1、Telnetlib模块:
支持telnet/ssh远程访问的模块很多,常见的有telnetlib、ciscolib、paramiko、netmiko、pexpect,其中telnetlib和ciscolib对应telnet协议,后面3个对应SSH协议。
①-通过ENSP环境搭建实验环境
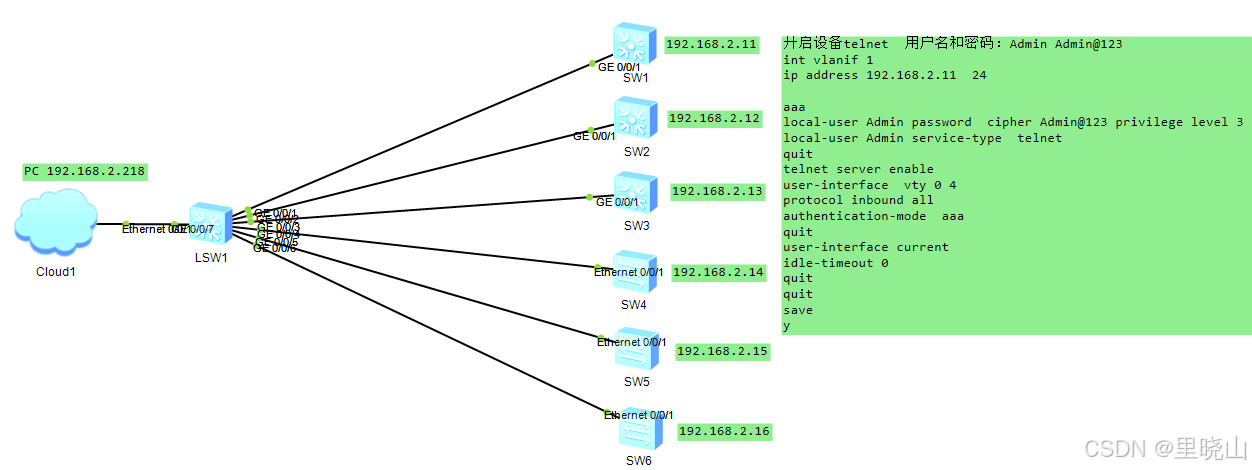
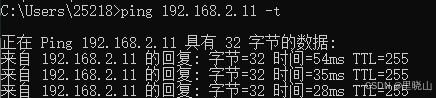
②-基础语法-telnetlib案例1:
注意:python3中,telnetlib模块下所有的返回值都是字节流,而默认输入的都是普通字符串str,因此在python3中使用tlenetlib需要注意:
①在字符串的前面需要加上一个b; 转换成字节流
②在变量和tlentlib函数后面需要加上.encode('ascii')函数 进行解码
③在read_all()函数后面需要加上decode('ascii')函数
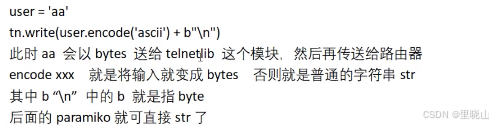
python
import telnetlib
import time
host = '192.168.2.11'
user = '111'
password = '111'
#执行telnet 并输入用户名和密码
tn = telnetlib.Telnet(host,port=23,timeout=1) #tn实例化函数 telnet设备ip地址,(端口号,超时时间也可以不写,默认即可)
tn.read_until(b"Username",timeout=1) #read函数 读取设备返回的Username字符串
tn.write(user.encode('ascii') + b'\n') #将用户名转为ascii编码方式将字符串转换为bytes \n回车
tn.read_until(b"Password:",timeout=1)
tn.write(password.encode('ascii') + b'\n')
print('已登录进设备'+ host)
tn.write(b"sys\n") #写入命令
tn.write(b"int loopback 1\n")
tn.write(b"ip address 1.1.1.1 32\n")
tn.write(b"quit\n")
tn.write(b"quit\n")
tn.write(b"save\n")
tn.write(b"y\n")
time.sleep(2) #时间模块,暂停2s 等待设备响应
output = (tn.read_very_eager().decode("ascii")) #输出结果区域
print(output)
tn.close()
③-登录多台设备(for 循环)-telnetlib案例2:
通过列表添加设备ip,然后for循环调用执行
python
import telnetlib
import time
hosts =['192.168.2.11','192.168.2.12','192.168.2.13','192.168.2.14','192.168.2.15','192.168.2.16']
user = '111'
password = '111'
for ip in hosts: #循环执行hosts列表
tn = telnetlib.Telnet(ip) #tn实例化函数 telnet设备ip地址,(端口号,超时时间也可以不写,默认即可)
tn.read_until(b"Username:") #read函数 读取设备返回的Username字符串
tn.write(user.encode('ascii') + b'\n') #将用户名转为ascii编码方式将字符串转换为bytes \n回车
tn.read_until(b"Password:",timeout=1)
tn.write(password.encode('ascii') + b'\n')
print('已登录进设备'+ ip)
tn.write(b"sys\n") #写入命令
tn.write(b"int loopback 1\n")
tn.write(b"ip address 1.1.1.1 32\n")
tn.write(b"quit\n")
tn.write(b"quit\n")
tn.write(b"save\n")
tn.write(b"y\n")
time.sleep(2) #时间模块,暂停2s 等待设备响应
output = (tn.read_very_eager().decode("ascii")) #输出结果区域 转为ascii编码方式将字符串转换为bytes
print(output)
tn.close()
④-循环执行多条命令-telnetlib案例3:
定义主机ip列表
定义命令字符串列表
然后for循环执行以上列表
python
import telnetlib
import time
hosts =['192.168.2.11','192.168.2.12','192.168.2.13','192.168.2.14','192.168.2.15','192.168.2.16'] #s表示列表
user = '111'
password = '111'
commands = ['sys','int loopback 1','ip address 1.1.1.1 32','quit','quit','save','y'] #相当于把命令刷到文档里,s表示列表
for ip in hosts:
tn = telnetlib.Telnet(ip) #tn实例化函数 telnet设备ip地址,(端口号,超时时间也可以不写,默认即可)
tn.read_until(b"Username:") #read函数 读取设备返回的Username字符串
tn.write(user.encode('ascii') + b'\n') #将用户名转为ascii编码方式将字符串转换为bytes \n回车
tn.read_until(b"Password:",timeout=1)
tn.write(password.encode('ascii') + b'\n')
print('已登录进设备'+ ip)
for command in commands:
tn.write(command.encode('ascii')+b'\n') #循环调用commands内的命令
time.sleep(2) #时间模块,暂停2s 等待设备响应
output = (tn.read_very_eager().decode("ascii")) #输出结果区域
print(output)
tn.close()
⑤-异常处理-telnetlib案例:
增加异常处理,防止某台交换机登录失败,导致程序中断
python
import telnetlib
import time
hosts =['192.168.2.11','192.168.2.12','192.168.2.13','192.168.2.14','192.168.2.15','192.168.2.16'] #s表示列表
user = '111'
password = '111'
commands = ['sys','int loopback 1','ip address 1.1.1.1 32','quit','quit','save','y'] #相当于把命令刷到文档里,s表示列表
Error= [] #创建Error队列
for ip in hosts: #循环登录ip
try:
tn = telnetlib.Telnet(ip) #tn实例化函数 telnet设备ip地址
tn.read_until(b"Username:",timeout=1) #read函数 读取设备返回的Username字符串
tn.write(user.encode('ascii') + b'\n') #将用户名转为ascii编码方式将字符串转换为bytes \n回车
tn.read_until(b"Password:",timeout=1)
tn.write(password.encode('ascii') + b'\n')
print('已登录进设备'+ ip)
for command in commands:
tn.write(command.encode('ascii')+b'\n') #循环调用commands内的命令
time.sleep(2) #时间模块,暂停2s 等待设备响应
output = (tn.read_very_eager().decode("ascii")) #输出结果区域
print(output)
tn.close()
except: #只要登录错误都按如下打印
# except TimeoutError: # 只会处理timeoutError的错误
print("登录失败,登录超时的设备IP:"+ip )
Error.append(ip) #append添加,附加,将失败的ip添加到Error的列表
print(Error) #打印失败的设备ip
连接异常和密码错误异常 处理判断
⑥-通过文本读取IP和命令-telnetlib案例:
将ip地址或者命令放在txt文本文件中,通过脚本读取文本文件
python
import telnetlib
import time
hosts = open('hosts.txt','r') #使用open函数 打开文本,并read 读取
user = '111'
password = '111'
commands = open('commands.txt','r')
ConnectionError= [] #连接失败的列表
for ip in hosts.readlines(): #readline 逐行读取文本文件
try:
ip =ip.strip() #strip函数可以去除文本中的回车和空格
tn = telnetlib.Telnet(ip) #tn实例化函数 telnet设备ip地址
tn.read_until(b"Username:") #read函数 读取设备返回的Username字符串
tn.write(user.encode('ascii') + b'\n') #将用户名转为ascii编码方式将字符串转换为bytes \n回车
tn.read_until(b"Password:")
tn.write(password.encode('ascii') + b'\n')
print('已登录进设备'+ ip)
#print(f'已登录进设备:{ip}')
commands.seek(0) #光标每次读取完都会在最后,需要用seek函数将光标置于最前面
for command in commands.readlines():
tn.write(command.encode('ascii')+b'\n') #循环调用commands内的命令
time.sleep(2)
output = (tn.read_very_eager()).decode("ascii") #输出结果区域
print(output)
tn.close()
except: #只要登录错误都按如下打印
