一、从FinalShell获取服务器基本信息
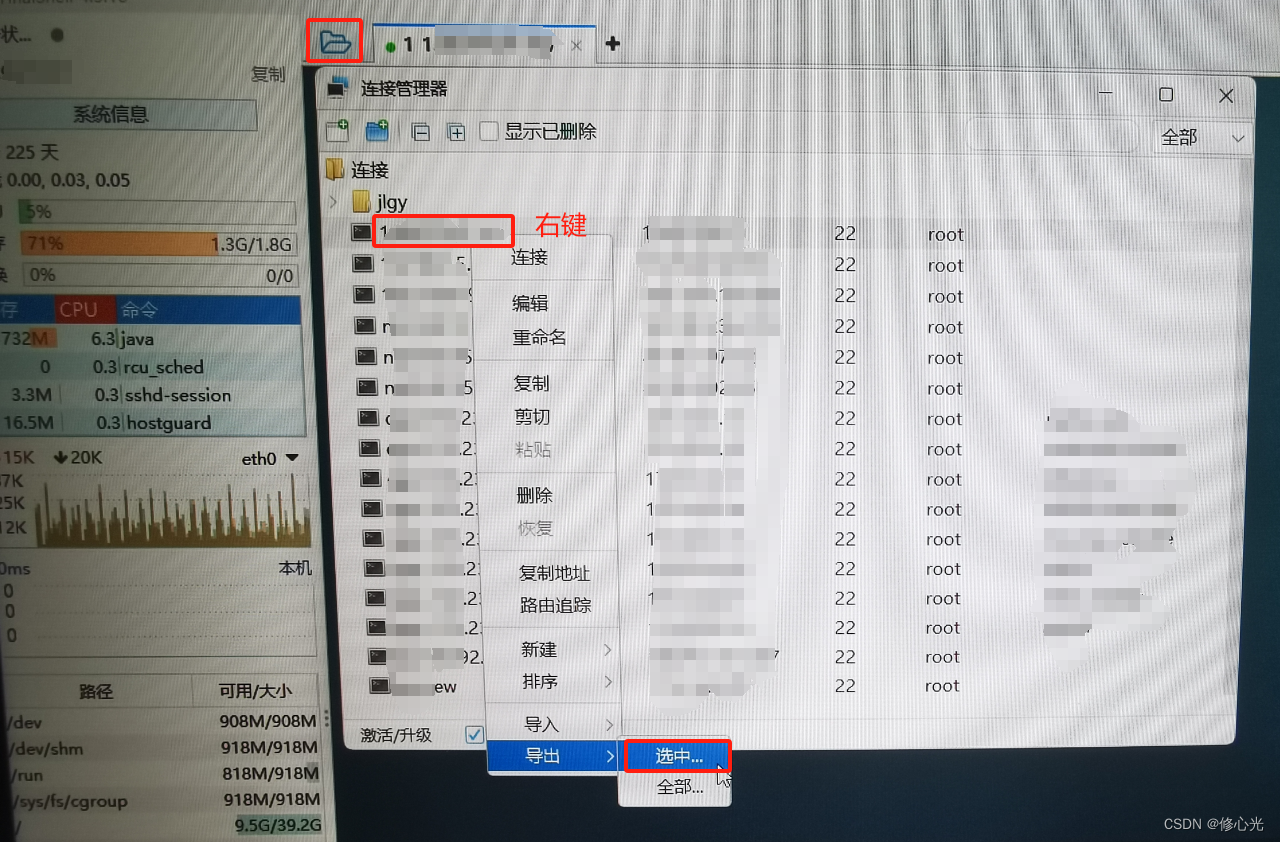
如图操作会导出一个json文件,可以直接保存在桌面,或者其他位置
json格式如下:
{"forwarding_auto_reconnect":false
,"custom_size":false
,"delete_time":0
,"secret_key_id":""
,"user_name":"root"
,"remote_port_forwarding":{}
,"conection_type":100
,"sort_time":0
,"description":""
,"proxy_id":"0"
,"authentication_type":1
,"drivestoredirect":true
,"delete_key_sequence":0
,"password":"xE4F2Pi13TnbkO8vo6wwmQNbqB77PUeI"
,"modified_time":1700460445433
,"host":"x.xx.xx.xx"
,"accelerate":false
,"id":"sn1xqbrr5womw787"
,"height":0
,"order":0
,"create_time":1700460445433
,"port_forwarding_list":[]
,"parent_update_time":0
,"rename_time":0
,"backspace_key_sequence":2
,"fullscreen":false
,"port":22
,"terminal_encoding":"UTF-8"
,"parent_id":"root"
,"exec_channel_enable":true
,"width":0
,"name":"xx.xx.xx.xx"
,"access_time":1719895278353}
二、代码读取并解析获取账号明文信息
package com.base.info.controller;
import com.alibaba.fastjson.JSON;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.Data;
import lombok.NoArgsConstructor;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.FilenameUtils;
import org.apache.commons.lang3.StringUtils;
import javax.crypto.Cipher;
import javax.crypto.SecretKey;
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.DESKeySpec;
import java.io.ByteArrayOutputStream;
import java.io.DataOutputStream;
import java.io.File;
import java.io.IOException;
import java.math.BigInteger;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.util.*;
public class FinalShellDecodePass {
public static void main(String[] args) throws Exception {
// 获取finalshell的所有json数据,json数据所在文件夹
File file = new File("C:\\Users\\12118\\Desktop"); //我的放在桌面了,如果json文件在其他位置,需要更改为对应路径
// 获取所有的json文件
File[] files = file.listFiles(pathname -> "json".equalsIgnoreCase(FilenameUtils.getExtension(pathname.getName())));
// 主要信息: ip/端口/用户名/密码
List<Map<String, Object>> infoList = new ArrayList<>(files.length);
for (File jsonFile : files) {
String s = FileUtils.readFileToString(jsonFile);
FinalShellConfig shellConfig = JSON.parseObject(s, FinalShellConfig.class);
// 密文改明文
String password = shellConfig.getPassword();
if (StringUtils.isNotBlank(password)) {
shellConfig.setPassword(decodePass(password));
}
String s1 = JSON.toJSONString(shellConfig);
System.err.println(s1);
Map<String, Object> map = new LinkedHashMap<>();
map.put("主机", shellConfig.getHost());
map.put("端口", shellConfig.getPort());
map.put("用户名", shellConfig.getUserName());
map.put("密码", shellConfig.getPassword());
infoList.add(map);
}
for (Map<String, Object> stringObjectMap : infoList) {
System.err.println(JSON.toJSONString(stringObjectMap));
}
}
public static byte[] desDecode(byte[] data, byte[] head) throws Exception {
SecureRandom sr = new SecureRandom();
DESKeySpec dks = new DESKeySpec(head);
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES");
SecretKey securekey = keyFactory.generateSecret(dks);
Cipher cipher = Cipher.getInstance("DES");
cipher.init(2, securekey, sr);
return cipher.doFinal(data);
}
public static String decodePass(String data) throws Exception {
if (data == null) {
return null;
} else {
String rs = "";
byte[] buf = Base64.getDecoder().decode(data);
byte[] head = new byte[8];
System.arraycopy(buf, 0, head, 0, head.length);
byte[] d = new byte[buf.length - head.length];
