目录
[一、 RadioButton控件](#一、 RadioButton控件)
前言
在上一篇中,我们讲解了三个常见的控件:TextView控件、Button控件、ImageView控件,那么本篇我们就接着讲剩下的一些常见的界面控件。
一、 RadioButton控件
RadioButton 控件是单选控件,是Button的子类 。每一个按钮都有**"选中"和"未选中** "两种状态。我们可以通过android:checked属性来指定是否选择,true为选中,false为未选中。
RadioButton控件一般搭配RadioGroup使用,RadioGroup是单选复合框,能够容纳多个RadioButton控件,在RadioGroup中不会存在多个RadioButton同时选中的情况。在XML布局文件中的格式:
XML
<RadioGroup
android:属性名="属性值"
.....>
<RadioButton
android:属性名="属性值"
....../>
</RadioGroup>
注意:由于RadioGroup继承于LinearLayoout,所以我们可以指定水平还是竖直布局。
设置RadioGroup的监听事件
我们在设置完XML布局文件之后,可以在java类中来设置RadioGroup的监听事件,哪个RadioButton被点击,就处理被点击控件的点击事件。
通过调用setOnCheckChangeListener() 方法为RadioGroup来设置监听布局内的控件状态是否改变,同时需要重写方法中的onCheackChanged方法,在该方法中来实现我们的功能。
示例:
我们来实现一个选择性别的单选界面,并且当哪个按钮被点击后,要在下方显示用户选择的性别。
XML布局如下:
XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<RadioGroup
android:id="@+id/rg"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<RadioButton
android:id="@+id/male"
android:text="男"
android:textSize="40sp"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<RadioButton
android:id="@+id/female"
android:text="女"
android:textSize="40sp"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</RadioGroup>
<TextView
android:id="@+id/result"
android:text="您的性别是:"
android:textSize="30sp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
定义一个Demo1类
java
import android.os.Bundle;
import android.widget.RadioGroup;
import android.widget.TextView;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
