目录
一、链表的概念及结构
概念:链表是一种 物理存储结构上非连续 、非顺序的存储结构,数据元素的 逻辑顺序 是通过链表
中的 指针链接 次序实现的 。
二、链表的分类
实际中链表的结构非常多样,以下情况组合起来就有 8 种链表结构:
-
单向或者双向
-
带头或者不带头
-
循环或者非循环
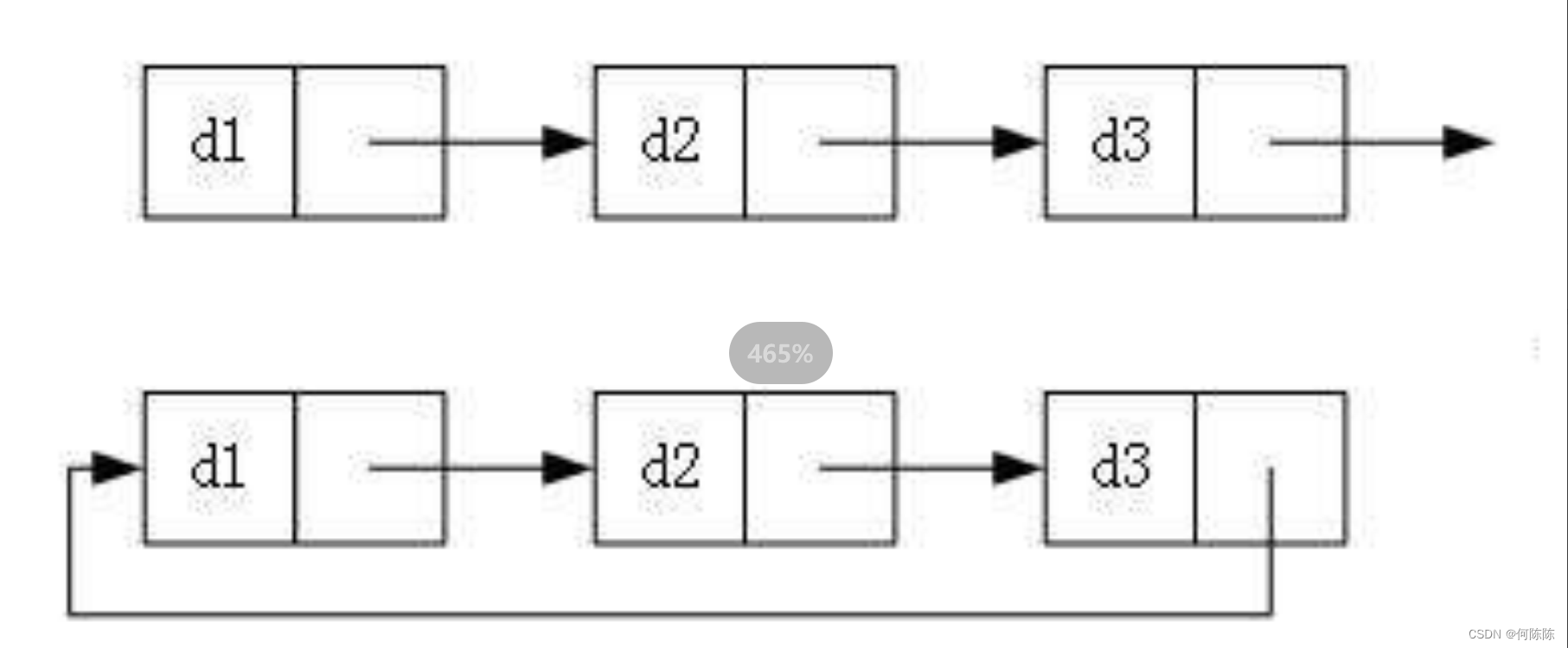
虽然有这么多的链表的结构,但是我们实际中最常用还是两种结构:
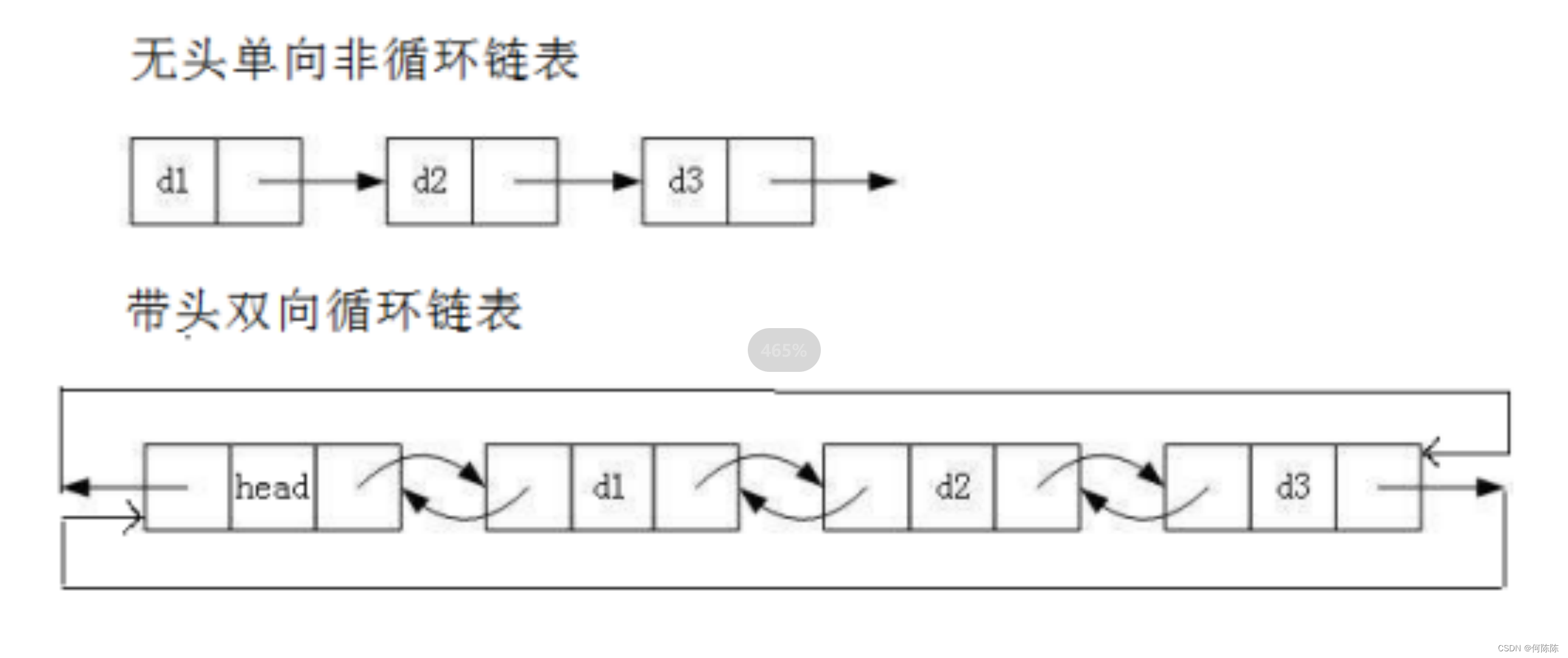
- 无头单向非循环链表: 结构简单 ,一般不会单独用来存数据。实际中更多是作为 其他数据结 构的子结构 ,如哈希桶、图的邻接表等等。另外这种结构在 笔试面试 中可能出现比较多。
- 带头双向循环链表: 结构最复杂 ,一般用在单独存储数据。实际中使用的链表数据结构,都是带头双向循环链表。另外这个结构虽然结构复杂,但是使用代码实现以后会发现结构会带来很多优势,实现反而简单了。
三、单链表的实现
建立链表的节点:
链表中的节点结构体大概有这些内容:节点数据,下一个节点的地址
cpp
typedef int SLTDateType;
typedef struct SListNode
{
SLTDateType data;
struct SListNode* next;
}SListNode;
尾插------尾删:
不管是尾插还头插还是任意位置插入,我们都需要先创建一个新节点。
尾插和尾删都需要注意一些点:
用二级指针接收:
因为我们这个是无头的链表,在链表没有一个节点的时候,我们需要创建一个节点。然后将链表的头指向这个新节点,这个时候就涉及到需要修改一级结构体的一级指针。一级指针类型的变量需要修改的话,就要用到二级指针。
尾插:是否链表一个数据都没有,这个时候我们要特殊处理一下,检查链表有效性
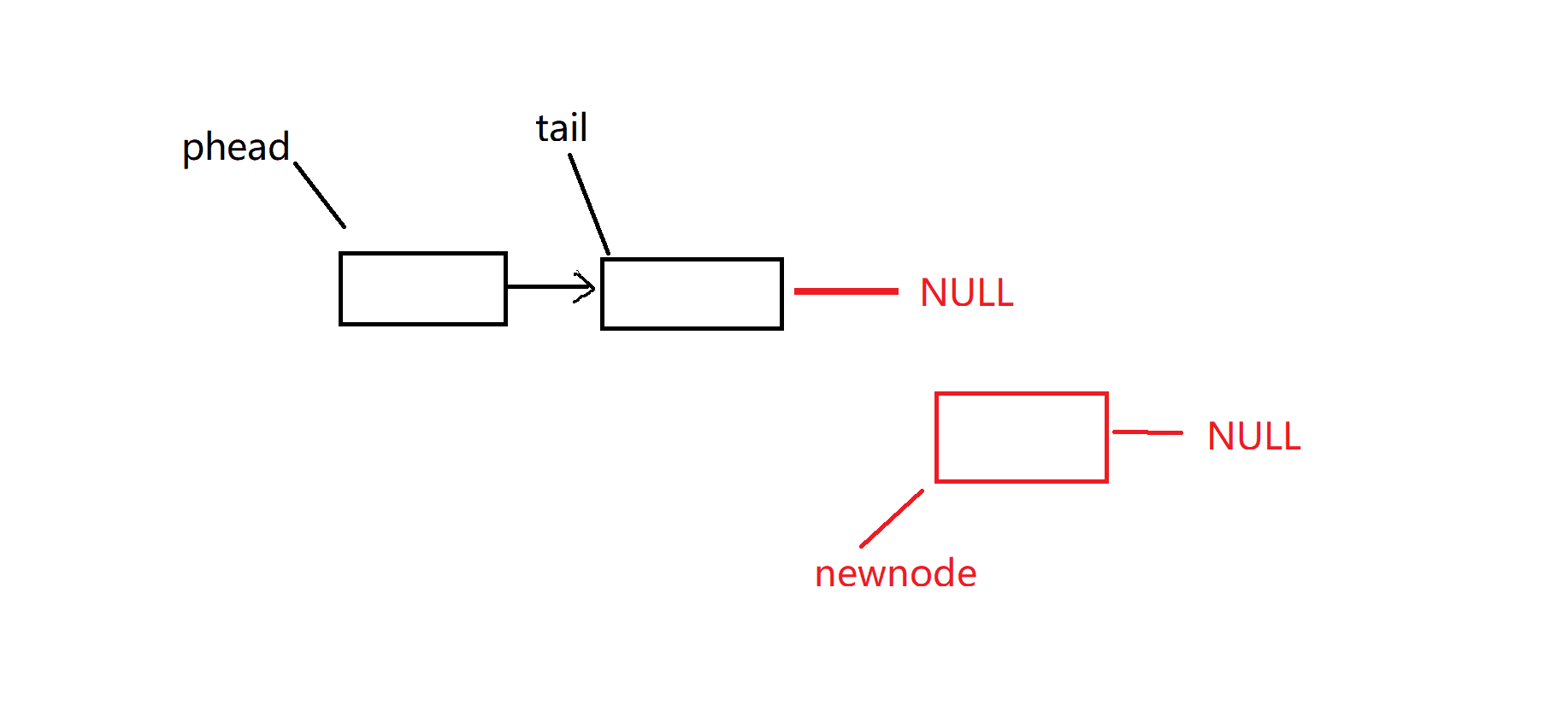
尾删:是否链表只有一个数据 ,检查是否有数据可以删除,检查链表有效性
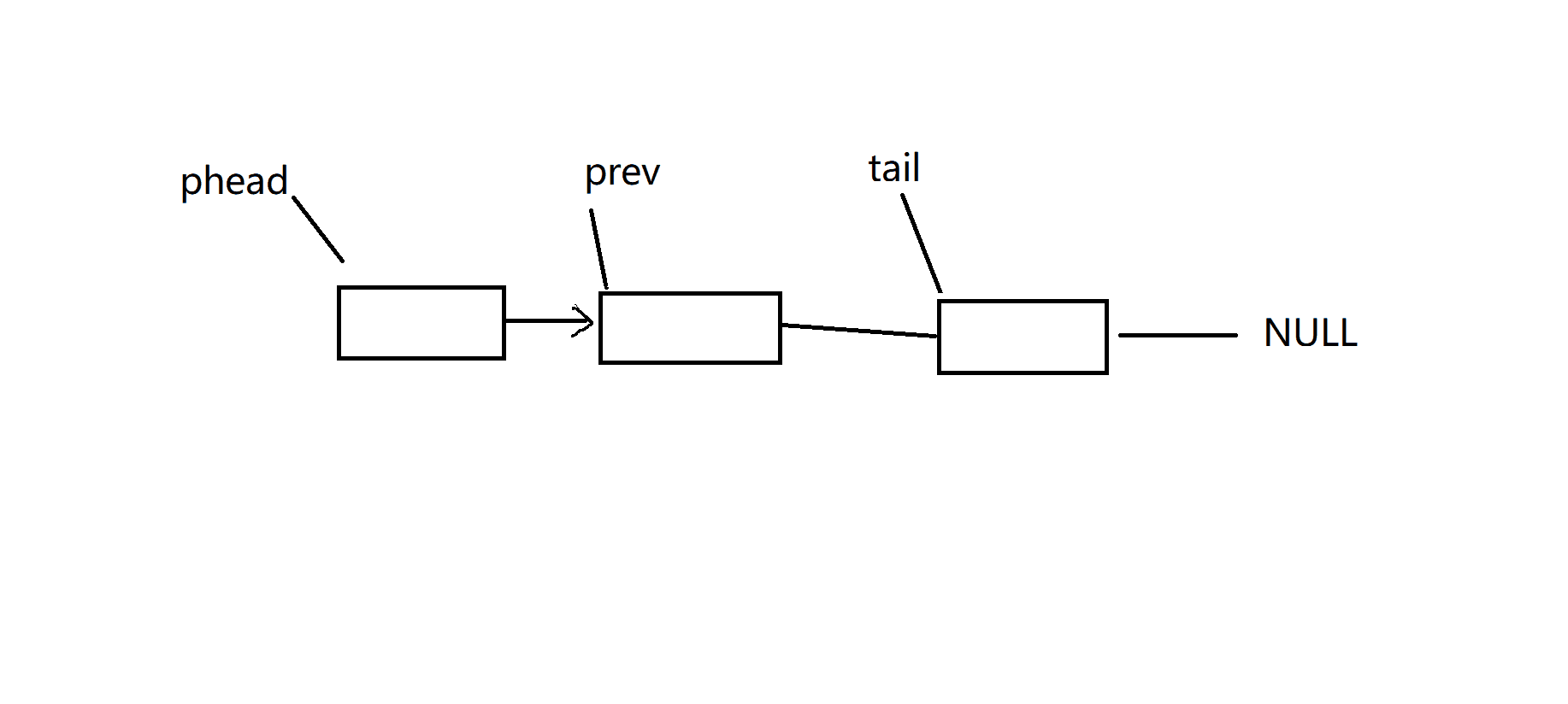
cpp
// 动态申请一个节点
SListNode* BuySListNode(SLTDateType x)
{
SListNode* newnode = (SListNode*)malloc(sizeof(SListNode));
if (newnode == NULL)
{
perror("malloc fail");
}
newnode->data = x;
newnode->next = NULL;
return newnode;
}
// 单链表尾插
void SListPushBack(SListNode** pplist, SLTDateType x)
{
assert(pplist);
SListNode* newnode = BuySListNode(x);
if (*pplist == NULL)
{
*pplist = newnode;
return;
}
//找尾巴
SListNode* tail = *pplist;
while (tail->next != NULL)
tail = tail->next;
tail->next = newnode;
}
// 单链表的尾删
void SListPopBack(SListNode** pplist)
{
assert(pplist);
