目录
[1 界面设计](#1 界面设计)
[2 代码](#2 代码)
[2.1 登录界面](#2.1 登录界面)
[2.2 注册界面](#2.2 注册界面)
[2.3 登陆后的界面](#2.3 登陆后的界面)
[3 完整资源](#3 完整资源)
这里主要记录了如何使用Qt Creator创建一个用户登录界面,能够实现用户的注册和登录功能,注册的用户信息存储在了一个文件之中,在登录时可以比对登录信息和文件存储信息,已确认用户是否存在,如果不存在也可以通过注册功能进行注册。
1 界面设计
主要分为3个界面:登录界面、注册界面、登录后的界面
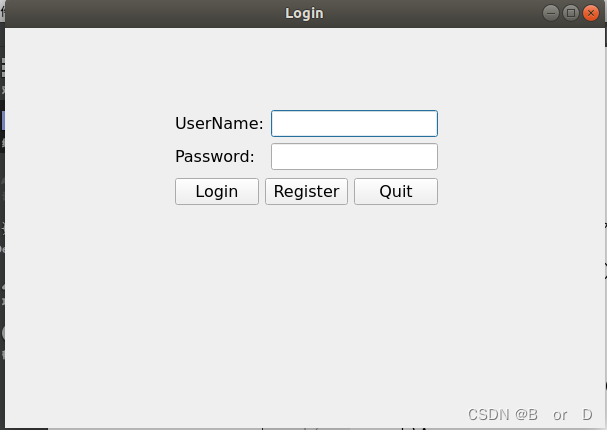
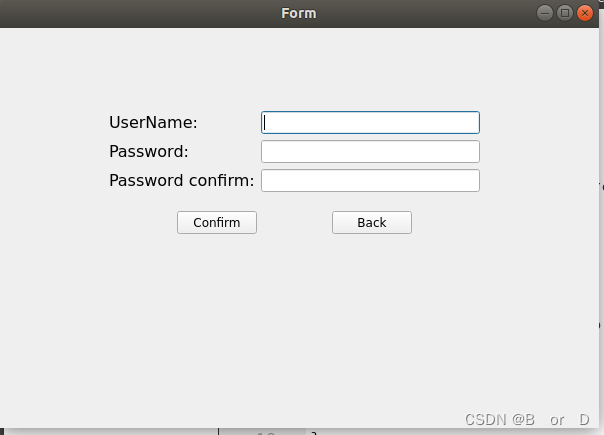
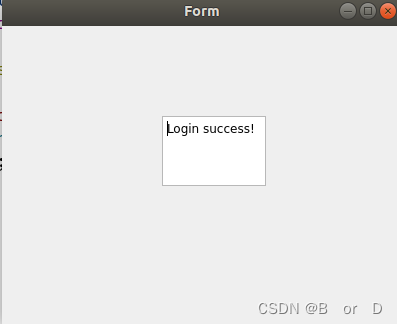
2 代码
2.1 登录界面
登录界面命名对于文件为widget.h、widget.c
widget.h
cpp
#ifndef WIDGET_H
#define WIDGET_H
#include <QWidget>
#include <form.h>
#include <form01.h>
QT_BEGIN_NAMESPACE
namespace Ui { class Widget; }
QT_END_NAMESPACE
class Widget : public QWidget
{
Q_OBJECT
public:
Form *form = new Form(); // define a object
Form01 *form01 = new Form01(); // Login
public:
Widget(QWidget *parent = nullptr);
~Widget();
private slots:
void on_pushButton_clicked();
void on_pushButton_2_clicked();
void on_pushButton_3_clicked();
private:
Ui::Widget *ui;
};
#endif // WIDGET_H
widget.c
cpp
#include "widget.h"
#include "ui_widget.h"
#include "QDebug"
#include "main.h"
#include "QDir"
#include "QMessageBox"
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
connect(this->form, &Form::BackSig, this, [=](){
this->form->hide();
this->show();
});
}
Widget::~Widget()
{
delete ui;
}
void Widget::on_pushButton_clicked()
{
// this->hide();
// this->close();
qDebug() << "Change page to Login";
// gain context about lineEdit and judge and ...
