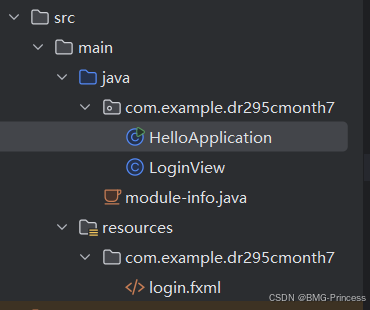
HelloApplication:
package com.example.dr295cmonth7; import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.stage.Stage; import java.io.IOException; public class HelloApplication extends Application { @Override public void start(Stage stage) throws IOException { FXMLLoader fxmlLoader = new FXMLLoader(HelloApplication.class.getResource("login.fxml")); Scene scene = new Scene(fxmlLoader.load(), 0, 0); stage.setTitle("DR295C数据采集传输仪"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(); } }
login.fxml:
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.layout.*?>
<?import javafx.scene.control.*?>
<GridPane xmlns:fx="http://javafx.com/fxml"
fx:controller="com.example.dr295cmonth7.LoginView" alignment="center" hgap="10" vgap="10">
<VBox alignment="CENTER" maxWidth="300.0" prefWidth="300.0" spacing="10.0">
<HBox alignment="CENTER" maxWidth="300.0" prefWidth="300.0" spacing="10.0">
<Label text="登录" GridPane.columnIndex="0" GridPane.rowIndex="0"/>
</HBox>
<HBox alignment="CENTER" maxWidth="300.0" prefWidth="300.0" spacing="10.0">
<Label text="账号:" GridPane.columnIndex="0" GridPane.rowIndex="0"/>
<TextField fx:id="usernameField" GridPane.columnIndex="1" GridPane.rowIndex="0"/>
</HBox>
<HBox alignment="CENTER" maxWidth="300.0" prefWidth="300.0" spacing="10.0">
<Label text="密码:" GridPane.columnIndex="0" GridPane.rowIndex="1"/>
<PasswordField fx:id="passwordField" GridPane.columnIndex="1" GridPane.rowIndex="1"/>
</HBox>
<Label fx:id="welcomeText" alignment="CENTER" maxWidth="1.7976931348623157E308"
style="-fx-text-fill: red;"/>
<Button text="登录" onAction="#handleLogin" GridPane.columnIndex="1" GridPane.rowIndex="2"/>
</VBox>
</GridPane>
handleLogin.java:
package com.example.dr295cmonth7;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.control.Alert;
import javafx.scene.control.Label;
import javafx.scene.control.PasswordField;
import javafx.scene.control.TextField;
public class LoginView {
@FXML
private TextField usernameField;
@FXML
private PasswordField passwordField;
@FXML
private Label welcomeText;
@FXML
void handleLogin(ActionEvent event) {
String username = usernameField.getText();
String password = passwordField.getText();
String emailPattern = "^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$";
if (username.isEmpty() || password.length() < 6||!username.matches(emailPattern)) {
Alert alert = new Alert(Alert.AlertType.ERROR);
alert.setTitle("错误");
if (username.isEmpty()) {
alert.setHeaderText("账号不能为空");
} else if (password.isEmpty()) {
alert.setHeaderText("密码不能为空");
} else {
alert.setHeaderText("账号格式不正确,应为有效的邮箱地址");
}
alert.showAndWait();
return;
}
// 假设正确的账号为 "admin",密码为 "123456"
if (!"admin".equals(username) ||!"123456".equals(password)) {
Alert alert = new Alert(Alert.AlertType.ERROR);
alert.setTitle("提示");
alert.setHeaderText("账号或密码错误");
alert.showAndWait();
welcomeText.setText("登录失败");
return;
}
// 登录成功的处理
System.out.println("登录成功");
}
}