文件的相关操作方法
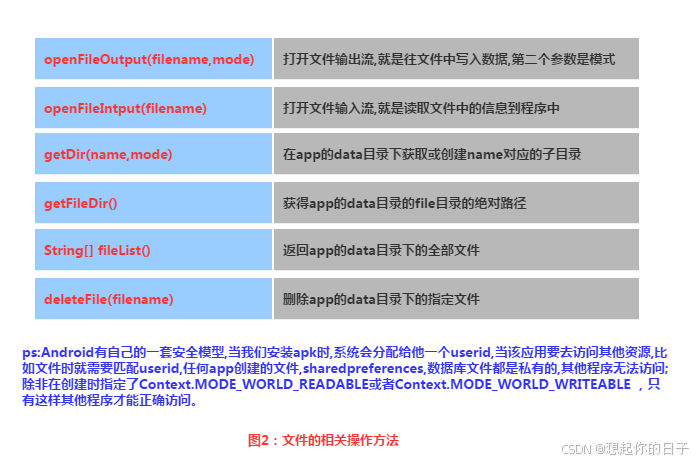
文件协助类:FileHelper.java
public class FileHelper {
private Context mContext;
public FileHelper() {
}
public FileHelper(Context mContext) {
super();
this.mContext = mContext;
}
/*
* 这里定义的是一个文件保存的方法,写入到文件中,所以是输出流
* */
public void save(String filename, String filecontent) throws Exception {
//这里我们使用私有模式,创建出来的文件只能被本应用访问,还会覆盖原文件哦
FileOutputStream output = mContext.openFileOutput(filename, Context.MODE_PRIVATE);
output.write(filecontent.getBytes()); //将String字符串以字节流的形式写入到输出流中
output.close(); //关闭输出流
}
/*
* 这里定义的是文件读取的方法
* */
public String read(String filename) throws IOException {
//打开文件输入流
FileInputStream input = mContext.openFileInput(filename);
byte[] temp = new byte[1024];
StringBuilder sb = new StringBuilder("");
int len = 0;
//读取文件内容:
while ((len = input.read(temp)) > 0) {
sb.append(new String(temp, 0, len));
}
//关闭输入流
input.close();
return sb.toString();
}
}
打开DDMS 的File Exploer可以看到,在data/data/<包名>/files中有我们写入的文件:
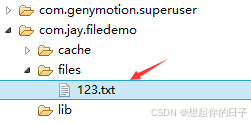
读取SD卡上的文件
在AndroidManifest.xml写上读写SD卡的权限哦!
<!-- 在SDCard中创建与删除文件权限 -->
<uses-permission android:name="android.permission.MOUNT_UNMOUNT_FILESYSTEMS"/>
<!-- 往SDCard写入数据权限 -->
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
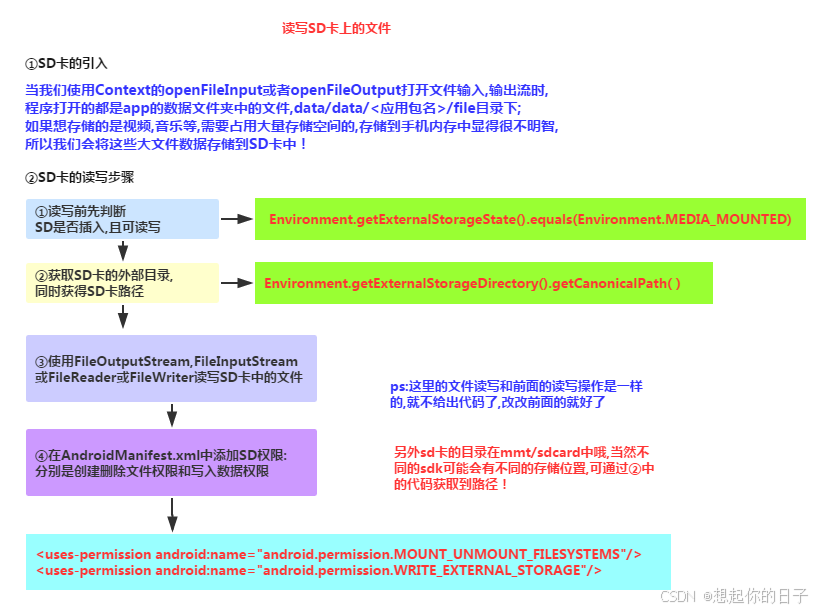
写一个SD操作类: SDFileHelper.java
public class SDFileHelper {
private Context context;
public SDFileHelper() {
}
public SDFileHelper(Context context) {
super();
this.context = context;
}
//往SD卡写入文件的方法
public void savaFileToSD(String filename, String filecontent) throws Exception {
//如果手机已插入sd卡,且app具有读写sd卡的权限
if (Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {
filename = Environment.getExternalStorageDirectory().getCanonicalPath() + "/" + filename;
//这里就不要用openFileOutput了,那个是往手机内存中写数据的
FileOutputStream output = new FileOutputStream(filename);
output.write(filecontent.getBytes());
//将String字符串以字节流的形式写入到输出流中
output.close();
//关闭输出流
} else Toast.makeText(context, "SD卡不存在或者不可读写", Toast.LENGTH_SHORT).show();
}
//读取SD卡中文件的方法
//定义读取文件的方法:
public String readFromSD(String filename) throws IOException {
StringBuilder sb = new StringBuilder("");
if (Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {
filename = Environment.getExternalStorageDirectory().getCanonicalPath() + "/" + filename;
//打开文件输入流
FileInputStream input = new FileInputStream(filename);
byte[] temp = new byte[1024];
int len = 0;
//读取文件内容:
while ((len = input.read(temp)) > 0) {
sb.append(new String(temp, 0, len));
}
//关闭输入流
input.close();
}
return sb.toString();
}
}
在/storage/shell/emilated/0 中有我们写入的文件:
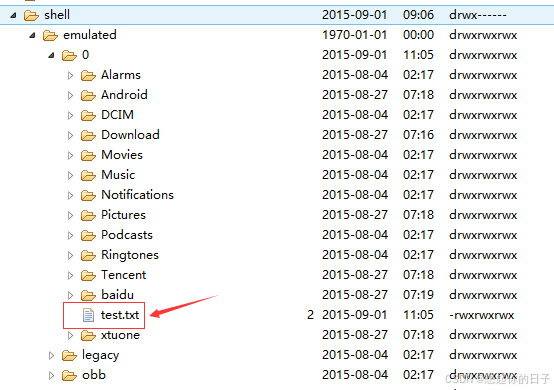
读取raw和assets文件夹下的文件
- res/raw:文件会被映射到R.java文件中,访问的时候直接通过资源ID即可访问,而且 他不能有目录结构,就是不能再创建文件夹
- assets:不会映射到R.java文件中,通过AssetManager来访问,能有目录结构,即, 可以自行创建文件夹
读取文件资源:
res/raw:
InputStream is =getResources().openRawResource(R.raw.filename);
assets:
AssetManager am = getAssets();
InputStream is = am.open("filename");