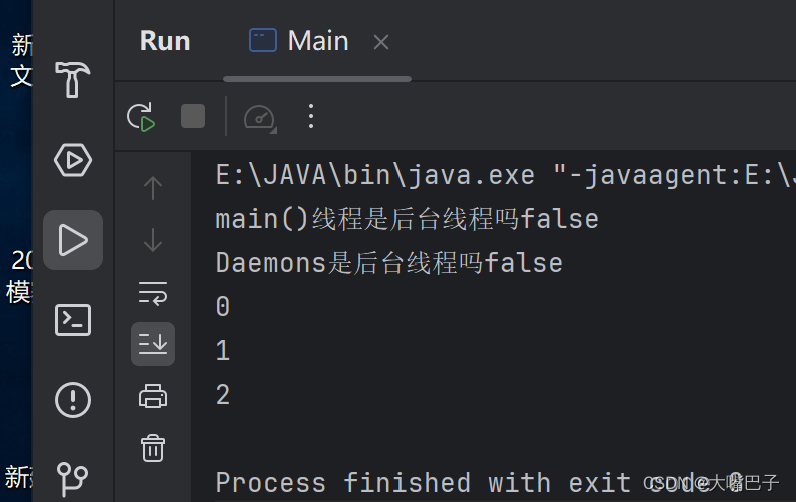
线程休眠
当前线程暂停运行,进入阻塞状态哦使用sleep()方法。传入参数单位为毫秒。
sleep调用时,只能让当前正在运行的线程休眠,
java
class Main {
public static void main(String[] args) {
new Thread(new Sleepthread()).start();//开启新线程
for(int i=1;i<=8;i++){
try{ if(i==5){
Thread.sleep(1000);
}
System.out.println("主线程正在输出"+i);
Thread.sleep(500);}
catch(InterruptedException e){
e.printStackTrace();
}
}
}
}
class Sleepthread implements Runnable{
public void run(){
for(int i=1;i<=8;i++){
if(i==3){
try{
Thread.sleep(1000);
}catch(Exception e){
e.printStackTrace();
}
}
System.out.println("Sleep线程正在输出"+i);
try{ Thread.sleep(500);}
catch(Exception e){
e.printStackTrace();
}
}
}
}
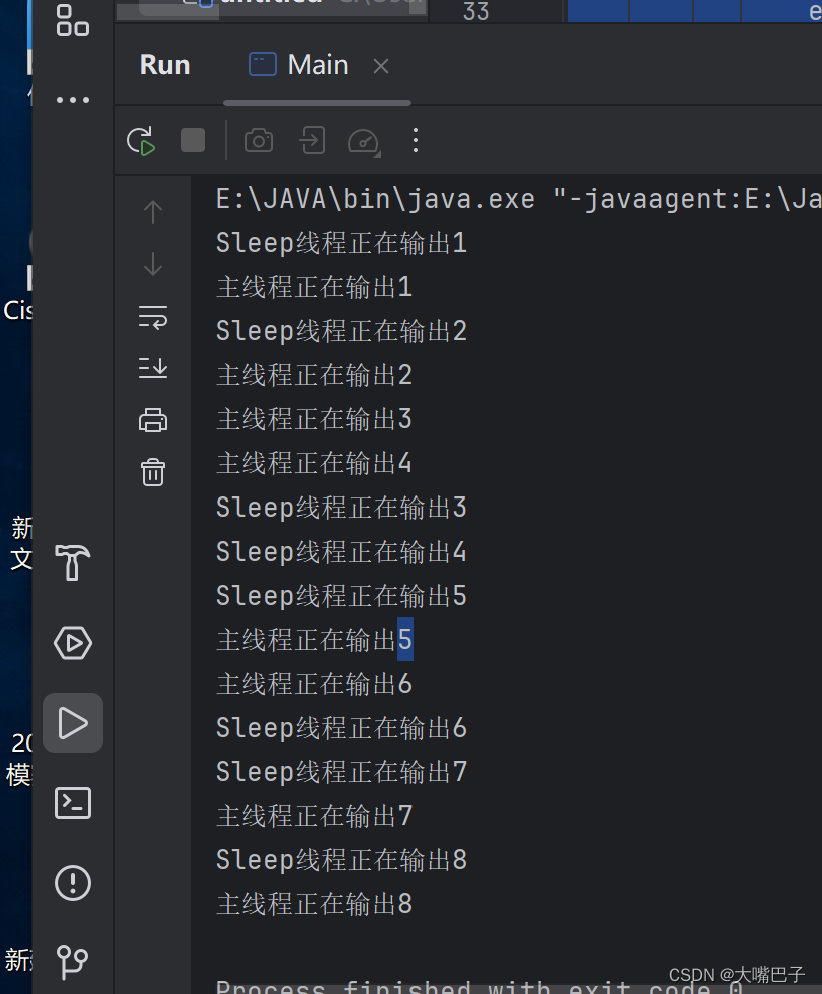
主线程在Sleep线程输出3之前连续输出2,3,4;说明此时Sleep线程在输出值3时先休眠了一段时间;
另外,Sleep线程再输出6之前,主线程也连续输出5,6;说明此时Sleep在输出6时休眠了一段时间。
线程插队
java
class Main {