QString path = QDir::currentPath(); // 获取当前工程所在路径
std::string user_pwd; //
std::string part1; // useranme
std::string part2; // password
int flag = 1;
std::ifstream infile("/root/QT_developer/File/user_table.txt");
if (!infile.is_open()) {
std::cerr << "Unable to open file!" << std::endl;
}//
std::string line;
QString In_username = ui->lineEdit->text();
QString In_password = ui->lineEdit_2->text();
part1 = In_username.toStdString();
part2 = In_password.toStdString();
user_pwd = part1 + ":" + part2;
if(In_password.isEmpty())
{
QMessageBox::information(this, "Tips", "In_password is empty!");
}else if(In_username.isEmpty())
{
QMessageBox::information(this, "Tips", "In_usename is empty!");
}else
{
while (std::getline(infile, line)) { // gain data on a line
if(user_pwd == line)
{
flag = 0;
infile.close();
this->close();
ui->lineEdit->clear();
ui->lineEdit_2->clear();
QMessageBox::information(this, "Tips", "Login success!");
In_username.clear();
In_password.clear();
form01->show();
break;
}
}
if(flag == 1)
{
ui->lineEdit->clear();
ui->lineEdit_2->clear();
QMessageBox::information(this, "Tips", "username or password is error!");
In_username.clear();
In_password.clear();
}
}
}
void Widget::on_pushButton_2_clicked()
{
// this->hide();
// this->close();
qDebug() << "Change page to Register";
form->show();
}
void Widget::on_pushButton_3_clicked()
{
this->close();
qDebug() << "Quit";
}
2.2 注册界面
注册界面命名对于文件为form.h、form.c
form.h
cpp
#ifndef FORM_H
#define FORM_H
#include <QWidget>
namespace Ui {
class Form;
}
class Form : public QWidget
{
Q_OBJECT
public:
explicit Form(QWidget *parent = nullptr);
~Form();
public:
void Open_file();
private slots:
void on_pushButton_2_clicked();
void on_pushButton_clicked();
private:
Ui::Form *ui;
signals:
void BackSig(); // define a signal without arg.
};
#endif // FORM_H
form.c
cpp
#include "form.h"
#include "ui_form.h"
#include "qdebug.h"
#include "widget.h"
#include "QLineEdit"
#include "QMessageBox"
#include "main.h"
#include "QDir"
Form::Form(QWidget *parent) :
QWidget(parent),
ui(new Ui::Form)
{
ui->setupUi(this);
// this->show();
}
Form::~Form()
{
delete ui;
}
void Form::on_pushButton_2_clicked()
{
qDebug() << "Back";
emit this->BackSig();
}
void Form::on_pushButton_clicked()
{
///
QString path = QDir::currentPath(); // 获取当前工程所在路径
std::size_t found; // 查找冒号的位置
std::string user_pwd; // Concatenated username and password
std::string part1; // useranme
std::string part2; // password
std::string line;
int flag = 1;
std::ofstream outfile; // ready for writing
// std::ifstream infile(path.toStdString() + "user_table.txt");
std::ifstream infile("/root/QT_developer/File/user_table.txt"); // Absolute path
if (!infile.is_open()) { // Determine whether the opening is successful
std::cerr << "Unable to open file!" << std::endl;
}
///
// gain data
QString username = ui->lineEdit->text();
QString password = ui->lineEdit_2->text();
QString password_firm = ui->lineEdit_3->text();
// pan duan yong hu ming shi fou chong fu
if(username.isEmpty())
{
qDebug() << "username can't is empty";
QMessageBox::information(this, "Tips", "username can't is empty");
}else if(password.isEmpty()){
QMessageBox::information(this, "Tips", "password can't is empty");
}else if(password_firm.isEmpty())
{
QMessageBox::information(this, "Tips", "password_firm can't is empty");
}else{
// judge
if(password != password_firm)
{
ui->lineEdit->clear(); // clear
ui->lineEdit_2->clear();
ui->lineEdit_3->clear();
QMessageBox::information(this, "Tips", "password != password_firm!");
username.clear(); // clear
password.clear();
password_firm.clear();
}else{
while (std::getline(infile, line)) { // gain data on a line
found = line.find(':'); // find :
if (found != std::string::npos) {
part1 = line.substr(0, found); // 从开始到冒号前的部分
qDebug() << "part1-username: ";
cout << "part1-username: " << part1;
}
//
if(QString::fromStdString(part1) == username)
{
flag = 0;
infile.close();
ui->lineEdit->clear();
ui->lineEdit_2->clear();
ui->lineEdit_3->clear();
QMessageBox::information(this, "Tips", "username has been exist!");
username.clear();
password.clear();
password_firm.clear();
break;
}
}
if(flag == 1){
QMessageBox::information(this, "Tips", "Register success!");
part1 = username.toStdString();
part2 = password.toStdString();
user_pwd = part1 + ":" + part2;
outfile.open("/root/QT_developer/File/user_table.txt", ios::in | std::ios::out | std::ios::app);
outfile << user_pwd << endl;
outfile.close();
ui->lineEdit->clear();
ui->lineEdit_2->clear();
ui->lineEdit_3->clear();
username.clear();
password.clear();
password_firm.clear();
}
}
}
}
2.3 登陆后的界面
登录后的界面命名对于文件为form01.h、form01.c
form01.h
cpp
#ifndef FORM01_H
#define FORM01_H
#include <QWidget>
namespace Ui {
class Form01;
}
class Form01 : public QWidget
{
Q_OBJECT
public:
explicit Form01(QWidget *parent = nullptr);
~Form01();
private:
Ui::Form01 *ui;
};
#endif // FORM01_H
form01.c
cpp
#include "form01.h"
#include "ui_form01.h"
Form01::Form01(QWidget *parent) :
QWidget(parent),
ui(new Ui::Form01)
{
ui->setupUi(this);
}
Form01::~Form01()
{
delete ui;
}
3 完整资源
按照以上代码就能实现,如果有需要这是完整代码。也可以私我。