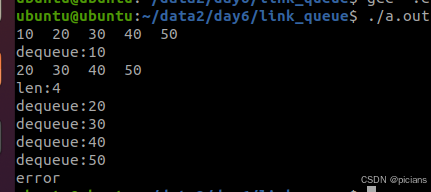
主程序
#include "fun.h"
int main(int argc, const char *argv[])
{
que_p Q=create();
enqueue(Q,10);
enqueue(Q,20);
enqueue(Q,30);
enqueue(Q,40);
enqueue(Q,50);
show_que(Q);
dequeue(Q);
show_que(Q);
printf("len:%d\n",que_len(Q));
free_que(Q);
Q=NULL;
show_que(Q);
return 0;
}
源程序
#include "fun.h"
que_p create()
{
que_p Q=(que_p)malloc(sizeof(que));
if(Q==NULL)
{
printf("error\n");
return NULL;
}
node_p H=(node_p)malloc(sizeof(node));
if(H==NULL)
{
printf("error\n");
free(Q);
Q=NULL;
return NULL;
}
H->next=NULL;
H->len=0;
Q->head=H;
Q->tail=H;
return Q;
}
int empty_que(que_p Q)
{
if(Q==NULL)
{
printf("error\n");
return -1;
}
return Q->head==Q->tail;
}
node_p create_new(typdata data)
{
node_p new =(node_p)malloc(sizeof(node));
if(new==NULL)
{
printf("error");
}
new->data=data;
new->next=NULL;
return new;
}
void enqueue(que_p Q,typdata data)
{
if(Q==NULL)
{
printf("erro\n");
}
node_p new=create_new(data);
Q->tail->next=new;
Q->tail=new;
Q->head->len++;
}
void show_que(que_p Q)
{
if(Q==NULL)
{
printf("error\n");
return;
}
if(empty_que(Q))
{
printf("Queue empty\n");
return;
}
node_p H=Q->head;
while(H->next!=NULL)
{
H=H->next;
printf("%-4d",H->data);
}
putchar(10);
}
void dequeue(que_p Q)
{
if(Q==NULL)
{
printf("error\n");
return;
}
if(empty_que(Q))
{
printf("Queue empty\n");
return;
}
printf("dequeue:%d\n",Q->head->next->data);
node_p p=Q->head->next;
Q->head->next=Q->head->next->next;
free(p);
p=NULL;
Q->head->len--;
}
int que_len(que_p Q)
{
if(Q==NULL)
{
printf("error\n");
return-1;
}
return Q->head->len;
}
void free_que(que_p Q)
{
if(Q==NULL)
{
printf("error\n");
return;
}
while(Q->head->next!=NULL)
{
dequeue(Q);
}
free(Q->head);
Q->head=NULL;
free(Q);
Q=NULL;
}
头文件
#ifndef _FUN_H
#define _FUN_H
#include <myhead.h>
typedef int typdata;
typedef struct node
{
union
{
int len;
typdata data;
};
struct node *next;
}node,*node_p;
typedef struct queue
{
node_p head;
node_p tail;
}que,*que_p;
que_p create();
int empty_que(que_p Q);
node_p create_new(typdata data);
void enqueue(que_p Q,typdata data);
void show_que(que_p Q);
void dequeue(que_p Q);
int que_len(que_p Q);
void free_que(que_p Q);
#endif
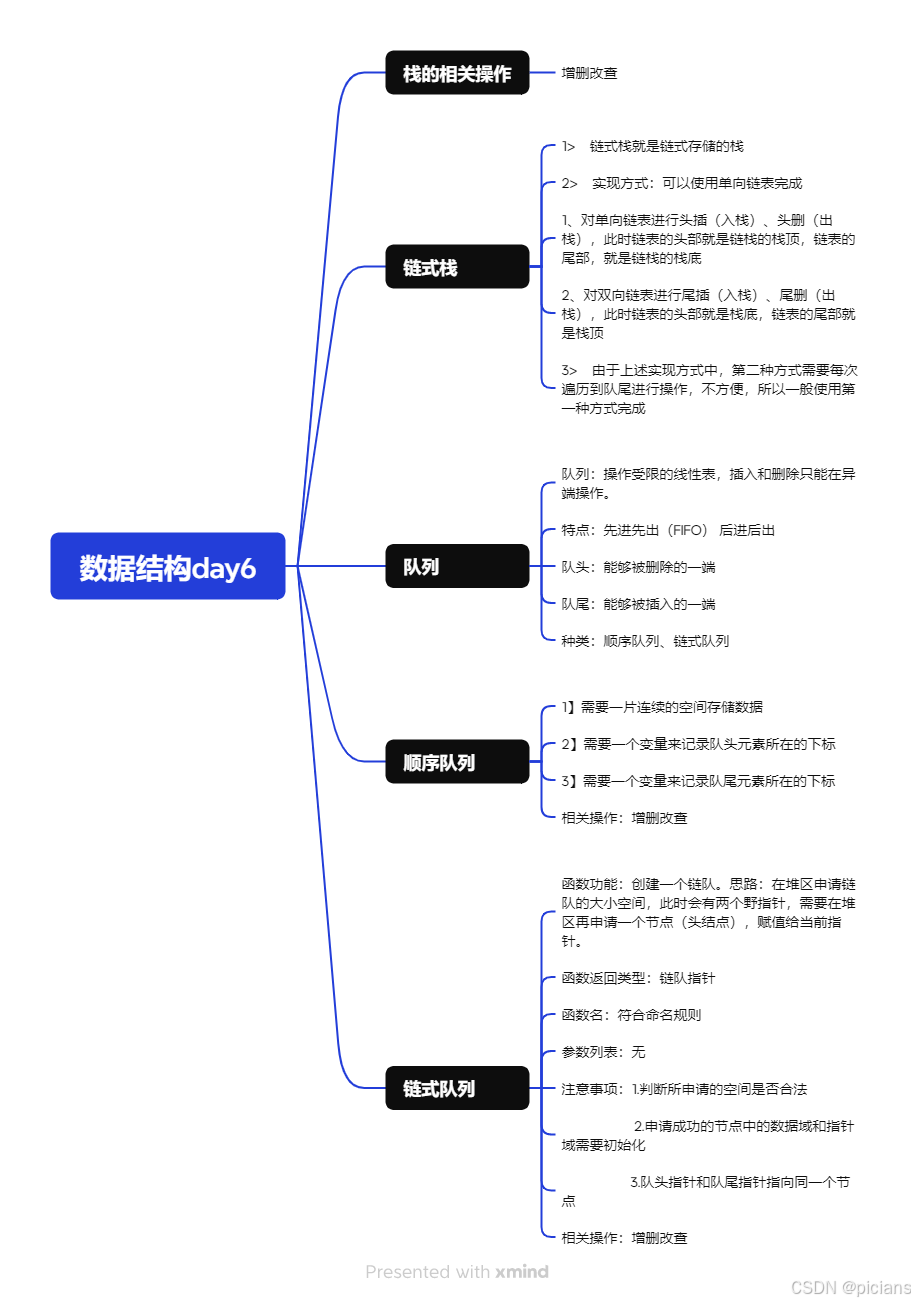