目录
2.1使用MyBatisP1us提供有业务层通用接口(ISerivce)与业务层通用实现类(ServiceImpl),t>
1.业务层标准开发
1.1接口定义
创建BookService接口文件,如下图所示:
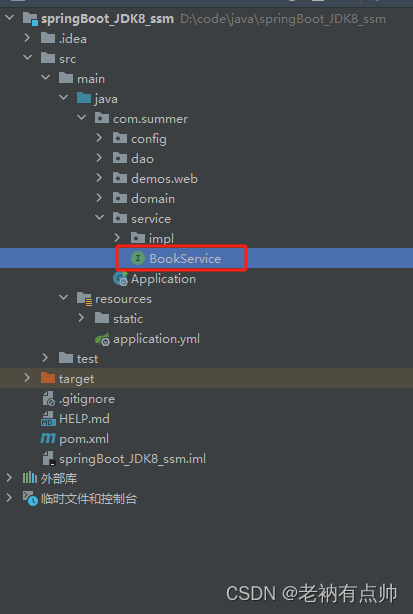
代码如下图所示:
java
package com.summer.service;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.summer.domain.Book;
import java.util.List;
public interface BookService {
Boolean save(Book book);
Boolean update(Book book);
boolean delete(Integer id);
Book getById(Integer id);
List<Book> getAll();
IPage<Book> getPage(Integer currentPage,Integer pageSize);
}
1.2实现类定义
创建BookService实现类文件,如下图所示:
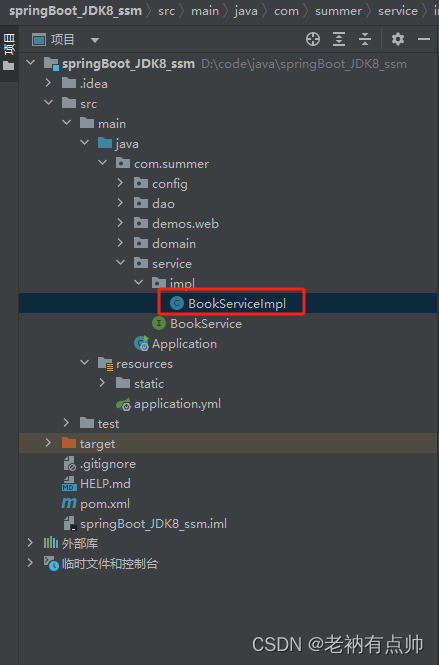
代码如下图所示:
java
package com.summer.service.impl;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.summer.dao.BookDao;
import com.summer.domain.Book;
import com.summer.service.BookService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class BookServiceImpl implements BookService {
@Autowired
private BookDao bookDao;
@Override
public Boolean save(Book book) {
return bookDao.insert(book)>0;
}
@Override
public Boolean update(Book book) {
return bookDao.updateById(book) >0 ;
}
@Override
public boolean delete(Integer id) {
return bookDao.deleteById(id)>0;
}
@Override
public Book getById(Integer id) {
return bookDao.selectById(id);
}
@Override
public List<Book> getAll() {
return bookDao.selectList(null);
}
@Override
public IPage<Book> getPage(Integer currentPage, Integer pageSize) {
IPage page = new Page(currentPage,pageSize);
return bookDao.selectPage(page,null);
}
}
1.3测试类定义
创建BookServiceTestCase测试类文件,如下图所示:
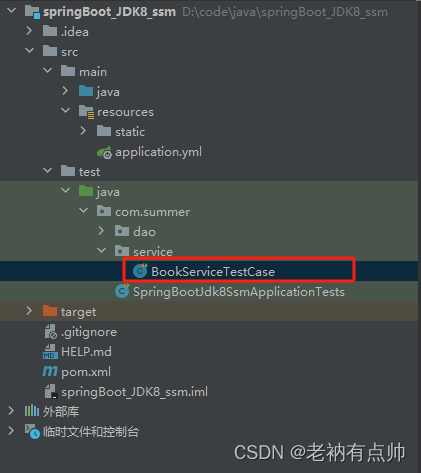
代码如下图所示:
java
package com.summer.service;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.summer.domain.Book;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class BookServiceTestCase {
@Autowired
private BookService bookService;
@Test
void testGetById()
{
System.out.println(bookService.getById(1));
}
@Test
void testSave() {
Book book = new Book();
book.setType("历史");
