文章目录
前言
C++日期类的完整实现,以及this指针的const修饰等的介绍
一、日期类的实现
cpp
// Date.h
#pragma once
#include <iostream>
using namespace std;
#include <assert.h>
class Date
{
// 友元函数
friend ostream& operator<<(ostream& out, const Date& d);
friend istream& operator>>(istream& in, Date& d);
public:
// 构造函数
Date(int year = 1970, int month = 1, int day = 1);
void Print() const
{
cout << _year << '-' << _month << '-' << _day << endl;
}
// 拷贝构造函数
Date(const Date& d);
// 析构函数
~Date();
// 赋值运算符重载
Date& operator=(const Date& d);
// 运算符重载
bool operator<(const Date& d) const;
bool operator==(const Date& d) const;
bool operator<=(const Date& d) const;
bool operator>(const Date& d) const;
bool operator>=(const Date& d) const;
bool operator!=(const Date& d) const;
// 获取每月天数
int GetMonthDay(int year, int month);
// + += - -= 天数
Date& operator+=(const int day);
Date operator+(const int day) const;
Date& operator-=(const int day);
Date operator-(const int day) const;
// 前置++
Date& operator++();
// 后置++
Date operator++(int);
// 前置--
Date& operator--();
// 后置--
Date operator--(int);
// 日期-日期
int operator-(const Date& d) const;
private:
int _year;
int _month;
int _day;
};
// 流插入
ostream& operator<<(ostream& out, const Date& d);
// 流提取
istream& operator>>(istream& in, Date& d);
cpp
// Date.cpp
#define _CRT_SECURE_NO_WARNINGS
#include "Date.h"
// 构造函数
Date::Date(int year, int month, int day)
{
if (month > 0 && month < 13 && day > 0 && day <= GetMonthDay(year, month))
{
_year = year;
_month = month;
_day = day;
}
else
{
cout << "非法日期" << endl;
assert(false);
}
}
// 拷贝构造函数
Date::Date(const Date& d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
// 析构函数
Date::~Date()
{
_year = 0;
_month = 0;
_day = 0;
}
// 赋值运算符重载
Date& Date::operator=(const Date& d)
{
if (this != &d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
return *this;
}
// 运算符重载
bool Date::operator<(const Date& d) const
{
if (_year < d._year)
{
return true;
}
else if (_year == d._year && _month < d._month)
{
return true;
}
else if (_year == d._year && _month == d._month && _day == d._day)
{
return true;
}
else
{
return false;
}
}
bool Date::operator==(const Date& d) const
{
if (_year != d._year)
{
return false;
}
else if (_year == d._year && _month != d._month)
{
return false;
}
else if (_year == d._year && _month == d._month && _day != d._day)
{
return false;
}
else
{
return true;
}
}
bool Date::operator<=(const Date& d) const
{
return (*this < d || *this == d);
}
bool Date::operator>(const Date& d) const
{
return !(*this <= d);
}
bool Date::operator>=(const Date& d) const
{
return !(*this < d);
}
bool Date::operator!=(const Date& d) const
{
return !(*this == d);
}
// 获取月份天数
int Date::GetMonthDay(int year, int month)
{
static int dayArray[13] = { 0, 31,28,31,30,31,30,31,31,30,31,30,31 };
if (month == 2 && year % 4 == 0 && year % 100 != 0 || year % 400 == 0)
{
return 29;
}
else
{
return dayArray[month];
}
}
// + += - -= 天数
Date& Date::operator+=(const int day)
{
if (_day < 0)
{
return *this -= -_day;
}
_day += day;
while (_day > GetMonthDay(_year, _month))
{
_day -= GetMonthDay(_year, _month);
_month++;
if (_month == 13)
{
_year++;
_month = 1;
}
}
return *this;
}
Date Date::operator+(const int day) const
{
Date tmp(*this);
tmp += day;
return tmp;
}
Date& Date::operator-=(const int day)
