效果图
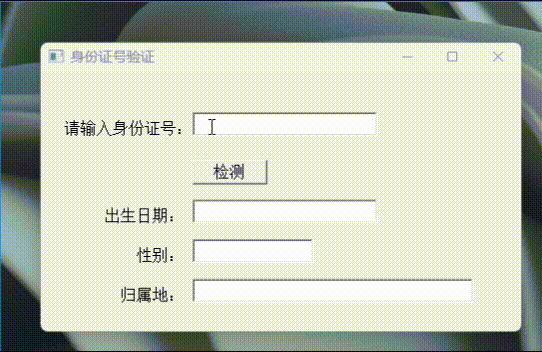
身份证行政区划分代码
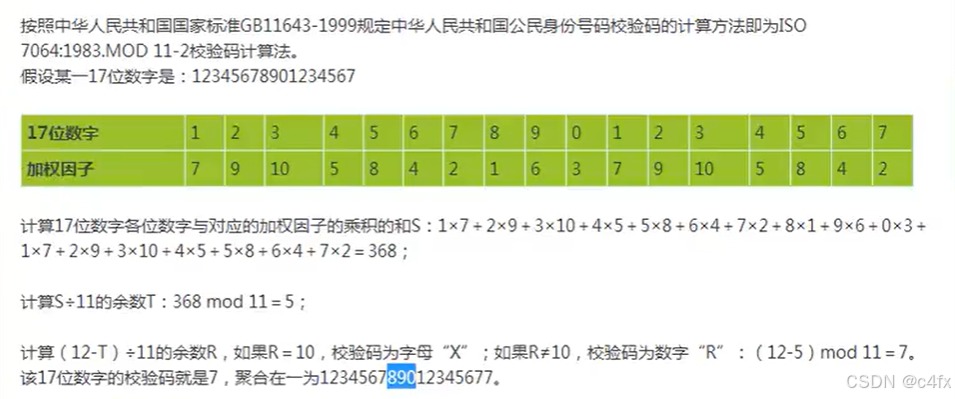
识别归属地需要行政区划分,都在data.txt文档里面了。
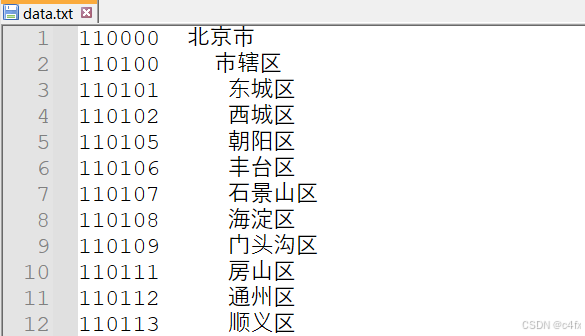
最后一位校验码
根据上面的原理编写程序即可。
d
{这个函数计算最后一位检验码是否正确,ID是18位身份证号字符串,结果返回字符串}
function IDcheck(ID:string):string;
const
//权重
W:array[1..17] of Integer = (7,9,10,5,8,4,2,1,6,3,7,9,10,5,8,4,2);
//最后一位校验码
A:array[0..10] of Char = ('1','0','x','9','8','7','6','5','4','3','2');
var
newID:string;
j,i,S:Integer;
begin
newID:=ID;
S:=0;
for i:=1 to 17 do
begin
//求加权因子的乘积。
j:=strtoint(newID[i])*W[i];
//求和
S:=S+j;
end;
//求模
S:=S mod 11;
//查表
Result:=A[S];
end;
识别出生日期
传入的身份证号就有出生日期,直接截取相应字符串就好。有趣的是,使用到了参数传递,直接修改变量的值。
g
{年月日这三个是通过 var 关键字传递的变量,
意味着它们在函数内部被修改后,其变化会反映到调用函数时的变量上。}
function IDcard(IDNo:string;var year,month,day:Integer):Boolean;stdcall;
begin
if Length(IDNo)<>18 then
begin
year:=-1;
Result:=False;
end
//判断最后一位检验码是否正确
else if IDcheck(IDNo)=Copy(IDNo,18,1) then
begin
//将年月日截取出来
year:=StrToInt(Copy(IDNo,7,4));
month:=StrToInt(Copy(IDNo,11,2));
day:=StrToInt(Copy(IDNo,13,2));
Result:=True;
end
else
Result:=False;
end;
识别性别
原理同上表,偶数为女生,奇数为男生。
d
{检查性别}
function sexcheck(IDNo:string):string;stdcall;
var
str:string;
begin
str:=Copy(IDNo,17,1);
if StrToInt(str) mod 2 =1 then
Result:='男'
else
Result:='女';
end;
识别归属地
需要查data.txt,这个文件已经上传到CSDN了,上面有链接,可以自取。
d
{判断归属地}
function Addcheck(IDNo:string):string;stdcall;
var
F1:TextFile;
str_temp,str1,str2,str3,str4:string;
begin
//AssignFile 函数是用于将文件变量与磁盘上的文件关联起来的。
//这是进行文件操作(如读写文件)的第一步。
AssignFile(F1,'data.txt');
Reset(F1); // 尝试以读取模式打开文件
try
Readln(F1,str1); //将F1的内容第一行读到str1
while not Eof(F1) do // 检查文件是否成功打开
begin
str_temp:=str1;
str1:=Copy(str1,1,6); //截取前六位行政区号
//截取前两位判断省份
if str1=Copy(IDNo,1,2)+'0000' then
begin
str2:=Trim(Copy(str_temp,7,40));
end;
//判断市
if str1=Copy(IDNo,1,4)+'00' then
begin
str3:=Trim(Copy(str_temp,7,40));
end;
//判断县
if str1=Copy(IDNo,1,6) then
begin
str4:=Trim(Copy(str_temp,7,40));
result:=str2+str3+str4;
Exit;
end;
Readln(F1,str1);
end;
Result:='输入不合法,请重新输入!';
finally
CloseFile(F1);
end;
end;
完整DLL文件
h
library Project1;
{这是动态链接库了}
{ Important note about DLL memory management: ShareMem must be the
first unit in your library's USES clause AND your project's (select
Project-View Source) USES clause if your DLL exports any procedures or