book.setName("历史的温度");
book.setDescription("通过讲述历史人物的故事,展现了历史的温情和人性的光辉");
bookService.save(book);
}
@Test
void testGetAll()
{
System.out.println(bookService.getAll());
}
@Test
void testUpdate(){
Book book = new Book();
book.setId(7);
book.setType("历史");
book.setName("历史的温度1");
book.setDescription("通过讲述历史人物的故事,展现了历史的温情和人性的光辉");
System.out.println(bookService.update(book));
}
@Test
void testDelete()
{
bookService.delete(15);
}
@Test
void testGetPage() {
IPage<Book> result= bookService.getPage(1,5);
System.out.println(result.getPages());
System.out.println(result.getSize());
System.out.println(result.getCurrent());
System.out.println(result.getRecords());
System.out.println(result.getTotal());
}
}
1.4小结:
1.Service接口名称定义成业务名称,并与Dao接口名称进行区分
2.制作测试类测试Service功能是否有效
2.业务层快速开发
2.1使用MyBatisP1us提供有业务层通用接口(ISerivce<T>)与业务层通用实现类(ServiceImpl<M,T>)
接口定义:
新建接口类文件,位置如下图所示:
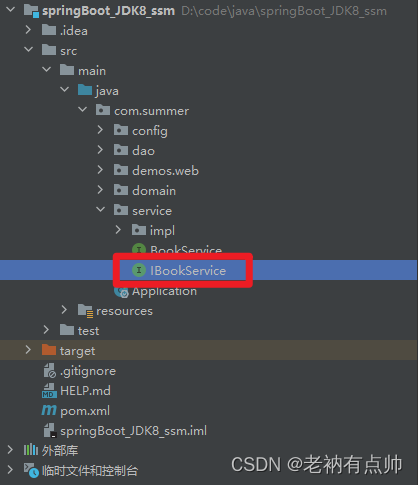
接口代码定义,如下所示:
java
package com.summer.service;
import com.baomidou.mybatisplus.extension.service.IService;
import com.summer.domain.Book;
public interface IBookService extends IService<Book> {
}
实现类定义:
java
package com.summer.service.impl;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.summer.dao.BookDao;
import com.summer.domain.Book;
import com.summer.service.IBookService;
import org.springframework.stereotype.Service;
@Service
public class BookServiceImpl extends ServiceImpl<BookDao, Book> implements IBookService {
}
测试类:
java
package com.summer.service;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.summer.domain.Book;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class BookServiceTestCase {
@Autowired
private IBookService bookService;
@Test
void testGetById()
{
System.out.println(bookService.getById(1));
}
@Test
void testSave() {
Book book = new Book();
book.setType("历史");
book.setName("历史的温度");
book.setDescription("通过讲述历史人物的故事,展现了历史的温情和人性的光辉");
bookService.save(book);
}
@Test
