页面分类动画
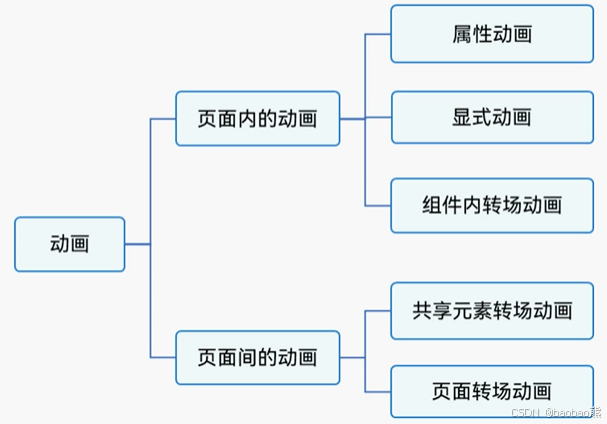
显示动画
typescript
function animateTo(value: AnimateParam, event: () => void): void;
代码如下:(实现属性变化引发的动画)
typescript
@Entry
@Component
struct Animate_Page1 {
@State boxWidth: number = 100;
@State boxHeight: number = 100;
@State flag: boolean = true;
build() {
Stack({ alignContent: Alignment.BottomEnd }) {
Column() {
Row() {
}
.width(this.boxWidth)
.height(this.boxHeight)
.backgroundColor(Color.Red)
}
.height('100%')
.width('100%')
.justifyContent(FlexAlign.Center)
Button("动画")
.width(50)
.height(50)
.margin(20)
.onClick(() => {
animateTo({
duration: 1000 //动画时间
}, () => {
if (this.flag) {
this.boxWidth = 240;
this.boxHeight = 240;
} else {
this.boxWidth = 100;
this.boxHeight = 100;
}
this.flag = !this.flag;
})
})
}
.width('100%')
}
}
常见属性
-
duration:动画执行时间
-
iterations:动画播放次数,-1表示无限次播放
-
curve:播放曲线
-
playMode:动画播放模式
-
delay:动画延迟播放时间
属性动画
使用animation属性去定义动画的属性,需要注意的点是,如果在animation方法后定义的属性,在改变时将不会触发动画。
typescript
@Entry
@Component
struct Animate_Page2 {
@State boxWidth: number = 100;
@State boxHeight: number = 100;
@State flag: boolean = true;
build() {
Stack({ alignContent: Alignment.BottomEnd }) {
Column() {
Row() {
}
.width(this.boxWidth)
.height(this.boxHeight)
.backgroundColor(Color.Red)
.animation({
duration: 1000
})
}
.height('100%')
.width('100%')
.justifyContent(FlexAlign.Center)
Button("动画")
.width(50)
.height(50)
.margin(20)
.onClick(() => {
if (this.flag) {
this.boxWidth = 240;
this.boxHeight = 240;
} else {
this.boxWidth = 100;
this.boxHeight = 100;
}
this.flag = !this.flag;
})
}
.width('100%')
}
}
常见属性
-
duration:动画执行时间
-
iterations:动画播放次数,-1表示无限次播放
-
curve:播放曲线
-
playMode:动画播放模式
-
delay:动画延迟播放时间
弹簧曲线动画(springCurve属性)
实现组件的左右抖动
typescript
import curves from '@ohos.curves';
@Entry
@Component
struct Animate_Page3 {
@State message: string = '登录框';
@State translateX: number = 0;
@State translateY: number = 0;
jumpWithSpeed(velocity: number) {
this.translateX = -10;
animateTo({
duration: 2000,
//速度(velocity)质量(mass)刚度(stiffness)阻尼(damping)
curve: curves.springCurve(velocity, 1, 1, 1)
}, () => {
this.translateX = 0;
})
}
build() {
Column() {
Row() {
Text(this.message).fontSize(40).width('100%').textAlign(TextAlign.Center)
}
.width(200)
.height(200)
.backgroundColor(Color.Gray)
.margin({
top: 20,
bottom: 50
})
.translate({
x: this.translateX,
y: this.translateY
})
Row() {
Button("jump10").width(100).onClick(() => {
this.jumpWithSpeed(10)
})
Button("jump200").width(100)
.onClick(() => {
this.jumpWithSpeed(200)
})
}.justifyContent(FlexAlign.SpaceAround)
.width('100%')
}
.height('100%')
.width('100%')
}
}
路径动画(motionPath属性)
需要在改变的外层轮廓添加animation属性,才能实现。需要注意点坐标需要乘2.
typescript
@Entry
@Component
struct Animate_Page4 {
@State message: string = 'Hello World';
@State flag: boolean = true;
build() {
Column() {
Row() {
Text(this.message).fontSize(20).width('100%').textAlign(TextAlign.Center)
}
.width(100)
.height(100)
.backgroundColor(Color.Gray)
.margin(10)
.onClick(() => {
this.flag = !this.flag;
})
.motionPath({
path: "M start.x start.y L10 400 L400 400 Lend.x end.y",
from: 0,
to: 1,
rotatable: false//控制组件是否沿运动方向旋转
})
}
.height('100%')
.width('100%')
.alignItems(this.flag ? HorizontalAlign.Start : HorizontalAlign.End)
.animation({
duration: 2000
})
}
}
页面间动画-放大缩小视图
关键属性:sharedTransition属性
sharedTransition属性中的type类型包含Exchange和Static两种,详细简介如下图:
实现两个页面之间的图片放大缩小的方式跳转
Animate_Page5:
typescript
import router from '@ohos.router';
@Entry
@Component
struct Animate_Page5 {
@State message: string = 'Hello World';
build() {
Column() {
Image($r('app.media.app_icon'))
.width(50)
.height(50)
.margin({
top: 30,
bottom: 30
})
.sharedTransition("icon1", {
duration: 1000,
type: SharedTransitionEffectType.Exchange
})
Button("点我跳转")
.width(200)
.fontSize(20)
.fontColor(Color.White)
.onClick((event: ClickEvent) => {
router.pushUrl({
url: "pages/Animate_Page5_Image"
})
})
}
.height('100%')
.width('100%')
}
}
Animate_Page5_Image:
typescript
import router from '@ohos.router';
@Entry
@Component
struct Animate_Page5_Image {
@State message: string = 'Hello World';
build() {
Column() {
Image($r('app.media.app_icon'))
.width(50)
.height(50)
.onClick(() => {
router.back();
})
.sharedTransition("icon1")
}
.height('100%')
.width('100%')
.justifyContent(FlexAlign.Center)
}
}
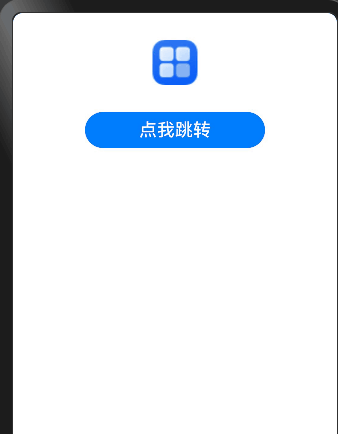
页面转场动画
可以设置转场动画方向和透明度等属性
typescript
pageTransition() {
//页面打开时候的动画
PageTransitionEnter({})
.onEnter((type: RouteType, progress: number) => {
})
//页面离开时候的动画
PageTransitionExit({})
.onExit((type: RouteType, progress: number) => {
})
}