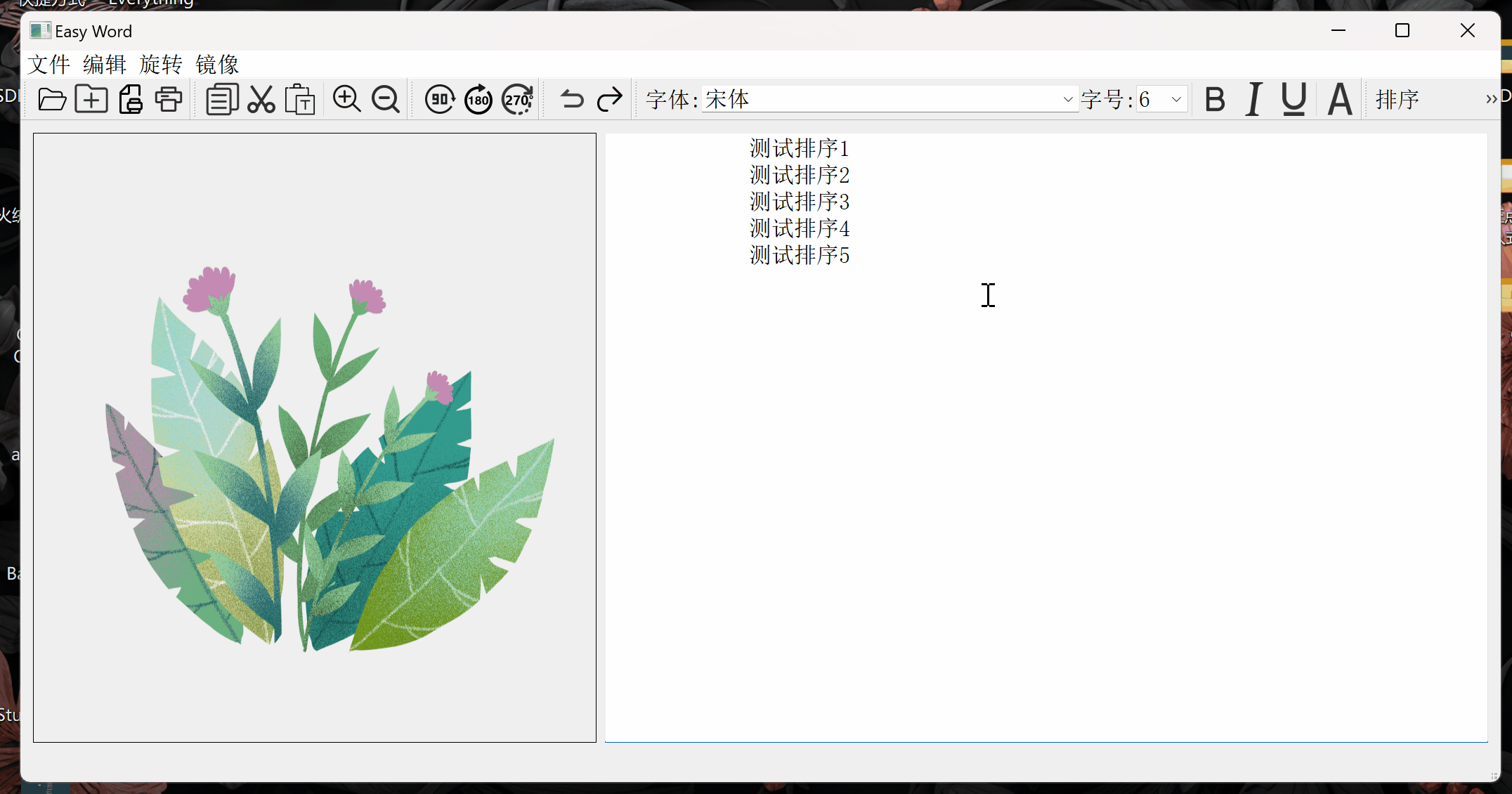
目录
一、搭建框架
(1) 在imgprocessor.h文件中添加private变量:
cpp
QLabel *listLabel; //排序设置项
QComboBox *listComboBox;
QActionGroup *actGrp;
QAction *leftAction;
QAction *rightAction;
QAction *centerAction;
QAction *justifyAction;
QToolBar *listToolBar; //排序工具栏
(2) 在文件中添加protected slots函数:
cpp
void ShowList(int);
void ShowAlignment(QAction *act);
void ShowCursorPositionChanged();
(3) 在ImgProcessor构造函数中,添加如下代码:
cpp
//排序
listLabel =new QLabel(tr("排序"));
listComboBox =new QComboBox;
listComboBox->addItem("Standard");
listComboBox->addItem("QTextListFormat::ListDisc");
listComboBox->addItem("QTextListFormat::ListCircle");
listComboBox->addItem("QTextListFormat::ListSquare");
listComboBox->addItem("QTextListFormat::ListDecimal");
listComboBox->addItem("QTextListFormat::ListLowerAlpha");
listComboBox->addItem("QTextListFormat::ListUpperAlpha");
listComboBox->addItem("QTextListFormat::ListLowerRoman");
listComboBox->addItem("QTextListFormat::ListUpperRoman");
(4) 在构造函数的最后部分添加相关的事件关联:
cpp
connect(listComboBox,SIGNAL(activated(int)),this,SLOT(ShowList(int)));
connect(showWidget->text->document(),SIGNAL(undoAvailable(bool)),redoAction,SLOT(setEnabled(bool)));
connect(showWidget->text->document(),SIGNAL(redoAvailable(bool)),redoAction,SLOT(setEnabled(bool)));
connect(showWidget->text,SIGNAL(cursorPositionChanged()),this,SLOT(ShowCursorPositionChanged()));
(5) 在相对应的工具栏 createActions 函数中添加如下代码:
cpp
//排序:左对齐、右对齐、居中和两端对齐
actGrp =new QActionGroup(this);
leftAction =new QAction(QIcon("left.png"),"左对齐",actGrp);
leftAction->setCheckable(true);
rightAction =new QAction(QIcon("right.png"),"右对齐",actGrp);
rightAction->setCheckable(true);
centerAction =new QAction(QIcon("center.png"),"居中",actGrp);
centerAction->setCheckable(true);
justifyAction =new QAction(QIcon("justify.png"),"两端对齐",actGrp);
justifyAction->setCheckable(true);
connect(actGrp,SIGNAL(triggered(QAction*)),this,SLOT(ShowAlignment(QAction*)));
(6) 在相对应的工具栏 createToolBars()函数中添加如下代码:
cpp
//排序工具条
listToolBar =addToolBar("list");
listToolBar->addWidget(listLabel);
listToolBar->addWidget(listComboBox);
listToolBar->addSeparator(); //添加一个分隔符
listToolBar->addActions(actGrp->actions());
二、实现段落对齐
完成对按下某个对齐按钮的响应用ShowAlignment()函数,根据比较判断触发的是哪个对齐按钮,调用 QTextEdit 的 setAlignment 函数可以实现当前段落的对齐调整。具体代码如下:
cpp
void ImgProcessor::ShowAlignment(QAction *act)
{
if(act==leftAction)
showWidget->text->setAlignment(Qt::AlignLeft);
if(act==rightAction)
showWidget->text->setAlignment(Qt::AlignRight);
if(act==centerAction)
showWidget->text->setAlignment(Qt::AlignCenter);
if(act==justifyAction)
showWidget->text->setAlignment(Qt::AlignJustify);
}
响应文本中光标位置处发生改变的信号的ShowCursorPositionChanged()函数代码如下:
cpp
//显示光标处的对齐方式
void ImgProcessor::ShowCursorPositionChanged()
{
if(showWidget->text->alignment()==Qt::AlignLeft)
leftAction->setChecked(true);
if(showWidget->text->alignment()==Qt::AlignRight)
rightAction->setChecked(true);
if(showWidget->text->alignment()==Qt::AlignCenter)
centerAction->setChecked(true);
if(showWidget->text->alignment()==Qt::AlignJustify)
justifyAction->setChecked(true);
}
完成四个对齐按钮的状态更新。通过调用 QTextEdit 类的alignment()函数获得当前光标所在处段落的对齐方式,设置相应的对齐按钮为按下状态。
三、实现文本排序
文本排序功能实现的基本流程,如下图所示:
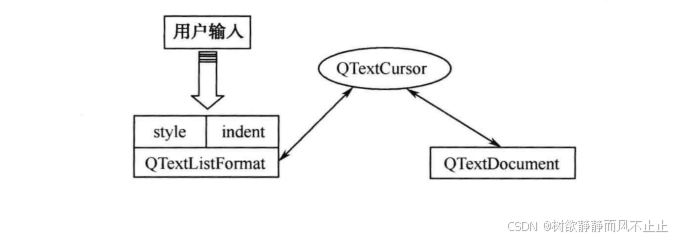
主要用于描述文本排序格式的 QTextListFormat 包含两个基本的属性,一个为QTextListFormat::style , 表 示文本 采用哪 种排序 方式;另 一 个为QTextListFormat::indent, 表示排序后的缩进值。因此,若要实现文本排序的功能则只需设置好 QTextListFormat 的以上两个属性,并将整个格式通过 QTextCursor 类应用到文本中即可。
cpp
void ImgProcessor::ShowList(int index)
{
QTextCursor cursor=showWidget->text->textCursor();
if(index!=0)
{
QTextListFormat::Style style=QTextListFormat::ListDisc; //用于描述文本排序格式QTextListFormat
switch(index) //设置style属性值
{
default:
case 1:
style=QTextListFormat::ListDisc; break;
case 2:
style=QTextListFormat::ListCircle; break;
case 3:
style=QTextListFormat::ListSquare; break;
case 4:
style=QTextListFormat::ListDecimal; break;
case 5:
style=QTextListFormat::ListLowerAlpha; break;
case 6:
style=QTextListFormat::ListUpperAlpha; break;
case 7:
style=QTextListFormat::ListLowerRoman; break;
case 8:
style=QTextListFormat::ListUpperRoman; break;
}
cursor.beginEditBlock(); //设置缩进值
QTextBlockFormat blockFmt=cursor.blockFormat();
QTextListFormat listFmt;
if(cursor.currentList())
{
listFmt= cursor.currentList()->format();
}
else
{
listFmt.setIndent(blockFmt.indent()+1);
blockFmt.setIndent(0);
cursor.setBlockFormat(blockFmt);
}
listFmt.setStyle(style);
cursor.createList(listFmt);
cursor.endEditBlock();
}
else
{
QTextBlockFormat bfmt;
bfmt.setObjectIndex(-1);
cursor.mergeBlockFormat(bfmt);
}
}