你仔细看上面的图片吧
经常有这样的需求吧。
这些列表都是查询出来的。
后端
你的后端必须要有 api 。
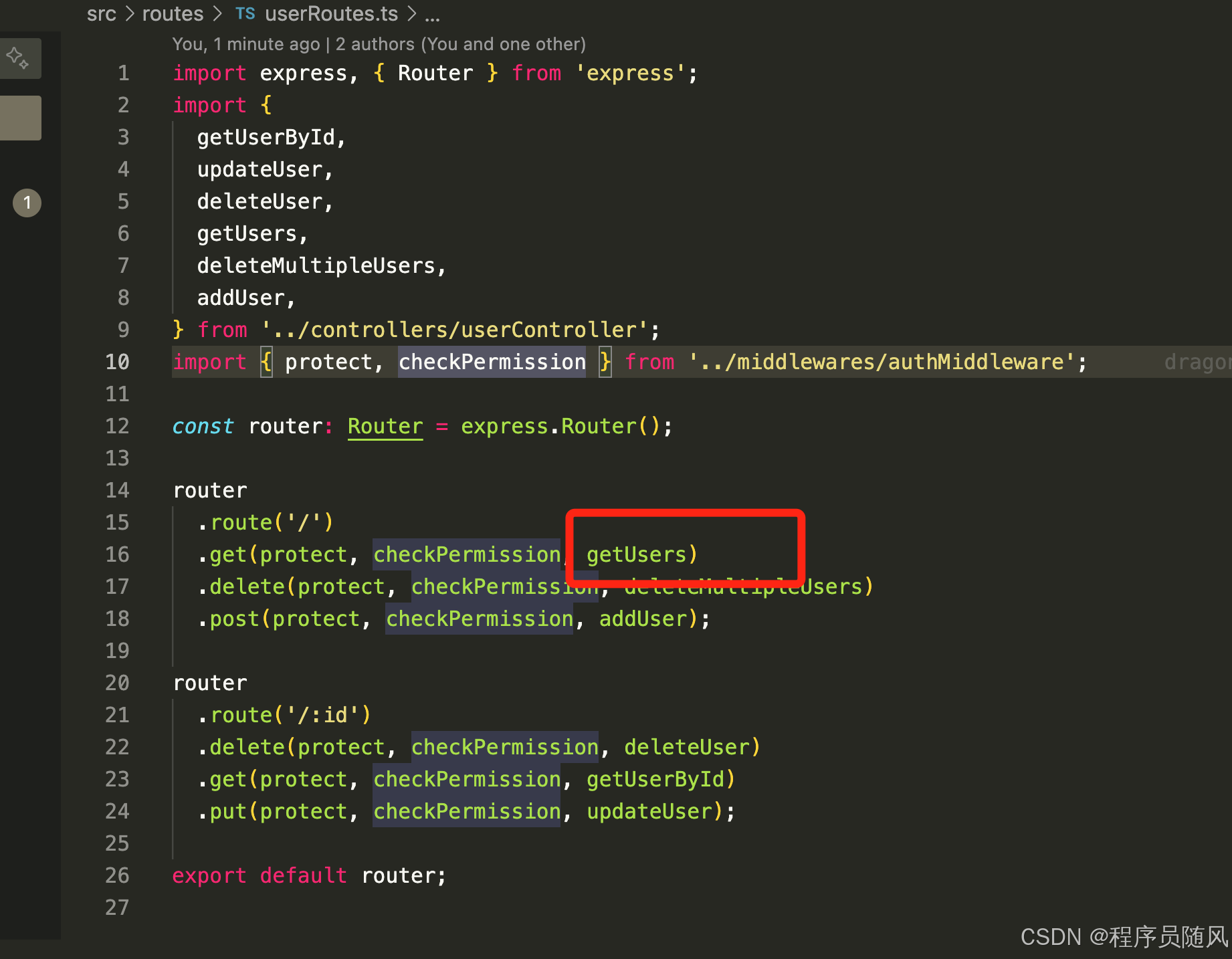
c
const getUsers = handleAsync(async (req: Request, res: Response) => {
const { email, name, live, current = '1', pageSize = '10' } = req.query;
const query: any = {};
if (email) {
query.email = email;
}
if (name) {
query.name = { $regex: name, $options: 'i' };
}
if (live) {
query.live = live === 'true';
}
// 执行查询
const users = await User.find({
...query,
})
.populate('roles')
.sort('-createdAt') // Sort by creation time in descending order
.skip((+current - 1) * +pageSize)
.limit(+pageSize)
.exec();
const total = await User.countDocuments({
...query,
}).exec();
res.json({
success: true,
data: users.map((user) => exclude(user.toObject(), 'password')),
total,
current: +current,
pageSize: +pageSize,
});
});
很简单理解,你只要查询好数据库把列表返回就行
至于分页,你可以跳过的,把每页的数量弄大一些传过来就行。后面会提到。
前端
前端只要把 ProFormSelect 组件放上去
c
import { ProFormSelect } from '@ant-design/pro-components';
import React from 'react';
import { useIntl } from '@umijs/max';
import useQueryList from '@/hooks/useQueryList';
const UserSelect: React.FC = () => {
const intl = useIntl();
const { items: users, loading } = useQueryList('/users');
const filteredUsers = users.filter(
(user: any) =>
user.role !== 'ADMIN' && user.role !== 'ORDER_PLACER' && user.role !== 'REVIEWER',
);
return (
<ProFormSelect
rules={[{ required: true }]}
options={filteredUsers.map((user: any) => ({
label: user.name,
value: user._id,
}))}
width="md"
name="user"
label={intl.formatMessage({ id: 'user' })}
showSearch
fieldProps={{ loading }}
/>
);