assert(*pplist);//有数据才能删,没有数据不删,至少有一个及其以上节点
if ((*pplist)->next == NULL)
{
free(*pplist);
*pplist = NULL;
}
else
{
//找尾巴
SListNode* prev = NULL;
SListNode* tail = *pplist;
while (tail->next != NULL)
{
prev = tail;
tail = tail->next;
}
free(tail);
prev->next = NULL;
}
}
头插------头删:
头插和头删在链表中还是比较简单,一个是把头节点保存起来,另一个是头节点的下一个节点保存起来,然后进行删除就行了。
头插:注意需要检查链表有效性
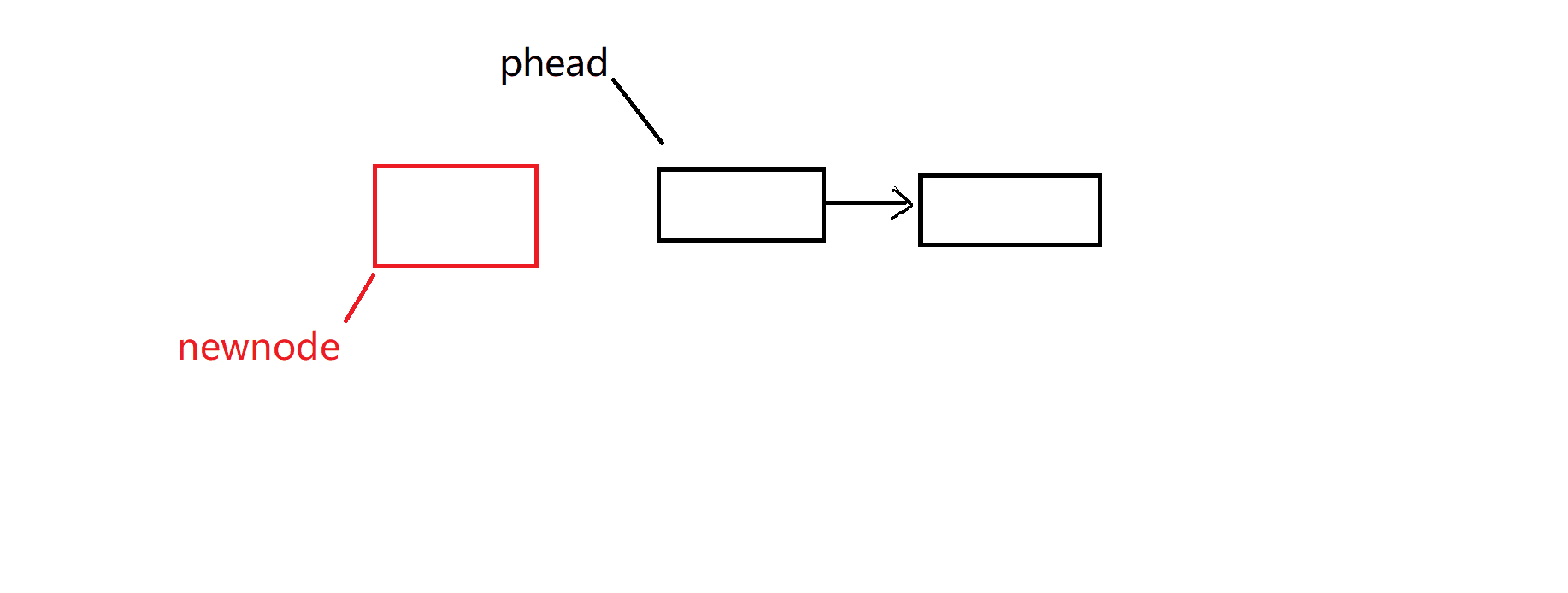
头删:注意这里需要检查数据个数,有数据才能删除;
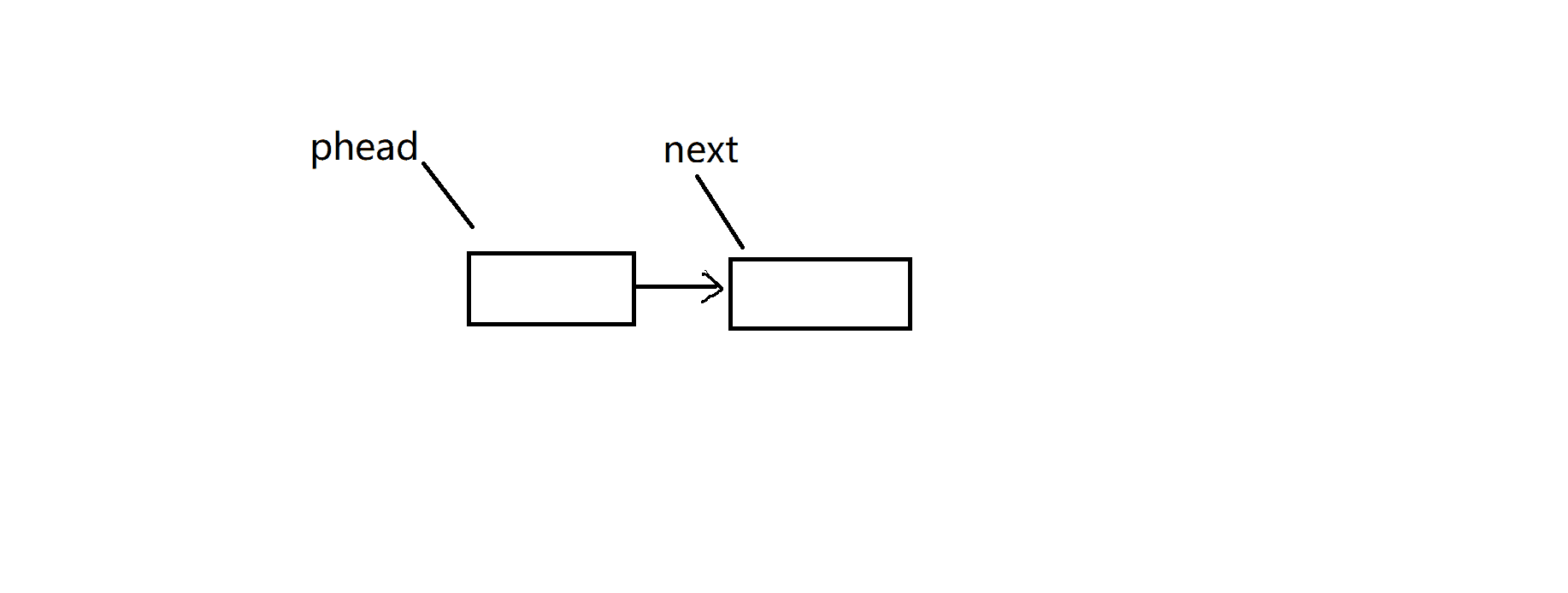
cpp
// 单链表的头插
void SListPushFront(SListNode** pplist, SLTDateType x)
{
assert(pplist);
SListNode* newnode = BuySListNode(x);
newnode->next = *pplist;
*pplist = newnode;
}
// 单链表头删
void SListPopFront(SListNode** pplist)
{
assert(pplist);
assert(*pplist);
SListNode* next = (*pplist)->next;
free(*pplist);
*pplist = next;
}
查找:
通过简单遍历就行了,注意返回的是节点的指针;
我们在外面定义的是一个链表节点的指针,打印的时候按照传值的方式传递变量,他会把变量的内容(链表中首节点的地址)拷贝过来,最终返回的也是链表节点的地址
cpp
// 单链表查找
SListNode* SListFind(SListNode* plist, SLTDateType x)
{
assert(plist);
SListNode* cur = plist;
while (cur)
{
if (cur->data == x)
return cur;
cur = cur->next;
}
return NULL;
}
指定位置之后删除------插入:
**注意:**写这两个接口,我们首先就要想到的是检查 指定位置的有效性 还有检查 链表有效性。
代码还是很简单的,这里的删除要检查是不是最后一个节点;
cpp
// 单链表在pos位置之后插入x
void SListInsertAfter(SListNode* pos, SLTDateType x)
{
assert(pos);
SListNode* newnode = BuySListNode(x);
newnode->next = pos->next;
pos->next = newnode;
}
// 单链表删除pos位置之后的值void SListEraseAfter(SListNode* pos)
{
assert(pos);
assert(pos->next);//检查是不是最后一个
SListNode* next = pos->next;
pos->next = next->next;
free(next);
}
指定位置之前插入------删除指定位置:
这两个接口:我们需要像尾插那个样子 遍历找到指定位置前的位置:然后进行插入,删除,同时也要注意检查链表有效性,
**注意:**如果链表只有一个位置或者指定位置为第一个节点,那删除就变成了头删,可以复用原来的头删接口。尾删则没有必要,我们已经遍历一遍找到尾了,不需要调用尾删接口再遍历一遍了,直接像尾删一样删除就行了。
cpp
// 在pos的前面插入
void SLTInsert(SListNode** pphead, SListNode* pos, SLTDateType x)
{
assert(pphead);
assert((!pos&&!(*pphead))||(pos&&(*pphead)))
//这里我们让他都为空(头插)或者都不为空
//我们想暴露出一个问题,不允许乱位置插入 限定pos一定是有效节点
if (pos == *pphead)
{
SListPushFront(pphead, x);
return;
}
SListNode* prev = *pphead;
while (prev->next!=pos)
{
prev = prev->next;
}
SListNode* newnode = BuySListNode(x);
newnode->next = pos;
prev->next = newnode;
}
// 删除pos位置
void SLTErase(SListNode** pphead, SListNode* pos)
{
assert(pphead);
assert(*pphead);
assert(pos);//这里进行检查pos,必须有这个节点才能删除
if (pos == *pphead)
{
SListPopFront(pphead);
return;
}
SListNode* prev = *pphead;
while (prev->next != pos)
{
prev = prev->next;
}
prev->next = pos->next;
free(pos);
pos = NULL;
}
销毁链表:
这个按照遍历的同时free就行了。
注意:遍历完了,全部空间释放了把链表置空
cpp
void SLTDestroy(SListNode** pphead)
{
assert(pphead);
assert(*pphead);
SListNode* cur = *pphead;
while (cur)
{
SListNode* next = cur->next;
free(cur);
cur = next;
}
*pphead = NULL;
}
打印:
为了好测试写的接口是否正确,我们还是写一个打印接口,方便我们观察:同样的,循环遍历打印就行了;这里不需要检查链表是否为空,空链表也可以打印
cpp
// 单链表打印
void SListPrint(SListNode* plist)
{
SListNode* cur = plist;
while (cur)
{
printf("%d-> ", cur->data);
cur = cur->next;
}
printf("\n");
}
四、链表面试题
- 删除链表中等于给定值 val的所有结点。 203. 移除链表元素 - 力扣(LeetCode)
这个题我们找到与 给定值相等的节点和他的前一个节点就可以进行删除了,但是我们要处理两种情况:
1:链表为空
2:需要删除头(需要循环删除,因为有可能连续好几个都需要删)
参考代码:
cpp
