源码:
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Textarea Character Counter with Draggable Resizing</title>
<style>
.container {
position: relative;
width: 100%;
max-width: 280px; /* Adjust as needed */
}
#inputText {
width: 100%;
min-width: 280px; /* Minimum width to prevent shrinking */
height: 100px; /* Adjust initial height as needed */
min-height: 100px; /* Minimum height to prevent shrinking */
box-sizing: border-box;
padding: 10px;
font-size: 14px; /* Adjust font size as needed */
overflow: auto; /* Handle text overflow */
}
.counter {
position: absolute;
bottom: 10px;
right: 10px;
color: black;
font-size: 12px; /* Adjust font size as needed */
}
.counter.max {
color: red;
}
</style>
</head>
<body>
<div class="container">
<textarea id="inputText" rows="6" maxlength="150"></textarea>
<div id="charCount" class="counter">0/150</div>
</div>
<script>
const textArea = document.getElementById('inputText');
const charCount = document.getElementById('charCount');
const minWidth = 280; // Minimum width for the textarea
textArea.addEventListener('input', function() {
const textLength = textArea.value.length;
charCount.textContent = `${textLength}/150`;
if (textLength >= 150) {
charCount.classList.add('max');
} else {
charCount.classList.remove('max');
}
});
// Function to handle mousemove event for resizing
function handleResize(event) {
const mouseX = event.clientX;
const textareaRect = textArea.getBoundingClientRect();
const containerRect = textArea.parentNode.getBoundingClientRect();
let newWidth = mouseX - textareaRect.left;
// Ensure new width respects minimum width
if (newWidth < minWidth) {
newWidth = minWidth;
}
// Set new width to textarea
textArea.style.width = newWidth + 'px';
// Adjust counter position based on textarea width change
charCount.style.right = `${containerRect.right - textareaRect.right + 10}px`;
}
// Listen for mousedown event on textarea for starting resize
textArea.addEventListener('mousedown', function(event) {
if (event.offsetX > textArea.offsetWidth - 10) {
// Only start resize if mouse is near the right edge
document.addEventListener('mousemove', handleResize);
}
});
// Stop resizing on mouseup event
document.addEventListener('mouseup', function() {
document.removeEventListener('mousemove', handleResize);
});
// Initial check on load
window.addEventListener('load', function() {
const currentWidth = textArea.clientWidth;
if (currentWidth < minWidth) {
textArea.style.width = minWidth + 'px';
}
});
</script>
</body>
</html>
运行效果:
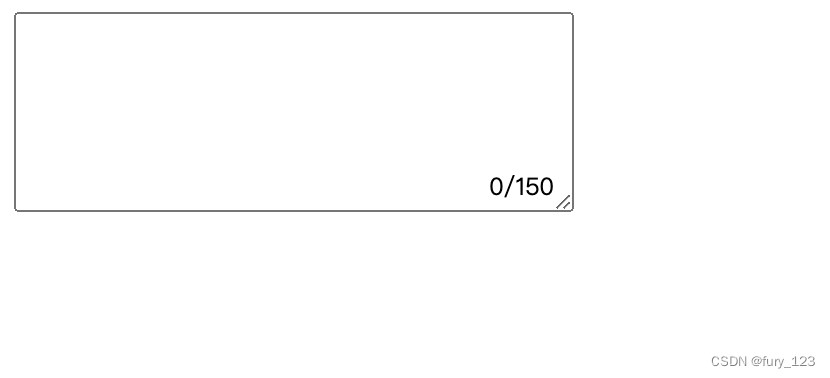