public class Demo1 extends AppCompatActivity implements RadioGroup.OnCheckedChangeListener {
private TextView textView;
private RadioGroup rg;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.radio);
textView = findViewById(R.id.result);
if (textView == null) {
throw new IllegalStateException("Result TextView not found.");
}
rg = findViewById(R.id.rg);
if (rg == null) {
throw new IllegalStateException("RadioGroup not found.");
}
rg.setOnCheckedChangeListener(this);
}
@Override
public void onCheckedChanged(RadioGroup radioGroup, int i) {
if (i==R.id.male){
textView.setText("您的性别是:男");
}else if (i==R.id.female){
textView.setText("您的性别是:女");
}
}
}
运行结果如下:
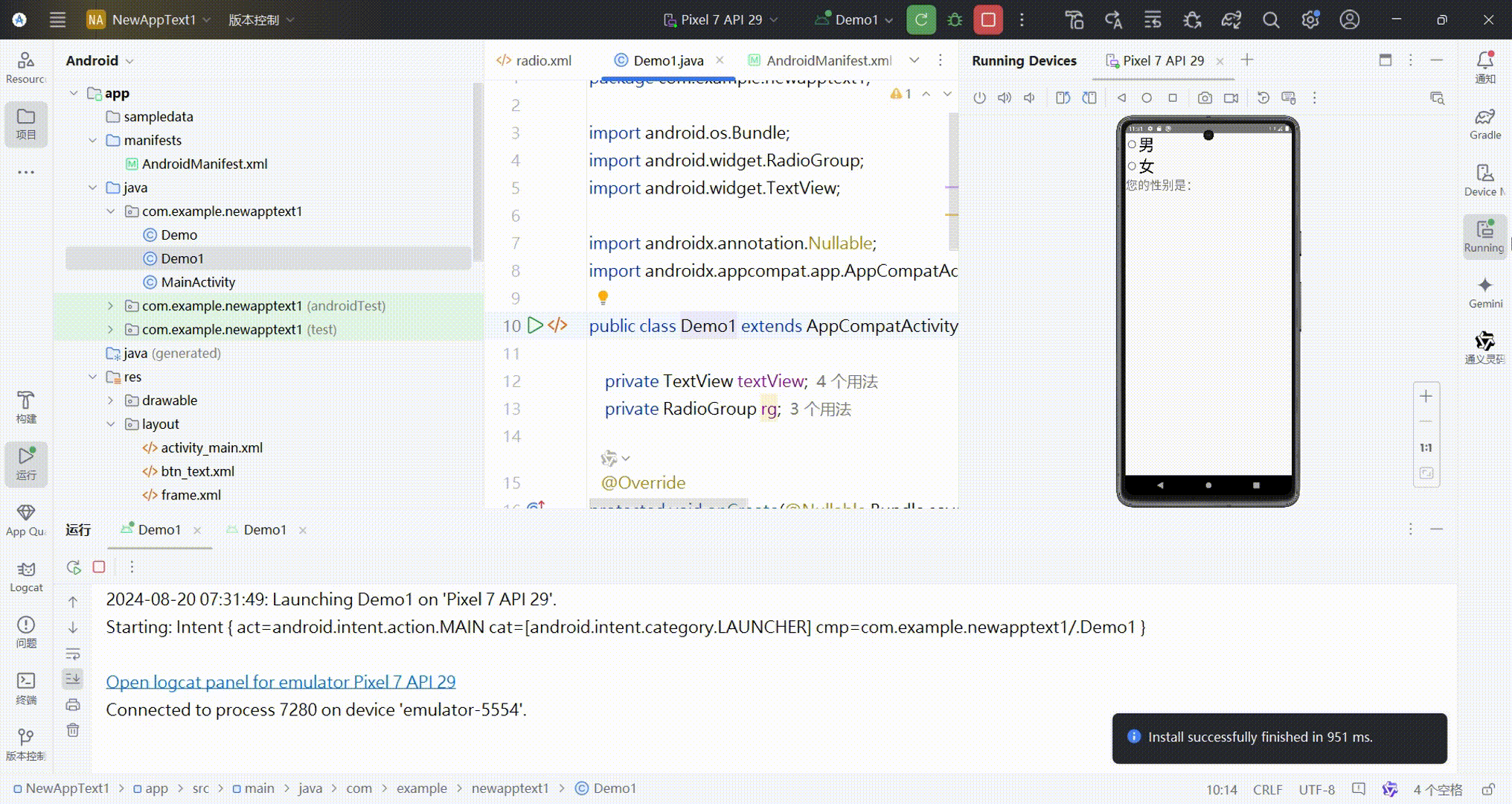
二、CheckBox控件
CheckBox控件表示复选框,是Button的子类,用于实现多选功能 。与单选类似,也有两种状态,也是通过android:checked属性来指定状态。
示例:通过CheckBox来统计用户的兴趣爱好。
设置一个名为check_box的XML布局文件
XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:text="请选择你的兴趣爱好:"
android:textSize="20sp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<CheckBox
android:id="@+id/ball"
android:text="篮球"
android:textSize="20sp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<CheckBox
android:id="@+id/ping"
android:text="乒乓球"
android:textSize="20sp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<CheckBox
android:id="@+id/shuttle"
android:text="羽毛球"
android:textSize="20sp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<TextView
android:text="您的爱好为:"
android:textSize="20sp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/result"
