回顾
DOM原生事件event
对象
当事件发生时,浏览器会创建一个event
对象,并将其作为参数传递给事件处理函数。这个对象包含了事件的详细信息,比如:
type
:事件的类型(如 'click')target
:触发事件的元素currentTarget
:绑定事件监听器的元素(对于捕获和冒泡阶段,这可能不是触发事件的元素)stopPropagation()
:阻止事件进一步传播(冒泡或捕获)preventDefault()
:阻止事件的默认行为(比如,阻止链接的跳转)timeStamp
:事件发生的时间戳
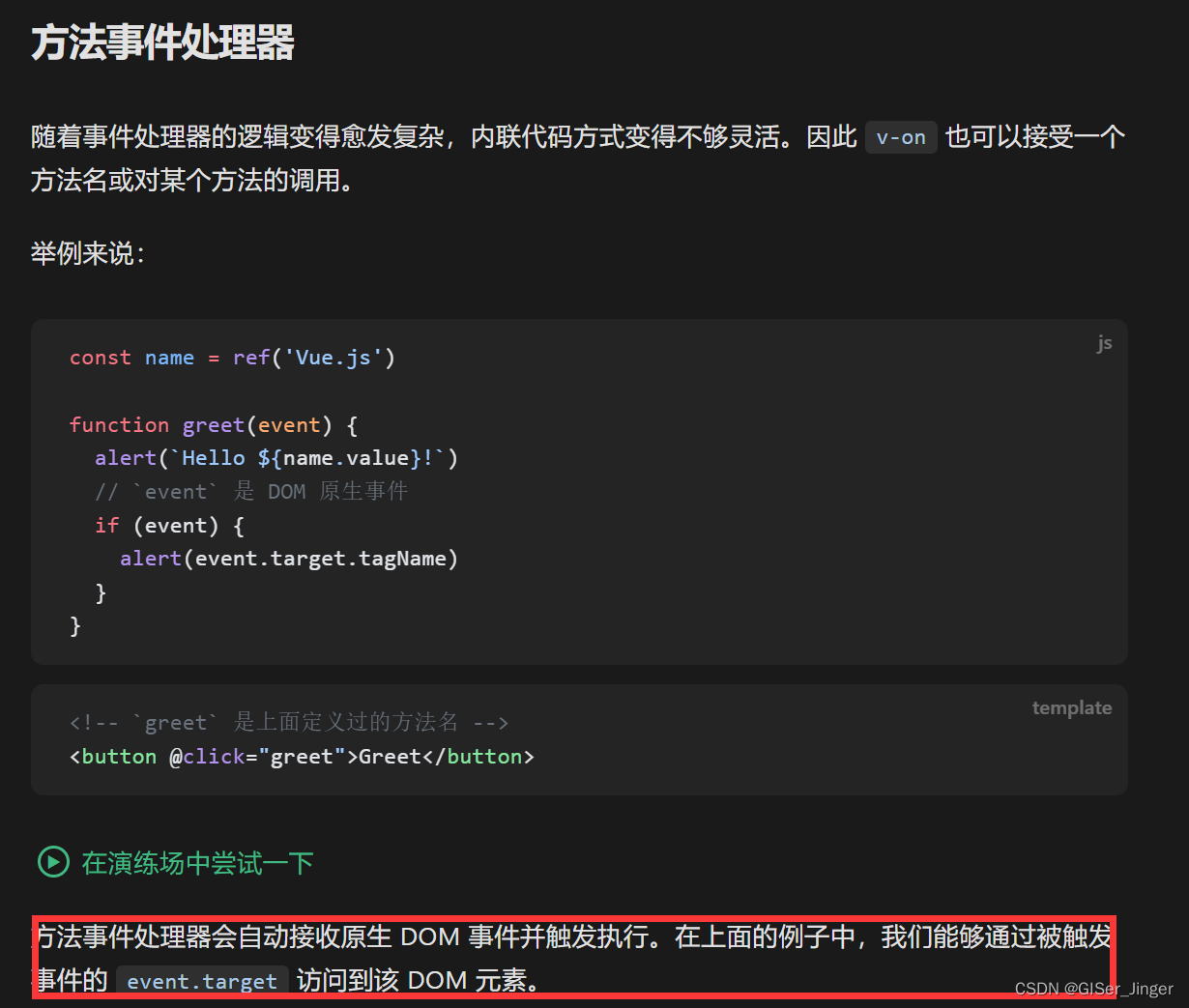
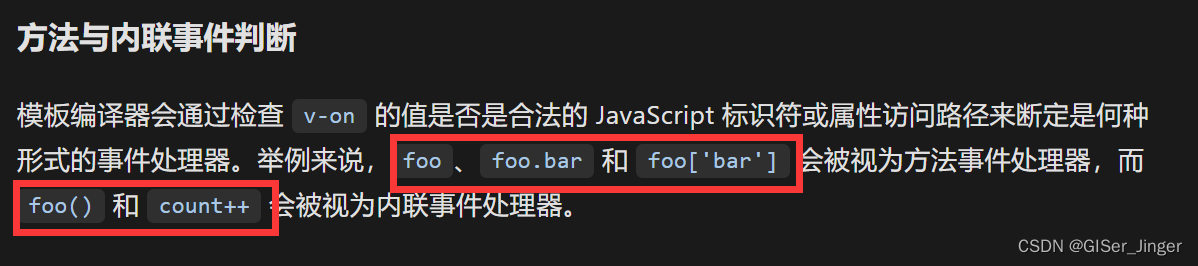
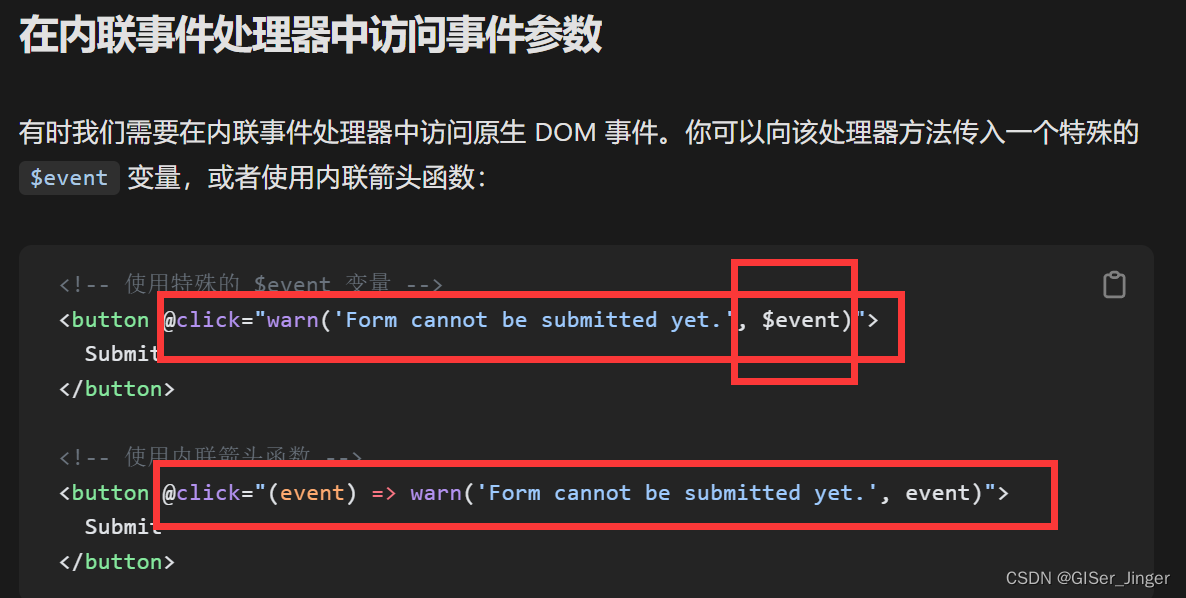
watch
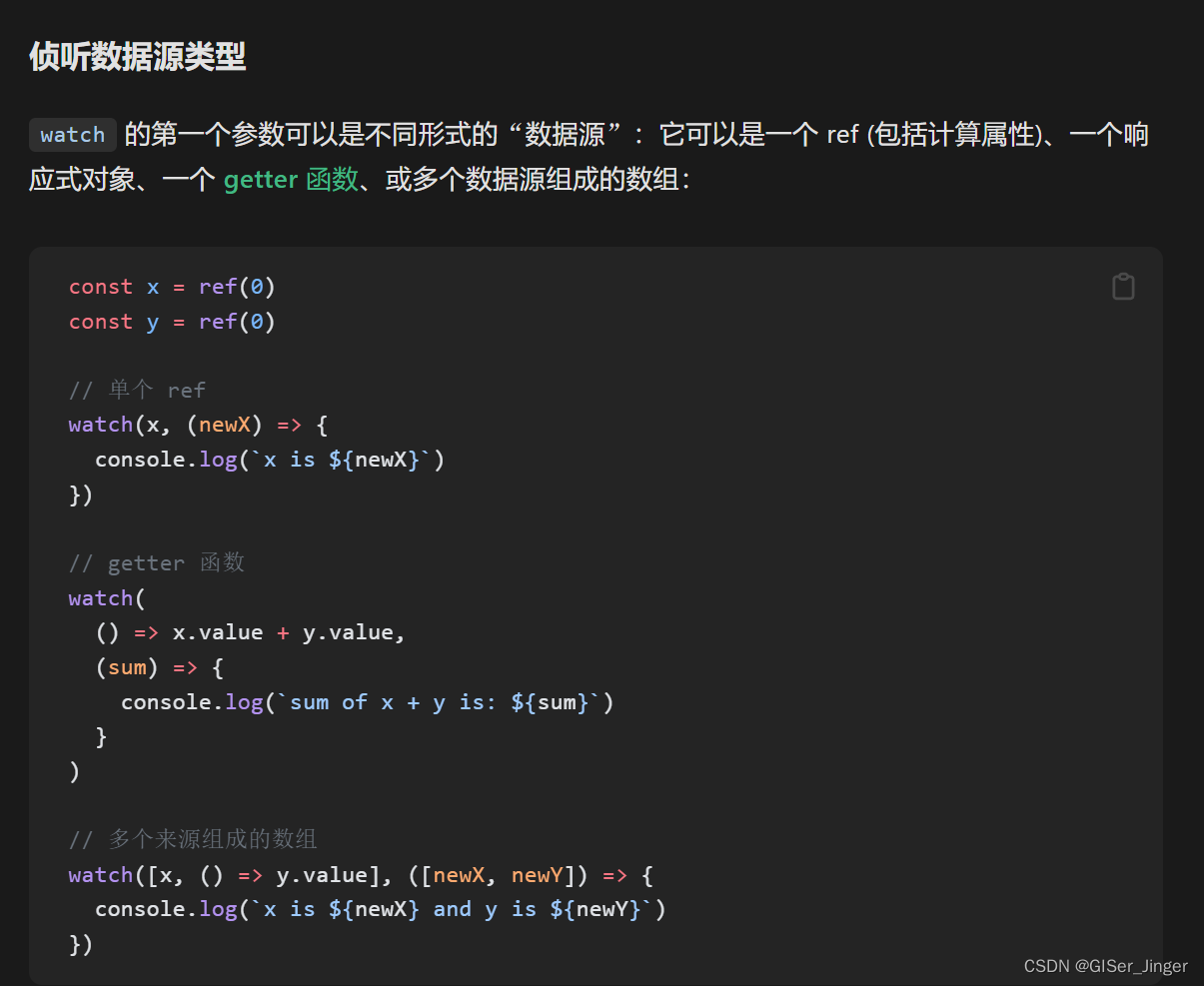
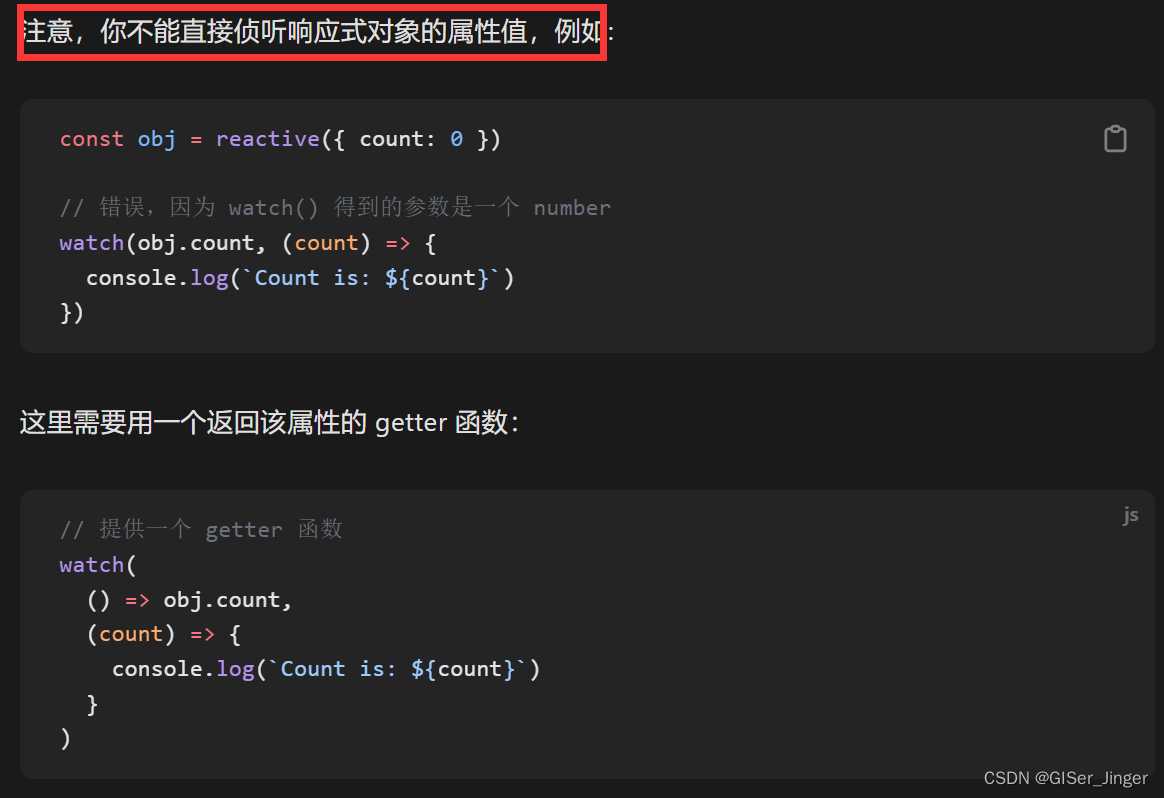
Today
组件注册
全局
import { createApp } from 'vue'
const app = createApp({})
app.component(
// 注册的名字
'MyComponent',
// 组件的实现
{
/* ... */
}
)
全局注册虽然很方便,但有以下几个问题:
全局注册,但并没有被使用的组件无法在生产打包时被自动移除 (也叫"tree-shaking")。如果你全局注册了一个组件,即使它并没有被实际使用,它仍然会出现在打包后的 JS 文件中。
