Prism视图传参方式。
实际应用场景
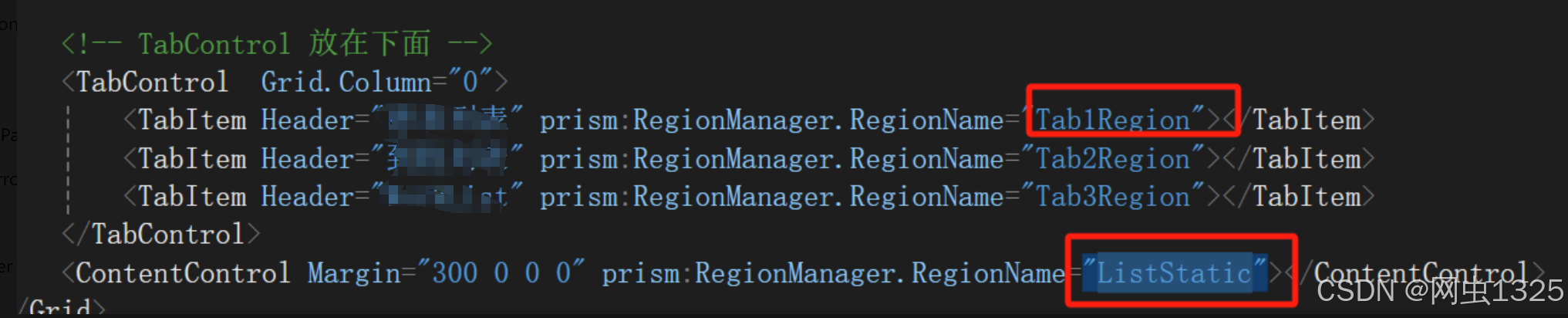
点击tabitem中的列表数据,同步更新到ListStatic Region对应的界面。目前用两种方式实现了传参数据同步。
第一,事件聚合器(EventAggregator)
1. 定义事件
创建一个事件类,用于传递数据。
cs
using Prism.Events;
public class DataUpdateEvent : PubSubEvent<string>
{
}
点击tabitem中的列表 ,示例传参数据是string类型,什么参数类型都可以。
2. 注册事件聚合器
在 App.xaml.cs
中注册事件聚合器。
cs
protected override void RegisterTypes(IContainerRegistry containerRegistry)
{
containerRegistry.RegisterSingleton<IEventAggregator, EventAggregator>();
containerRegistry.RegisterForNavigation<Tab1View, Tab1ViewModel>();
containerRegistry.RegisterForNavigation<ListStaticView, ListStaticViewModel>();
}
3. 定义视图和视图模型
Tab1View.xaml
cs
<UserControl x:Class="YourNamespace.Tab1View"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:i="http://schemas.microsoft.com/expression/2010/interactivity"
xmlns:prism="http://prismlibrary.com/">
<Grid>
<DataGrid ItemsSource="{Binding DataList}" SelectedItem="{Binding SelectedItem}">
<i:Interaction.Triggers>
<i:EventTrigger EventName="SelectionChanged">
<prism:InvokeCommandAction Command="{Binding ItemSelectedCommand}" CommandParameter="{Binding SelectedItem, RelativeSource={RelativeSource AncestorType=DataGrid}}"/>
</i:EventTrigger>
</i:Interaction.Triggers>
</DataGrid>
</Grid>
</UserControl>
Tab1ViewModel.cs
cs
using Prism.Commands;
using Prism.Mvvm;
using Prism.Events;
using System.Collections.ObjectModel;
public class Tab1ViewModel : BindableBase
{
private readonly IEventAggregator _eventAggregator;
private string _selectedItem;
public ObservableCollection<string> DataList { get; private set; }
public string SelectedItem
{
get { return _selectedItem; }
set { SetProperty(ref _selectedItem, value); }
}
public DelegateCommand<string> ItemSelectedCommand { get; private set; }
public Tab1ViewModel(IEventAggregator eventAggregator)
{
_eventAggregator = eventAggregator;
DataList = new ObservableCollection<string> { "Item 1", "Item 2", "Item 3" };
ItemSelectedCommand = new DelegateCommand<string>(OnItemSelected);
}
private void OnItemSelected(string selectedItem)
{
if (selectedItem != null)
{
_eventAggregator.GetEvent<DataUpdateEvent>().Publish(selectedItem);
}
}
}
ListStaticView.xaml
cs
<UserControl x:Class="YourNamespace.ListStaticView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<Grid>
<TextBlock Text="{Binding UpdatedData}" />
</Grid>
</UserControl>
ListStaticViewModel.cs
cs
using Prism.Mvvm;
using Prism.Events;
public class ListStaticViewModel : BindableBase
{
private readonly IEventAggregator _eventAggregator;
private string _updatedData;
public string UpdatedData
{
get { return _updatedData; }
set { SetProperty(ref _updatedData, value); }
}
public ListStaticViewModel(IEventAggregator eventAggregator)
{
_eventAggregator = eventAggregator;
_eventAggregator.GetEvent<DataUpdateEvent>().Subscribe(OnDataUpdate);
}
private void OnDataUpdate(string updatedData)
{
UpdatedData = updatedData;
}
}
4. 定义主窗口布局
MainWindow.xaml
cs
<Window x:Class="YourNamespace.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:prism="http://prismlibrary.com/">
<Grid>
