一、创建项目
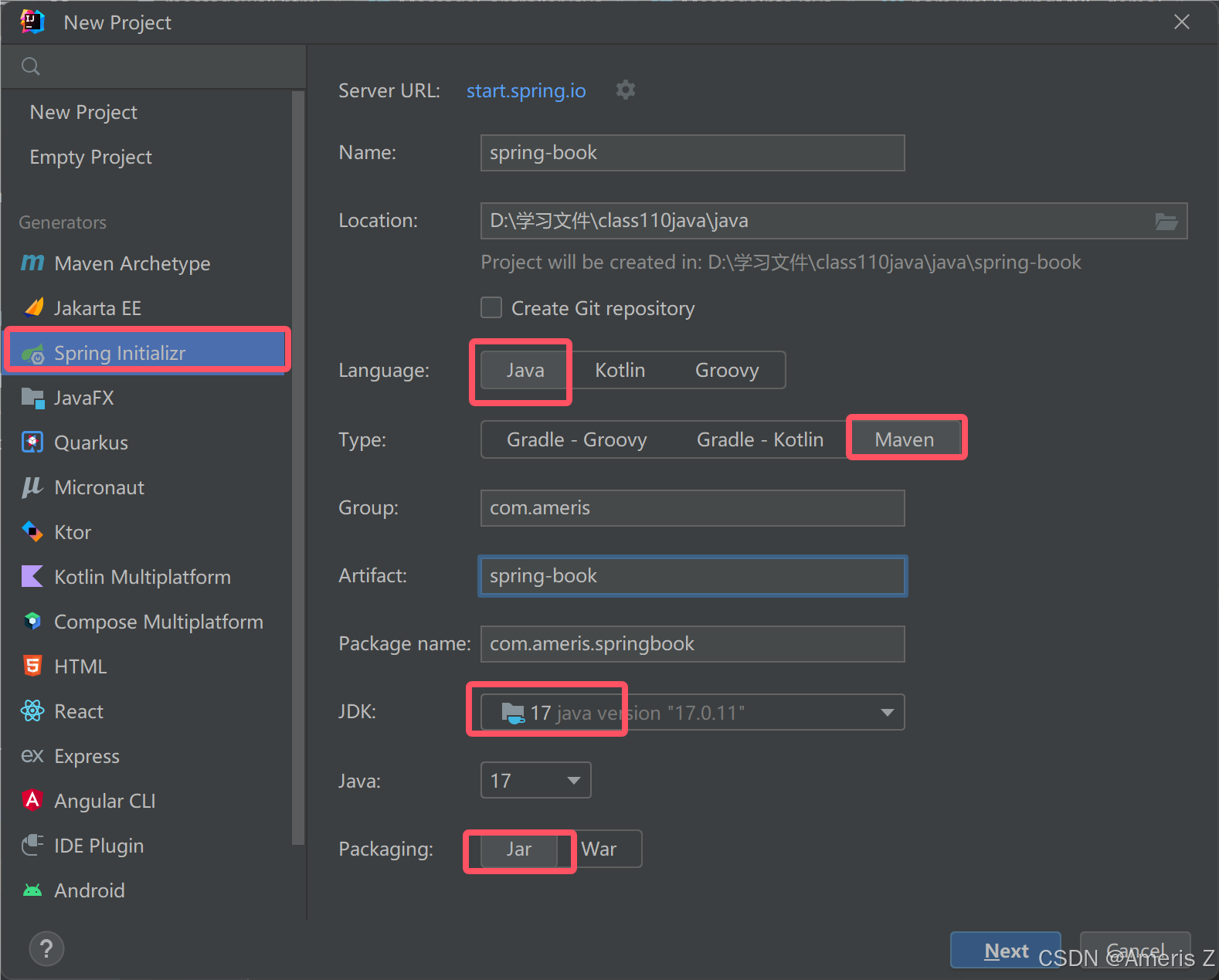
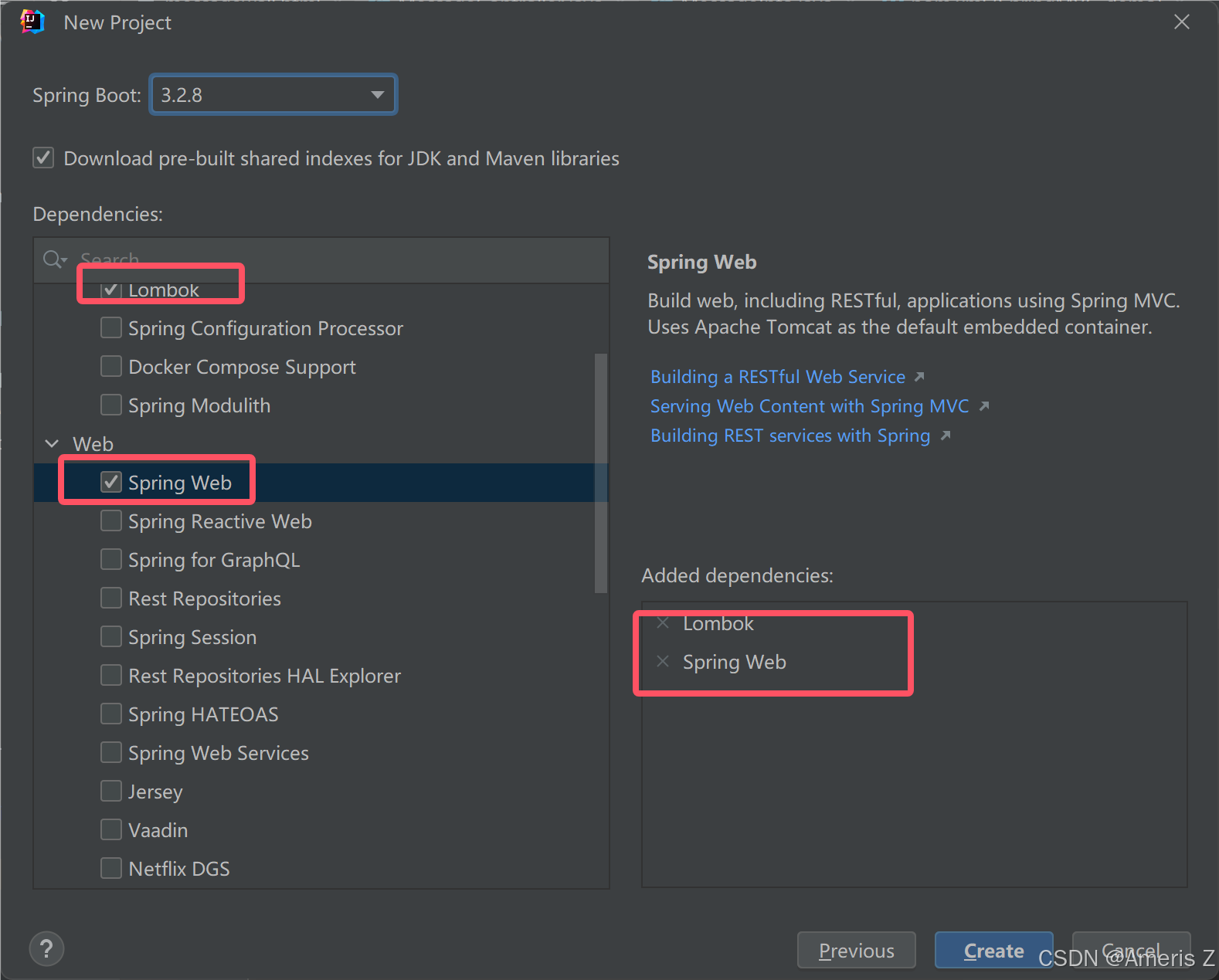
最后修改以下 spring 版本 为 2.7.17
Java 版本为 8
同时在
XML
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.17</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.ameris</groupId>
<artifactId>spring-book</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>spring-book</name>
<description>spring-book</description>
<url/>
<licenses>
<license/>
</licenses>
<developers>
<developer/>
</developers>
<scm>
<connection/>
<developerConnection/>
<tag/>
<url/>
</scm>
<properties>
<java.version>8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
二、前端代码
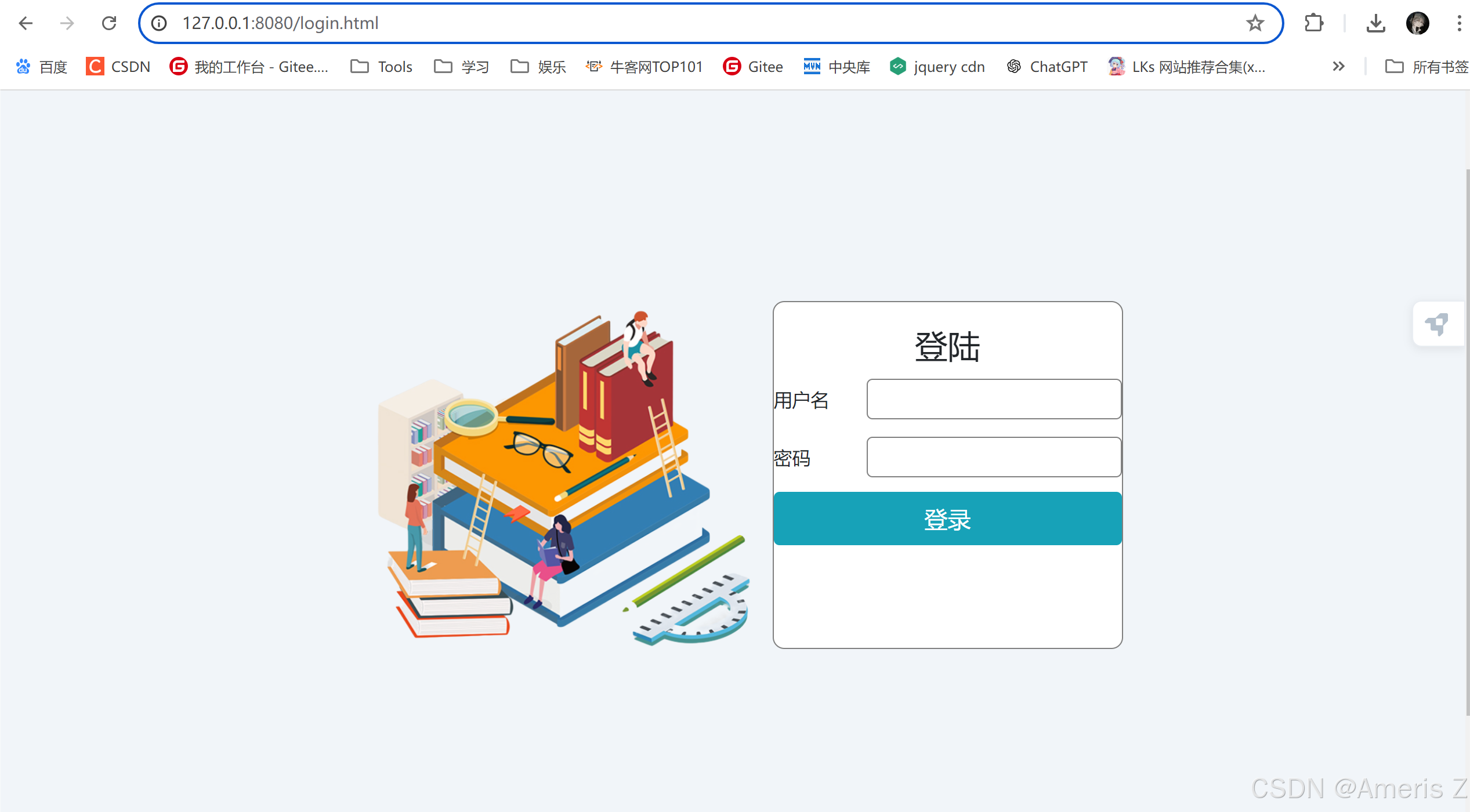
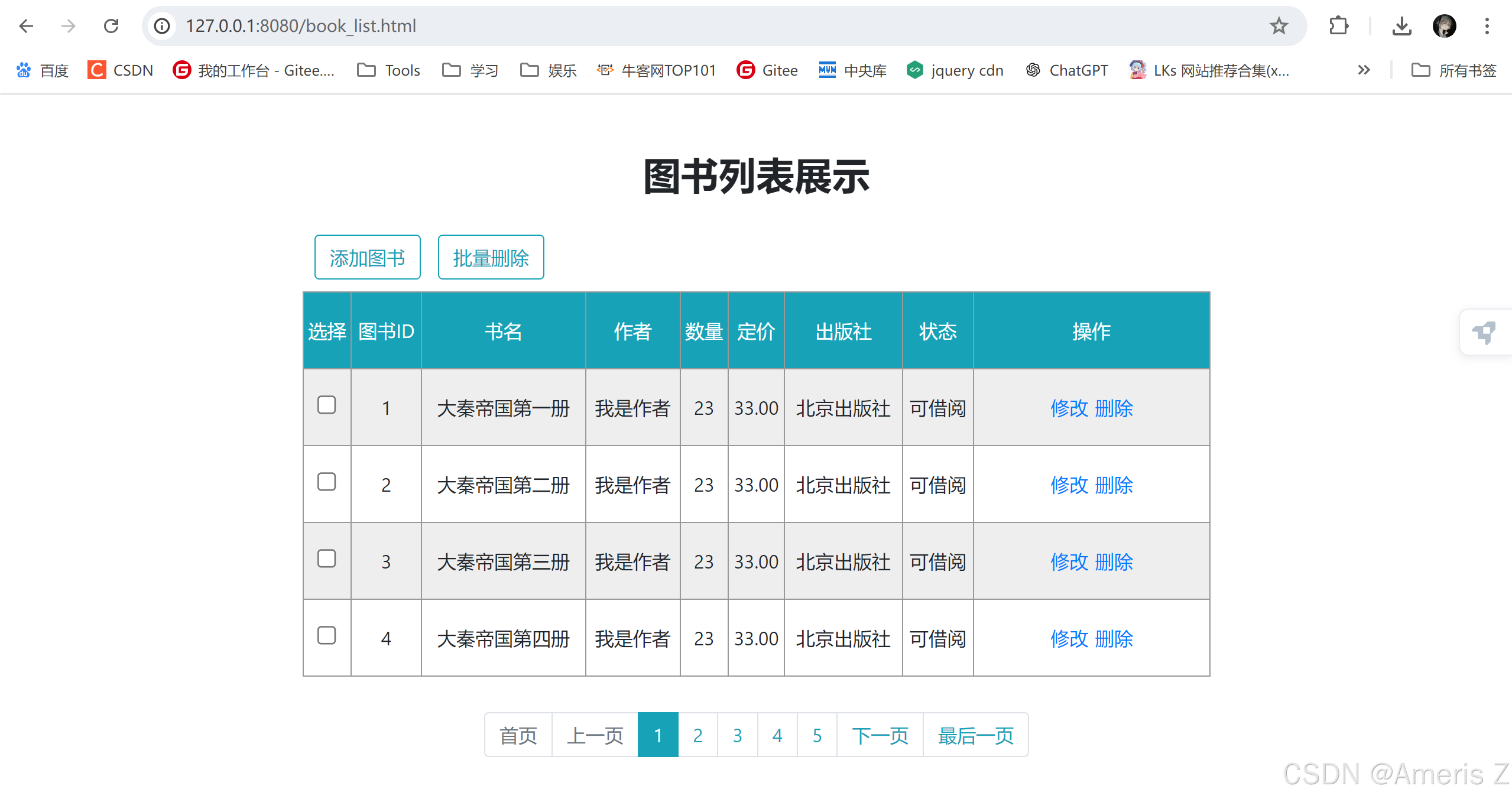
三、接口定义
客户端:
1.我需要什么服务
2.我能提供什么参数,是否有这个参数
3.对方提供给我的信息,是否满足我的需求
服务器:
1.提供什么服务
2.需要什么参数
3.处理之后,需要给客户端响应什么数据
3.1 登录
URL:/user/login
参数:
userName 用户名
password 密码
返回:
true:用户名和密码正确
false:用户名或密码错误
3.2 书籍列表
URL:/book/getList
参数:
无
返回:图书列表
List<BookInfo>
四、创建类
4.1 图书信息 BookInfo
id,书名,作者,数量,定价,出版社,状态
4.2 图书控制器 BookController
4.3 用户控制器 UserController
五、编写后端代码
5.1 userController
java
package com.ameris.springbook;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpSession;
@RestController
@RequestMapping("/user")
public class UserController {
@RequestMapping("/login")
public boolean login(String userName, String password, HttpSession session){
//校验参数
if(!StringUtils.hasLength(userName)||
"".equals(userName)||
!StringUtils.hasLength(password)||
"".equals(password)
){
return false;
}
//校验用户名密码是否正确
if("admin".equals(userName) && "admin".equals(password)){
//存session
session.setAttribute("userName",userName);
return true;
}
return false;
}
}
5.2 BookController
java
package com.ameris.springbook;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
@RestController
@RequestMapping("/book")
public class BookController {
@RequestMapping("/getList")
public List<BookInfo> getList(){
//1.从数据库获取 list
//采用Mock的方式(构造虚假的数据进行测试)
List<BookInfo> bookInfos = mockBookData();
//2.对数据进行处理(例如:对书记借阅状态进行转换)
for (BookInfo bookinfo:
bookInfos) {
if (bookinfo.getState() == 1){
bookinfo.setStateCN("可借阅");
} else if (bookinfo.getState() == 2) {
bookinfo.setStateCN("不可借阅");
}
}
//3.返回数据
return bookInfos;
}
/**
* 构造假的数据 进行测试
* @return
*/
public List<BookInfo> mockBookData(){
List<BookInfo> bookInfos = new ArrayList<>();
for (int i = 1; i <= 15; i++) {
BookInfo bookInfo = new BookInfo();
bookInfo.setId(i);
bookInfo.setBookName("图书"+i);
bookInfo.setAuthor("作者"+i);
bookInfo.setCount(i*15+3);
bookInfo.setPrice(new BigDecimal(new Random().nextInt(100)));
bookInfo.setPublish("出版社"+1);
bookInfo.setState(i%5==0?2:1);
bookInfos.add(bookInfo);//放入list
}
return bookInfos;
}
}
六、测试后端接口
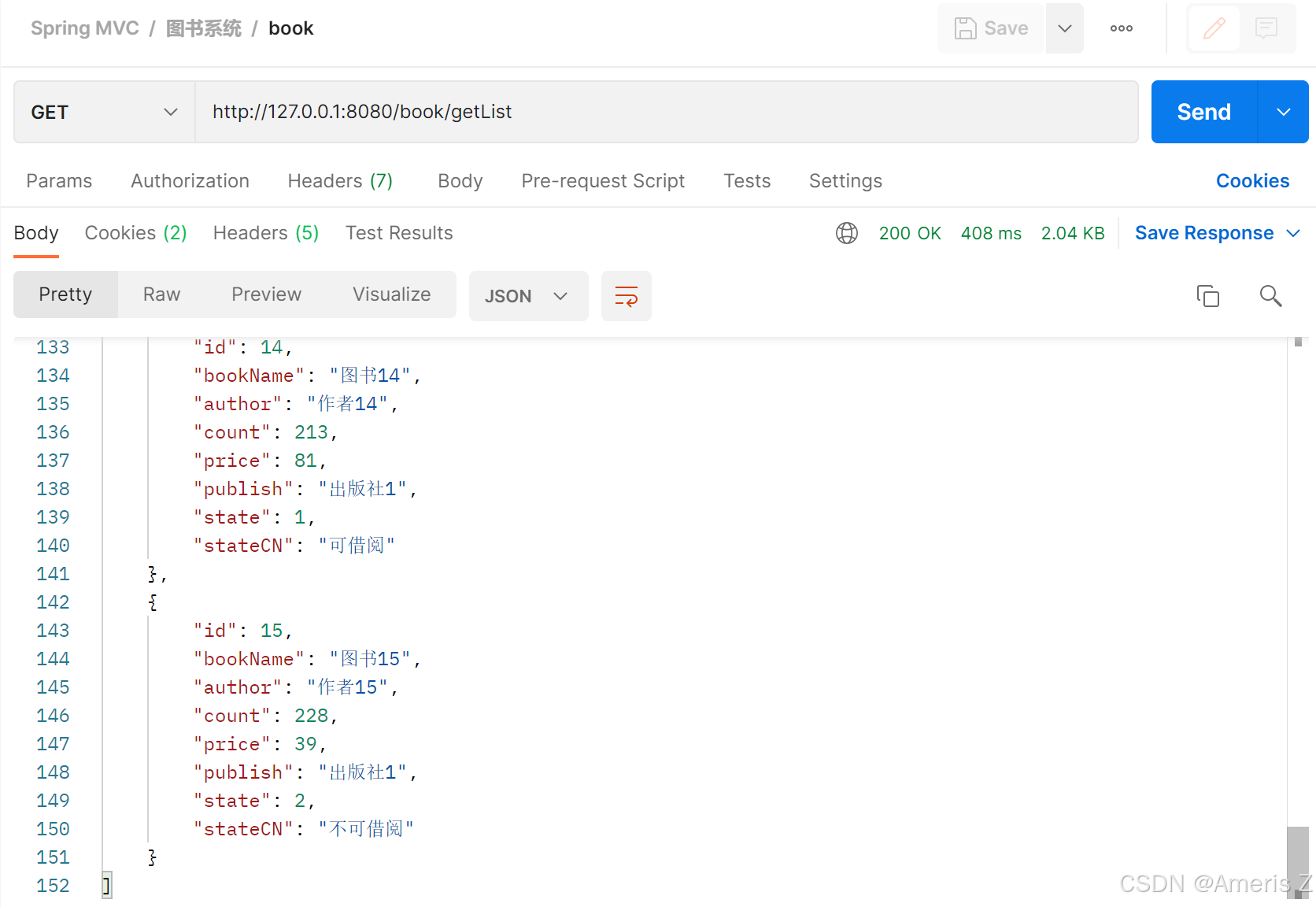
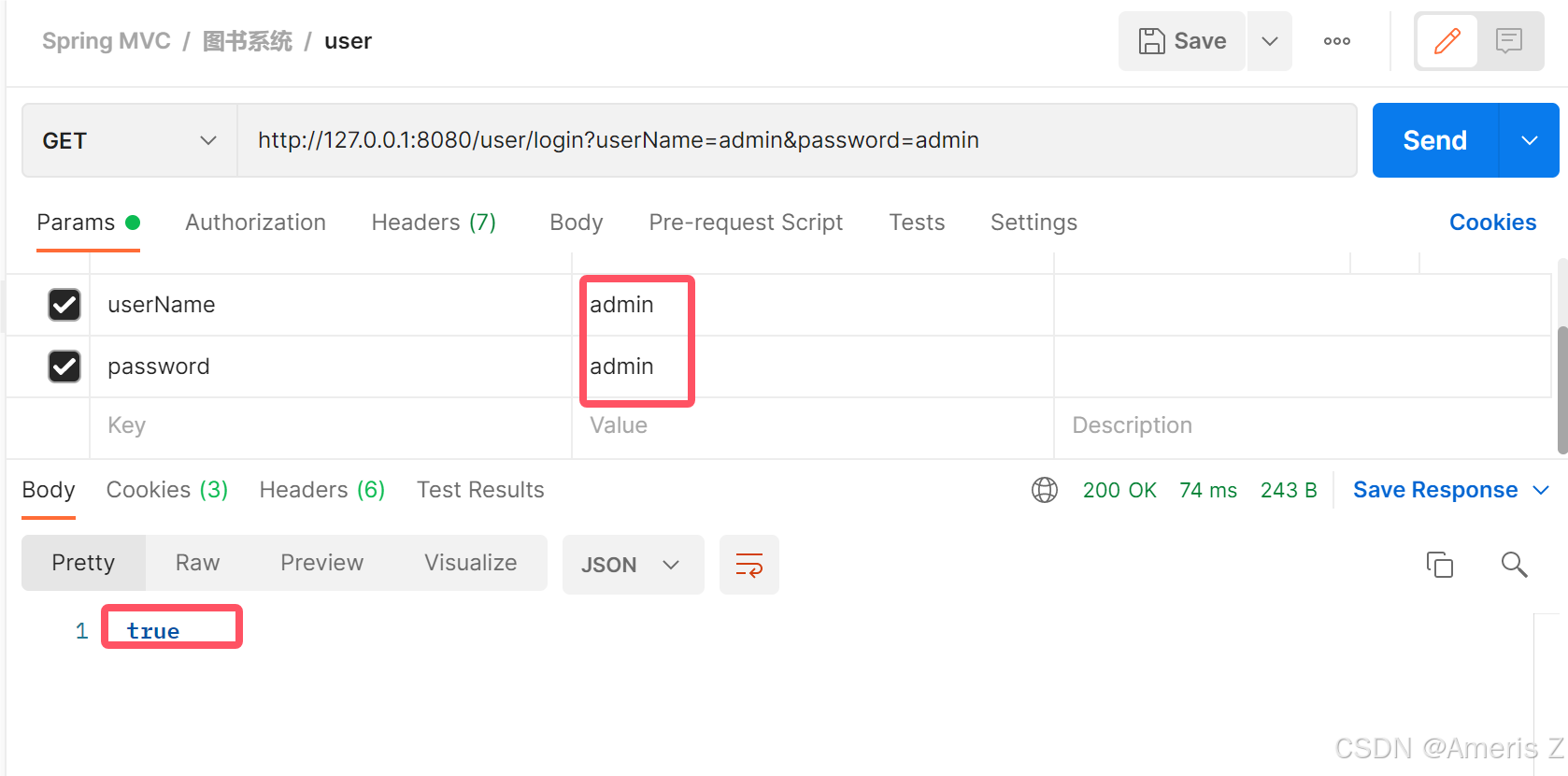
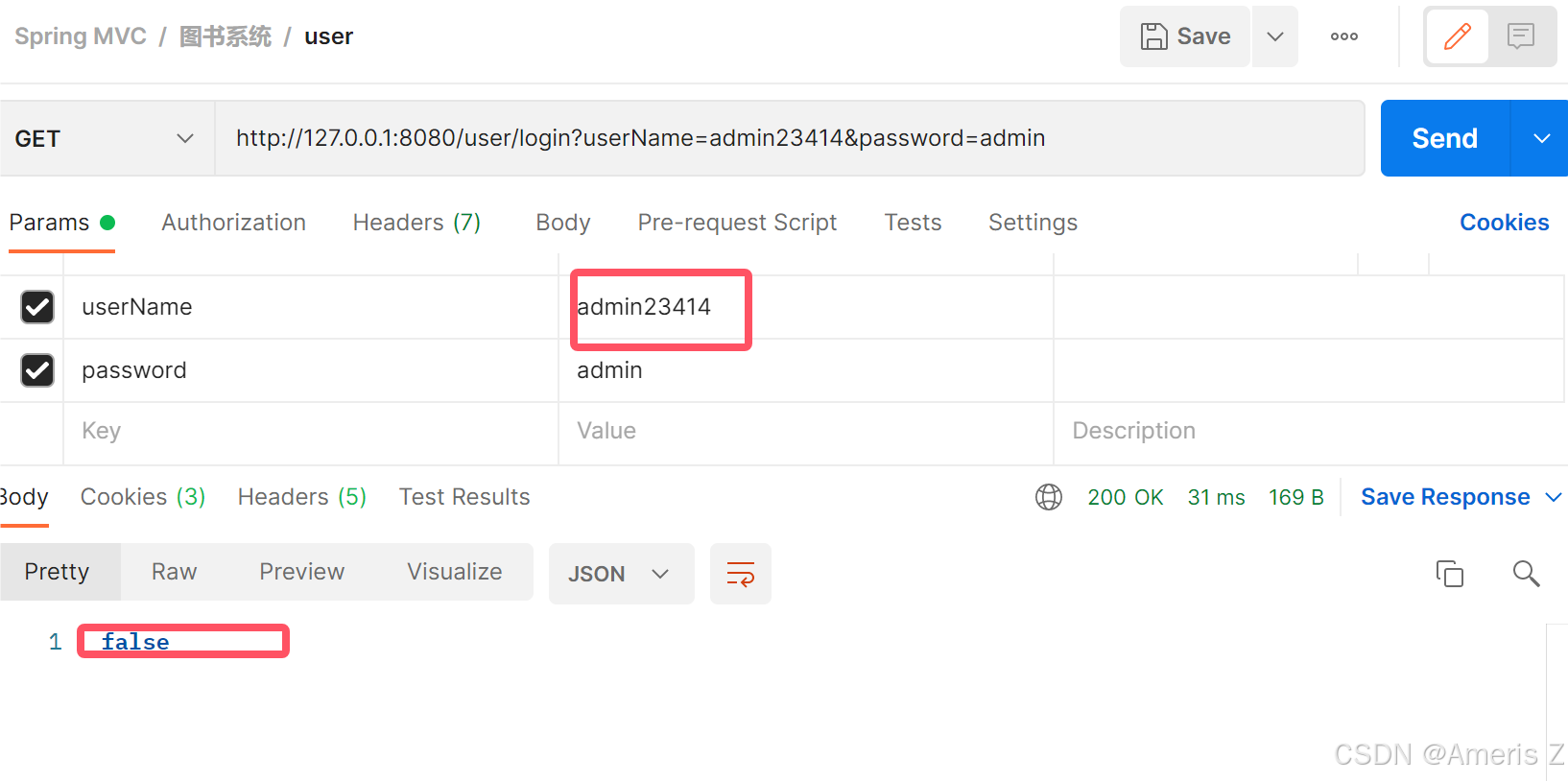
七、完成前端代码
