jQuery
1.jQuery操作DOM
DOM为文档提供了一种结构化表示方法,通过该方法可以改变文档的内容和展示形式
在访问页面时,需要与页面中的元素进行交互式的操作。在操作中,元素的访问是最频繁、最常用的,主要包括对元素属性attr、内容html、值value、CSS 的操作
1.1 操作内容
获取和设置元素内容
操作元素内容的方法包括html()和text(),比如文本框后面的提示信息
获取和设置元素值
要获取元素的值通过val()方法实现
比如文本框的值之类的
html
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
$("#btn01").click(function(){
console.log($("#span01").text());
})
$("#btn02").click(function(){
$("#span01").text("<h1>做真实的自己</h1>");
})
$("#btn03").click(function(){
console.log($("#span02").html());
})
$("#btn04").click(function(){
$("#span02").html("<h1>做真实的自己</h1>");
})
})
</script>
</head>
<body>
<button id="btn01">获取内容 - text</button>
<button id="btn02">设置内容 - text</button>
<br />
<span id="span01">用良心做教育</span>
<br />
<button id="btn03">获取内容 - html</button>
<button id="btn04">设置内容 - html</button>
<br />
<span id="span02">用良心做教育</span>
</body>
</html>
1.2 操作属性
获取元素属性:
使用attr(name)方法获取元素的属性
设置元素的属性:
通过attr(name,value)的方式设置元素的属性
删除元素的属性
使用removeAttr(name)方法可以将元素的属性删除
html
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
$("#btn01").click(function(){
console.log($("img").attr("src"));
console.log($("img").attr("width"));
console.log($("img").attr("height"));
})
$("#btn02").click(function(){
$("img").attr("src","../img/樱井步.jpg");
$("img").attr("width","100px");
$("img").attr("height","100px");
})
$("#btn03").click(function(){
$("img").removeAttr("width");
$("img").removeAttr("height");
})
})
</script>
</head>
<body>
<button id="btn01">获取属性</button>
<button id="btn02">设置属性</button>
<button id="btn03">删除属性</button>
<br />
<img src="../img/波多野结衣.jpg" width="50px" height="50px" />
</body>
</html>
val () 方法返回或设置被选元素的value值
html
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
$("#btn01").click(function(){
//console.log($("input").attr("value"));
console.log($("input").val());
})
$("#btn02").click(function(){
//$("input").attr("value","用良心做教育");
$("input").val("用良心做教育");
})
})
</script>
</head>
<body>
<input type="text" />
<button id="btn01">获取输入框的数据</button>
<button id="btn02">设置输入框的数据</button>
</body>
</html>
操作属性案例
html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style type="text/css">
span{color: red;}
</style>
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
//给表单绑定一个提交事件,false就提交不了,true就可以提交
$("form").submit(function(){
//先清空
$("#username+span").text("");
$("#password+span").text("");
$("#repassword+span").text("");
var bool = true;
//trim去空格
if($.trim($("#username").val()) == ""){
$("#username+span").text("账号不能为空");
bool = false;
}
if($.trim($("#password").val()) == ""){
$("#password+span").text("密码不能为空");
bool = false;
}
if($.trim($("#repassword").val()) == ""){
$("#repassword+span").text("确认密码不能为空");
bool = false;
}else if($.trim($("#repassword").val()) != $.trim($("#password").val())){
$("#repassword+span").text("确认密码和密码不一致");
bool = false;
}
return bool;
})
})
</script>
</head>
<body>
<form action="#" method="post">