public static void main(String[] args) throws Exception {
Thread thread=new Thread(new Joinrunable(),"thread");
thread.start();
for(int i=1;i<=4;i++){
if(i==2){
thread.join();//调用join()方法插队实现
}
System.out.println(Thread.currentThread().getName()+"线程正在输出"+i);
}
}
}
class Joinrunable implements Runnable{
public void run(){
for(int i=1;i<=3;i++){
System.out.println(Thread.currentThread().getName()+"线程正在输出"+i);
}
}
}
main()线程和thread线程互相争夺CPU使用权,然后当i=3时候
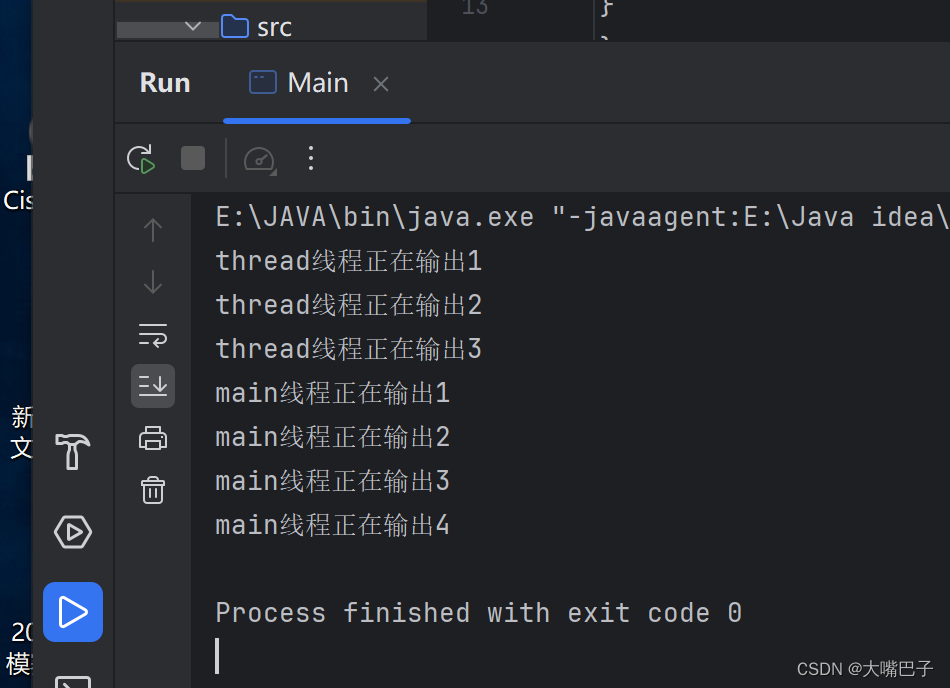
线程生命周期的六种基本状态
(1)新建状态
创建一个线程对象后,还没有调用start()方法启动之前的状态
(2)可运行状态
就绪状态,调用了start()方法之后进入的状态。
(3)锁阻塞状态
当一个线程想要获取一个对象锁,该aii锁被其他线程持有,该线程进入锁阻塞状态。
(4)无限等待状态
一个线程等待另一个线程执行一个唤醒动作,该线程进入的状态。
(5)计时等待状态
具有指定等待时间的状态,一直保持到超时或被唤醒
(6)被终止状态
终止运行,由于正常退出或者异常没有被捕获而结束。
线程让步
某个特定时间点,线程暂停抢夺CPU,采用yield()方法实现。
java
class Main {
