目录
[2. 顺序表经典算法](#2. 顺序表经典算法)
1.基于动态顺序表实现通讯录项⽬
1.1功能要求
1)⾄少能够存储100个⼈的通讯信息
2)能够保存⽤⼾信息:名字、性别、年龄、电话、地址等
3)增加联系⼈信息
4)删除指定联系⼈
5)查找制定联系⼈
6)修改指定联系⼈
7)显⽰联系⼈信息
1.2代码实现
SeqList.h
cpp
#define _CRT_SECURE_NO_WARNINGS 1
#define _CRT_SECURE_NO_WARNINGS 1
#include <stdio.h>
#include <stdlib.h>
#include <assert.h>
#include "Contact.h"
//定义顺序表的结构
//#define N 100
//
//静态顺序表
//struct Seqlist
//{
// int arr[N];
// int size;
//};
//typedef int SLDataType;//方便定义
typedef peoInfo SLDataType;
//动态顺序表
typedef struct SeqList
{
SLDataType* arr;
int size;
int capacity;
}SL;//(写法一)
//typedef struct SeqList SL;(写法二)
//顺序表初始化
void SLInit(SL* ps);
//顺序表的摧毁
void SLDestory(SL* ps);
//void SLPrint(SL s);
//头部插入删除/尾部插入删除
void SLPushBack(SL* ps, SLDataType x);
void SLPushFront(SL* ps, SLDataType x);
void SLPopBack(SL* ps);
void SLPopFront(SL* ps);
//指定位置之前插入/删除数据
void SLInsert(SL* ps, int pos, SLDataType x);
void SLErase(SL* ps, int pos);
//void SLFind(SL* ps, SLDataType x);
SeqList.c
cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include"SeqList.h"
void SLInit(SL* ps)
{
ps->arr = NULL;
ps->size = ps->capacity = 0;
}
//顺序表的销毁
void SLDestory(SL* ps)
{
if (ps->arr) //等价于 if(ps->arr != NULL)
{
free(ps->arr);
}
ps->arr = NULL;
ps->size = ps->capacity = 0;
}
void SLCheckCapacity(SL* ps)
{
//插入数据之前先看空间够不够
if (ps->capacity == ps->size)
{
//申请空间
//malloc calloc realloc int arr[100] --->增容realloc
//三目表达式
int newCapacity = ps->capacity == 0 ? 4 : 2 * ps->capacity;
SLDataType* tmp = (SLDataType*)realloc(ps->arr, newCapacity * sizeof(SLDataType));//要申请多大的空间
if (tmp == NULL)
{
perror("realloc fail!");
exit(1);//直接退出程序,不再继续执行
}
//空间申请成功
ps->arr = tmp;
ps->capacity = newCapacity;
}
}
//尾插
void SLPushBack(SL* ps, SLDataType x)
{
温柔的解决方式
//if (ps == NULL)
//{
// return;
//}
assert(ps); //等价与assert(ps != NULL)
//ps->arr[ps->size] = x;
//++ps->size;
SLCheckCapacity(ps);
ps->arr[ps->size++] = x;
}
//头插
void SLPushFront(SL* ps, SLDataType x)
{
assert(ps);
SLCheckCapacity(ps);
//先让顺序表中已有的数据整体往后挪动一位
for (int i = ps->size; i > 0; i--)
{
ps->arr[i] = ps->arr[i - 1];//arr[1] = arr[0]
}
ps->arr[0] = x;
ps->size++;
}
//void SLPrint(SL s)
//{
// for (int i = 0; i < s.size; i++)
// {
// printf("%d ", s.arr[i]);
// }
// printf("\n");
//}
void SLPopBack(SL* ps)
{
assert(ps);
assert(ps->size);
//顺序表不为空
//ps->arr[ps->size - 1] = -1;
--ps->size;
}
void SLPopFront(SL* ps)
{
assert(ps);
assert(ps->size);
//数据整体往前挪动一位
for (int i = 0; i < ps->size - 1; i++)
{
ps->arr[i] = ps->arr[i + 1]; //arr[size-2] = arr[size-1]
}