账号:<input type="text" id="username" name="username" /><span></span><br/>
密码:<input type="password" id="password" name="password" /><span></span><br/>
确认密码:<input type="password" id="repassword" name="repassword" /><span></span><br/>
<input type="submit" value="提交" />
</form>
</body>
</html>
运行结果:
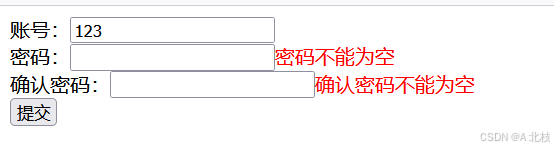
1.3 操作样式(CSS)
在页面中,元素样式的操作包含:直接设置样式、增加CSS类别、删除类别、类别切换
调用css(name,value)方法直接设置元素的值
html
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
/**
* 案例:点击图片,使图片自动变大
* 案例:点击字体,是字体变粗,背景颜色变蓝
*/
$("img").click(function(){
$(this).css("width","200px");
$(this).css("height","200px");
})
$("p").click(function(){
$(this).css("font-size","50px");
$(this).css("background-color","blue");
})
})
</script>
</head>
<body>
<img src="../img/波多野结衣.jpg" width="100px" height="100px" />
<p>用良心做教育</p>
</body>
</html>
html
<style type="text/css">
.big{
width: 200px;
height: 200px;
}
</style>
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
/**
* 案例:点击图片,使图片自动变大,再次点击又变小,循环往复
*/
$("img").click(function(){
//判断当前对象的class属性是否有big,有就返回true
if($(this).hasClass("big")){
$(this).removeClass("big");
}else{
$(this).addClass("big");
}
})
})
</script>
</head>
<body>
<img src="../img/波多野结衣.jpg" width="100px" height="100px" />
</body>
</html>
更好的方法
html
<style type="text/css">
.big{
width: 200px;
height: 200px;
}
</style>
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
$("img").click(function(){
//判断当前对象的class属性是否有big,有就删除,没有就添加
$(this).toggleClass("big");
})
})
</script>
</head>
<body>
<img src="../img/波多野结衣.jpg" width="100px" height="100px" />
</body>
</html>
1.4 操作节点
1.4.1 创建元素节点
使用$(html)函数动态创建节点元素
函数$(html)只完成DOM元素创建,加入到页面还需要通过元素节点的插入或追加操作;同时,在创建DOM元素时,要注意字符标记是否完全闭合,否则达不到预期效果。
案例:给页面添加图片元素节点
在页面中动态创建元素需要执行节点的插入或追加操作,append
html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style type="text/css">
img{
width: 100px;
height: 100px;
}
</style>
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
$("#btn01").click(function(){
var img = $("<img src='../img/波多野结衣.jpg'/>");//创建一个jQuery对象
//将图片添加到div里 -- 末尾
//$("#manager").append(img);
//$(img).appendTo($("#manager"));
//将图片添加到div里 -- 首位
//$("#manager").prepend(img);
//$(img).prependTo($("#manager"));
//将图片添加到div标签后面 -- 并列关系
//$("#manager").after(img);
//$(img).insertAfter($("#manager"));
//将图片添加到div标签前面 -- 并列关系
//$("#manager").before(img);
$(img).insertBefore($("#manager"));
})
$("#btn02").click(function(){
$("img:first").remove();
})
})
</script>
</head>
<body>
<button id="btn01">添加节点</button>
<button id="btn02">删除节点</button>
<div id="manager">
<img src="../img/樱井步.jpg"/>
</div>
</body>
</html>
运行结果:
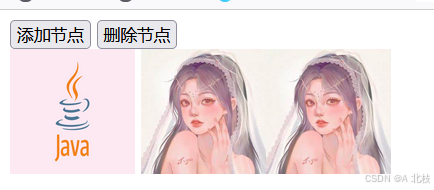
1.4.2 遍历元素
在DOM元素操作中,有时需要对同一标记的全部元素进行统一操作。
在传统的JavaScript中,先获取元素的总长度,然后以for循环语句递减总长度,访问其中的某个元素,代码相对复杂;
而在jQuery中,可以直接使用each(callback)方法实现元素的遍历
$("img").each(function(){ ...})
案例:点击图片,就会变成其他的图片