System.arraycopy(buf, head.length, d, 0, d.length);
byte[] bt = desDecode(d, ranDomKey(head));
rs = new String(bt);
return rs;
}
}
static byte[] ranDomKey(byte[] head) {
long ks = 3680984568597093857L / (long) (new Random((long) head[5])).nextInt(127);
Random random = new Random(ks);
int t = head[0];
for (int i = 0; i < t; ++i) {
random.nextLong();
}
long n = random.nextLong();
Random r2 = new Random(n);
long[] ld = new long[]{(long) head[4], r2.nextLong(), (long) head[7], (long) head[3], r2.nextLong(), (long) head[1], random.nextLong(), (long) head[2]};
ByteArrayOutputStream bos = new ByteArrayOutputStream();
DataOutputStream dos = new DataOutputStream(bos);
long[] var15 = ld;
int var14 = ld.length;
for (int var13 = 0; var13 < var14; ++var13) {
long l = var15[var13];
try {
dos.writeLong(l);
} catch (IOException var18) {
var18.printStackTrace();
}
}
try {
dos.close();
} catch (IOException var17) {
var17.printStackTrace();
}
byte[] keyData = bos.toByteArray();
keyData = md5(keyData);
return keyData;
}
public static byte[] md5(byte[] data) {
String ret = null;
byte[] res = null;
try {
MessageDigest m;
m = MessageDigest.getInstance("MD5");
m.update(data, 0, data.length);
res = m.digest();
ret = new BigInteger(1, res).toString(16);
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
return res;
}
@NoArgsConstructor
@Data
static class FinalShellConfig {
@JsonProperty("forwarding_auto_reconnect")
private Boolean forwardingAutoReconnect;
@JsonProperty("custom_size")
private Boolean customSize;
@JsonProperty("delete_time")
private Integer deleteTime;
@JsonProperty("secret_key_id")
private String secretKeyId;
@JsonProperty("user_name")
private String userName;
@JsonProperty("conection_type")
private Integer conectionType;
@JsonProperty("sort_time")
private Integer sortTime;
@JsonProperty("description")
private String description;
@JsonProperty("proxy_id")
private String proxyId;
@JsonProperty("authentication_type")
private Integer authenticationType;
@JsonProperty("drivestoredirect")
private Boolean drivestoredirect;
@JsonProperty("delete_key_sequence")
private Integer deleteKeySequence;
@JsonProperty("password")
private String password;
@JsonProperty("modified_time")
private Long modifiedTime;
@JsonProperty("host")
private String host;
@JsonProperty("accelerate")
private Boolean accelerate;
@JsonProperty("id")
private String id;
@JsonProperty("height")
private Integer height;
@JsonProperty("order")
private Integer order;
@JsonProperty("create_time")
private Long createTime;
@JsonProperty("port_forwarding_list")
private List<?> portForwardingList;
@JsonProperty("parent_update_time")
private Integer parentUpdateTime;
@JsonProperty("rename_time")
private Long renameTime;
@JsonProperty("backspace_key_sequence")
private Integer backspaceKeySequence;
@JsonProperty("fullscreen")
private Boolean fullscreen;
@JsonProperty("port")
private Integer port;
@JsonProperty("terminal_encoding")
private String terminalEncoding;
@JsonProperty("parent_id")
private String parentId;
@JsonProperty("exec_channel_enable")
private Boolean execChannelEnable;
@JsonProperty("width")
private Integer width;
@JsonProperty("name")
private String name;
@JsonProperty("access_time")
private Long accessTime;
}
}
三、执行main方法,获取信息

这样就获取到了!