functions that pass strings as parameters or function results. This
applies to all strings passed to and from your DLL--even those that
are nested in records and classes. ShareMem is the interface unit to
the BORLNDMM.DLL shared memory manager, which must be deployed along
with your DLL. To avoid using BORLNDMM.DLL, pass string information
using PChar or ShortString parameters. }
uses
SysUtils,Classes,Windows,Controls,Messages,Dialogs;
{$R *.RES}
{第一步:编写DLL文件的函数,加上stdcall,表明函数被外部调用}
{这个函数计算最后一位检验码是否正确,ID是18位身份证号字符串,结果返回字符串}
function IDcheck(ID:string):string;
const
//权重
W:array[1..17] of Integer = (7,9,10,5,8,4,2,1,6,3,7,9,10,5,8,4,2);
//最后一位校验码
A:array[0..10] of Char = ('1','0','x','9','8','7','6','5','4','3','2');
var
newID:string;
j,i,S:Integer;
begin
newID:=ID;
S:=0;
for i:=1 to 17 do
begin
//求加权因子的乘积。
j:=strtoint(newID[i])*W[i];
//求和
S:=S+j;
end;
//求模
S:=S mod 11;
//查表
Result:=A[S];
end;
{年月日这三个是通过 var 关键字传递的变量,
意味着它们在函数内部被修改后,其变化会反映到调用函数时的变量上。}
function IDcard(IDNo:string;var year,month,day:Integer):Boolean;stdcall;
begin
if Length(IDNo)<>18 then
begin
year:=-1;
Result:=False;
end
//判断最后一位检验码是否正确
else if IDcheck(IDNo)=Copy(IDNo,18,1) then
begin
//将年月日截取出来
year:=StrToInt(Copy(IDNo,7,4));
month:=StrToInt(Copy(IDNo,11,2));
day:=StrToInt(Copy(IDNo,13,2));
Result:=True;
end
else
Result:=False;
end;
{检查性别}
function sexcheck(IDNo:string):string;stdcall;
var
str:string;
begin
str:=Copy(IDNo,17,1);
if StrToInt(str) mod 2 =1 then
Result:='男'
else
Result:='女';
end;
{判断归属地}
function Addcheck(IDNo:string):string;stdcall;
var
F1:TextFile;
str_temp,str1,str2,str3,str4:string;
begin
//AssignFile 函数是用于将文件变量与磁盘上的文件关联起来的。
//这是进行文件操作(如读写文件)的第一步。
AssignFile(F1,'data.txt');
Reset(F1); // 尝试以读取模式打开文件
try
Readln(F1,str1); //将F1的内容第一行读到str1
while not Eof(F1) do // 检查文件是否成功打开
begin
str_temp:=str1;
str1:=Copy(str1,1,6); //截取前六位行政区号
//截取前两位判断省份
if str1=Copy(IDNo,1,2)+'0000' then
begin
str2:=Trim(Copy(str_temp,7,40));
end;
//判断市
if str1=Copy(IDNo,1,4)+'00' then
begin
str3:=Trim(Copy(str_temp,7,40));
end;
//判断县
if str1=Copy(IDNo,1,6) then
begin
str4:=Trim(Copy(str_temp,7,40));
result:=str2+str3+str4;
Exit;
end;
Readln(F1,str1);
end;
Result:='输入不合法,请重新输入!';
finally
CloseFile(F1);
end;
end;
{第二步:导出函数}
exports
IDcard,sexcheck,Addcheck;
begin
end.
完整代码
放在CSDN了,可以自取。
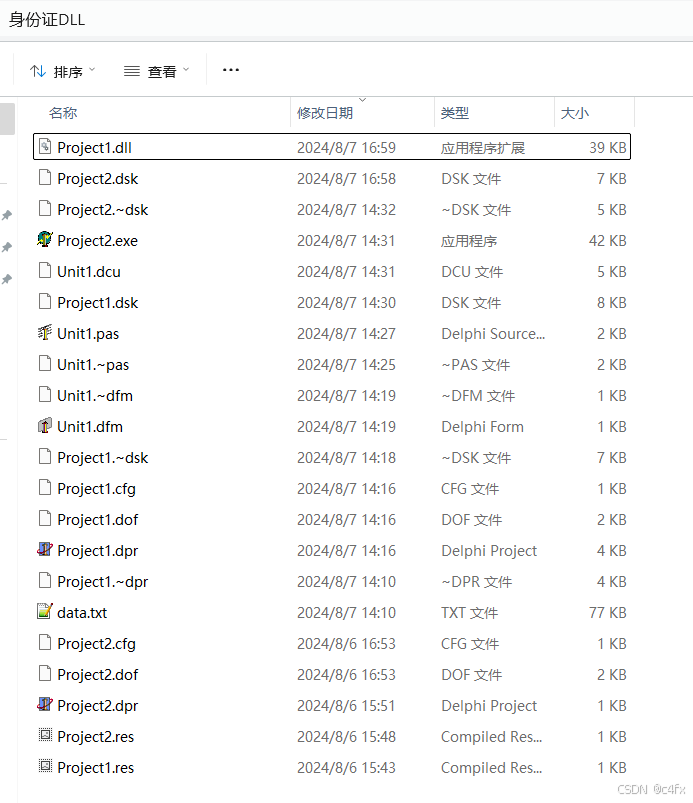