7.1 login.html
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="css/bootstrap.min.css">
<link rel="stylesheet" href="css/login.css">
<script type="text/javascript" src="js/jquery.min.js"></script>
</head>
<body>
<div class="container-login">
<div class="container-pic">
<img src="pic/computer.png" width="350px">
</div>
<div class="login-dialog">
<h3>登陆</h3>
<div class="row">
<span>用户名</span>
<input type="text" name="userName" id="userName" class="form-control">
</div>
<div class="row">
<span>密码</span>
<input type="password" name="password" id="password" class="form-control">
</div>
<div class="row">
<button type="button" class="btn btn-info btn-lg" onclick="login()">登录</button>
</div>
</div>
</div>
<script src="js/jquery.min.js"></script>
<script>
function login() {
$.ajax({
type: "post",
url: "/user/login",
data: {
userName: $("#userName").val(),
password: $("#password").val()
},
success: function (result) {
if (result == true) {
//验证成功,跳转页面到图书列表页
location.href = "book_list.html";
} else {
alert("用户名或密码错误!");
}
}
});
}
</script>
</body>
</html>
7.2 book_list.html
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>图书列表展示</title>
<link rel="stylesheet" href="css/bootstrap.min.css">
<link rel="stylesheet" href="css/list.css">
<script type="text/javascript" src="js/jquery.min.js"></script>
<script type="text/javascript" src="js/bootstrap.min.js"></script>
<script src="js/jq-paginator.js"></script>
</head>
<body>
<div class="bookContainer">
<h2>图书列表展示</h2>
<div class="navbar-justify-between">
<div>
<button class="btn btn-outline-info" type="button" onclick="location.href='book_add.html'">添加图书</button>
<button class="btn btn-outline-info" type="button" onclick="batchDelete()">批量删除</button>
</div>
</div>
<table>
<thead>
<tr>
<td>选择</td>
<td class="width100">图书ID</td>
<td>书名</td>
<td>作者</td>
<td>数量</td>
<td>定价</td>
<td>出版社</td>
<td>状态</td>