android:textSize="20sp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
设置一个名为CheckActivity的类。
java
import android.os.Bundle;
import android.widget.CheckBox;
import android.widget.CompoundButton;
import android.widget.TextView;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
/**
* CheckActivity 类继承自 AppCompatActivity,并实现了 CompoundButton.OnCheckedChangeListener 接口,
* 用于处理 CheckBox 的选中状态变化事件。
*/
public class CheckActivity extends AppCompatActivity implements CompoundButton.OnCheckedChangeListener {
// 定义一个字符串变量,用于存储用户选择的爱好
private String hobbys;
// 定义一个 TextView 变量,用于显示用户选择的爱好
private TextView hobby;
/**
* 在 onCreate 方法中设置布局和初始化 CheckBox 和 TextView,
* 并为每个 CheckBox 设置监听器,以便在选中状态变化时调用 onCheckedChanged 方法。
*/
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.cheack_box);
CheckBox ball=findViewById(R.id.ball);
CheckBox ping=findViewById(R.id.ping);
CheckBox shuttle=findViewById(R.id.shuttle);
ball.setOnCheckedChangeListener(this);
ping.setOnCheckedChangeListener(this);
shuttle.setOnCheckedChangeListener(this);
hobby = findViewById(R.id.result);
hobbys =new String();
}
/**
* 当 CompoundButton 的选中状态被改变时,这个方法被调用。
*
* @param compoundButton 被改变的 CompoundButton 对象
* @param b 新的选中状态,true 表示选中,false 表示未选中
*/
@Override
public void onCheckedChanged(CompoundButton compoundButton, boolean b) {
String motion=compoundButton.getText().toString();
if(b){
if(!hobbys.contains(motion)){
hobbys=hobbys+motion;
hobby.setText(hobbys);
}
}else{
if(hobbys.contains(motion)){