ps->size--;
}
//在指定位置之前插入数据
// 1 2 size = 2
//pos 0 -1 100000
void SLInsert(SL* ps, int pos, SLDataType x)
{
assert(ps);
assert(pos >= 0 && pos <= ps->size);
//插入数据:空间够不够
SLCheckCapacity(ps);
//让pos及之后的数据整体往后挪动一位
for (int i = ps->size; i > pos; i--)
{
ps->arr[i] = ps->arr[i - 1];//arr[pos+1] = arr[pos]
}
ps->arr[pos] = x;
ps->size++;
}
//删除指定位置的数据
void SLErase(SL* ps, int pos)
{
assert(ps);
assert(pos >= 0 && pos < ps->size);
for (int i = pos; i < ps->size - 1; i++)
{
ps->arr[i] = ps->arr[i + 1];
}
ps->size--;
}
//查找
//int SLFind(SL* ps, SLDataType x)
//{
// assert(ps);
// for (int i = 0; i < ps->size; i++)
// {
// if (ps->arr[i] == x)
// {
// //找到啦
// return i;
// }
// }
// //没有找到
// return -1;
//}
Contact.h
cpp
#define _CRT_SECURE_NO_WARNINGS 1
#pragma once
#define NAME_MAX 20
#define GENDER_MAX 10
#define TEL_MAX 20
#define ADDR_MAX 100
//定义联系人数据结构
struct PersonInfo
{
char name[NAME_MAX];
char gender[GENDER_MAX];
int age;
char tel[TEL_MAX];
char addr[ADDR_MAX];
};
typedef struct PersonInfo peoInfo;
typedef struct SeqList Contact;//前置声明
//通讯录的初始化
void ContactInit(Contact* con);
//通讯录的销毁
void ContactDestory(Contact* con);
//通讯录添加数据
void ContactAdd(Contact* con);
//通讯录删除数据
void ContactDel(Contact* con);
//通讯录的修改
void ContactModify(Contact* con);
//通讯录查找
void ContactFind(Contact* con);
//展示通讯录数据
void ContactShow(Contact* con);
Contact.c
cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include"Contact.h"
#include"SeqList.h"
//初始化
void ContactInit(Contact* con)
{
SLInit(con);
}
//通讯录的销毁
void ContactDestory(Contact* con)
{
SLDestory(con);
}
//通讯录添加数据
void ContactAdd(Contact* con)
{
peoInfo info;
printf("请输入添加人姓名:\n");
scanf("%s", info.name);
printf("请输入要添加的联系人性别:\n");
scanf("%s", info.gender);
printf("请输入要添加的联系人年龄:\n");
scanf("%d", &info.age);
printf("请输入要添加的联系人电话:\n");
scanf("%s", info.tel);
printf("请输入要添加的联系人住址:\n");
scanf("%s", info.addr);
SLPushBack(con, info);
}
int FindByName(Contact* con, char name[])
{
for (int i = 0; i < con->size; i++)
{
if (0 == strcmp(con->arr[i].name, name))
{
return i;
}
}
return -1;
}
void ContactDel(Contact* con)
{
char name[NAME_MAX];
printf("请输入要删除的联系人姓名:\n");
scanf("%s", name);
int find = FindByName(con, name);
if (find < 0)
{
printf("要删除的联系人数据不存在!\n");
return;
}
SLErase(con, find);
printf("删除成功!\n");
}
//展示联系人数据
void ContactShow(Contact* con)
{
printf("%s %s %s %s %s\n", "姓名", "性别", "年龄", "电话", "地址");
for (int i = 0; i < con->size; i++)
{
printf("%3s %3s %3d %3s %3s\n", con->arr[i].name, con->arr[i].gender, con->arr[i].age, con->arr[i].tel, con->arr[i].addr);
