目录
[一、创建 Spring Boot Starter 项目](#一、创建 Spring Boot Starter 项目)
[2.配置 pom.xml:](#2.配置 pom.xml:)
[二、实现 EasyExcel 导出功能](#二、实现 EasyExcel 导出功能)
[5.配置 spring.factories:](#5.配置 spring.factories:)
[三、使用 Spring Boot Starter](#三、使用 Spring Boot Starter)
[2.启用 EasyExcel 导出功能:](#2.启用 EasyExcel 导出功能:)
[3.使用 EasyExcel 导出服务:](#3.使用 EasyExcel 导出服务:)
背景
在面试的时候,说spring boot核心特性之后,可以告诉面试官自己是如何实现一个spring-boot-starter来帮助项目简化某些通用的业务的。比如项目中有很多列表接口需要导出excel。我利用自动化配置写了一个excel导出模块
使用 EasyExcel
作为 Excel 导出工具,可以大幅简化 Excel 操作的复杂性。EasyExcel
是阿里巴巴开源的一个高性能的 Excel 处理库,专注于快速且低内存消耗地处理大数据量的 Excel 文件。接下来我们将使用 EasyExcel
来实现一个 Spring Boot Starter,用于简化项目中的 Excel 导出功能。
一、创建 Spring Boot Starter 项目
1.创建项目结构:
项目结构可以与之前类似,保持模块化和清晰的层次结构。
css
my-easyexcel-starter/
├── src/
│ ├── main/
│ │ ├── java/
│ │ │ └── com/
│ │ │ └── example/
│ │ │ └── easyexcel/
│ │ │ ├── config/
│ │ │ │ └── EasyExcelAutoConfiguration.java
│ │ │ ├── service/
│ │ │ │ └── EasyExcelExportService.java
│ │ │ ├── annotation/
│ │ │ │ └── EnableEasyExcelExport.java
│ │ │ └── model/
│ │ │ └── UserData.java
│ │ └── resources/
│ │ └── META-INF/
│ │ └── spring.factories
│ └── test/
└── pom.xml
2.配置 pom.xml
:
在
pom.xml
中添加EasyExcel
和 Spring Boot 依赖。
XML
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-easyexcel-starter</artifactId>
<version>1.0.0</version>
<packaging>jar</packaging>
<dependencies>
<!-- Spring Boot Dependency -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<!-- EasyExcel Dependency -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel</artifactId>
<version>3.0.5</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
二、实现 EasyExcel 导出功能
1.创建数据模型:
在
com.example.easyexcel.model
包下创建一个数据模型类UserData
,它代表要导出的 Excel 数据结构。
java
package com.example.easyexcel.model;
import com.alibaba.excel.annotation.ExcelProperty;
public class UserData {
@ExcelProperty("ID")
private Long id;
@ExcelProperty("Name")
private String name;
@ExcelProperty("Email")
private String email;
// Constructors, Getters and Setters
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public UserData(Long id, String name, String email) {
this.id = id;
this.name = name;
this.email = email;
}
}
这里使用 @ExcelProperty
注解来指定 Excel 列的标题。
2.实现导出服务
在
com.example.easyexcel.service
包下创建EasyExcelExportService
类,封装导出逻辑。
java
package com.example.easyexcel.service;
import com.alibaba.excel.EasyExcel;
import org.springframework.stereotype.Service;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.List;
@Service
public class EasyExcelExportService {
public <T> byte[] export(List<T> data, Class<T> clazz) {
try (ByteArrayOutputStream outputStream = new ByteArrayOutputStream()) {
EasyExcel.write(outputStream, clazz).sheet("Sheet 1").doWrite(data);
return outputStream.toByteArray();
} catch (IOException e) {
throw new RuntimeException("Error generating Excel file", e);
}
}
}
该服务类可以根据传入的数据列表和模型类导出 Excel 文件。
3.自动配置类
在
com.example.easyexcel.config
包下创建EasyExcelAutoConfiguration
类,配置自动装配。
java
package com.example.easyexcel.config;
import com.example.easyexcel.service.EasyExcelExportService;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class EasyExcelAutoConfiguration {
@Bean
public EasyExcelExportService easyExcelExportService() {
return new EasyExcelExportService();
}
}
4.启用注解:
创建
@EnableEasyExcelExport
注解,用于启用该功能。
java
package com.example.easyexcel.annotation;
import com.example.easyexcel.config.EasyExcelAutoConfiguration;
import org.springframework.context.annotation.Import;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Import(EasyExcelAutoConfiguration.class)
public @interface EnableEasyExcelExport {
}
5.配置 spring.factories
:
在
src/main/resources/META-INF/spring.factories
文件中,配置自动装配类。
XML
org.springframework.boot.autoconfigure.EnableAutoConfiguration=\
com.example.easyexcel.config.EasyExcelAutoConfiguration
三、使用 Spring Boot Starter
1.集成到项目:
在目标 Spring Boot 项目中引入该 Starter。
XML
<dependency>
<groupId>com.example</groupId>
<artifactId>my-easyexcel-starter</artifactId>
<version>1.0.0</version>
</dependency>
2.启用 EasyExcel 导出功能:
在应用的主类或配置类上添加 @EnableEasyExcelExport
注解:
java
@SpringBootApplication
@EnableEasyExcelExport
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
3.使用 EasyExcel 导出服务:
在控制器中使用 EasyExcelExportService
进行 Excel 导出。
java
@RestController
@RequestMapping("/api/export")
public class ExportController {
@Autowired
private EasyExcelExportService easyExcelExportService;
@GetMapping("/excel")
public ResponseEntity<byte[]> exportExcel() {
UserData userData = new UserData(1L,"小明","john@");
UserData userData1 = new UserData(1L,"小明","john@");
List<UserData> data = new ArrayList<>();
data.add(userData);
data.add(userData1);
byte[] excelContent = easyExcelExportService.export(data, UserData.class);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDisposition(ContentDisposition.builder("attachment").filename("users.xlsx").build());
return new ResponseEntity<>(excelContent, headers, HttpStatus.OK);
}
}
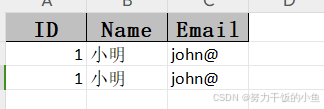
总结
通过使用
EasyExcel
,我们可以更高效地处理 Excel 文件的导出任务,并通过 Spring Boot Starter 将其封装为可复用的模块。这种方式可以简化业务代码,使项目中的重复代码减少,并确保代码的一致性和可维护性。这个 Starter 可以根据需求扩展功能,例如支持多样式、多 sheet 导出、模板支持等,更加符合企业级应用的需求。