struct ListNode* removeElements(struct ListNode* head, int val) {
//处理链表为空
if(!head) return NULL;
//处理链表第一个就是该删的元素
if(head->val==val)
{
while(head&&head->val==val)
{
struct ListNode* next=head->next;
free(head);
head=next;
}
}
struct ListNode* cur=head;
struct ListNode* prev=NULL;
while(cur)
{
if(cur->val==val)
{
prev->next=cur->next;
free(cur);
cur=prev->next;
}
else
{
prev=cur;
cur=cur->next;
}
}
return head;
}
- 反转一个单链表。 206. 反转链表 - 力扣(LeetCode)
这个题,就是一个简单头插就行了
当然我们也可以用三个指针将链表的指向反转**(注意检查链表是否为空)**,这里我们用两种方法实现,两种方法任选其一都能通过,
cpp
struct ListNode* reverseList(struct ListNode* head) {
//头插处理
// struct ListNode* newhead=NULL;
// while(head)
// {
// struct ListNode* next=head->next;
// head->next=newhead;
// newhead=head;
// head=next;
// }
// return newhead;
//直接反转
if(!head)
return head;
struct ListNode* n1=NULL,*n2=head,*n3=head->next;
while(n2)
{
n2->next=n1;
n1=n2;
n2=n3;
if(n3)
n3=n3->next;
}
return n1;
}
- 给定一个带有头结点 head 的非空单链表,返回链表的中间结点。如果有两个中间结点,则返回第二个中间结点。 876. 链表的中间结点 - 力扣(LeetCode)
这个题是一个标准的 快慢指针
值得注意的是 :我们判断条件是快指针为NULL或者快指针的下一个节点为NULL,他们在循环中判断的先后为先判断自己再判断下一个,因为先判断下一个的话,有可能出现野指针问题。
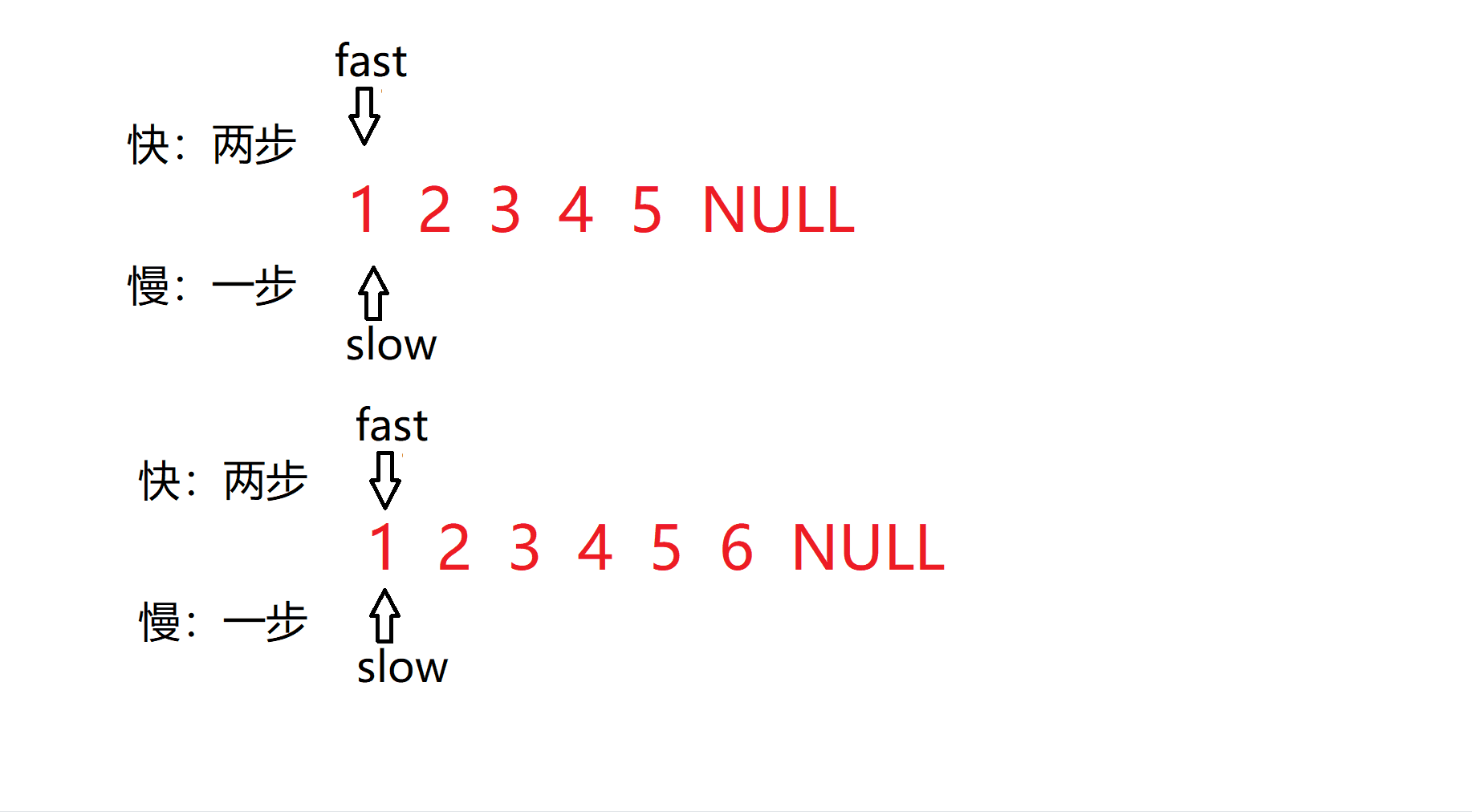
cpp
struct ListNode* middleNode(struct ListNode* head) {
//快慢指针法
struct ListNode* fast=head;
struct ListNode* slow=head;
while(fast&&fast->next)
{
fast=fast->next->next;
slow=slow->next;
}
return slow;
}
- 输入一个链表,输出该链表中倒数第k个结点。 面试题 02.02. 返回倒数第 k 个节点 - 力扣(LeetCode)
这个题也是一个经典的双指针,可以让快指针先走k次,然后快慢指针同时走,每次走一下:
cpp
int kthToLast(struct ListNode* head, int k){
