蓝桥杯 小蜜蜂 单元训练09:定时器实现秒闪功能
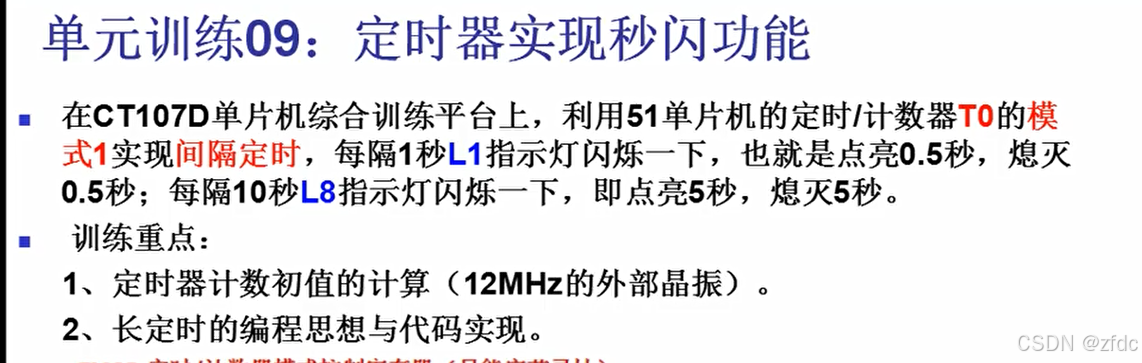
cpp
#include "stc15f2k60s2.h"
#define LED(x) \
{ \
P0 = x; \
P2 = P2 & 0x1f | 0x80; \
P2 &= 0x1f; \
}
#define L1 0xFE; // 定义L1
#define L8 0x7F; // 定义L8
typedef unsigned char uint8_t;
uint8_t timerCounterHalfSec = 0; // 0.5s,500ms计时
uint8_t EnableHalfSec = 0; // 0.5s状态达到
uint8_t timerCounterFiveSec = 0; // 5s,5000ms计时
uint8_t EnableFiveSec = 0; // 5s状态达到
uint8_t StatusHalfSec; // 用于L1切换状态
uint8_t StatusFiveSec; // 用于L8切换状态
void Timer0_Init(void) // 5毫秒@12MHz
{
AUXR |= 0x80; // 定时器时钟1T模式
TMOD &= 0xF0; // 设置定时器模式
TL0 = 0xA0; // 设置定时初始值
TH0 = 0x15; // 设置定时初始值
TF0 = 0; // 清除TF0标志
TR0 = 1; // 定时器0开始计时
ET0 = 1; // 使能定时器0中断
EA = 1;
}
void blinkL1()
{
switch (StatusHalfSec)
{
case 0:
LED(P0 & L1); // 点亮L1
if (EnableHalfSec) // 0.5s延时
{
StatusHalfSec = 1;
EnableHalfSec = 0;
}
break;
case 1:
LED(P0 | 0x01); // 熄灭L1
if (EnableHalfSec) // 0.5秒延时
{
StatusHalfSec = 0;
EnableHalfSec = 0;
}
break;
default:
StatusHalfSec = 0;
break;
}
}
void blinkL8()
{
switch (StatusFiveSec) // L8状态切换处理
{
case 0:
LED(P0 & L8); // 点亮L8
if (EnableFiveSec) // 5秒延时
{
StatusFiveSec = 1;
EnableFiveSec = 0;
}
break;
case 1:
LED(P0 | 0x80); // 熄灭L8
if (EnableFiveSec) // 5秒延时
{
StatusFiveSec = 0;
EnableFiveSec = 0;
}
break;
default:
StatusFiveSec = 0;
break;
}
}
void main()
{
LED(0xFF); // 初始化,全灭
Timer0_Init(); // 初始化定时器
while (1)
{
blinkL1();
blinkL8();
}
}
void Timer0_Isr(void) interrupt 1
{
if (++timerCounterHalfSec == 100) // 500ms,500ms/5ms = 100
{
EnableHalfSec = 1;
timerCounterHalfSec = 0;
if (++timerCounterFiveSec == 10) // 5s,5000ms,5000ms/500ms = 10
{
EnableFiveSec = 1;
timerCounterFiveSec = 0;
}
}
}