html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style type="text/css">
img{
width: 100px;
height: 100px;
}
</style>
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
$("img").each(function(){//遍历所有img标签
$("img").click(function(){//给每一个img便签绑定一个点击事件
$(this).attr("src","../img/乐乐.jfif");
})
})
})
</script>
</head>
<body>
<img src="../img/1.webp" />
<img src="../img/1.webp" />
<img src="../img/1.webp" />
<img src="../img/1.webp" />
<img src="../img/1.webp" />
<img src="../img/1.webp" />
<img src="../img/1.webp" />
<img src="../img/1.webp" />
<img src="../img/1.webp" />
<img src="../img/1.webp" />
</body>
</html>
1.4.3 练习案例
实现管理页面的全选功能
实现批量删除的功能
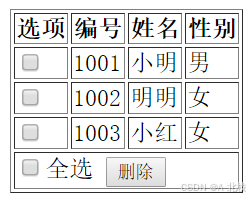
php+HTML
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
//点击全选按钮,就可以选三个按钮
$("#chkAll").click(function(){
if($(this).attr("checked")){
$("td>input").attr("checked","checked");
}else{
$("td>input").removeAttr("checked");
}
})
$("td>input").each(function(){
$(this).click(function(){
if($("td>input").length == $("td>input:checked").length){
$("#chkAll").attr("checked","checked");
}else{
$("#chkAll").removeAttr("checked");
}
})
})
//删除功能
$("#btnDel").click(function(){
$("td>input:checked").each(function(){
var deleteId = $(this).val();
$("tr[id=" + deleteId + "]").remove();
})
if($("td>input").length == 0){
$("#chkAll").removeAttr("checked");
}
})
})
</script>
</head>
<body>
<table border="1">
<tr>
<th>选项</th>
<th>编号</th>
<th>姓名</th>
<th>性别</th>
</tr>
<tr id="1">
<td><input type="checkbox" value="1"/></td>
<td>1001</td>
<td>小明</td>
<td>男</td>
</tr>
<tr id="2">
<td><input type="checkbox" value="2"/></td>
<td>1002</td>
<td>明明</td>
<td>女</td>
</tr>
<tr id="3">
<td><input type="checkbox" value="3"/></td>
<td>1003</td>
<td>小红</td>
<td>女</td>
</tr>
<tr>
<td colspan="4">
<span><input id="chkAll" type="checkbox" />全选</span>
<span><input id="btnDel" type="button" value="删除"/></span>
</td>
</tr>
</table>
</body>
</html>
2.jQuery事件
众所周知,页面在加载时,会触发Load事件。当用户单击某个按钮时,触发该按钮的Click事件,通过种种事件实现各项功能或执行某项操作。事件在元素对象与功能代码中起着重要的桥梁作用
2.1 事件冒泡现象:(可能会考)
事件在触发后分为两个阶段,一个是捕获(Capture),另一个则是冒泡(Bubbling);但大多数浏览器并不是都支持捕获阶段,jQuery也不支持。因此在事件触发后,往往执行冒泡过程。所谓的冒泡其实质就是事件执行中的顺序。
案例:掌握什么是事件的冒泡现象
冒泡现象:子元素的事件向上传递给了父级元素(一直往上传,直到找到body为止,类似于绝对定位)就是一层层往上冒
如何阻止冒泡的发生在jQuery中,可以通过e.stopPropagation()方法可以阻止冒泡过程的发生。
还可以通过语句return false实现停止事件的冒泡过程
html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style type="text/css">
#manager{
width: 200px;
height: 200px;
border: orange 1px solid;
}
</style>
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
/**
* 冒泡现象:子元素的事件向上传递给了父级元素
*/
$("#manager").click(function(){
alert("div被点击了");
})
$("#btn").click(function(event){
alert("按钮被点击了");
//阻止冒泡现象 -- 解决方案一
//event.stopPropagation();
//阻止冒泡现象 -- 解决方案二
return false;
})
})
</script>
</head>
<body>
<div id="manager">
<input id="btn" type="button" value="普通按钮" />
</div>
</body>
</html>
2.2 页面载入事件$(function(){})