public static void main(String[] args) throws Exception {
//新建两个线程
Thread th1=new Fieldthread("th1");
Thread th2=new Fieldthread("th2");
//开启两个线程
th1.start();
th2.start();
}
}
class Fieldthread extends Thread {
public Fieldthread(String name) {
super(name);//调用父类带参构造方法
}
public void run() {
for(int i=1;i<4;i++){
System.out.println(Thread.currentThread().getName()+"线程输出"+i);
if(i==2){
System.out.println("线程让步");
//线程让步
Thread.yield();
}
}
}
}
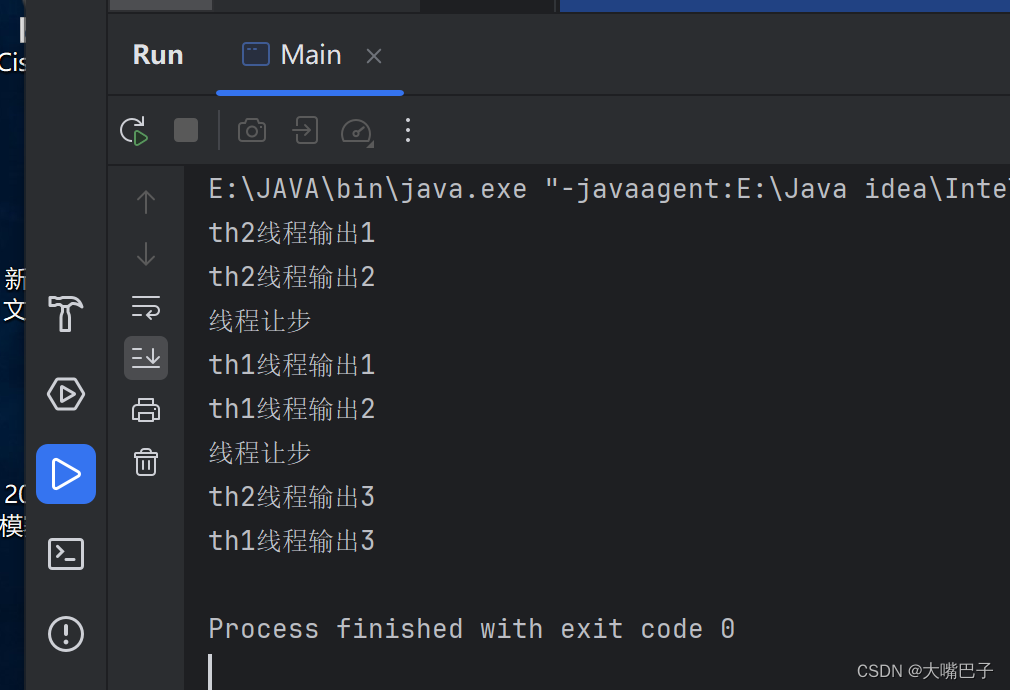
线程中断
调用两种方法实现:
interrupt()方法和isInterrupted()方法
isInterrupted方法判断中断标志位,如果为真表示中断。
java
class Main {
public static void main(String[] args) throws Exception {
Thread thread=new Thread(
new Runnable() {
public void run() {
for (int i = 0; i < 4; i++) {
if(i==2){
Thread.currentThread().interrupt();
System.out.println("线程是否中断"+Thread.currentThread().isInterrupted());