<TabControl Grid.Column="0">
<TabItem Header="患者列表" prism:RegionManager.RegionName="Tab1Region"/>
<TabItem Header="到检列表" prism:RegionManager.RegionName="Tab2Region"/>
<TabItem Header="WorkList" prism:RegionManager.RegionName="Tab3Region"/>
</TabControl>
<ContentControl Margin="300 0 0 0" prism:RegionManager.RegionName="ListStatic"/>
</Grid>
</Window>
5. 配置导航
确保在应用启动时正确导航到初始视图。
MainWindowViewModel.cs
cs
using Prism.Mvvm;
using Prism.Regions;
public class MainWindowViewModel : BindableBase
{
private readonly IRegionManager _regionManager;
public MainWindowViewModel(IRegionManager regionManager)
{
_regionManager = regionManager;
_regionManager.RegisterViewWithRegion("Tab1Region", typeof(Tab1View));
_regionManager.RegisterViewWithRegion("ListStatic", typeof(ListStaticView));
}
}
第二,使用共享服务
使用共享服务可以在视图之间共享数据,并在一个视图中更新数据时通知另一个视图进行更新。
1. 定义共享服务
cs
public interface ISharedDataService
{
string SharedData { get; set; }
event Action<string> DataChanged;
void UpdateData(string data);
}
public class SharedDataService : ISharedDataService
{
private string _sharedData;
public string SharedData
{
get => _sharedData;
set
{
_sharedData = value;
DataChanged?.Invoke(_sharedData);
}
}
public event Action<string> DataChanged;
public void UpdateData(string data)
{
SharedData = data;
}
}
2. 注册服务
在 App.xaml.cs
中注册服务。
cs
protected override void RegisterTypes(IContainerRegistry containerRegistry)
{
containerRegistry.RegisterSingleton<ISharedDataService, SharedDataService>();
containerRegistry.RegisterForNavigation<Tab1View, Tab1ViewModel>();
containerRegistry.RegisterForNavigation<ListStaticView, ListStaticViewModel>();
}
3. 使用共享服务
Tab1ViewModel.cs
cs
using Prism.Commands;
using Prism.Mvvm;
using System.Collections.ObjectModel;
public class Tab1ViewModel : BindableBase
{
private readonly ISharedDataService _sharedDataService;
private string _selectedItem;
public ObservableCollection<string> DataList { get; private set; }
public string SelectedItem
{
get { return _selectedItem; }
set { SetProperty(ref _selectedItem, value); }
}
public DelegateCommand<string> ItemSelectedCommand { get; private set; }
public Tab1ViewModel(ISharedDataService sharedDataService)
{
_sharedDataService = sharedDataService;
DataList = new ObservableCollection<string> { "Item 1", "Item 2", "Item 3" };
ItemSelectedCommand = new DelegateCommand<string>(OnItemSelected);
}
private void OnItemSelected(string selectedItem)
{
if (selectedItem != null)
{
_sharedDataService.UpdateData(selectedItem);
}
}
}
ListStaticViewModel.cs
cs
using Prism.Mvvm;
public class ListStaticViewModel : BindableBase
{
private readonly ISharedDataService _sharedDataService;
private string _updatedData;
public string UpdatedData
{
get { return _updatedData; }
set { SetProperty(ref _updatedData, value); }
}
public ListStaticViewModel(ISharedDataService sharedDataService)
{
_sharedDataService = sharedDataService;
_sharedDataService.DataChanged += OnDataChanged;
}
private void OnDataChanged(string updatedData)
{
UpdatedData = updatedData;
}
}
以上两种方法都可以实现从 Tab1Region
中的列表数据同步更新到 ListStatic
区域。