hobbys=hobbys.replace(motion,"");
hobby.setText(hobbys);
}
}
}
}
当我们将这个类注册之后并运行,就可以得到:
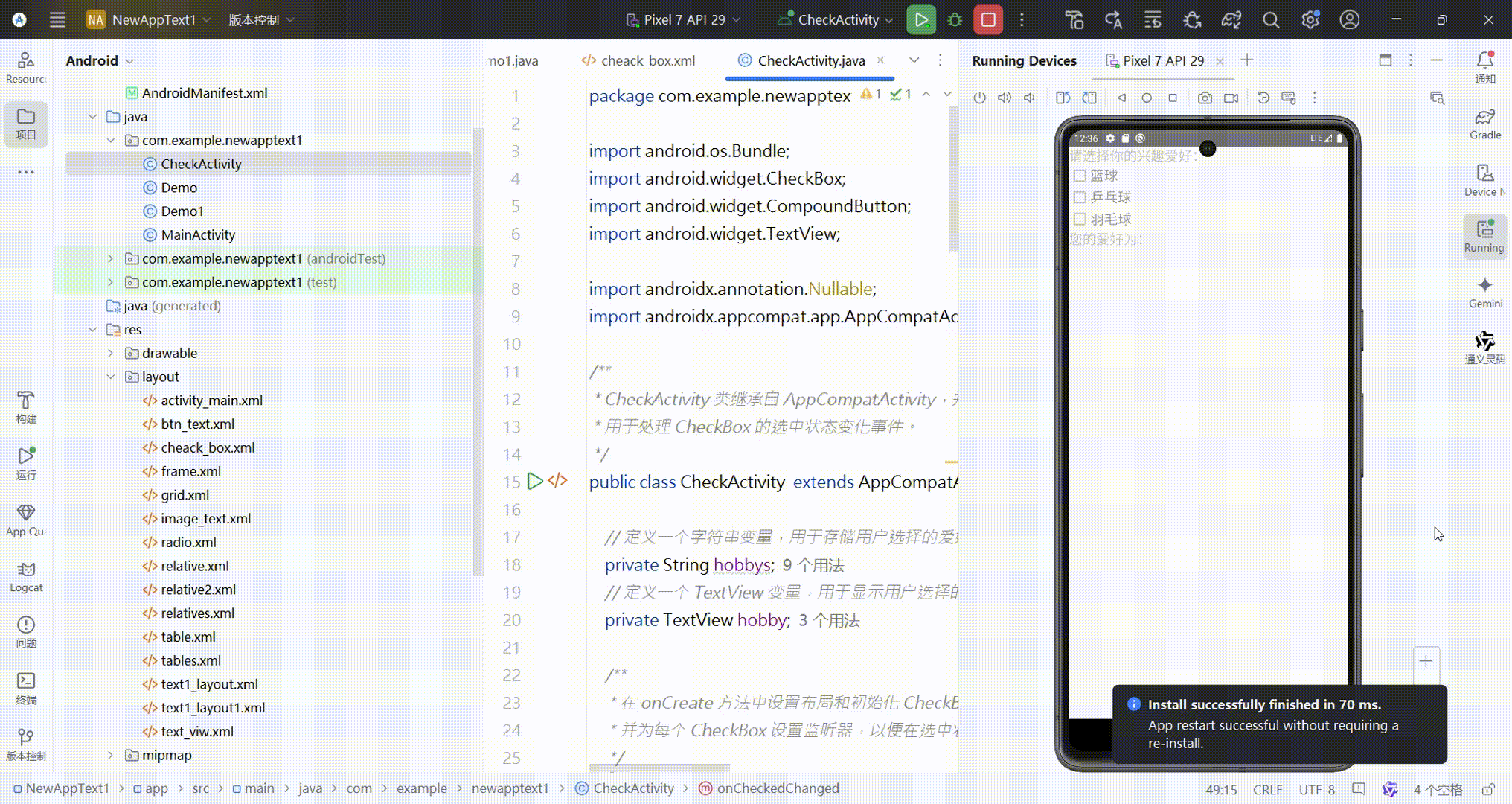
三、Toast类
Toast类是android中提供的一个轻量级信息提醒机制,用于向用户提示即时信息,显示在应用程序界面的最上层,显示一段时间之后就会自动消失不会打断当前操作,也不获得焦点。
想要向用户使用Toast类提示即时信息需要调用其中的makeText()方法设置即时信息,再调用show()方法将提示信息显示到界面上。
java
Toast.makeText(Context,Text.Time).show();
我们来解释一下上面几个参数的含义:
- Context:表示应用程序环境的信息,即当前组件的上下文环境。Context是一个抽象类,如果在Activity中使用Toast类的提示信息,那么该参数可以设置为为"Activity.this"。
- Text:表示提示的字符串信息。
- Time:表示显示信息的时长。有两个属性值:
|------------------|-------|------------------------------|
| 参数名 | 值 | 含义 |
| LENGTH_SHORT | 0 | Toast类显示较短的时间后消失(4000ms) |
| LENGTH_LONG | 1 | Toast类显示较长的时间后消失(7000ms) |
示例:
java
Toast.makeText(this,"请选择你的爱好",Toast.LENGTH_LONG).show();
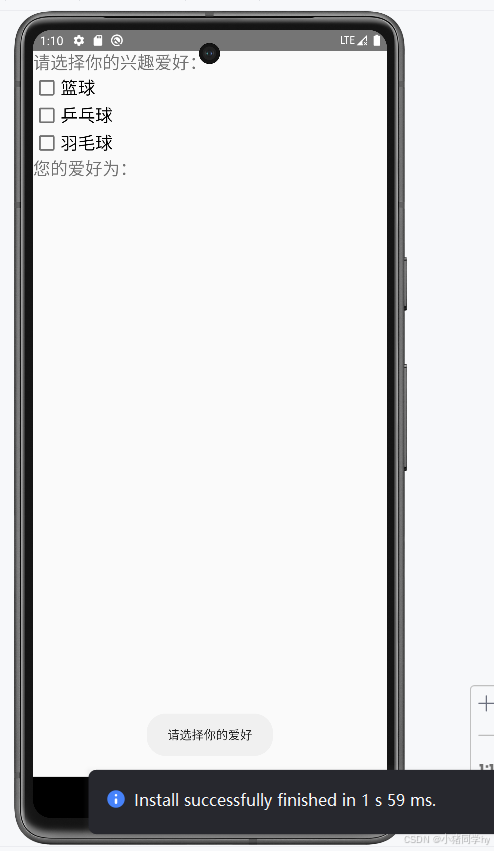
改变Toast窗口的提示位置
如果我们想要改变提示的位置,我们可以使用Toast类中的**setGravity()**方法。
java
public void setGravity(int gravity, int xOffset, int yOffset) {
throw new RuntimeException("Stub!");
}
- gravity :表示具体的位置。可以使用Gravity.CENTER、Gravity.TOP、Gravity.left表示
- xoffset:表示移动的水平位置,若想向右移动,大于0即可。如果是0则不移动。
- yoffset:表示移动的竖直位置。想向下移动,增大参数值即可。
示例:通过三个按钮来显示设置提醒的位置。
设置toast.xml界面布局
XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<Button
android:id="@+id/defaultt"
android:text="点击显示默认位置的位置"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/top"
android:text="点击显示居中上部的位置"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<Button
android:id="@+id/center"
android:text="点击显示居中的位置"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
