import router from '@ohos.router';
import { ShopCarEntity } from '../../entity/ShopCarEntity';
import LocDataModel from '../../viewmodel/LocDataModel';
import data from '@ohos.telephony.data';
@Entry
@Component
export default struct CarPage {
@State carList:ShopCarEntity[]=LocDataModel.DEFAULT_SHOP_CART_LIST
@State totalPrice :number =0
@State totalCount:number =0
//计算总价和数量
countTotalNumber(){
let tempTotalPrice=0
let temTotalCount=0
this.carList.forEach((item:ShopCarEntity)=>{
if (item.isSelected) {
tempTotalPrice+=item.goods_default_price*item.count
temTotalCount+=item.count
}
})
this.totalPrice=tempTotalPrice
this.totalCount=temTotalCount
}
//处理商品单选操作
carItemSelectChanged(state:boolean,index:number){
let newCarlist:ShopCarEntity[]=[]
this.carList.forEach((item:ShopCarEntity,itemIndex:number)=>{
if (itemIndex===index) {
item.isSelected=state
item.itemkey=`${Math.random()*10}-${Math.random()}`
}
newCarlist.push(item)
})
this.carList=newCarlist
}
build() {
Flex({direction:FlexDirection.Column}){
//标题部分
this.TitleBarComponent()
//商品列表
this.CarListCompoent()
//底部
this.BottomOptionsCompoent()
}
.width('100%')
.height('100%')
.backgroundColor($r('app.color.page_background'))
}
/**
* 标题
*/
@Builder TitleBarComponent(){
Stack({alignContent:Alignment.End}){
Text('购物车')
.width('100%')
.textAlign(TextAlign.Center)
.fontColor(Color.White)
.fontSize(16)
.fontWeight(FontWeight.Bold)
Text('删除已选')
.borderWidth(1).
borderColor(Color.White).
borderRadius(12).
fontColor(Color.White).
fontSize(12).
fontWeight(FontWeight.Bold)
.padding({
top:5,
right:8,
bottom:5,
left:8
})
.textAlign(TextAlign.Center)
.lineHeight(12)
.margin({
right:15
})
}.width('100%')
.height('56vp')
.backgroundColor('#ff0000')
}
/**
* 添加商品数量
*
*/
addGoodsCount(index:number){
let newCarList:ShopCarEntity[]=[]
this.carList.forEach((item:ShopCarEntity,itemIndex:number)=>{
if (itemIndex===index) {
item.count++
item.itemkey=`${Math.random()*10}-${item.id}-${Math.random()}}`
}
newCarList.push(item)
})
//重新赋值
this.carList=newCarList
}
/**
* 减少商品数量
*/
reduceGoodsCount(index:number){
let newCarList:ShopCarEntity[]=[]
this.carList.forEach((item:ShopCarEntity,itemIndex:number)=>{
if (itemIndex===index) {
item.count--
item.itemkey=`${Math.random()*10}-${item.id}-${Math.random()}`
}
newCarList.push(item)
})
//重新赋值
this.carList=newCarList
}
/**
* 给itemView生成唯一的key
*
*/
generatorItemViewKey(data:ShopCarEntity):string{
if (data.itemkey) {
return data.itemkey
}
return `${Math.random()*10}*${data.id}--${Math.random()}]`
}
/**
* 商品列表
*/
@Builder CarListCompoent(){
Scroll(){
Column(){
if (this.carList.length>0){
List({space:2}){
ForEach(this.carList,(item:ShopCarEntity,index:number)=>{
ListItem(){
this.CarItemComponent(item,index)
}
},(item:ShopCarEntity) => this.generatorItemViewKey(item))
}
}else {
