这里我们使用一个广泛使用且免费处理 .docx
文件的库,github.com/nguyenthenguyen/docx
.
安装 github.com/nguyenthenguyen/docx
库
首先,确保你已经安装了 docx
库:
sh
go get github.com/nguyenthenguyen/docx
使用 docx
库处理 Word 模板
以下是如何实现的完整示例:
示例模板内容
假设我们的模板 template.docx
的内容如下:
plaintext
张三:{{TASK_NAME}}
李四:{{DETAILS}}
实现代码逻辑
go
package main
import (
"fmt"
"log"
"strings"
"github.com/nguyenthenguyen/docx"
)
func replacePlaceholder(text, placeholder, value string) string {
return strings.ReplaceAll(text, "{{"+placeholder+"}}", value)
}
func main() {
// 打开 Word 模板
r, err := docx.ReadDocxFile("/Users/songfayuan/GolandProjects/dsms-admin/template/template.docx")
if err != nil {
log.Fatalf("failed to open word template: %v", err)
}
doc := r.Editable()
// 填充数据
taskName := "Sample Task"
details := "This is a sample task detail."
content := doc.GetContent()
content = replacePlaceholder(content, "TASK_NAME", taskName)
content = replacePlaceholder(content, "DETAILS", details)
doc.SetContent(content)
// 保存为新文件
outputFilePath := "/Users/songfayuan/GolandProjects/dsms-admin/template/filled_template.docx"
err = doc.WriteToFile(outputFilePath)
if err != nil {
log.Fatalf("failed to save word document: %v", err)
}
fmt.Printf("Document saved to %s\n", outputFilePath)
}
结果
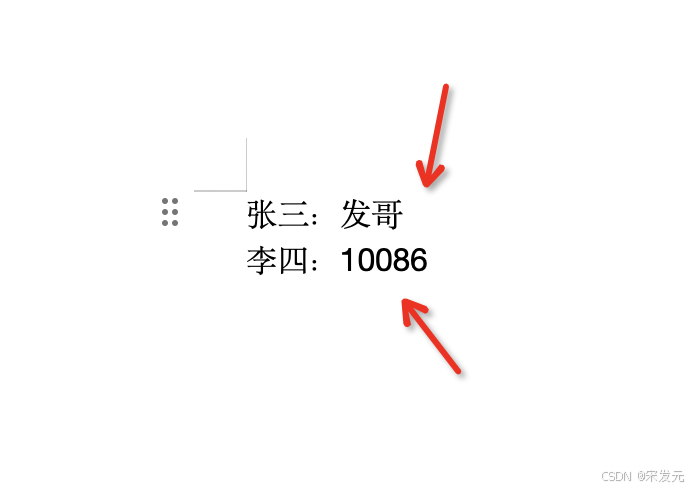