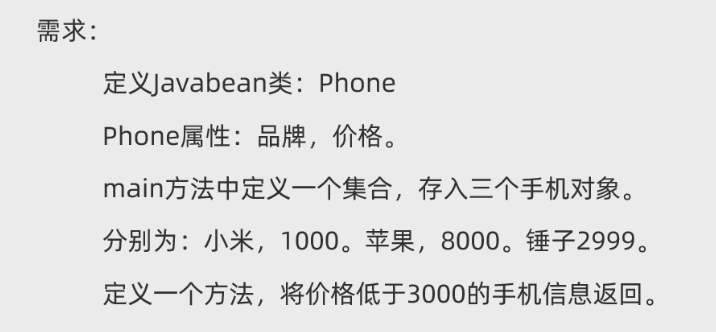
java
import java.util.ArrayList;
/**
* @author gyf
* @ClassName Test
* @Date 2024/8/3 23:54
* @Version V1.0
* @Description :
*/
public class Test {
public static void main(String[] args) {
ArrayList<Phone> list = new ArrayList<>();
Phone phone1 = new Phone("小木", 1000);
Phone phone2 = new Phone("小红", 8000);
Phone phone3 = new Phone("小左", 5000);
list.add(phone1);
list.add(phone2);
list.add(phone3);
ArrayList<Phone> phones = searchInfo(list);
for (int i = 0; i < phones.size(); i++) {
System.out.println(phone1.getBrand() + "," + phone1.getPrice());
}
}
public static ArrayList<Phone> searchInfo(ArrayList<Phone> list) {
ArrayList<Phone> phones = new ArrayList<>();
for (int i = 0; i < list.size(); i++) {
Phone phone = list.get(i);
int price = phone.getPrice();
if (price < 3000) {
phones.add(phone);
}
}
return phones;
}
}
java
/**
* @ClassName Phone
* @author gyf
* @Date 2024/8/3 23:47
* @Version V1.0
* @Description :
*/
public class Phone {
private String brand;
private int price;
public Phone() {
}
public Phone(String brand, int price) {
this.brand = brand;
this.price = price;
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
}