{
if (_day < 0)
{
return *this += -_day;
}
_day -= day;
while (_day <= 0)
{
_month--;
if (_month == 0)
{
_year--;
_month = 12;
}
_day += GetMonthDay(_year, _month);
}
return *this;
}
Date Date::operator-(const int day) const
{
Date tmp(*this);
tmp -= day;
return tmp;
}
// 前置++
Date& Date::operator++()
{
*this += 1;
return *this;
}
// 后置++
Date Date::operator++(int)
{
Date tmp = *this;
*this += 1;
return tmp;
}
// 前置--
Date& Date::operator--()
{
*this -= 1;
return *this;
}
// 后置--
Date Date::operator--(int)
{
Date tmp = *this;
*this -= 1;
return tmp;
}
// 日期-日期
int Date::operator-(const Date& d) const
{
Date max = *this;
Date min = d;
int flag = 1;
if (max < min)
{
max = d;
min = *this;
flag = -1;
}
int num = 0;
while (min != max)
{
num++;
++min;
}
return num * flag;
}
ostream& operator<<(ostream& out, const Date& d)
{
out << d._year << "年" << d._month << "月" << d._day << "日" << endl;
return out;
}
istream& operator>>(istream& in, Date& d)
{
int year, month, day;
in >> year >> month >> day;
if (month > 0 && month < 13 && day > 0 && day <= d.GetMonthDay(year, month))
{
d._year = year;
d._month = month;
d._day = day;
}
else
{
cout << "非法日期" << endl;
assert(false);
}
return in;
}
cpp
// test.cpp
#define _CRT_SECURE_NO_WARNINGS
#include "Date.h"
void TestDate1()
{
Date d1(2023, 9, 1);
Date d2(2000, 1, 1);
Date d3(2000, 3, 1);
cout << (d1 < d2) << endl;
cout << (d1 == d2) << endl;
cout << (d2 == d3) << endl;
cout << (d1 <= d2) << endl;
cout << (d1 > d2) << endl;
cout << (d2 <= d3) << endl;
cout << (d2 > d3) << endl;
Date d4(1949, 10, 1);
Date d5(1949, 10, 1);
cout << (d4 != d5) << endl;
}
void TestDate2()
{
Date d1(2024, 5, 5);
d1 += 1000;
d1.Print();
Date d2(2024, 10, 1);
(d2 + 100).Print();
Date d3(2024, 12, 31);
d3 -= 100;
d3.Print();
}
void TestDate3()
{
Date d1(2024, 5, 5);
Date d2(2050, 12, 30);
cout << d1 - d2 << endl;
cout << d2 - d1 << endl;
}
void TestDate4()
{
Date d1(2024, 5, 5);
Date d2(2050, 12, 30);
Date d3(1328, 1, 4);
d1 = d2 = d3;
}
void TestDate5()
{
Date d1(2024, 5, 5);
Date d2(2050, 12, 30);
Date d3(1328, 1, 4);
d1 += 1000;
d2 -= 50;
d3 += 500;
cout << d1;
cout << d2;
cout << d3;
}
void TestDate6()
{
Date d1;
Date d2;
cin >> d2 >> d1;
cout << d1 << d2;
}
void TestDate7()
{
Date d1(1328, 1, 1);
d1.Print();
const Date d2(1368, 1, 4);
d2.Print();
}
void TestDate8()
{
Date d1(1328, 1, 1);
const Date d2(1368, 1, 4);
cout << (d1 < d2) << endl;
}
int main()
{
TestDate8();
return 0;
}
二、this指针的const修饰
cpp
// 简单的日期类
#include <iostream>
using namespace std;
class Date
{
public:
Date(int year = 1368, int month = 1, int day = 4)
{
_year = year;
_month = month;
_day = day;
}
void Print()
{
cout << _year << "-" << _month << "-" << _day << endl;
}
Date(const Date& d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
~Date()
{
_year = 0;
_month = 0;
_day = 0;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(1949, 10, 1);
d1.Print();
const Date d2(1945, 8, 15);
d2.Print();
return 0;
}
上述日期类实现无法打印d2,原因如下:
使用const修饰创建类,使d2在调用Print函数时,传入的this指针是有const修饰的,与日期类定义类型冲突,所以报错。如下:
修改如下:
cpp
void Print() const
{
cout << _year << "-" << _month << "-" << _day << endl;
}
使this指针变为const修饰,需要在成员函数名后加const修饰。
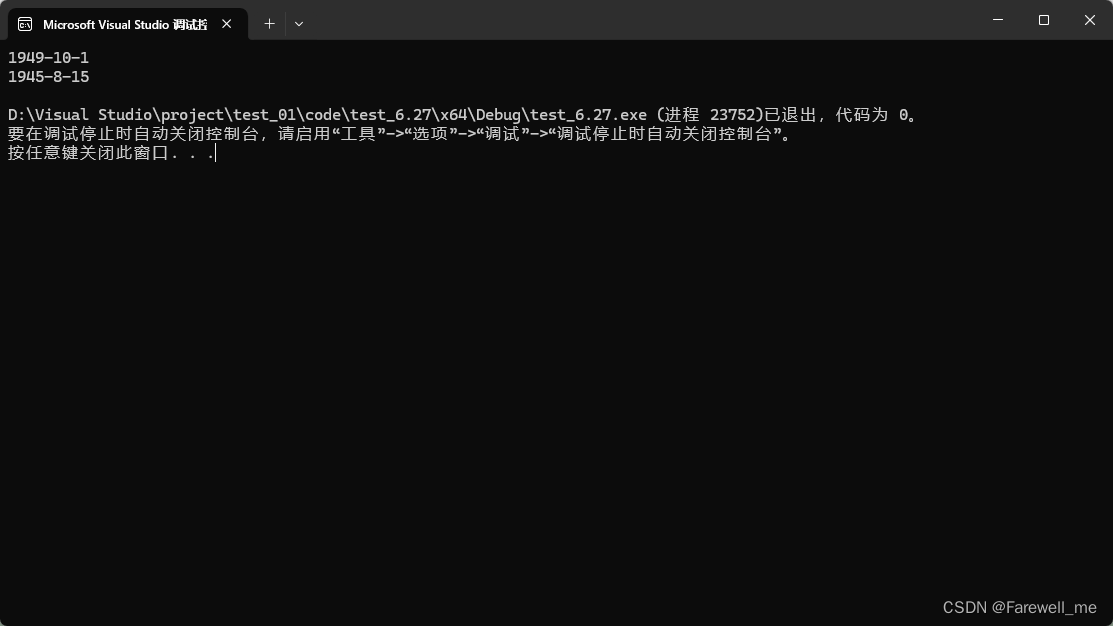
总结
C++日期类的完整实现,以及this指针的const修饰等的介绍