了解数据库基本概念
什么是数据库?
• 长期存放在计算机内,有组织、可共享的大量数据的集合,是一个数据"仓库"
MySQL数据库的特点
• 开源免费,小巧但功能齐全
• 可在Windows和Linux系统上运行
• 操作方便,适用于中小型甚至大型网站应用
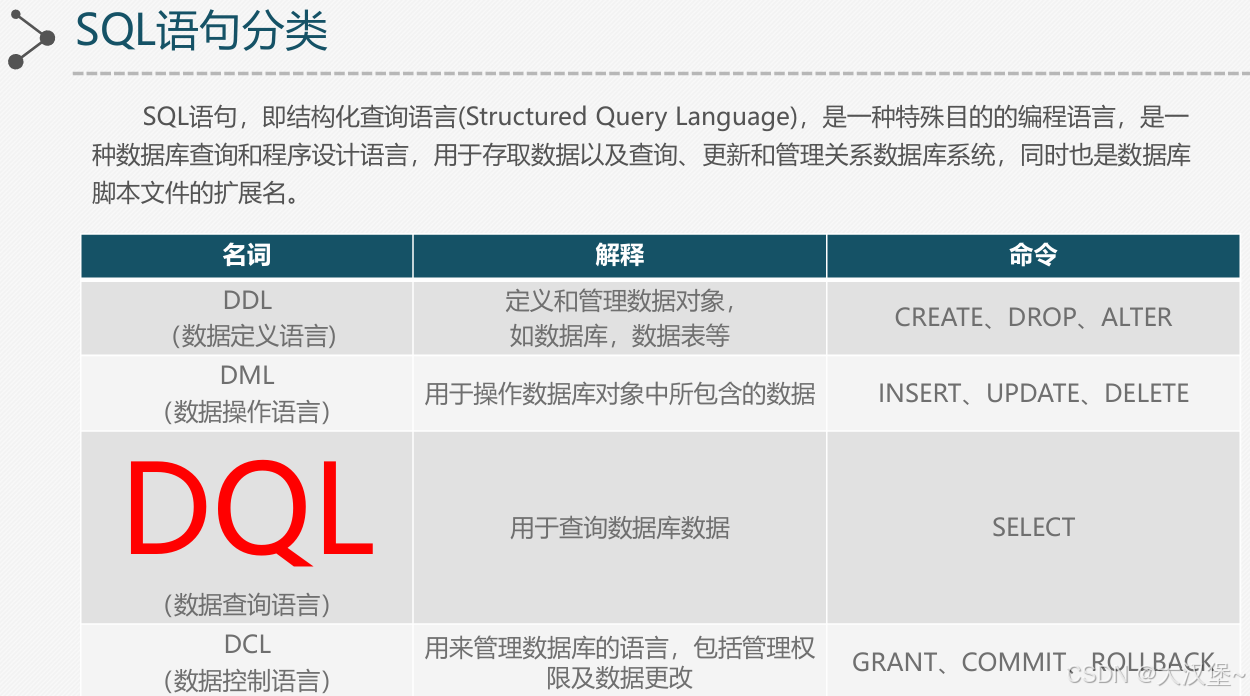
DDL建库建表
注释
-- 注释
/*
多行
注释
*/
1.sql不区分大小写
2._(下划线)进行名字的分割,不适用驼峰命名法
3.;语句sql结尾处写一个;来表示结束
4.一般关键词建议使用大写
5.所有名称不允许使用中文
#库
-- 查看所有的库
show databases;
-- 创建库
-- create database 数据库名;
-- 删除库(危险操作)
drop database 数据库名;
-- 使用库/切换库
use 数据库名;
#表的操作
-- 查看该库中所有的表
show tables;
-- 创建表
/*create table 表名(
字段名 类型 属性,
字段名 类型 属性,
...