struct ListNode* fast=head;
struct ListNode* slow=head;
while(k--)
fast=fast->next;
while(fast)
{
fast=fast->next;
slow=slow->next;
}
return slow->val;
}
- 将两个有序链表合并为一个新的有序链表并返回。新链表是通过拼接给定的两个链表的所有结点组成的。 21. 合并两个有序链表 - 力扣(LeetCode)
这个题就要用到并归和尾插了,选择小的尾插到新链表里面,这个尾插是移动他的链表节点到我们新的链表里面。
需要注意的是:1.需要处理空链表情况,一个为空或者两个为空
2.处理头节点
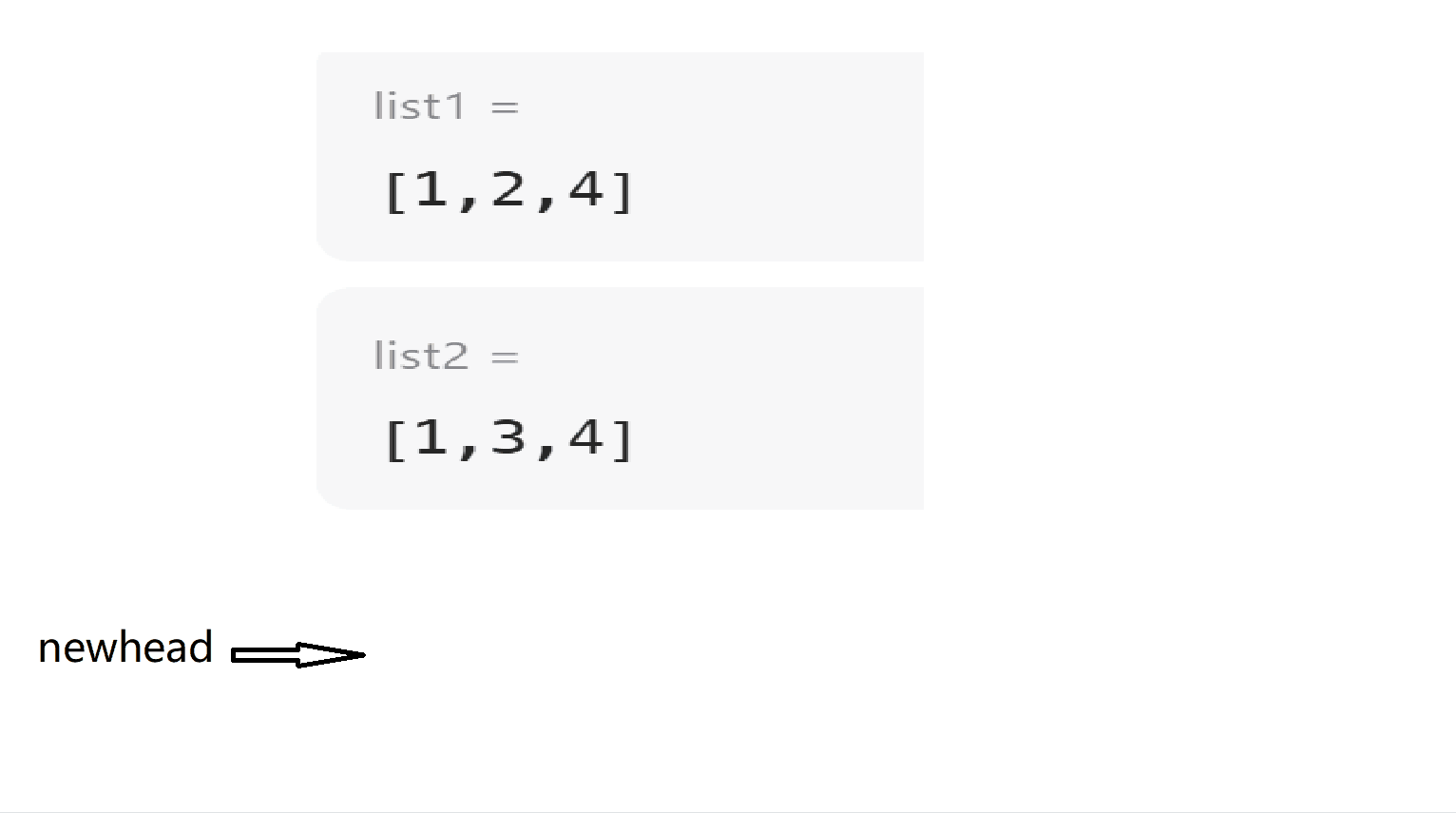
cpp
struct ListNode* mergeTwoLists(struct ListNode* list1, struct ListNode* list2) {
//处理为空的情况
if(!list1)
return list2;
if(!list2)
return list1;
struct ListNode* head=NULL;
struct ListNode* tail=NULL;
while(list1&&list2)
{
if(list1->val<list2->val)
{
struct ListNode* next=list1->next;
if(head==NULL)//处理头节点
head=tail=list1;
else
{
tail->next=list1;
tail=tail->next;
tail->next=NULL;//这个可以不处理,后面剩余节点的尾巴也一定是NULL
}
list1=next;
}
else
{
struct ListNode*next=list2->next;
if(head==NULL)//处理头节点
head=tail=list2;
else
{
tail->next=list2;
tail=tail->next;
tail->next=NULL;
}
list2=next;
}
}
if(list1==NULL)//处理剩余未插入的节点
tail->next=list2;
else
tail->next=list1;
return head;
}
- 编写代码,以给定值 x 为基准将链表分割成两部分,所有小于 x 的结点排在大于或等于 x 的结
点之前 。 链表分割_牛客题霸_牛客网 (nowcoder.com)
这个题呢,我们用把链表分成两条,小于给定值的在一条,大于给定值的在一条,然后在连接起来就行了。
注意:这里我们要用到 带头的单链表,无头的单链表会有很多问题要处理。我们还需要将链表移动后 置空,不然后面连接起来可能会出现很多问题
cpp
class Partition {
public:
ListNode* partition(ListNode* pHead, int x) {
ListNode* head1, *tail1, *head2, *tail2;
head1 = tail1 = (ListNode*)malloc(sizeof(ListNode));//第一条链表
head2 = tail2 = (ListNode*)malloc(sizeof(ListNode));//第二条链表
tail1->next = tail2->next = NULL;
while (pHead)