全局注册在大型项目中使项目的依赖关系变得不那么明确。在父组件中使用子组件时,不太容易定位子组件的实现。和使用过多的全局变量一样,这可能会影响应用长期的可维护性。
局部
<script setup>
import ComponentA from './ComponentA.vue'
</script>
<template>
<ComponentA />
</template>
-----------------------------------------------
import ComponentA from './ComponentA.js'
export default {
components: {
ComponentA
},
setup() {
// ...
}
}
props
defineProps()
宏来声明- props: ['foo']选项式声明
修改props
校验
$emit
![]()
Vue 3带来了许多新特性和改进,包括对Composition API的官方支持、更好的TypeScript集成、性能改进等。以下是一些Vue 3中常见的语法写法:
1. Composition API
Composition API 是 Vue 3 的核心特性之一,它提供了一种更加灵活和强大的方式来组织和重用逻辑。
<template>
<div>{{ count }}</div>
<button @click="increment">Increment</button>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const count = ref(0);
function increment() {
count.value++;
}
return { count, increment };
}
};
</script>
2. 选项式API(仍受支持)
虽然Composition API是Vue 3的亮点,但Vue 3仍然完全支持Vue 2中的选项式API。
<template>
<div>{{ message }}</div>
<button @click="reverseMessage">Reverse</button>
</template>
<script>
export default {
data() {
return {
message: 'Hello Vue!'
};
},
methods: {
reverseMessage() {
this.message = this.message.split('').reverse().join('');
}
}
};
</script>
4. Teleport
Teleport
是一种能够将模板内容"传送"到DOM中Vue应用之外的其他位置的技术。
<template>
<teleport to="#modal-container">
<div class="modal">
...
</div>
</teleport>
</template>
5. 自定义指令
Vue 3中的自定义指令与Vue 2类似,但在使用Composition API时,注册方式略有不同。
<template>
<div v-focus></div>
</template>
<script>
export default {
directives: {
focus: {
mounted(el) {
el.focus();
}
}
}
};
</script>
// 在Composition API中
<script>
import { onMounted, ref } from 'vue';
export default {
setup() {
const el = ref(null);
onMounted(() => {
el.value?.focus();
});
return { el };
},
directives: {
focus: {
mounted(el) {
// 注意:这里不直接操作DOM,而是传递一个引用
el.value = el;
}
}
}
};
</script>
注意:在Composition API中使用自定义指令时,可能需要一些变通方法来获取DOM元素,因为setup
函数中没有this
上下文。
6. Props 和 Emits
Props 和 Emits 的使用与Vue 2相似,但在Composition API中,你可能需要使用defineProps
和defineEmits
。
<script setup>
import { defineProps, defineEmits } from 'vue';
const props = defineProps({
title: String
});
const emit = defineEmits(['update:title']);
function updateTitle(newTitle) {
emit('update:title', newTitle);
}
</script>
这些是Vue 3中一些常见的语法和特性。Vue 3提供了更灵活、更强大的开发方式,同时也保持了与Vue 2的兼容性。