效果:
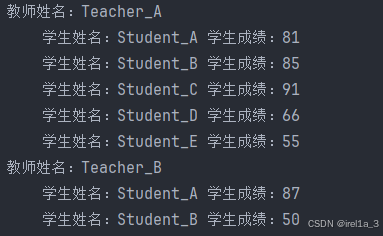
代码:
cpp
#include <iostream>
#include <string>
using namespace std;
#include "random"
int get_random_num(int min,int max)
{
random_device rd;
mt19937 gen(rd());
uniform_int_distribution<> dis(min,max);
int random_number = dis(gen);
return random_number;
}
//结构体,学生(姓名,分数)
struct Student
{
string sName;
int score;
};
//结构体,教师(姓名,管理打学生)
struct Teacher
{
string tName;
struct Student sArray[5];
};
//函数,分配空间
void allocateSpace(struct Teacher tArray[],int len)
{
string nameSeed="ABCDE";
for(int i=0;i<len;i++)
{
tArray[i].tName="Teacher_"; //教师姓名
tArray[i].tName+=nameSeed[i];
for(int j=0;j<5;j++)
{
tArray[i].sArray[j].sName="Student_"; //学生姓名
tArray[i].sArray[j].sName+=nameSeed[j];
// int random=rand()%61+40; //学生成绩 rand()%60是0-59随机数
int random = get_random_num(40,100);
tArray[i].sArray[j].score=random;
}
}
}
//函数,打印信息
void printInfo(struct Teacher tArray[],int len)
{
for(int i=0;i<len;i++)
{
cout<<"教师姓名:"<<tArray[i].tName<<endl;
for(int j=0;j<5;j++)
{
cout<<"\t学生姓名:"<<tArray[i].sArray[j].sName<<" 学生成绩:"<<tArray[i].sArray[j].score<<endl;
}
}
}
int main(){
struct Teacher tArray[3];
int len=sizeof(tArray)/sizeof (tArray[0]);
allocateSpace(tArray,len);
printInfo(tArray,len);
return 0;
}
总结:
1)使用字符串要先声明#include <string>;
2)结构体结尾需要加分号,结构体可以包含结构体;
3)函数的定义如图,参数列表要写全,例:void allocateSpace(struct Teacher tArray[],int len);
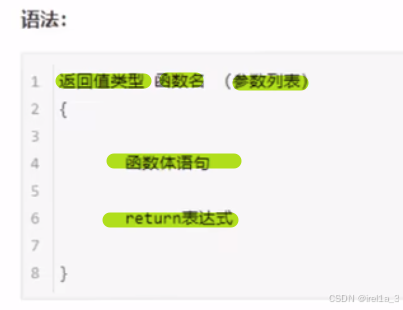
4)随机数int num=random()%60是随机生成0-59的意思;
5)len表长度,可以用int len=sizeof(Array)/sizeof(Array[0]);来计算长度;