}
}
//通讯录修改
void ContactModify(Contact* con)
{
char name[NAME_MAX];
printf("请输入要修改的用户名:\n");
scanf("%s", name);
int find = FindByName(con, name);
if (find < 0)
{
printf("要修改的联系人数据不存在!\n");
return;
}
//直接修改
printf("请输入新的姓名:\n");
scanf("%s", con->arr[find].name);
printf("请输入新的性别:\n");
scanf("%s", con->arr[find].gender);
printf("请输入新的年龄:\n");
scanf("%d", &con->arr[find].age);
printf("请输入新的电话:\n");
scanf("%s", con->arr[find].tel);
printf("请输入新的住址:\n");
scanf("%s", con->arr[find].addr);
printf("修改成功!\n");
}
//通讯录查找
void ContactFind(Contact* con)
{
//11
char name[NAME_MAX];
printf("请输入要查找的联系人姓名\n");
scanf("%s", name);
int find = FindByName(con, name);
if (find < 0)
{
printf("要查找的联系人数据不存在!\n");
return;
}
// 姓名 性别 年龄 电话 地址
// 11 11 11 11 11
printf("%s %s %s %s %s\n", "姓名", "性别", "年龄", "电话", "地址");
printf("%3s %3s %3d %3s %3s\n",con->arr[find].name,con->arr[find].gender,con->arr[find].age,con->arr[find].tel,con->arr[find].addr);
}
test.c
cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include"SeqList.h"
void menu()
{
printf("******************通讯录******************\n");
printf("*******1.增加联系人 2.删除联系人********\n");
printf("*******3.修改联系人 4.查找联系人********\n");
printf("*******5.展示联系人 0. 退出 *********\n");
printf("******************************************\n");
}
int main()
{
int op = -1;
Contact con;
ContactInit(&con);
do {
menu();
printf("请选择您的操作:\n");
scanf("%d", &op);
//要根据对应的op执行不同的操作
switch (op)
{
case 1:
ContactAdd(&con);
break;
case 2:
ContactDel(&con);
break;
case 3:
ContactModify(&con);
break;
case 4:
ContactFind(&con);
break;
case 5:
ContactShow(&con);
break;
case 0:
printf("退出通讯录....\n");
break;
default:
printf("输入错误,请重新选择您的操作!\n");
break;
}
} while (op != 0);
ContactDestory(&con);
return 0;
}
2. 顺序表经典算法
经典算法OJ题1:移除元素
经典算法OJ题2:合并两个有序数组
题1:
思路一:(无脑写)
cpp
int removeElement(int* nums, int numsSize, int val) {
int k=0,j=0;
for(int i=0;i<numsSize;i++)
{
if(nums[i]!=val)
{
k++;
nums[j++]=nums[i];
}
}
return k;
}
思路二:(双指针)
创建两个变量:src,dst
1.若src指向的值为val,则src++
2.若src指向的值不为val,nums[dst]=nums[src],dst++,src++
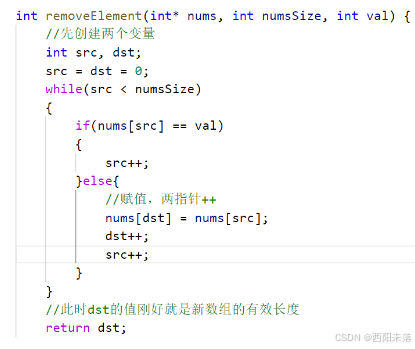
题2:
思路一:
将nums2中数据依次放入到num1数组的后面,然后用排序算法对nums1进行排序
思路二:
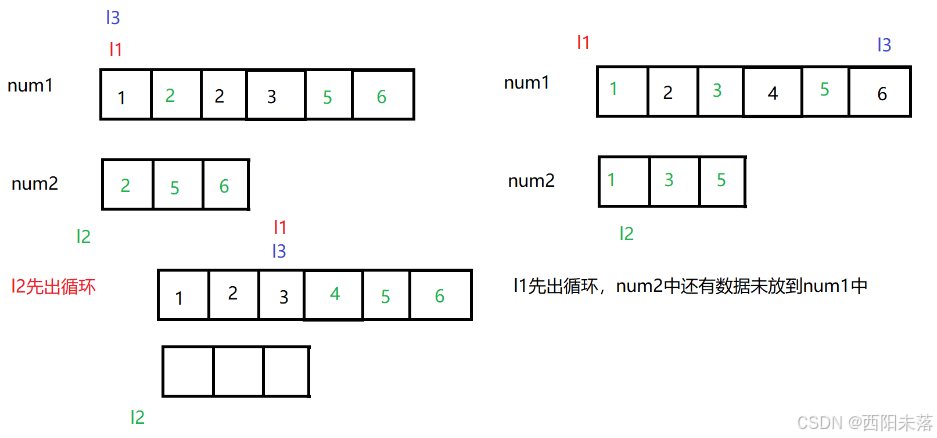
cpp
void merge(int* nums1, int nums1Size, int m, int* nums2, int nums2Size, int n) {
int l1=m-1;
int l2=n-1;
int l3=m+n-1;
while(l1>=0&&l2>=0)
{
if(nums1[l1]<nums2[l2])
{
nums1[l3--]=nums2[l2--];
}
else
nums1[l3--]=nums1[l1--];
}
while(l2>=0)
{
nums1[l3--]=nums2[l2--];
}
}