设置Demo2.java类
java
package com.example.newapptext1;
import static android.widget.Toast.LENGTH_LONG;
import static android.widget.Toast.LENGTH_SHORT;
import android.os.Bundle;
import android.view.Gravity;
import android.view.View;
import android.widget.Toast;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
public class Dmo2 extends AppCompatActivity implements View.OnClickListener {
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.toast);
findViewById(R.id.defaultt).setOnClickListener(this);
findViewById(R.id.top).setOnClickListener(this);
findViewById(R.id.center).setOnClickListener(this);
}
@Override
public void onClick(View view) {
Toast toast;
if(view.getId()==R.id.defaultt){
toast = Toast.makeText(this,"默认",LENGTH_SHORT);
toast.show();
}else if(view.getId()==R.id.top){
toast = Toast.makeText(this,"顶部",LENGTH_SHORT);
toast.setGravity(Gravity.TOP,0,0);
toast.show();
}else if (view.getId()==R.id.center){
toast = Toast.makeText(this,"居中",LENGTH_SHORT);
toast.setGravity(Gravity.CENTER,0,0);
toast.show();
}
}
}
运行结果如下:
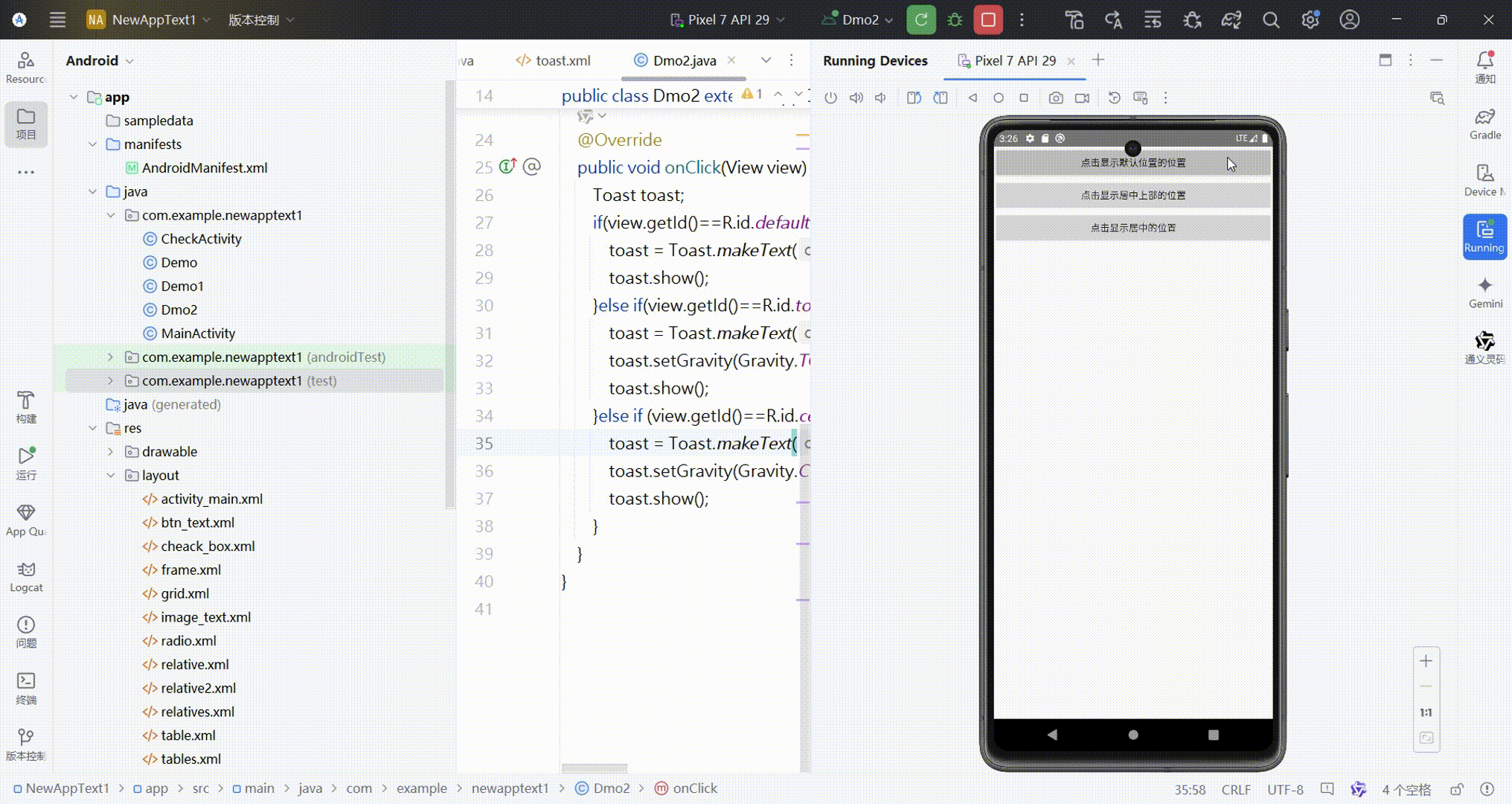
四、EditText控件
EditText控件表示编辑框,是TextView的子类,用户可以在此控件中输入信息。除了支持TextView控件的属性,还具备一些其他常用的属性。
在java中调用:
java
EditText testEditText = (EditText) findViewById(R.id.edittext);
int inputType = InputType.TYPE_CLASS_NUMBER | InputType.TYPE_NUMBER_VARIATION_NORMAL;
testEditText.setInputType(inputType);
常用属性
|--------------------------------|----------------------------------------------|