{
if (pHead->val < x)
{
ListNode* next = pHead->next;
tail1->next = pHead;
tail1 = tail1->next;
tail1->next = NULL;
pHead = next;
}
else
{
ListNode* next = pHead->next;
tail2->next = pHead;
tail2 = tail2->next;
tail2->next = NULL;
pHead = next;
}
}
tail1->next=head2->next;
ListNode* re=head1->next;
free(head2);
free(head1);
return re;
}
};
- 链表的回文结构。OJ链接
这个题,我们需要找到中间节点(先遍历找出节点个数即可找到),然后翻转一半节点到创建的另一个链表当中,然后进行比对即可得到是否为回文结构;
cpp
class PalindromeList {
public:
bool chkPalindrome(ListNode* A) {
if(!A) return true;
int count=0;
ListNode* cur=A;
while(cur)
{
cur=cur->next;
count++;
}
count=(count+1)/2;
int i=count;
ListNode*head=NULL;
while(i--)
{
ListNode*next=A->next;
A->next=head;
head=A;
A=next;
}
while(head&&A)
{
if(head->val!=A->val)
return false;
head=head->next;
A=A->next;
}
return true;
}
};
- 输入两个链表,找出它们的第一个公共结点。 160. 相交链表 - 力扣(LeetCode)
这个题我们可以首先想到暴力解法:先在定一条链表中的某个节点,然后再另一个链表中找该节点,如果找到了有返回该节点,不过这样的解法时间复杂度太高了
第二种方法是我们先遍历各自链表,找出其中个数,先让长的那一条链表先走他们的节点个数差,然后两条链表同时遍历,找出相交节点;
cpp
struct ListNode *getIntersectionNode(struct ListNode *headA, struct ListNode *headB) {
int cnt1=0,cnt2=0;
struct ListNode* cur1=headA;
while(cur1)
{
cur1=cur1->next;
cnt1++;
}
struct ListNode* cur2=headB;
while(cur2)
{
cur2=cur2->next;
cnt2++;
}
if(cur2!=cur1)//如果链表到最后都不相等,说明不相交,没有交点
return NULL;
//假定A比B长
struct ListNode* ha=headA;
struct ListNode* hb=headB;
if(cnt1<cnt2)
{
ha=headB;
hb=headA;
}
int cnt=abs(cnt1-cnt2);
while(cnt--)//让长的先走个数差
ha=ha->next;
while(ha)//两条链表同时遍历
{
if(ha==hb)//注意两个节点相等,不是节点值相等 !!!!!!!
