例如字符串数组:
cs
string[] arr = { "1","3-4-5-6-7", "2","3-4","3-4-5","3-4-5-6", "3", "6", "4", "6-1", "6-2", "5", "6-1-1","1-1","2-1", "1-2-2", "1-1-2", "2-2","1-1-1" };
目的想要树型结构化:
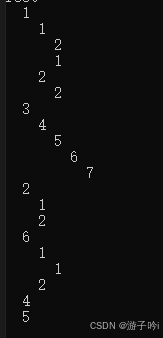
下面开始实现:
先定义一个TreeNode类
cs
public class TreeNode
{
public string Value { get; set; }
public List<TreeNode> Children { get; set; }
public TreeNode(string value)
{
Value = value;
Children = new List<TreeNode>();
}
}
具体实现
cs
TreeNode root = new TreeNode("root");
Dictionary<string, TreeNode> nodesMap = new Dictionary<string, TreeNode>();
foreach (var item in arr)
{
if (item.Contains("-"))
{
// 如果元素包含"-",则拆分并构建层级关系
string[] parts = item.Split('-');
TreeNode currentNode = root;
string parentPath = "";
for (int i = 0; i < parts.Length; i++)
{
string part = parts[i];
string fullPath = parentPath + (string.IsNullOrEmpty(parentPath) ? "" : "-") + part;
if (!nodesMap.TryGetValue(fullPath, out TreeNode childNode))
{
childNode = new TreeNode(part);
if (currentNode.Children == null)
currentNode.Children = new List<TreeNode>();
currentNode.Children.Add(childNode);
nodesMap[fullPath] = childNode;
}
currentNode = childNode;
parentPath = fullPath;
}
}
else
{
// 如果元素不包含"-",则添加为根节点的子节点
if (!nodesMap.ContainsKey(item))
{
TreeNode node = new TreeNode(item);
root.Children.Add(node);
nodesMap[item] = node;
}
}
}
打印:
cs
public static void PrintTree(TreeNode node, int level)
{
Console.WriteLine(new string(' ', level * 2) + node.Value);
foreach (var child in node.Children)
{
PrintTree(child, level + 1);
}
}
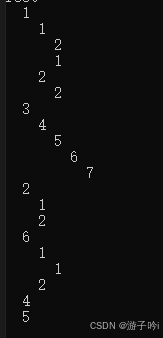