| 属性 | 说明 |
| android:hint | 控件内容为空时显示的提示文本信息 |
| android:textColorHint | 控件内容为空时显示的提示文本信息的颜色 |
| android:password | 输入文本框中的内容为"." |
| android:phoneNumber | 设置输入文本框的内容只能是数字(过时了) |
| android:minLines | 设置文本的最小行数 |
| android:scrollHorizontally | 设置文本信息超出EditText控件的宽度下是否出现横拉条 |
| android:editable | 设置是否可以编辑 |
| android:inputType | 输入内容的类型(number - 数字;numberDecimal - 浮点数) |
当然,不止有这些属性,还有其他的属性,这里就不细讲。
inputType属性的属性值:
java
Android:inputType="none"----输入普通字符
android:inputType="text"----输入普通字符
android:inputType="textCapCharacters" ----字母大写
android:inputType="textCapWords" ----首字母大写
android:inputType="textCapSentences" ----仅第一个字母大写
android:inputType="textAutoCorrect"---- 自动完成
android:inputType="textAutoComplete" ----自动完成
android:inputType="textMultiLine"---- 多行输入
android:inputType="textImeMultiLine"----输入法多行(如果支持)
android:inputType="textNoSuggestions" ----不提示
android:inputType="textUri" ----网址
android:inputType="textEmailAddress" ----电子邮件地址
android:inputType="textEmailSubject" ----邮件主题
android:inputType="textShortMessage" ----短讯
android:inputType="textLongMessage" ----长信息
android:inputType="textPersonName" ----人名
android:inputType="textPostalAddress" ----地址
android:inputType="textPassword" ----密码
android:inputType="textVisiblePassword" ----可见密码
android:inputType="textWebEditText" ----作为网页表单的文本
android:inputType="textFilter" ----文本筛选过滤
android:inputType="textPhonetic" ----拼音输入
java
<---数值类型->
android:inputType="number" ----数字
android:inputType="numberSigned" ----带符号数字格式