return ha;
ha=ha->next;
hb=hb->next;
}
return NULL;
}
9.给定一个链表,判断链表中是否有环。141. 环形链表 - 力扣(LeetCode)
这是一个经典快慢指针:
cpp
bool hasCycle(struct ListNode *head) {
struct ListNode* fast=head;
struct ListNode* slow=head;
while(fast&&fast->next)
{
fast=fast->next->next;
slow=slow->next;
if(fast==slow)
return true;
}
return false;
}
10.给定一个链表,返回链表开始入环的第一个结点。 如果链表无环,则返回 NULL
142. 环形链表 II - 力扣(LeetCode)
这个题需要我么用到简单的数学知识:
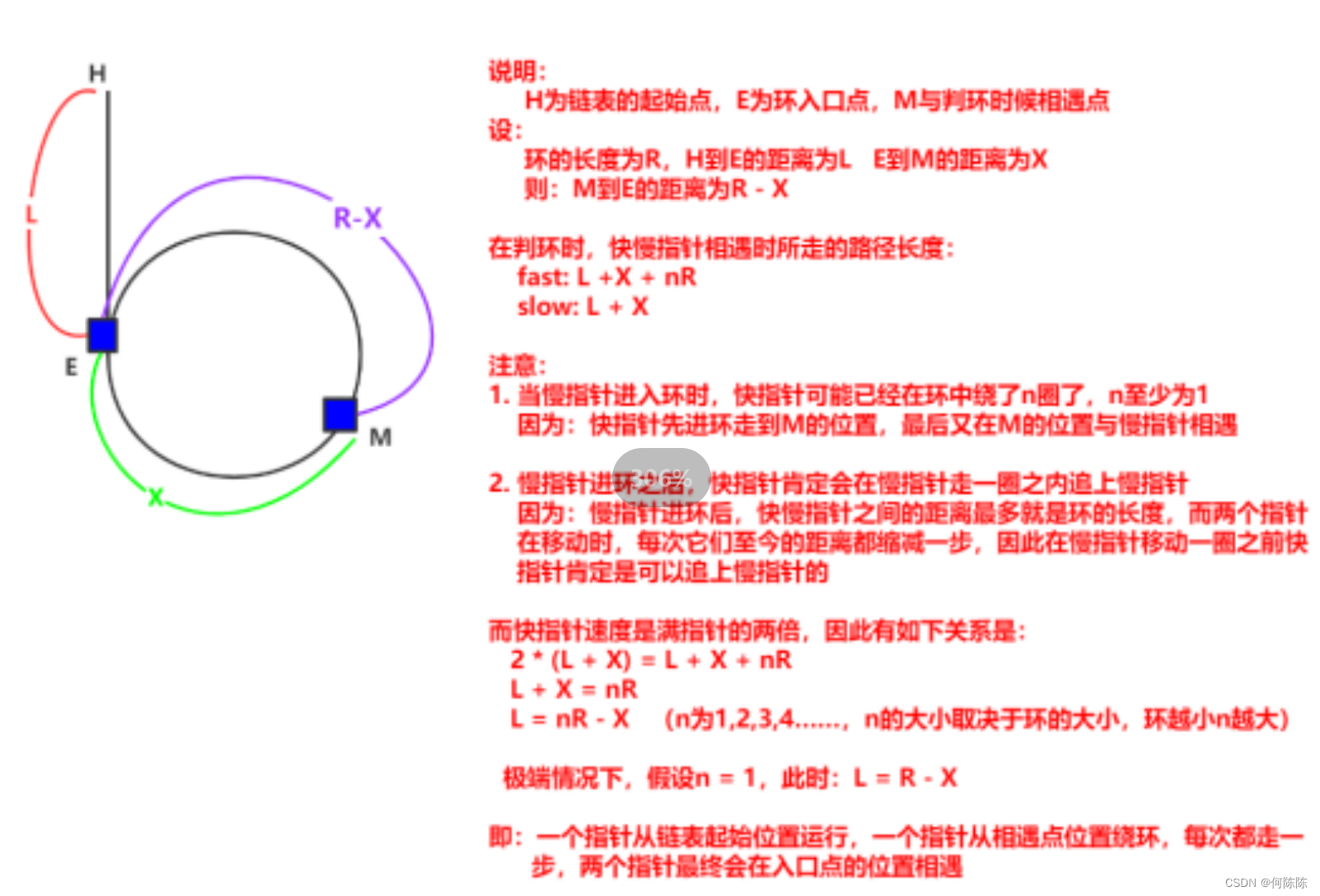
cpp
struct ListNode *detectCycle(struct ListNode *head) {
struct ListNode* fast=head;
struct ListNode* slow=head;
while(fast&&fast->next)
{
fast=fast->next->next;
slow=slow->next;
if(fast==slow)
{
struct ListNode* cur=head;
while(cur!=fast)
{
cur=cur->next;
fast=fast->next;
}
return cur;
}
}
return NULL;
}
- 给定一个链表,每个结点包含一个额外增加的随机指针,该指针可以指向链表中的任何结点或空结点。 要求返回这个链表的深度拷贝。 138. 随机链表的复制 - 力扣(LeetCode)
这个题我们可以用这个方法: 在原链表中,每个节点都复制一个节点插入在自己的后面,
然后进行random处理,如果原节点的random存在,则新节点的random指向原节点的random的下一个。最后,将两条链表分离即可。
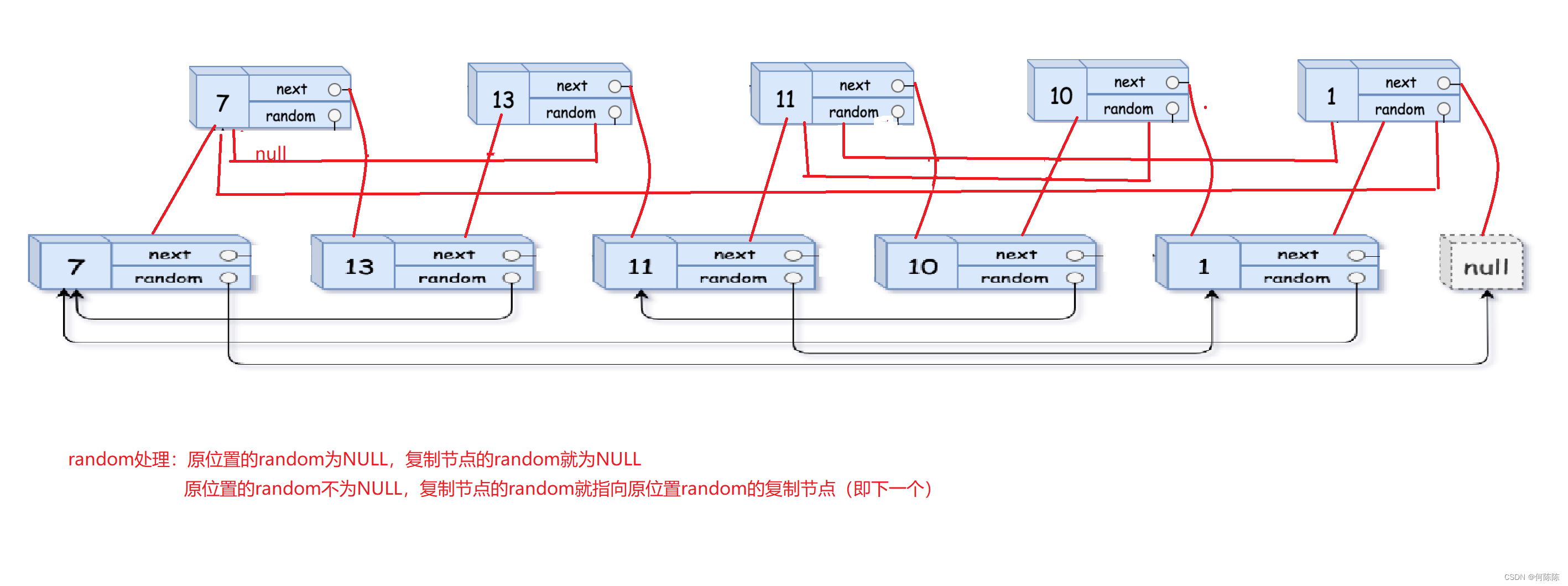
cpp
struct Node* copyRandomList(struct Node* head) {
if(!head) return NULL;
struct Node* cur=head;
while(cur)
{
struct Node* newnode=(struct Node*)malloc(sizeof(struct Node));
newnode->next=cur->next;
newnode->val=cur->val;
newnode->random=NULL;
cur->next=newnode;
cur=newnode->next;
}
cur=head;
while(cur)
{
struct Node* new=cur->next;
if(cur->random)
new->random=cur->random->next;
cur=new->next;
}
cur=head;
struct Node* newhead=cur->next;
struct Node* tail=cur->next;
while(cur)
{
cur->next=tail->next;
cur=cur->next;
if(cur)
tail->next=cur->next;
tail=tail->next;
}
return newhead;
}
五、总体代码
SList.h
cpp
#pragma once
#include<stdio.h>
#include<assert.h>
#include<stdlib.h>
// slist.h
typedef int SLTDateType;
typedef struct SListNode
{
SLTDateType data;
struct SListNode* next;
}SListNode;
// 动态申请一个节点
SListNode* BuySListNode(SLTDateType x);
// 单链表打印
void SListPrint(SListNode* plist);
// 单链表尾插
void SListPushBack(SListNode** pplist, SLTDateType x);
// 单链表的头插
void SListPushFront(SListNode** pplist, SLTDateType x);
// 单链表的尾删
void SListPopBack(SListNode** pplist);
// 单链表头删
void SListPopFront(SListNode** pplist);
// 单链表查找
SListNode* SListFind(SListNode* plist, SLTDateType x);
// 单链表在pos位置之后插入x
// 分析思考为什么不在pos位置之前插入?