一直在用
html
$(function(){ ......})
2.3 绑定事件 bind()
比如像这种方式来绑定事件click、mouseout
使用bind()方法绑定事件bind()功能是为每个选择元素的事件绑定处理函数,其语法格式如下:
bind(type,[data],fn)
这种方式相比之前:其中参数type为一个或多个类型的字符串,如"click"或"change"
html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
//绑定事件
//通过映射方式绑定事件(类似于json格式)
$("img").bind({
"click":function(){
console.log("图片被点击了");
},
"mouseout":function(){
console.log("鼠标移出图片了");
}
})
})
</script>
</head>
<body>
<img src="../img/波多野结衣.jpg" width="100px" height="100px" />
</body>
</html>
2.4 解绑事件 unbind()
unbind()方法移除元素绑定事件
unbind(type,[fn]])
html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
//绑定事件
$("img").bind("click mouseout",function(){
console.log("触发事件了");
})
$("#btn").click(function(){
//解绑所有的事件
//$("img").unbind();
//解绑指定的事件
$("img").unbind("click");
})
})
</script>
</head>
<body>
<button id="btn">解绑事件</button><br />
<img src="../img/波多野结衣.jpg" width="100px" height="100px" />
</body>
</html>
2.5 切换事件hover() toggle()
hover()方法
鼠标移入、移出事件来回切换
toggle()方法鼠标单击事件循环切换
html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
//切换事件:鼠标移入、移出事件来回切换
$("img").hover(
function(){
console.log("aaa");
},
function(){
console.log("bbb");
}
)
})
</script>
</head>
<body>
<img src="../img/波多野结衣.jpg" width="100px" height="100px" />
</body>
</html>
html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
//切换事件:鼠标单击事件循环切换
$("img").toggle(
function(){
console.log("aaa");
},
function(){
console.log("bbb");
},
function(){
console.log("ccc");
}
)
})
</script>
</head>
<body>
<img src="../img/波多野结衣.jpg" width="100px" height="100px" />
</body>
</html>
2.6 事件应用案例
2.6.1 表单验证
为各个表单项添加事件,检查其数据的合理性
为表单绑定提交事件,并做好正确的控制(事件的触发)
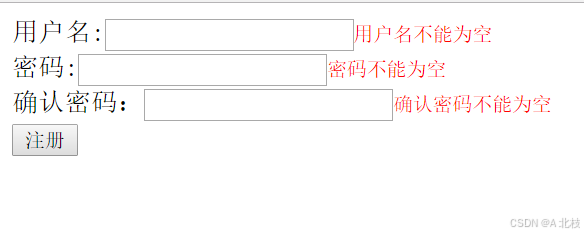
html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style type="text/css">
span{color: red;}
</style>
<script src="../js/jquery-1.8.2.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(function(){
var bool = true;
$("#username").blur(function(){
if($.trim($(this).val()) == ""){
$("#username+span").text("账号不能为空");
bool = false;
}else{
$("#username+span").text("");
}
})
$("#password").blur(function(){
if($.trim($(this).val()) == ""){
$("#password+span").text("密码不能为空");
bool = false;
}else{
$("#password+span").text("");
}
})
$("#repassword").blur(function(){
if($.trim($(this).val()) == ""){
$("#repassword+span").text("确认密码不能为空");
bool = false;
}else if($.trim($(this).val()) != $.trim($("#password").val())){
$("#repassword+span").text("确认密码与密码不一致");
bool = false;
}else{
$("#repassword+span").text("");
}
})
$("form").submit(function(){
bool = true;
//触发username、password、repassword的失去焦点事件
$("#username").trigger("blur");
$("#password").trigger("blur");
$("#repassword").trigger("blur");
return bool;
})
})
</script>
</head>
<body>
<form action="#" method="post">
账号:<input type="text" id="username" name="username" /><span></span><br/>
密码:<input type="password" id="password" name="password" /><span></span><br/>