}
}
}
}
);//创建实例对象
thread.start();//启动线程
}
}
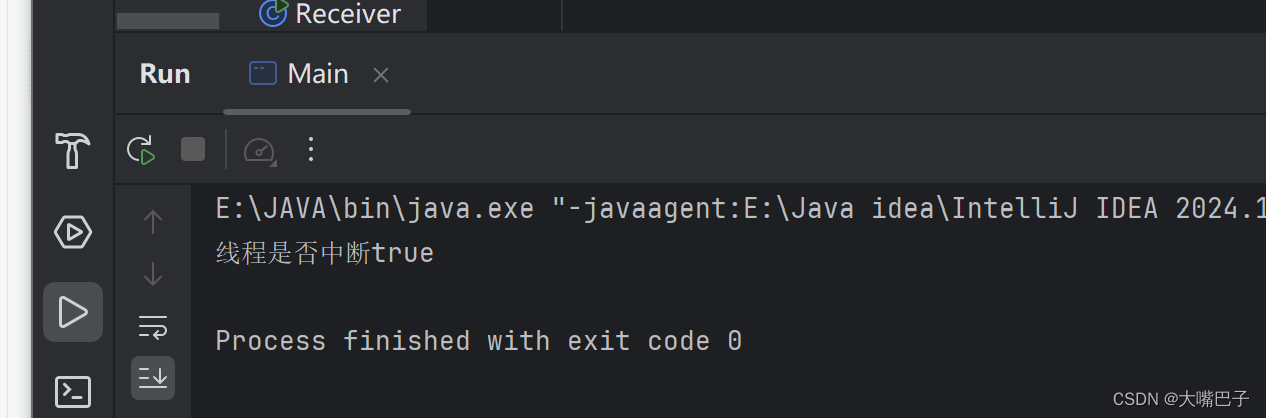
线程同步
java
class Main {
public static void main(String[] args) throws Exception {
Salethread salethread = new Salethread();
new Thread((salethread),"线程1").start();//创建并启动新线程
new Thread((salethread),"线程2").start();//创建并启动新线程
new Thread((salethread),"线程3").start();//创建并启动新线程
}
}
class Salethread implements Runnable{
private int tickets=10;
public void run(){
while(tickets>0){
try{
Thread.sleep(300);
}catch(Exception e){
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName()+"卖出票号是 "+tickets--);
}
}
}
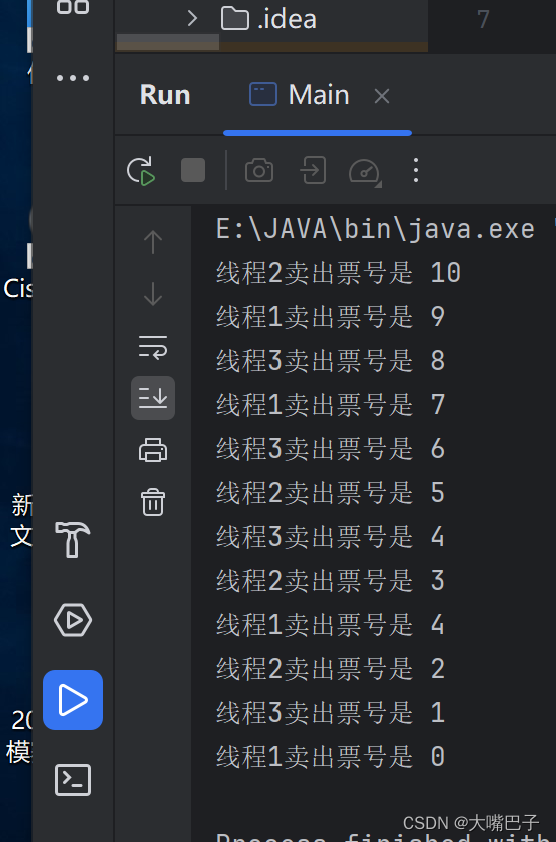
结果的票数中出现了0;之所以这样是因为每个线程都会先调用sleep方法进入休眠一段时间。即假设票数为3时候,线程2先进入while循环,然后调用sleepf方法休眠一段时间,在此期间票数值不变,因为票数只有在最后输出时候才会减少,线程3进入while循环,然后调用sleep()方法休眠一段时间,于此同时然后线程1进入while循环,然后调用sleep()方法进入休眠,最后三个线程依次结束休眠状态,相继售票,即票数由3变化到0;