void SListInsertAfter(SListNode* pos, SLTDateType x);
// 单链表删除pos位置之后的值
// 分析思考为什么不删除pos位置?
void SListEraseAfter(SListNode* pos);
// 在pos的前面插入
void SLTInsert(SListNode** pphead, SListNode* pos, SLTDateType x);
// 删除pos位置
void SLTErase(SListNode** pphead, SListNode* pos);
void SLTDestroy(SListNode** pphead);
SList.h
cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include"SList.h"
// 动态申请一个节点
SListNode* BuySListNode(SLTDateType x)
{
SListNode* newnode = (SListNode*)malloc(sizeof(SListNode));
if (newnode == NULL)
{
perror("malloc fail");
}
newnode->data = x;
newnode->next = NULL;
return newnode;
}
// 单链表打印
void SListPrint(SListNode* plist)
{
assert(plist);
SListNode* cur = plist;
while (cur)
{
printf("%d-> ", cur->data);
cur = cur->next;
}
printf("\n");
}
// 单链表尾插
void SListPushBack(SListNode** pplist, SLTDateType x)
{
assert(pplist);
SListNode* newnode = BuySListNode(x);
if (*pplist == NULL)
{
*pplist = newnode;
return;
}
//找尾巴
SListNode* tail = *pplist;
while (tail->next != NULL)
tail = tail->next;
tail->next = newnode;
}
// 单链表的头插
void SListPushFront(SListNode** pplist, SLTDateType x)
{
assert(pplist);
SListNode* newnode = BuySListNode(x);
newnode->next = *pplist;
*pplist = newnode;
}
// 单链表的尾删
void SListPopBack(SListNode** pplist)
{
assert(pplist);
assert(*pplist);//有数据才能删,没有数据不删
if ((*pplist)->next == NULL)
{
free(*pplist);
*pplist = NULL;
}
else
{
//找尾巴
SListNode* prev = NULL;
SListNode* tail = *pplist;
while (tail->next != NULL)
{
prev = tail;
tail = tail->next;
}
free(tail);
prev->next = NULL;
}
}
// 单链表头删
void SListPopFront(SListNode** pplist)
{
assert(pplist);
assert(*pplist);
SListNode* next = (*pplist)->next;
free(*pplist);
*pplist = next;
}
// 单链表查找
//plist是一个变量,保存的是地址,传的时候也是地址,传过去就是将变量里面的地址拷贝一份传过去了
SListNode* SListFind(SListNode* plist, SLTDateType x)
{
assert(plist);
SListNode* cur = plist;
while (cur)
{
if (cur->data == x)
return cur;
cur = cur->next;
}
return NULL;
}
// 单链表在pos位置之后插入x
// 分析思考为什么不在pos位置之前插入?
void SListInsertAfter(SListNode* pos, SLTDateType x)
{
assert(pos);
SListNode* newnode = BuySListNode(x);
newnode->next = pos->next;
pos->next = newnode;
}
// 单链表删除pos位置之后的值
// 分析思考为什么不删除pos位置?
void SListEraseAfter(SListNode* pos)
{
assert(pos);
assert(pos->next);//检查是不是最后一个
SListNode* next = pos->next;
pos->next = next->next;
free(next);
}
// 在pos的前面插入
void SLTInsert(SListNode** pphead, SListNode* pos, SLTDateType x)
{
assert(pphead);
assert(*pphead);
//这里没有检查pos是否为NULL,我认为在最后一个节点之后插入也是可以的
if (pos == *pphead)
{
SListPushFront(pphead, x);
return;
}
SListNode* prev = *pphead;
while (prev->next!=pos)
{
prev = prev->next;
}
SListNode* newnode = BuySListNode(x);
newnode->next = pos;
prev->next = newnode;
}
// 删除pos位置
void SLTErase(SListNode** pphead, SListNode* pos)
{
assert(pphead);
assert(*pphead);
assert(pos);//这里进行检查pos,必须有这个节点才能删除
if (pos == *pphead)
{
SListPopFront(pphead);
return;
}
SListNode* prev = *pphead;
while (prev->next != pos)
{
prev = prev->next;
}
prev->next = pos->next;
free(pos);
pos = NULL;
}
void SLTDestroy(SListNode** pphead)
{
assert(pphead);
assert(*pphead);
SListNode* cur = *pphead;
while (cur)
{
SListNode* next = cur->next;
free(cur);
cur = next;
}
*pphead = NULL;
}
Test.c
cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include"SList.h"
void test1()
{
SListNode* SL = NULL;
SListPushBack(&SL, 1);
SListPushBack(&SL, 2);
SListPushBack(&SL, 3);
SListPushBack(&SL, 4);
SListPushBack(&SL, 5);
SListPrint(SL);
SListPushFront(&SL, 6);
SListPushFront(&SL, 7);
SListPushFront(&SL, 8);
SListPushFront(&SL, 9);
SListPushFront(&SL, 10);
SListPushFront(&SL, 99);
SListPushFront(&SL, 88);
SListPushFront(&SL, 77);
SListPrint(SL);
SListPopBack(&SL);
SListPopBack(&SL);
SListPopBack(&SL);
SListPopBack(&SL);
SListPrint(SL);
SListPopFront(&SL);
SListPopFront(&SL);
SListPopFront(&SL);
SListPrint(SL);
SListInsertAfter(SListFind(SL, 1), 99);
SListInsertAfter(SListFind(SL, 10), 99);
SListPrint(SL);
SListEraseAfter(SListFind(SL, 1));
SListEraseAfter(SListFind(SL, 10));
//printf("%d\n", SListFind(SL, 1)->data);
SListPrint(SL);
SLTInsert(&SL, SListFind(SL, 10), 99);
SLTInsert(&SL, NULL, 99);
SListPrint(SL);
SLTErase(&SL, SListFind(SL, 99));
SLTErase(&SL, SListFind(SL, 99));
SListPrint(SL);
SLTDestroy(&SL);
}
int main()
{
test1();
return 0;
}