<td class="width200">操作</td>
</tr>
</thead>
<tbody>
<tr>
<td><input type="checkbox" name="selectBook" value="1" id="selectBook" class="book-select"></td>
<td>1</td>
<td>大秦帝国第一册</td>
<td>我是作者</td>
<td>23</td>
<td>33.00</td>
<td>北京出版社</td>
<td>可借阅</td>
<td>
<div class="op">
<a href="book_update.html?bookId=1">修改</a>
<a href="javascript:void(0)" onclick="deleteBook(1)">删除</a>
</div>
</td>
</tr>
</tbody>
</table>
<div class="demo">
<ul id="pageContainer" class="pagination justify-content-center"></ul>
</div>
<script>
//已进入列表网页,就开始加载书籍列表
getBookList();
function getBookList() {
//发起ajax请求
$.ajax({
type: "get",
url: "/book/getList",
success: function (bookinfos) {
console.log(bookinfos);
var finalHtml = "";//创建一个标签
for (var book of bookinfos) {
//拼接html
//将book的数据拼接到 finalhtml里
finalHtml += '<tr>';
finalHtml += '<td><input type="checkbox" name="selectBook" value="' + book.id + '" id="selectBook" class="book-select"></td>';
finalHtml += '<td>' + book.id + '</td>';
finalHtml += '<td>' + book.bookName + '</td>';
finalHtml += '<td>' + book.author + '</td>';
finalHtml += '<td>' + book.count + '</td>';
finalHtml += '<td>' + book.price + '</td>';
finalHtml += '<td>' + book.publish + '</td>';
finalHtml += '<td>' + book.stateCN + '</td>';
finalHtml += '<td>';
finalHtml += '<div class="op">';
finalHtml += '<a href="book_update.html?bookId=' + book.id + '">修改</a>';
finalHtml += '<a href="javascript:void(0)" onclick="deleteBook(' + book.id + ')">删除</a>';
finalHtml += '</div>';
finalHtml += '</td>';
finalHtml += '</tr>';
//将这个finalhtml加紧 <tbody>里
$("tbody").html(finalHtml);
}
}
})
}
//翻页信息
$("#pageContainer").jqPaginator({
totalCounts: 100, //总记录数
pageSize: 10, //每页的个数
visiblePages: 5, //可视页数
currentPage: 1, //当前页码
first: '<li class="page-item"><a class="page-link">首页</a></li>',
prev: '<li class="page-item"><a class="page-link" href="javascript:void(0);">上一页<\/a><\/li>',