确认密码:<input type="password" id="repassword" name="repassword" /><span></span><br/>
<input type="submit" value="提交" />
</form>
</body>
</html>
2.6.2 实现选项卡
案例:点击php,下面会显示php的内容,.net也是一样的
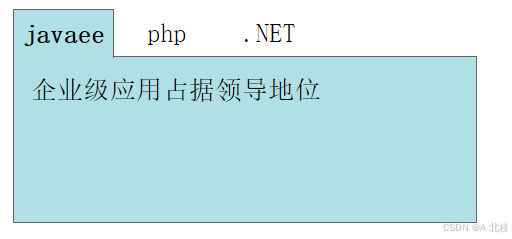
html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style type="text/css">
ul{
margin: 0;
padding: 0;
list-style: none;
}
#tab li {
text-align: center;
float: left;
padding: 5px;
margin-right: 2px;
width: 50px;
cursor: pointer
}
#tab li.tabFocus {
width: 50px;
font-weight: bold;
background-color: powderblue;
border: solid 1px #666;
border-bottom: 0;
z-index: 100;
position: relative
}
#content {
width: 260px;
height: 80px;
padding: 10px;
background-color: powderblue;
clear: left;
border: solid 1px #666;
position: relative;
top: -1px
}
#content li {
display: none
}
#content li.contentFocus {
display: block
}
</style>
<script type="text/javascript" src="../js/jquery-1.8.2.js">
</script>
<script type="text/javascript">
$(function() {
$("#tab>li").each(function(index){
$(this).click(function(){
$("#tab>li[class='tabFocus']").removeClass("tabFocus");
$(this).addClass("tabFocus");
$("#content>li[class='contentFocus']").removeClass("contentFocus");
$("#content>li:eq(" + index + ")").addClass("contentFocus");
})
})
})
</script>
</head>
<body>
<ul id="tab">
<li class="tabFocus">javaee</li>
<li>php</li>
<li>.NET</li>
</ul>
<ul id="content">
<li class="contentFocus">企业级应用占据领导地位</li>
<li>中小型网站首选</li>
<li>微软出品</li>
</ul>
</body>
</html>
3.Bootstrap
简介
Bootstrap,来自 Twitter,是目前最受欢迎的前端框架。Bootstrap 是基于 HTML、CSS、JAVASCRIPT 的,它简洁灵活,使得 Web 开发更加快捷
为什么使用Bootstrap
1.移动设备优先:自 Bootstrap 3 起,框架包含了贯穿于整个库的移动设备优先的样式。
2.浏览器支持:所有的主流浏览器都支持 Bootstrap。
3.容易上手:只要您具备 HTML 和 CSS 的基础知识,您就可以开始学习 Bootstrap。
4.响应式设计:Bootstrap 的响应式 CSS 能够自适应于台式机、平板电脑和手机。更多有关响应式设计的内容详见 Bootstrap 响应式设计
3.1 Bootstrap安装环境
CDN
注意:顺序不能乱
html
<link rel="stylesheet" href="https://cdn.staticfile.org/twitter-bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://cdn.staticfile.org/jquery/2.1.1/jquery.min.js"></script>
<script src="https://cdn.staticfile.org/twitter-bootstrap/3.3.7/js/bootstrap.min.js"></script>
3.2 常用控件
标签
html
<span class="label label-default">默认标签</span>
<span class="label label-primary">主要标签</span>
<span class="label label-success">成功标签</span>
<span class="label label-info">信息标签</span>
<span class="label label-warning">警告标签</span>
<span class="label label-danger">危险标签</span>
图片
html
<!--圆角-->
<img src="../img/纯蓝色.png" class="img-rounded"/>
<!--圆型-->
<img src="../img/纯蓝色.png" class="img-circle"/>
<!--缩略图-->
<img src="../img/纯蓝色.png" class="img-thumbnail"/>
列表组
html
<div class="list-group" style="width: 300px;">
<a href="#" class="list-group-item active">xxxx</a>
<a href="#" class="list-group-item">xxxx</a>
<a href="#" class="list-group-item">xxxx</a>
<a href="#" class="list-group-item">xxxx</a>