字段名 类型 属性
);
*/
create table pet(
dog_name varchar(10),
dog_age tinyint,
dog_birth datetime,
dog_id char(3),
dog_hobby varchar(25),
dog_weight decimal(4,1)
);
-- ``反引号:取消关键性
-- DEFAULT NULL 该字段的值可以为空
CREATE TABLE `pet` (
`dog_name` varchar(10) DEFAULT NULL,
`dog_age` tinyint DEFAULT NULL,
`dog_birth` datetime DEFAULT NULL,
`dog_id` char(3) DEFAULT NULL,
`dog_hobby` varchar(25) DEFAULT NULL,
`dog_weight` decimal(4,1) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci
#默认字符集和字符排序
DEFAULT CHARSET=utf8mb4
COLLATE=utf8mb4_0900_ai_ci
ENGINE=InnoDB 非常重要 存储:写磁盘的规则innodb 引擎:规则
-- 数据库的本质就是把数据写到磁盘上
#查看表中的字段
#方式一 desc 表名
desc pet;
#方式二
show create table pet;
#查看当前数据库支持的存储引擎:
show engines;
-- 表结构的修改alter table 表名 关键词 数据;
-- 1.修改表名
alter table 旧表名 rename as 新表名;
-- 2.添加一个字段
alter table 表名 add 新字段名 类型 属性;
-- 3.删除字段(危险操作)
alter table 表名 drop 字段名;
-- 4.修改字段
-- 覆盖式修改
1.一定的默认值default null
2.如果有已经存在具体的数据(tinyint转int char转varchar)--数据是可以做隐式转换的
一旦出现不是字符的数据(字符串) 转int 这种转不了,直接报错
-- 方式一 modify 只能修改数据类型和属性
alter table 表名 modify 字段名 要修改的类型 要修改的属性;
-- 方式二 change 不仅修改类型和属性 还可以修改字段名
alter table 表名 change 旧字段名 新字段名 要修改的类型 要修改的属性;
(危险操作)
-- drop table 表名
# 约束
1.非空约束
create table tb1(
username varchar(10) not null, -- 非空约束
userage int
);
create table tb2(
username varchar(10) not null default '无名', -- 非空约束
userage int
);
2.唯一约束
是可以为null 并且可以有多个null,因为null 是一个类型
create table tb3(
username varchar(10) unique, -- 唯一约束 行级约束
userage int unique -- 每个字段都是各自的唯一约束
);
create table tb4(
username varchar(10),
userage int,
unique(username,userage) -- 表级约束, 联合唯一约束
-- 字段是有关联的,必须每个字段的值都相同时才会触发唯一约束
);
create table tb5(
username varchar(10),
userage int,
CONSTRAINT name_age_unique unique(username,userage)
-- 表级约束可以给约束起名字(方便以后通过这个名字来删除这个约束)
);
3.主键约束
- 每张表必须有且只有一个主键
- 主键的值是唯一
- 主键是不能为null
create table tb6(
username varchar(10) primary key,
userage int primary key -- 错误,主键在一张表中只能有一个
);
create table tb8(
username varchar(10),
userage int,
primary key(username,userage) -- 联合主键,联合约束
-- 多个字段的值,只有完全相同时,才会触发约束
);
#一般主键的建立方式
主键的值是不会回补
create table tb9(
tid int primary key auto_increment, -- 自增
username varchar(10),
userage int
);
4.外键约束
- 外键的值可以重复
- 外键可以为null
- 外键必须要写 父表中有的数据
- 父表中的关联字段必须是一个具有唯一性的数据
- 父表的字段名和从表的字段名不一定要相同,但是数据类型必须一致
- 一个表中可以有多个外键,也可以有多个外键约束,但是只能有一个主键
- 使用外键关系,不去建立外键约束
create table a(
aid int primary key auto_increment,
aname varchar(10)
);
create table b(
bid int primary key auto_increment,
bname varchar(10),
aid int,
FOREIGN key(aid) references a(aid)
);
约束的添加:
- 加非空约束
- alter table 表名 modify test_student char(10) not null;
- 添加唯一约束
- alter table 表名 add unique(表字段名,字段,字段,字段);
- 添加主键约束
- alter table 表名 add primary key(表的字段名,字段,字段);
- 添加外键约束
- alter table 表名 add constraint N1 foreign key (表字段名) references 父表(父表字段名);
约束的删除:
- 删除not null约束
- alter table 表名 modify 列名 类型;
- 删除unique约束
- alter table 表名 drop index 唯一约束名;
- 删除primary key约束
- alter table 表名 drop primary key;
- 删除foreign key约束
- alter table 表名 drop foreign key 外键名;
DML数据操作语句
#.新增
insert into 表名 (字段名,字段名,...,字段名)values/value(值,值,...,值)
-- 日期 使用字符串的形式进行书写日期格式 (yyyy-MM-dd HH-MM-SS)
#全字段的插入
--方式一
insert into student(sid,sname,birthday,ssex,classid) values(9,'张三','2007-1-1','男',1);
--方式二 1.null 2.default
insert into student values(null,'李四','2010-2-9','女',1);
insert into student values(default,'lili','2009-8-20','女',1);
#部分字段插入
insert into student(sname,ssex) values('zz','女');
alter table student modify ssex varchar(10) not null default '保密';
insert into student(sname) values('peter');
#一次性添加多条数据
-- 方式一
-- insert into 表名 (字段名..) values(值..),(值..)...