this.EmptyComponent()
}
}.width('100%').height('100%')
}.width('100%')
.flexGrow(1)
}
@Builder
CarItemComponent(data:ShopCarEntity,index:number){
Flex({direction:FlexDirection.Row,alignItems:ItemAlign.Center}){
//复选框
Checkbox().width(20).height(20).select(true).selectedColor($r('app.color.focus_color'))
.onChange((value:boolean)=>{
this.carItemSelectChanged(value,index)
})
Image(data.goods_default_icon)
.margin({left:10,right:10})
.width(100).height(100).objectFit(ImageFit.Contain).key(data.goods_id.toString())
//商品描述和价格
Column(){
Text(data.goods_desc).fontSize(14).fontColor(Color.Gray).maxLines(2).textOverflow({overflow:TextOverflow.Ellipsis})
//商品价格
Text(){
Span('价格:').fontSize(12).fontColor(Color.Black)
Span(`${data.goods_default_price}`).fontColor($r('app.color.focus_color')).fontSize(12)
}.margin({top:5,bottom:5})
//数量加减
Row(){
Text('数量:').fontSize(12).fontColor(Color.Black)
Counter(){
Text(`${data.count}`).fontSize(12)
}.width(100).height(20)
.onInc(()=>{
if (data.count<50) {
this.addGoodsCount(index)
}
})
.onDec(()=>{
if (data.count>1) {
this.reduceGoodsCount(index)
}
})
//空白组件
Blank().layoutWeight(1)
//当前商品选中时候,才显示
if (data.isSelected){
Image($r('app.media.news_selected')).width(15)
.objectFit(ImageFit.Contain).onClick(()=>{
})
}
}.alignItems(VerticalAlign.Center)
}.alignItems(HorizontalAlign.Start)
.margin({right:5})
}.padding({left:5,right:5})
.backgroundColor(Color.White)
}
@Builder EmptyComponent(){
Column(){
Column(){
Image($r('app.media.caca')).width(70).objectFit(ImageFit.Contain)
Text('欢迎添加购物车!!!').fontColor(Color.Gray).fontSize(14).margin({
top:5
})
}.justifyContent(FlexAlign.Center)
.alignItems(HorizontalAlign.Center)
}.width('100%')
.height('100%')
.justifyContent(FlexAlign.Center)
}
/**
* 底部操作
*
*/
@Builder BottomOptionsCompoent(){
Flex({direction:FlexDirection.Row,justifyContent:FlexAlign.SpaceBetween,alignItems:ItemAlign.Center}){
//全选和总价
Row(){
Checkbox()
.width(15)
.height(15)
.select(true).selectedColor($r('app.color.select_color'))
.onChange((value:boolean)=>{
})
Text('全选').fontColor(Color.Gray).fontSize(12)
//总价
Text(){
Span('总价: ').fontColor(Color.Black).fontSize(12).fontWeight(FontWeight.Bold)
Span('qwqqeqeqeq').fontColor($r('app.color.focus_color')).fontSize(12)
Span('元').fontColor(Color.Black).fontSize(12).fontWeight(FontWeight.Bold)
Span('12节').fontColor(Color.Black).fontSize(12).fontWeight(FontWeight.Bold)
}.margin({left:20})
.textAlign(TextAlign.Start)
}
.margin({left:10})
.height('100%')
//立即结算
Button('立即结算').margin({right:10}).width(80).height(35).fontSize(12).backgroundColor($r('app.color.focus_color')).onClick(()=>{
})
}.width('100%')
.height('50vp')
.backgroundColor(Color.White)
}
}
购物车的代码,以及所有的代码点击加减事件结算
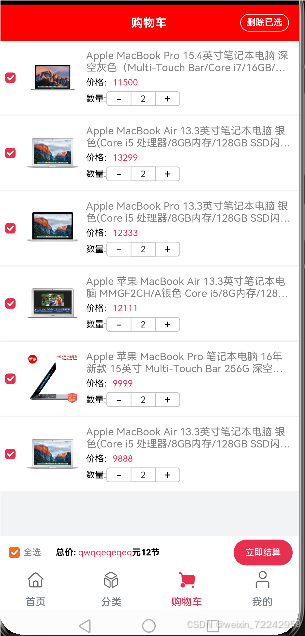
这是整体的代码,数量加减和多选删除以及结算
欢迎大家一起讨论,修改和补充代码中的不足之处