生成JWT令牌
依赖
<!--jwt令牌-->
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.0</version>
</dependency>
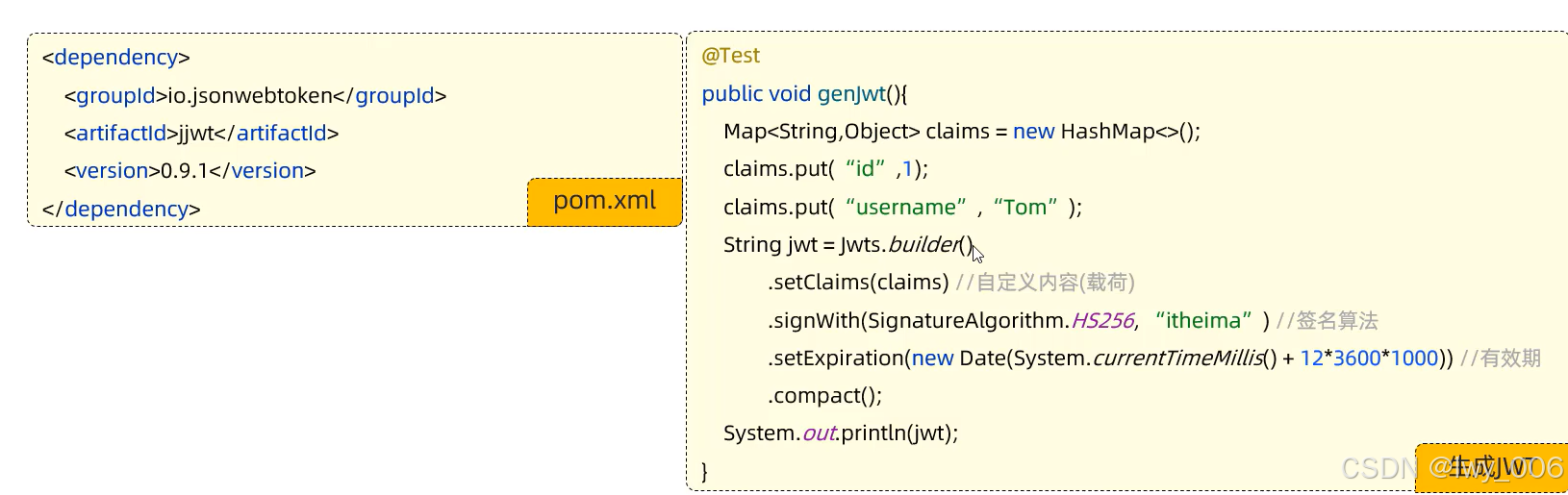
解析JWT令牌
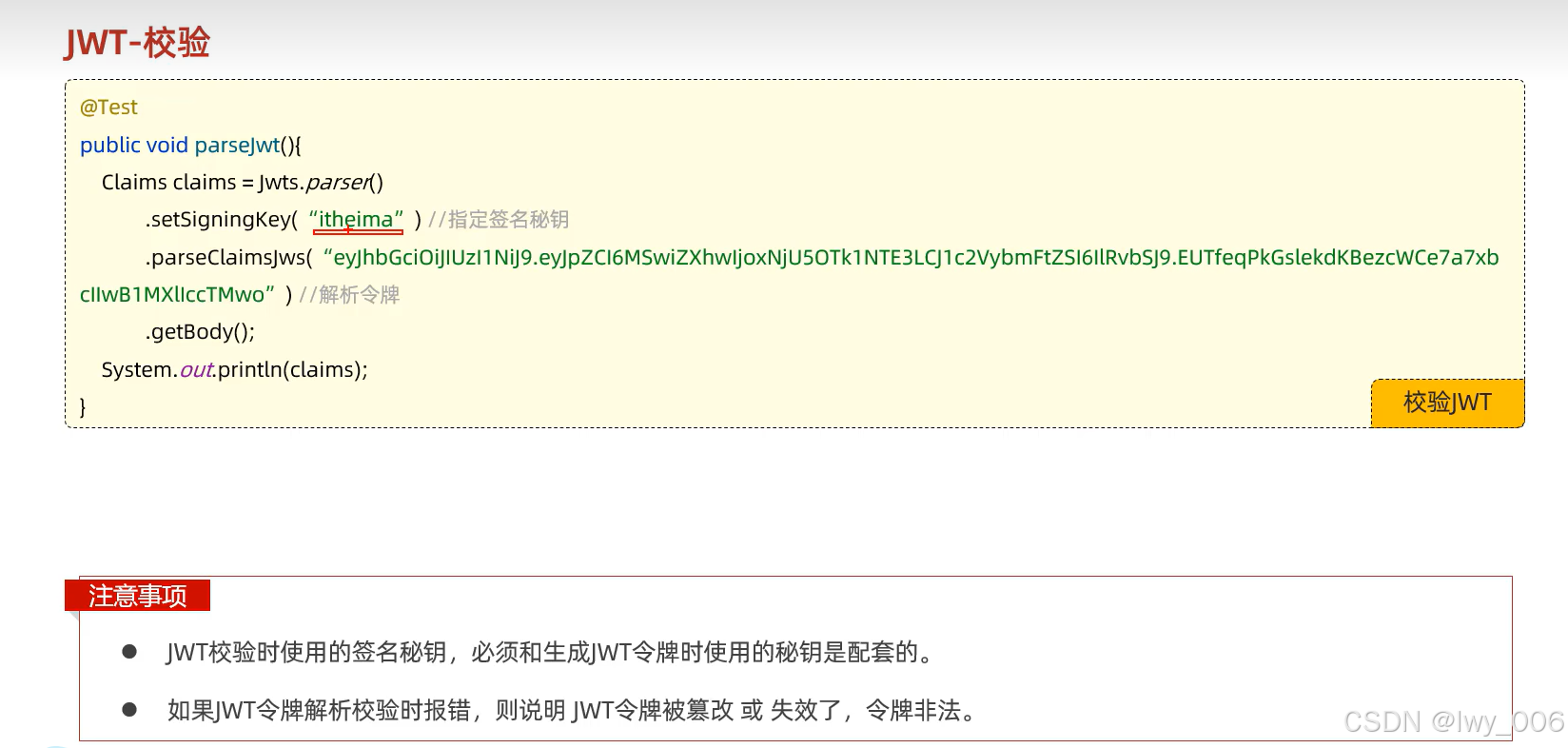
案例
java
package com.lwy.controller;
import com.lwy.pojo.Emp;
import com.lwy.pojo.Result;
import com.lwy.service.EmpService;
import com.lwy.utils.JwtUtils;
import io.jsonwebtoken.Claims;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import java.util.HashMap;
import java.util.Map;
@Slf4j
@RestController
public class LoginController {
@Autowired
private EmpService empService;
/**
* 登录
* @param emp
* @return
*/
@PostMapping("/login")
public Result login(@RequestBody Emp emp){
log.info("员工登录,{}",emp);
Emp result = empService.login(emp);
//登录成功,生成令牌jwt
if(result !=null){
Map<String,Object> claims = new HashMap<>();
claims.put("id", result.getId());
claims.put("name", result.getName());
claims.put("username",result.getUsername());
String jwt = JwtUtils.generateJwt(claims); //jwt包含了当前登录的信息
return Result.success(jwt);
}
return result !=null?Result.success("登录成功"):Result.error("用户名或密码错误");
}
}