需求
目的是要实现视图的自定义的渐变背景色,实现一个能够随时使用的工具。
实现讨论
在 iOS 中,如果设置视图单一的背景色,是很简单的。可是,如果要设置渐变的背景色,该怎么实现呢?其实也没有很是麻烦,其中使用到了 CAGradientLayer 类,只要设置好 CAGradientLayer 对象的属性,然后添加到指定视图即可。
CAGradientLayer 类
- locations: 指定每一个渐变段的结束
- startPoint:对应的是第一个渐变结束的点(指的是指定的颜色中,第一个颜色渐变停止的位置)
- endPoint:对应的是最后一个渐变结束的点(指的是指定的颜色中,最后一个颜色中渐变停止的位置)
- colors: 指定每个渐变区间的颜色
关于 locations 代码注释文档如下:
Swift
/* An optional array of NSNumber objects defining the location of each
* gradient stop as a value in the range [0,1]. The values must be
* monotonically increasing. If a nil array is given, the stops are
* assumed to spread uniformly across the [0,1] range. When rendered,
* the colors are mapped to the output colorspace before being
* interpolated. Defaults to nil. Animatable. */
open var locations: [NSNumber]?
locations 中的每一个值,指定的是在渐变方向上每一个渐变停止的位置。默认为空,如果为空,则为均匀渐变。数组的大小不必和渐变区间的数量相同。
渐变的方向就是两个点连接后指向的方向。
注意这里的坐标:[0, 0] 代表的是图层的左上角,[1, 1] 代表的算是右下角。苹果的代码文档中说的是错误的,注释中的是 macOS 的坐标系统,stackoverflow 上的相关问题 https://stackoverflow.com/questions/20387803/how-to-change-cagradientlayer-color-points
Swift
/* The start and end points of the gradient when drawn into the layer's
* coordinate space. The start point corresponds to the first gradient
* stop, the end point to the last gradient stop. Both points are
* defined in a unit coordinate space that is then mapped to the
* layer's bounds rectangle when drawn. (I.e. [0,0] is the bottom-left
* corner of the layer, [1,1] is the top-right corner.) The default values
* are [.5,0] and [.5,1] respectively. Both are animatable. */
open var startPoint: CGPoint
open var endPoint: CGPoint
示例
1 右下方向渐变
Swift
startPoint = CGPoint(x: 0, y: 0)
endPoint = CGPoint(x: 0.5, y: 0.5)
colors = [UIColor.green.cgColor, UIColor.red.cgColor]
绿色是从左上角 (0, 0) 开始渐变,红色是截止到 (0.5, 0.5) 不再渐变,右下部分的红色都是纯红色的
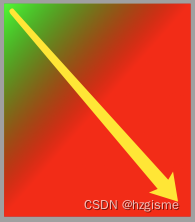
2. 整个视图从左上角到右下角渐变
Swift
startPoint = CGPoint(x: 0, y: 0)
endPoint = CGPoint(x: 1, y: 1)
colors = [UIColor.green.cgColor, UIColor.red.cgColor]
3. 调换颜色渐变方向
Swift
startPoint = CGPoint(x: 1, y: 1)
endPoint = CGPoint(x: 0, y: 0)
colors = [UIColor.green.cgColor, UIColor.red.cgColor]
4. 垂直渐变
Swift
startPoint = CGPoint(x: 0, y: 0)
endPoint = CGPoint(x: 0, y: 1)
colors = [UIColor.green.cgColor, UIColor.red.cgColor]
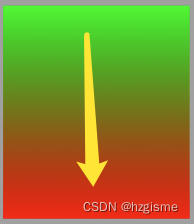
代码
Swift
/*
* 设置视图渐变背景色
*
* @param view 要设置的视图
* @param frame 区域大小
* @param colors 渐变颜色数组
* @param horizontal 渐变方向
* @param cornerRadius 圆角大小
*
*/
public static func setGradientBackgroundColor(view: UIView, frame: CGRect, colors: [CGColor], horizontal: Bool, cornerRadius: CGFloat = 0.0) -> CAGradientLayer {
let startPoint = CGPoint.init(x: 0.0, y: 0.0)
var endPoint = CGPoint.init(x: 1.0, y: 0.0)
if horizontal == false {
endPoint = CGPoint.init(x: 0.0, y: 1.0)
}
// 根据颜色的个数生成 locatios
var locations: [NSNumber] = [NSNumber]()
let interval = 1.0 / (Double(colors.count) - 1)
for (index, _) in colors.enumerated() {
locations.append(interval * Double(index) as NSNumber)
}
let gradientLayer: CAGradientLayer = getGradientLayer(frame: frame, startPoint: startPoint, endPoint: endPoint, locations: locations, colors: colors)
gradientLayer.zPosition = -10000
gradientLayer.cornerRadius = cornerRadius
view.layer.addSublayer(gradientLayer)
return gradientLayer
}
/**
*
* 获取一个颜色渐变层
*
* @param frame 大小
* @param startPoint 颜色渐变起点
* @param endPoint 颜色渐变终点
* @param locations 颜色数组对应的点
* @param colors 渐变颜色数组
*
* @return 颜色渐变层
*
*/
public static func getGradientLayer(frame: CGRect, startPoint: CGPoint, endPoint: CGPoint, locations: [NSNumber], colors: [CGColor]) -> CAGradientLayer {
let gradientLayer = CAGradientLayer.init()
gradientLayer.frame = frame
gradientLayer.startPoint = startPoint
gradientLayer.endPoint = endPoint
gradientLayer.locations = locations
gradientLayer.colors = colors
return gradientLayer
}