void testGetAll()
{
System.out.println(bookService.list());
}
@Test
void testUpdate(){
Book book = new Book();
book.setId(7);
book.setType("历史");
book.setName("历史的温度1");
book.setDescription("通过讲述历史人物的故事,展现了历史的温情和人性的光辉");
System.out.println(bookService.updateById(book));
}
@Test
void testDelete()
{
bookService.removeById(16);
}
@Test
void testGetPage() {
IPage<Book> page = new Page<Book>(1,5);
bookService.page(page,null);
System.out.println(page.getPages());
System.out.println(page.getSize());
System.out.println(page.getCurrent());
System.out.println(page.getRecords());
System.out.println(page.getTotal());
}
}
2.2在通用类基础上做功能重载或功能追加
接口定义
追加的操作与原始操作通过名称区分,功能类似,代码如下所示:
java
package com.summer.service;
import com.baomidou.mybatisplus.extension.service.IService;
import com.summer.domain.Book;
public interface IBookService extends IService<Book> {
Boolean delete(Integer id);
Boolean insert(Book book);
Boolean modify(Book book);
Book get(Integer id);
}
- 实现类定义:
java
package com.summer.service.impl;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.summer.dao.BookDao;
import com.summer.domain.Book;
import com.summer.service.IBookService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class BookServiceImpl extends ServiceImpl<BookDao, Book> implements IBookService {
@Autowired
private BookDao bookDao;
public Boolean insert(Book book) {
return bookDao.insert(book) > 0;
}
public Boolean modify(Book book) {
return bookDao.updateById(book) > 0;
}
public Boolean delete(Integer id) {
return bookDao.deleteById(id) > 0;
}
public Book get(Integer id) {
return bookDao.selectById(id);
}
}
2.3注意重载时不要覆盖原始操作,避免原始提供的功能丢失
自己定义的方法名尽量不要覆盖原始操作,避免原始提供的功能丢失,如果特殊情况,必须一样,必须在方法名上面添加注释 @Override
自定义方法:
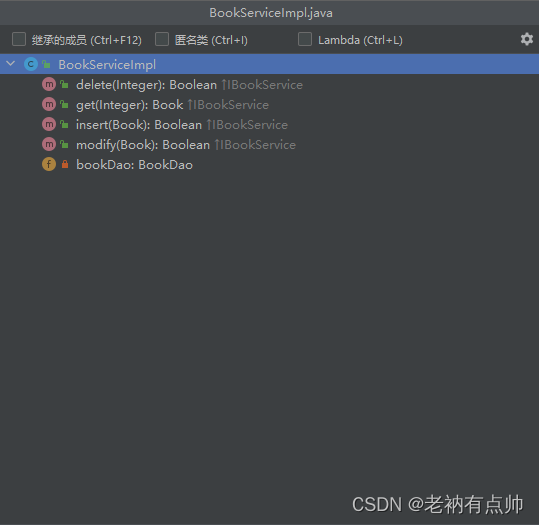
- 原始操作:
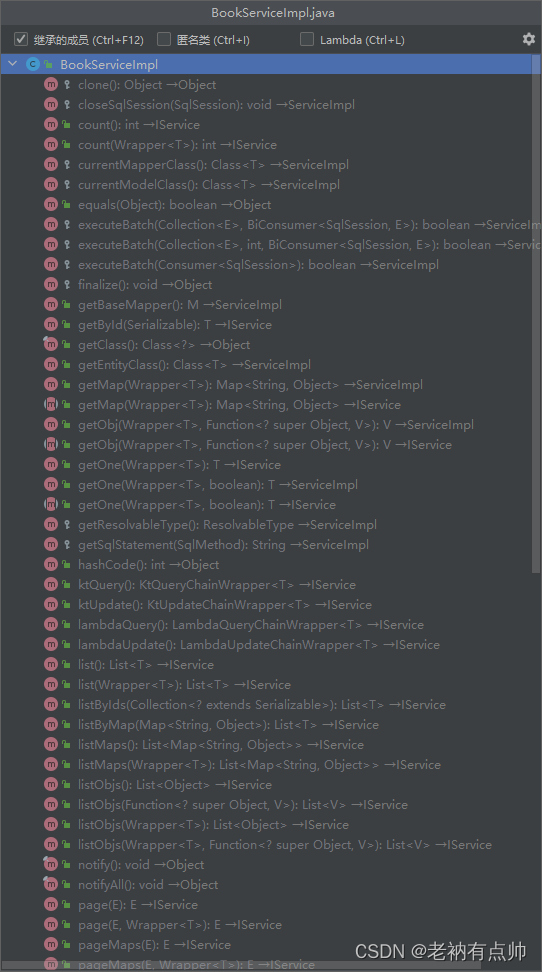
2.4小结:
1.使用通用接口(ISerivce<T>)快速开发Service
2.使用通用实现类(ServiceImpl<M,T>)快速开发ServiceImp1
3.可以在通用接口基础上做功能重载或功能追加
4.注意重载时不要覆盖原始操作,避免原始提供的功能丢失