};
export default UserSelect;
useQueryList
这里有两个东西要注意
第一
c
const { items: users, loading } = useQueryList('/users');
这个地方主要就是发请求了。
这里做了统一封装
src/hooks/useQueryList.ts
c
import { useEffect, useState } from 'react';
import { queryList } from '@/services/ant-design-pro/api';
const useQueryList = (url: string, hasPermission = true) => {
const [items, setItems] = useState([]);
const [loading, setLoading] = useState(false);
const query = async () => {
setLoading(true);
// Only proceed with the API call if the user has permission
if (hasPermission) {
const response = (await queryList(url, { pageSize: 10000 })) as any;
if (response.success) {
setItems(response.data);
}
}
setLoading(false);
};
useEffect(() => {
query().catch(console.error);
}, [hasPermission]); // Adding `hasPermission` to the dependency array to re-run the effect if it changes
return { items, setItems, loading };
};
export default useQueryList;
loading
fieldProps={{ loading }}
数据没出来前是有个 loading 显示的
跟这里的刚才结合了:
c
const { items: users, loading } = useQueryList('/users');
完结
- ant design pro 如何去保存颜色
- ant design pro v6 如何做好角色管理
- ant design 的 tree 如何作为角色中的权限选择之一
- ant design 的 tree 如何作为角色中的权限选择之二
- ant design pro access.ts 是如何控制多角色的权限的
- ant design pro 中用户的表单如何控制多个角色
- ant design pro 如何实现动态菜单带上 icon 的
- ant design pro 的表分层级如何处理
- ant design pro 如何处理权限管理
- ant design pro 技巧之自制复制到剪贴板组件
- ant design pro 技巧之实现列表页多标签
- 【图文并茂】ant design pro 如何对接登录接口
- 【图文并茂】ant design pro 如何对接后端个人信息接口
- 【图文并茂】ant design pro 如何给后端发送 json web token - 请求拦截器的使用
- 【图文并茂】ant design pro 如何使用 refresh token 可续期 token 来提高用户体验