next: '<li class="page-item"><a class="page-link" href="javascript:void(0);">下一页<\/a><\/li>',
last: '<li class="page-item"><a class="page-link" href="javascript:void(0);">最后一页<\/a><\/li>',
page: '<li class="page-item"><a class="page-link" href="javascript:void(0);">{{page}}<\/a><\/li>',
//页面初始化和页码点击时都会执行
onPageChange: function (page, type) {
console.log("第" + page + "页, 类型:" + type);
}
});
function deleteBook(id) {
var isDelete = confirm("确认删除?");
if (isDelete) {
//删除图书
alert("删除成功");
}
}
function batchDelete() {
var isDelete = confirm("确认批量删除?");
if (isDelete) {
//获取复选框的id
var ids = [];
$("input:checkbox[name='selectBook']:checked").each(function () {
ids.push($(this).val());
});
console.log(ids);
alert("批量删除成功");
}
}
</script>
</div>
</body>
</html>
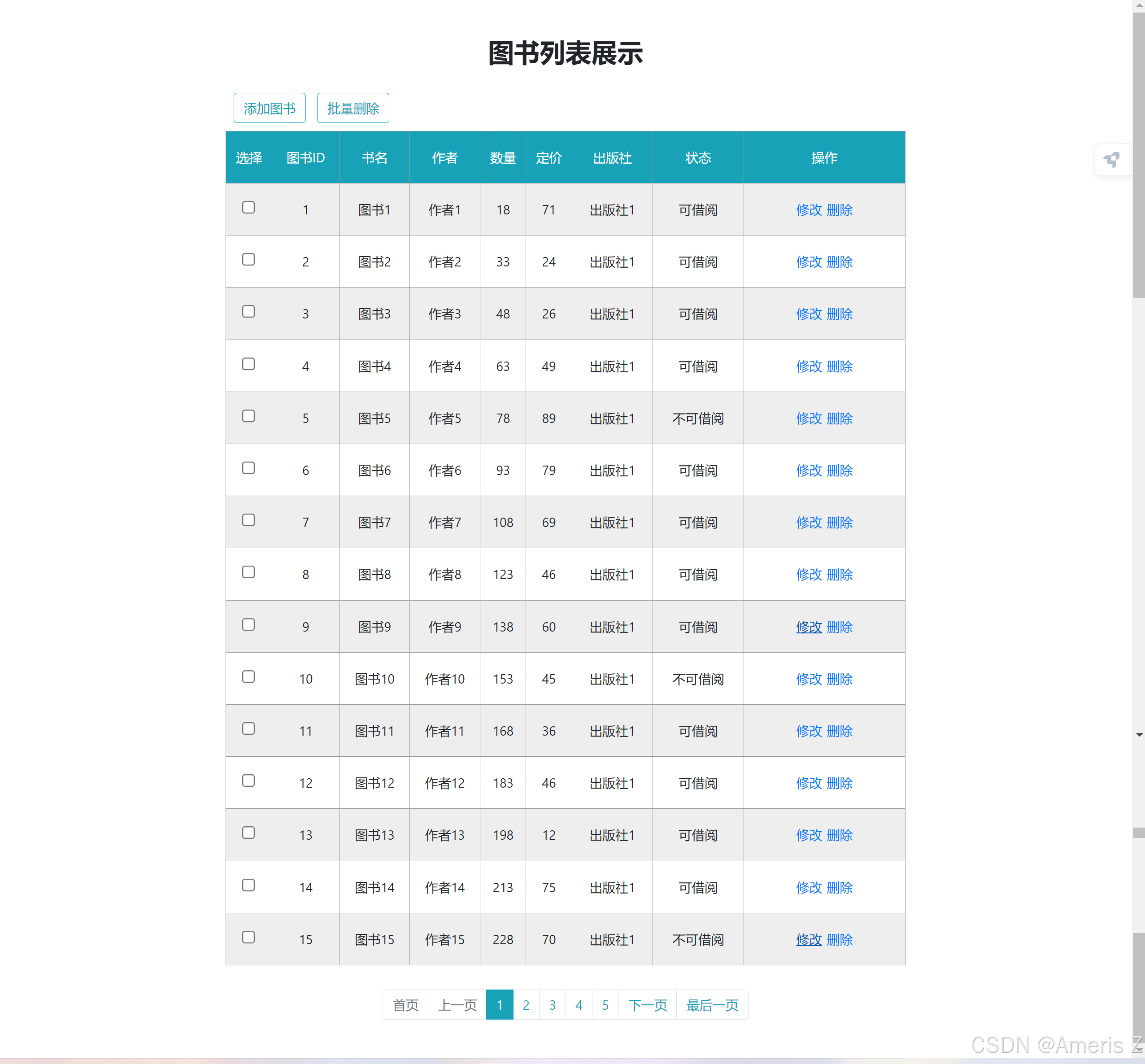
八、调试
如果遇到问题,打断点调试,shif+f9
然后发送请求,代码将会执行到断点的位置停下。
选中要分析的代码,右键 点击 evaluate,就可以分析了
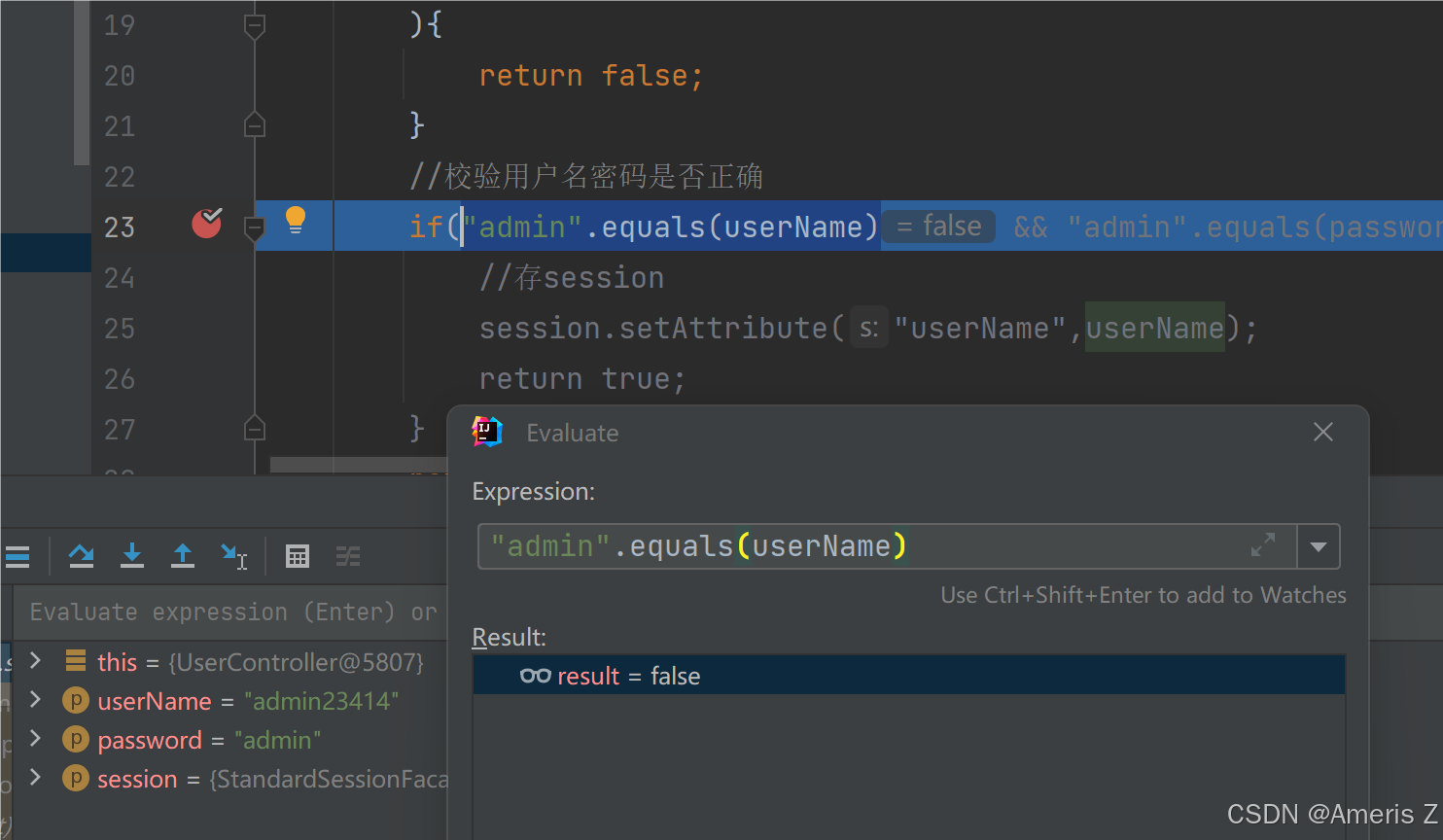