所以为了安全起见,使用同步代码块,使得多线程访问处理同一资源时候,任何时刻只能由一个线程访问处理。
将共享资源的代码放在synchronized(lock)关键字修饰的代码块中。
synchronized(lock){
处理共享资源的代码块
}
lock是指锁,即某个线程执行时,其他线程不能执行。
java
class Main {
public static void main(String[] args) throws Exception {
Salethread salethread = new Salethread();
new Thread((salethread),"线程1").start();//创建并启动新线程
new Thread((salethread),"线程2").start();//创建并启动新线程
new Thread((salethread),"线程3").start();//创建并启动新线程
}
}
class Salethread implements Runnable{
private int tickets=10;
Object lock=new Object();//定义锁
public void run(){
while(tickets>0){
synchronized (lock) { try{
Thread.sleep(300);
}catch(Exception e){
e.printStackTrace();
}
if(tickets>0){ System.out.println(Thread.currentThread().getName()+"卖出票号是 "+tickets--);}
else{
break;
}}
}
}
}
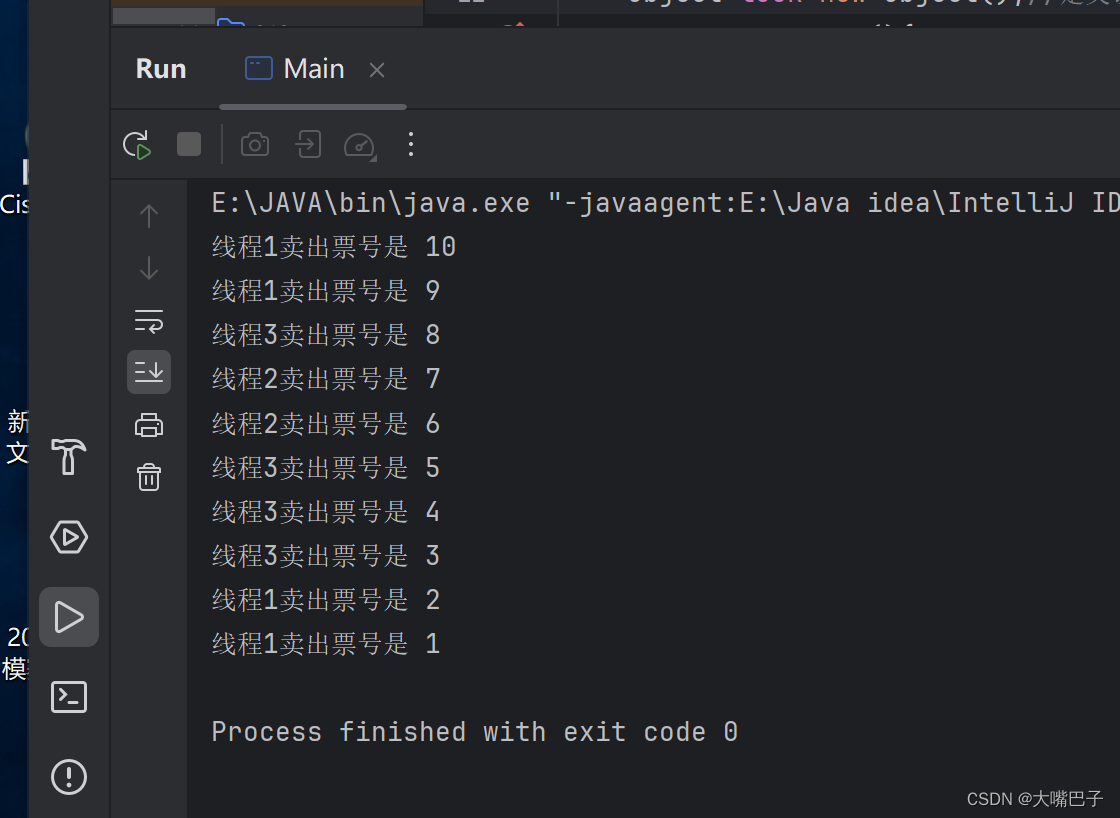
同步方法