insert into student(sname,ssex) values('pony','女'),('ken','女'),('daven','女');
-- 方式二 不常用
-- insert into select
-- 插入和被插入的表都必须存在
create table newstu(
stuname varchar(10),
stusex varchar(10),
classid int
);
insert into newstu(stuname,stusex,classid) select sname,ssex,classid from student;
-- 方式三
-- create table select
-- 被插入表不能存在 --被插入表没有任何约束 仅仅是将查询结果放到新表
create table stu1 select sid,sname,birthday from student;
-- io高 既要输入又要输出 容易锁表
#.修改
update 表名 set 字段名=值,字段名=值,...,字段名=值 where 子句条件;
where子句 中的条件是对表中每一条数据进行判断,判断成立,该数据的父句执行;判断不成立,该数据的父句不执行
update stu1 set birthday='1999-10-22' where sname='peter';
update newstu set classid=200 where stusex!='男';
#.删除
delete from 表名 where 子句
delete from newstu;-- 删除表中数据
drop table newstu;-- 删除表
delete from stu1 where sname='daven';
-- 清空表(截断表)
truncate 表名 效果和删除表一样 删除表中数据
truncate stu1;
delete truncate drop的区别:
delete只删数据; truncate不仅删数据 还把索引删掉了
drop 不仅删数据,还删除了索引,表结构也删了
DQL语句
所有的查询都会得到一张虚拟表 不会发生变化 除非重新查
#查询
-- 最简单的查询
select 123;
select 'abc';
select 1+2;
-- 从表中获取数据
-- select 字段名,字段名 from 表名
-- 全字段查询
select sid,sname,birthday,ssex,classid from student;
select * from student;-- 效率比全字段查询慢 不推荐使用
-- 部分字段查询
select sname,ssex from student;
-- 字段名起别名
select sname as '姓名',birthday '生日',ssex 性别 from student;
-- 添加字段
select sname,'university' 学校 from student;
#distinct 去重
所有字段的数据要一致才会去重
select distinct sname,ssex from student;
#带条件的查询 where子句
select * from student where sid=5;
select * from student where sid<>5;
select * from student where sid between 3 and 5;
#面试题常考
-- 查询年龄大于1990-1-1的学生
select * from student where birthday<'1990-1-1';
#in 在某个特定的范围内
-- or会让索引失效
select * from student where sid=3 or sid=5 or sid=7;
-- 推荐使用in 可以使用到索引
select * from student where sid in (3,5,7);
#like 模糊查询
- 模糊符号
- % 任意多的任意字符
- _ 一个任意字符
select * from student where sname like '%杨%';
#null
-- is 是一个什么
select * from student where birthday is null;
select * from student where birthday is not null;
#聚合函数
-- 把多个值变为一个值
- count() 统计个数
- max() 求最大值
- min() 求最小值
- sum() 总和
- avg() 平均值
1.count
#select count(字段\常量\*) from 表名;
select count(sid) from student;-- 主键
select count(classid) from student;-- 不统计null类型
select count('a') from student;-- 不推荐使用
select count(123) from student;-- 推荐使用
select count(*) from student;-- 推荐使用
2.sum avg min max 数值类型
select sum(score) from sc;
select avg(score) from sc;
select max(score) from sc;
select min(score) from sc;
例:统计出成绩表中一共有多少次考试,总成绩,平均分,最高分,最低分
select count(*) 考试次数,sum(score) 总成绩,avg(score) 平均分,max(score) 最高分,min(score) 最低分 from sc;
#group by 分组
select ssex,count(*) from student group by ssex;
having和where的区别:
- where是对表中每一条数据进行判断
- having是对分组聚合后的数据进行条件筛选
#order by 排序
默认是按主键升序排序
#按班级降序desc必须声明 默认不写就是升序asc
select * from student order by classid desc;
#先写先排
select * from sc order by score desc,cid desc;
#limit 分页
0 开始 (页码-1)*步长,步长
#select * from 表名 limit 位置,步长;
select * from student limit 3,3;-- 从3开始