android:inputType="numberDecimal" ----带小数点的浮点格式
android:inputType="phone" ----拨号键盘
android:inputType="datetime"---- 时间日期
android:inputType="date" ----日期键盘
android:inputType="time" ----时间键盘
案例:实现一个程序,在没有输入的时候提示输入用户名和密码还有验证码。在输入密码的时候进行加密操作,那么我们就得利用到inputType,指定为textPassword。
XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:text="登录界面"
android:gravity="center"
android:textSize="20sp"
android:background="@android:color/darker_gray"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<EditText
android:drawableStart="@drawable/user"
android:hint="用户名"
android:textSize="20sp"
android:inputType="text"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<EditText
android:hint="密码"
android:textSize="20sp"
android:inputType="textPassword"
android:drawableStart="@drawable/password"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<EditText
android:hint="验证码"
android:textSize="20sp"
android:drawableStart="@drawable/look"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<Button
android:text="登录"
android:id="@+id/btn"
android:textSize="20sp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
将布局显示到屏幕上,即:
java
import android.os.Bundle;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
public class Text extends AppCompatActivity {
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.edit);
}
}
可以得到:
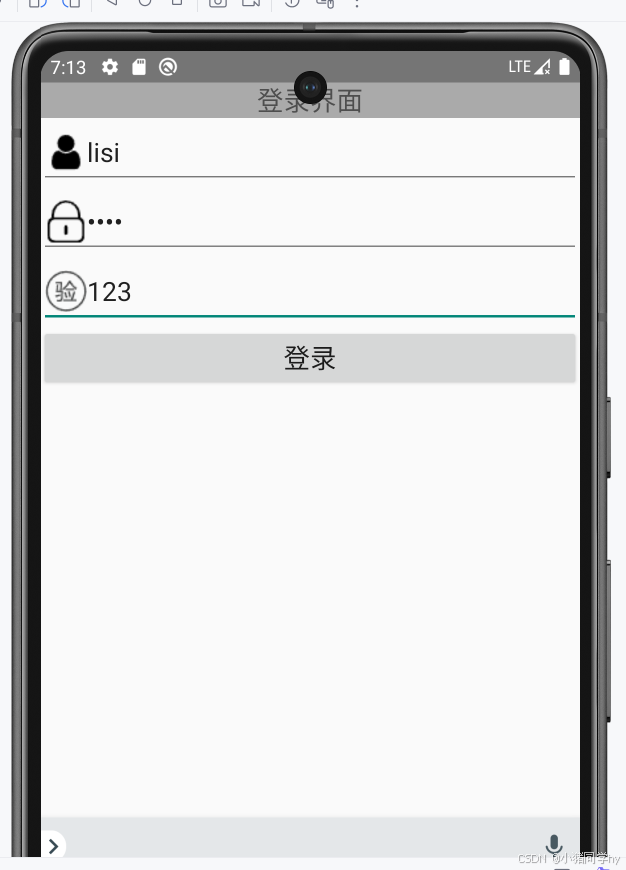
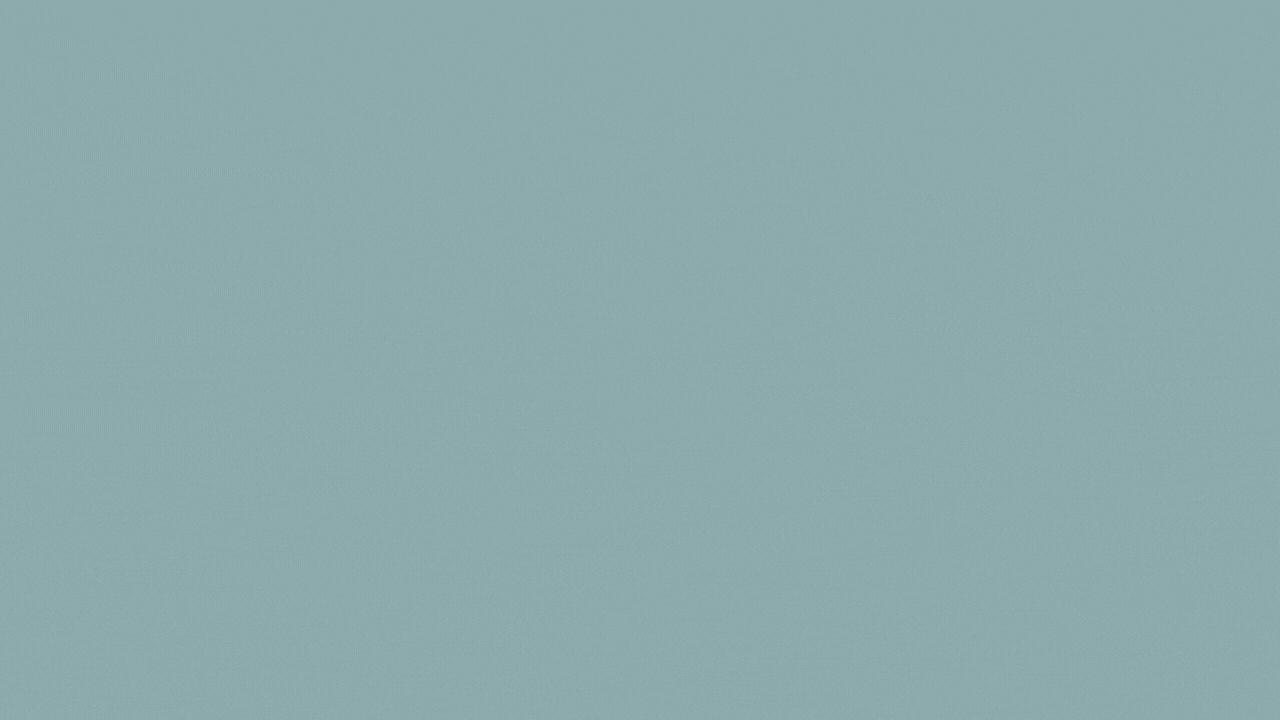
以上就是在Android中一些常见控件,下一篇我们将来实现一下,实现一个登录注册界面!
以上就是本篇所有内容,若有不足,欢迎指正~