print("##########登录失败,登录超时的设备IP:"+ip+'##########' )
ConnectionError.append(ip) #append添加,附加,将失败的ip添加到ConnectionError的列表
print(ConnectionError)
⑦-封装成函数解决问题-telnetlib案例:
将telnetlib封装成函数,方便小规模灵活应用,便于调用,同时增加input输入用户名和密码。允许部分交换机用户名和密码不同。
python
import telnetlib
import time
commands = open('commands.txt','r')
ConnectionError= []
AuthenFaill= []
def Telnet (host,username=input('请输入用户名:'),password=input('请输入密码:'),port=23): #封装定义函数,定义host username password
try:
tn = telnetlib.Telnet(host) #tn实例化函数 telnet设备ip地址
tn.read_until(b"Username:") #read函数 读取设备返回的Username字符串
tn.write(username.encode('ascii') + b'\n') #将用户名转为ascii编码方式将字符串转换为bytes \n回车
tn.read_until(b"Password:")
tn.write(password.encode('ascii') + b'\n')
index,obj,oup=tn.expect([b'Info',b'Error'],timeout=1)
if index ==0:
print('已经成功登录设备'+host)
elif index == 1:
print('设备用户或密码错误'+host)
AuthenFaill.append(host)
commands.seek(0) #光标每次读取完都会在最后,需要用seek函数将光标置于最前面
for command in commands.readlines():
tn.write(command.encode('ascii')+b'\n') #循环调用commands内的命令
time.sleep(2)
output = (tn.read_very_eager()).decode("ascii") #输出结果区域
print(output)
tn.close()
except :
print("连接异常失败IP:"+host)
ConnectionError.append(host) #append添加,附加,将失败的ip添加到ConnectionError的列表
Telnet('192.168.2.11')
Telnet('192.168.2.12')
Telnet('192.168.2.13',username='root',password='roo111') #如果此台主机密码不同于其他,可以直接赋予正确的密码
Telnet('192.168.2.14')
Telnet('192.168.2.15')
Telnet('192.168.2.16')
print('-'*100)
print('认证失败的设备如下:')
print(AuthenFaill)
print('-'*100)
print('连接异常的设备如下:')
print(ConnectionError)
⑧-ping案例:
可以使用os.system或者subprocess模块实现ping检测
python
import os
ip ='192.168.1.1'
response =os.system('ping -n 1 '+ip) #发1包
if response ==0:
print(ip,'is up!')
else:
print(ip,'is down!')
或者
python
import os
host = ['192.168.1.1']
for ip in host:
try:
ping_result = os.system(f"ping -n 1 {ip}")
if ping_result == 0:
print(f"{ip} 连通性正常")
else:
print(f"{ip} 连通性异常")
except Exception as e:
print("检查 {ip} 连通性时出现异常:{e}")
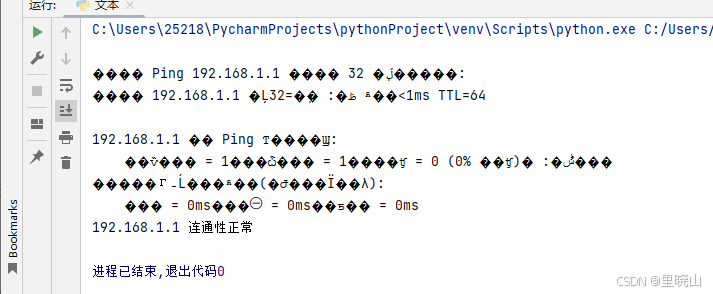
或者
python
import subprocess #subprocess模块执行ping命令(只返回结果不返回打印)
host = ['192.168.1.1']
for ip in host:
try:
command = ['ping', '-n', str(1), ip]
result = subprocess.run(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
#朱提部分subprocess.run执行命令,捕获输出但不打印
if result.returncode == 0: #检查是否执行成功
print('ok')
else:
print('pok')
#print('pok',result.stderr) 如果需要可以打印错误信息
except Exception as e:
print("检查 {ip} 连通性时出现异常:{e}")
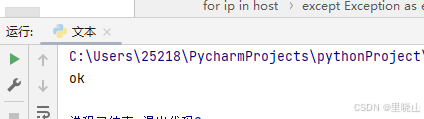