目录
jsonpath模块
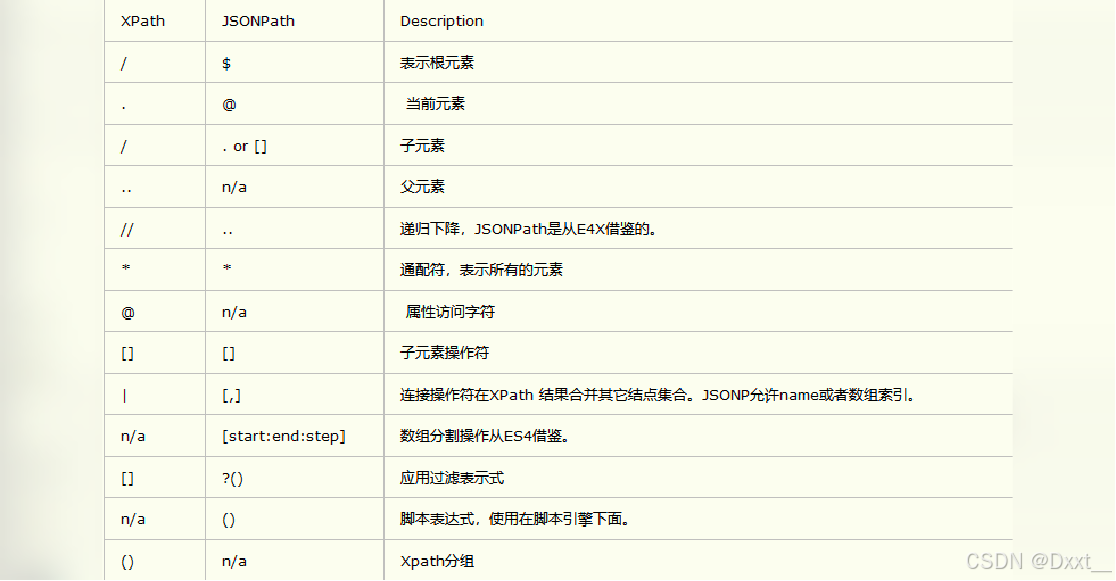
# pip install jsonpath -i https://pypi.tuna.tsinghua.edu.cn/simple
import jsonpath
data = {
"store": {
"book":
[{
"category": "reference",
"author": "Nigel Rees",
"title": "Sayings of the Century",
"price": 8.95
},{
"category": "fiction",
"author": "Evelyn Waugh",
"title": "Sword of Honour",
"price": 12.99
},{
"category": "fiction",
"author": "Herman Melville",
"title": "Moby Dick",
"isbn": "0-553-21311-3",
"price": 8.99
}, {
"category": "fiction",
"author": "J. R. R. Tolkien",
"title": "The Lord of the Rings",
"isbn": "0-395-19395-8",
"price": 22.99
}],
"bicycle":
{"color": "red",
"price": 19.95}
}
}
# print(data['store']['book'][0]['title'])
# print(jsonpath.jsonpath(data,'$.store.book[*].title'))
# print(jsonpath.jsonpath(data,'$..title'))
# 在jsonpath下标中,正数下标可以直接用,负数下标要通过切片来使用
# print(jsonpath.jsonpath(data,'$.store.book[-1].title')) # False
# print(jsonpath.jsonpath(data,'$.store.book[-1:].title'))
# print(jsonpath.jsonpath(data,'$.store.book[-2:-1].title')) #获取倒数第二个
# (@.length):获取当前元素的长度
# print(jsonpath.jsonpath(data,'$.store.book[(@.length-1)].title'))
# print(jsonpath.jsonpath(data,'$..book[?(@.isbn)]'))
print(jsonpath.jsonpath(data,'$..book[?(@.price>10)]'))
腾讯招聘数据获取
from requests_html import HTMLSession
import jsonpath
session = HTMLSession()
url = 'https://careers.tencent.com/tencentcareer/api/post/Query?timestamp=1722413528913&countryId=&cityId=&bgIds=&productId=&categoryId=&parentCategoryId=&attrId=&keyword=&pageIndex=1&pageSize=10&language=zh-cn&area=cn'
reponse = session.get(url).json()
print(jsonpath.jsonpath(reponse, '$..RecruitPostName'))