<a href="#" class="list-group-item">xxxx</a>
</div>
表格
html
<!--
<table class="table table-condensed">
<caption>精简表格布局</caption>
<table class="table table-striped">
<caption>条纹表格布局</caption>
<table class="table table-bordered">
<caption>边框表格布局</caption>
<table class="table table-hover">
<caption>悬停表格布局</caption>
-->
<table class="table">
<caption>基本的表格布局</caption>
<thead>
<tr>
<th>名称</th>
<th>年龄</th>
<th>性别</th>
</tr>
</thead>
<tbody>
<tr>
<td>小红</td>
<td>18</td>
<td>女</td>
</tr>
<tr>
<td>小黄</td>
<td>19</td>
<td>男</td>
</tr>
<tr>
<td>小绿</td>
<td>20</td>
<td>男</td>
</tr>
</tbody>
</table>
表单(输入框、密码框、单选、多选、下拉、各种按钮)
html
<form class="form-horizontal " role="form" >
<div class="form-group">
<label class="col-sm-2 control-label">输入框:</label>
<div class="col-sm-10 col-lg-4">
<input type="text" class="form-control" placeholder="请输入输入框">
</div>
</div>
<div class="form-group">
<label class="col-sm-2 control-label">密码框:</label>
<div class="col-sm-10 col-lg-4">
<input type="password" class="form-control" placeholder="请输入密码框">
</div>
</div>
<div class="form-group">
<label class="col-sm-2 control-label">单选框:</label>
<div class="radio col-sm-10">
<label>
<input name="sex" type="radio" checked="checked">选项 1
</label>
<label>
<input name="sex" type="radio" >选项 2
</label>
</div>
</div>
<div class="form-group">
<label class="col-sm-2 control-label">多选框:</label>
<div class="col-sm-10">
<label class="checkbox-inline">
<input type="checkbox"> 选项 1
</label>
<label class="checkbox-inline">
<input type="checkbox"> 选项 2
</label>
<label class="checkbox-inline">
<input type="checkbox"> 选项 3
</label>
</div>
</div>
<div class="form-group">
<label class="col-sm-2 control-label">下拉链表:</label>
<div class=" col-sm-10 col-lg-4">
<select class="form-control ">
<option>1</option>
<option>2</option>
<option>3</option>
<option>4</option>
<option>5</option>
</select>
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-2 col-sm-10">
<button type="submit" class="btn btn-default">提交按钮</button>
<button type="reset" class="btn btn-default">重置按钮</button>
<button type="button" class="btn btn-default">普通按钮</button>
</div>
</div>
</form>
模态框
html
<!-- 按钮触发模态框 -->
<button class="btn btn-primary btn-lg" data-toggle="modal" data-target="#myModal">开始演示模态框</button>
<!-- 模态框(Modal) -->
<div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
<h4 class="modal-title" id="myModalLabel">模态框(Modal)标题</h4>
</div>
<div class="modal-body">在这里添加一些文本</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal" onclick="fun01()">关闭</button>
<button type="button" class="btn btn-primary" onclick="fun02()">提交更改</button>
</div>
</div>
</div>
</div>
<script type="text/javascript">
function fun01(){
alert("no");
}
function fun02(){
alert("yes");
}
</script>
3.3 可视化定制
http://www.bootcss.com/p/layoutit/
学习网站:http://www.runoob.com/bootstrap/bootstrap-tutorial.html
总结
1.操作节点
2.各种事件
冒泡现象 -- 重要
注重:案例
3.Bootstrap